Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Permissions / IsolatedStorageFilePermission.cs / 1305376 / IsolatedStorageFilePermission.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // //[....] // // Purpose : This permission is used to controls/administer access to // IsolatedStorageFile // namespace System.Security.Permissions { using System.Globalization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class IsolatedStorageFilePermission : IsolatedStoragePermission, IBuiltInPermission { public IsolatedStorageFilePermission(PermissionState state) : base(state) { } internal IsolatedStorageFilePermission(IsolatedStorageContainment UsageAllowed, long ExpirationDays, bool PermanentData) : base(UsageAllowed, ExpirationDays, PermanentData) { } //------------------------------------------------------ // // IPERMISSION IMPLEMENTATION // //----------------------------------------------------- public override IPermission Union(IPermission target) { if (target == null) { return this.Copy(); } else if (!VerifyType(target)) { throw new ArgumentException( Environment.GetResourceString("Argument_WrongType", this.GetType().FullName) ); } IsolatedStorageFilePermission operand = (IsolatedStorageFilePermission)target; if (this.IsUnrestricted() || operand.IsUnrestricted()) { return new IsolatedStorageFilePermission( PermissionState.Unrestricted ); } else { IsolatedStorageFilePermission union; union = new IsolatedStorageFilePermission( PermissionState.None ); union.m_userQuota = max(m_userQuota,operand.m_userQuota); union.m_machineQuota = max(m_machineQuota,operand.m_machineQuota); union.m_expirationDays = max(m_expirationDays,operand.m_expirationDays); union.m_permanentData = m_permanentData || operand.m_permanentData; union.m_allowed = (IsolatedStorageContainment)max((long)m_allowed,(long)operand.m_allowed); return union; } } public override bool IsSubsetOf(IPermission target) { if (target == null) { return ((m_userQuota == 0) && (m_machineQuota == 0) && (m_expirationDays == 0) && (m_permanentData == false) && (m_allowed == IsolatedStorageContainment.None)); } try { IsolatedStorageFilePermission operand = (IsolatedStorageFilePermission)target; if (operand.IsUnrestricted()) return true; return ((operand.m_userQuota >= m_userQuota) && (operand.m_machineQuota >= m_machineQuota) && (operand.m_expirationDays >= m_expirationDays) && (operand.m_permanentData || !m_permanentData) && (operand.m_allowed >= m_allowed)); } catch (InvalidCastException) { throw new ArgumentException( Environment.GetResourceString("Argument_WrongType", this.GetType().FullName) ); } } public override IPermission Intersect(IPermission target) { if (target == null) return null; else if (!VerifyType(target)) { throw new ArgumentException( Environment.GetResourceString("Argument_WrongType", this.GetType().FullName) ); } IsolatedStorageFilePermission operand = (IsolatedStorageFilePermission)target; if(operand.IsUnrestricted()) return Copy(); else if(IsUnrestricted()) return target.Copy(); IsolatedStorageFilePermission intersection; intersection = new IsolatedStorageFilePermission( PermissionState.None ); intersection.m_userQuota = min(m_userQuota,operand.m_userQuota); intersection.m_machineQuota = min(m_machineQuota,operand.m_machineQuota); intersection.m_expirationDays = min(m_expirationDays,operand.m_expirationDays); intersection.m_permanentData = m_permanentData && operand.m_permanentData; intersection.m_allowed = (IsolatedStorageContainment)min((long)m_allowed,(long)operand.m_allowed); if ((intersection.m_userQuota == 0) && (intersection.m_machineQuota == 0) && (intersection.m_expirationDays == 0) && (intersection.m_permanentData == false) && (intersection.m_allowed == IsolatedStorageContainment.None)) return null; return intersection; } public override IPermission Copy() { IsolatedStorageFilePermission copy ; copy = new IsolatedStorageFilePermission(PermissionState.Unrestricted); if(!IsUnrestricted()){ copy.m_userQuota = m_userQuota; copy.m_machineQuota = m_machineQuota; copy.m_expirationDays = m_expirationDays; copy.m_permanentData = m_permanentData; copy.m_allowed = m_allowed; } return copy; } ///int IBuiltInPermission.GetTokenIndex() { return IsolatedStorageFilePermission.GetTokenIndex(); } internal static int GetTokenIndex() { return BuiltInPermissionIndex.IsolatedStorageFilePermissionIndex; } //----------------------------------------------------- // // IsolatedStoragePermission OVERRIDES // //----------------------------------------------------- #if FEATURE_CAS_POLICY [System.Runtime.InteropServices.ComVisible(false)] public override SecurityElement ToXml() { return base.ToXml( "System.Security.Permissions.IsolatedStorageFilePermission" ); } #endif // FEATURE_CAS_POLICY } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
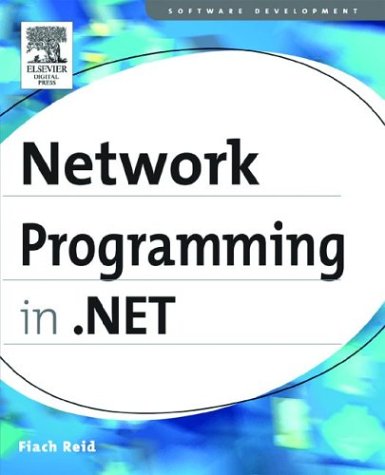
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymbolDocumentGenerator.cs
- ContextQuery.cs
- FlagsAttribute.cs
- ReliableReplySessionChannel.cs
- DbConnectionPoolGroup.cs
- XmlAnyElementAttribute.cs
- PolicyValidationException.cs
- XmlSerializerNamespaces.cs
- ContentElementAutomationPeer.cs
- HostingMessageProperty.cs
- BoundingRectTracker.cs
- WebBrowserSiteBase.cs
- XmlNamespaceMapping.cs
- SecurityKeyIdentifier.cs
- IPEndPointCollection.cs
- DataColumnSelectionConverter.cs
- SchemaComplexType.cs
- TextWriterTraceListener.cs
- ToolboxService.cs
- RoutedEvent.cs
- WebBrowserContainer.cs
- DataSet.cs
- SqlInfoMessageEvent.cs
- ChtmlPageAdapter.cs
- XsdDataContractImporter.cs
- AppDomainFactory.cs
- UnrecognizedPolicyAssertionElement.cs
- XmlSchemaSequence.cs
- Marshal.cs
- RefType.cs
- SinglePhaseEnlistment.cs
- AutomationPattern.cs
- CommandEventArgs.cs
- ImageMapEventArgs.cs
- DictionaryGlobals.cs
- OptimizerPatterns.cs
- OdbcHandle.cs
- MethodRental.cs
- DnsPermission.cs
- DataBoundControlAdapter.cs
- ADConnectionHelper.cs
- MethodRental.cs
- AssemblyAttributesGoHere.cs
- FontClient.cs
- ImmutableAssemblyCacheEntry.cs
- CustomAttributeSerializer.cs
- SqlMethodAttribute.cs
- AxHost.cs
- SubMenuStyle.cs
- ZeroOpNode.cs
- loginstatus.cs
- Brush.cs
- ObjectResult.cs
- SessionStateSection.cs
- DesignerActionPanel.cs
- DataListCommandEventArgs.cs
- PackageRelationship.cs
- WeakEventTable.cs
- ToolStripPanelDesigner.cs
- Funcletizer.cs
- SqlTransaction.cs
- UnsafeNativeMethods.cs
- XamlReaderConstants.cs
- BlurBitmapEffect.cs
- Types.cs
- GlobalEventManager.cs
- PolicyLevel.cs
- XamlBrushSerializer.cs
- ComAdminInterfaces.cs
- LineSegment.cs
- UnauthorizedAccessException.cs
- MorphHelpers.cs
- HyperLinkField.cs
- EventLogEntryCollection.cs
- Ports.cs
- GenericTypeParameterConverter.cs
- AcceleratedTokenProvider.cs
- ItemDragEvent.cs
- Block.cs
- CodeCommentStatementCollection.cs
- DoubleAnimationUsingPath.cs
- MatchAttribute.cs
- XPathParser.cs
- DecimalAnimationUsingKeyFrames.cs
- IRCollection.cs
- CodeRegionDirective.cs
- PropertyDescriptorComparer.cs
- TextBoxAutoCompleteSourceConverter.cs
- OleDbRowUpdatedEvent.cs
- SecurityHeaderLayout.cs
- DBSqlParserColumn.cs
- SchemaDeclBase.cs
- Range.cs
- AuthorizationSection.cs
- BaseTemplatedMobileComponentEditor.cs
- MenuEventArgs.cs
- StreamReader.cs
- HuffCodec.cs
- ToolStripActionList.cs
- WpfKnownMemberInvoker.cs