Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / OpacityConverter.cs / 1305376 / OpacityConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; ////// /// OpacityConverter is a class that can be used to convert /// opacity values from one data type to another. Access this /// class through the TypeDescriptor. /// public class OpacityConverter : TypeConverter { ////// /// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// /// Converts the given object to the converter's native type. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { string text = ((string)value).Replace('%', ' ').Trim(); double val = Double.Parse(text, CultureInfo.CurrentCulture); int indexOfPercent = ((string)value).IndexOf("%"); if (indexOfPercent > 0 && (val >= 0.0 && val <= 1.0)) { val /= 100.0; text = val.ToString(CultureInfo.CurrentCulture); } double percent = 1.0; try { percent = (double)TypeDescriptor.GetConverter(typeof(double)).ConvertFrom(context, culture, text); // assume they meant a percentage if it is > 1.0, else // they actually typed the correct double... // if (percent > 1.0) { percent /= 100.0; } } catch (FormatException e) { throw new FormatException(SR.GetString(SR.InvalidBoundArgument, "Opacity", text, "0%", "100%"), e); } // Now check to see if it is within our bounds. // if (percent < 0.0 || percent > 1.0) { throw new FormatException(SR.GetString(SR.InvalidBoundArgument, "Opacity", text, "0%", "100%")); } return percent; } return base.ConvertFrom(context, culture, value); } ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string)) { double val = (double)value; int perc = (int)(val * 100.0); return perc.ToString(CultureInfo.CurrentCulture) + "%"; } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
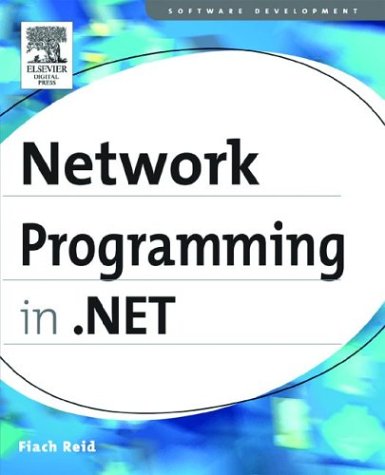
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConvertTextFrag.cs
- _NTAuthentication.cs
- FtpWebRequest.cs
- PrintSchema.cs
- DataGridViewRowPrePaintEventArgs.cs
- OneWayBindingElementImporter.cs
- ButtonAutomationPeer.cs
- SerializationEventsCache.cs
- TimeEnumHelper.cs
- IisTraceWebEventProvider.cs
- XmlDataSourceNodeDescriptor.cs
- UserUseLicenseDictionaryLoader.cs
- _ProxyChain.cs
- MissingSatelliteAssemblyException.cs
- BitmapCacheBrush.cs
- InvokeHandlers.cs
- DataGridViewRowHeaderCell.cs
- URL.cs
- DirtyTextRange.cs
- Form.cs
- Renderer.cs
- Double.cs
- ConcurrentDictionary.cs
- EntityTypeBase.cs
- DeflateStream.cs
- MemberDescriptor.cs
- NavigatingCancelEventArgs.cs
- CollectionCodeDomSerializer.cs
- SecurityTokenInclusionMode.cs
- StoreItemCollection.cs
- HwndKeyboardInputProvider.cs
- Wildcard.cs
- SafeFileHandle.cs
- SubclassTypeValidatorAttribute.cs
- String.cs
- CanonicalFontFamilyReference.cs
- WebRequestModuleElementCollection.cs
- ApplicationBuildProvider.cs
- RelationshipManager.cs
- HttpWriter.cs
- ClientConfigurationHost.cs
- RoutedEvent.cs
- RoutingChannelExtension.cs
- DataGridViewBand.cs
- EventsTab.cs
- GradientBrush.cs
- _HTTPDateParse.cs
- SupportsEventValidationAttribute.cs
- WebBrowserPermission.cs
- MissingSatelliteAssemblyException.cs
- PartialCachingControl.cs
- IconConverter.cs
- DocumentEventArgs.cs
- VisualTreeHelper.cs
- DataTableMapping.cs
- TextModifierScope.cs
- XmlEntityReference.cs
- mediaeventshelper.cs
- TemplateKeyConverter.cs
- RelationshipNavigation.cs
- SpanIndex.cs
- EncoderFallback.cs
- ConfigurationHandlersInstallComponent.cs
- MatchNoneMessageFilter.cs
- ObjectConverter.cs
- ConnectionInterfaceCollection.cs
- MobileUITypeEditor.cs
- WebCategoryAttribute.cs
- BinaryMethodMessage.cs
- HandlerFactoryCache.cs
- ResourceExpression.cs
- ChtmlCommandAdapter.cs
- MDIClient.cs
- SendingRequestEventArgs.cs
- Int32CollectionConverter.cs
- VerticalAlignConverter.cs
- CommandExpr.cs
- ElementNotEnabledException.cs
- OrderedDictionaryStateHelper.cs
- HMACSHA1.cs
- StreamGeometryContext.cs
- DataPager.cs
- CompiledIdentityConstraint.cs
- ActiveXHelper.cs
- BreakRecordTable.cs
- SqlBuilder.cs
- RuleSetDialog.cs
- versioninfo.cs
- future.cs
- SqlDataSourceWizardForm.cs
- HiddenFieldPageStatePersister.cs
- HttpApplicationFactory.cs
- CodeDOMProvider.cs
- mansign.cs
- Matrix3D.cs
- TextEditorContextMenu.cs
- StoragePropertyMapping.cs
- DataTemplateKey.cs
- DropTarget.cs
- ClientUtils.cs