Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Shapes / ellipse.cs / 1305600 / ellipse.cs
//---------------------------------------------------------------------------- // File: Ellipse.cs // // Description: // Implementation of Ellipse shape element. // // History: // 05/30/02 - [....] - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System.ComponentModel; using System; namespace System.Windows.Shapes { ////// The ellipse shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// ///public sealed class Ellipse : Shape { #region Constructors /// /// Instantiates a new instance of a Ellipse. /// ///public Ellipse() { } // The default stretch mode of Ellipse is Fill static Ellipse() { StretchProperty.OverrideMetadata(typeof(Ellipse), new FrameworkPropertyMetadata(Stretch.Fill)); } #endregion Constructors #region Dynamic Properties // For an Ellipse, RenderedGeometry = defining geometry and GeometryTransform = Identity /// /// The RenderedGeometry property returns the final rendered geometry /// public override Geometry RenderedGeometry { get { // RenderedGeometry = defining geometry return DefiningGeometry; } } ////// Return the transformation applied to the geometry before rendering /// public override Transform GeometryTransform { get { return Transform.Identity; } } #endregion Dynamic Properties #region Protected ////// Updates DesiredSize of the Ellipse. Called by parent UIElement. This is the first pass of layout. /// /// Constraint size is an "upper limit" that Ellipse should not exceed. ///Ellipse's desired size. protected override Size MeasureOverride(Size constraint) { if (Stretch == Stretch.UniformToFill) { double width = constraint.Width; double height = constraint.Height; if (Double.IsInfinity(width) && Double.IsInfinity(height)) { return GetNaturalSize(); } else if (Double.IsInfinity(width) || Double.IsInfinity(height)) { width = Math.Min(width, height); } else { width = Math.Max(width, height); } return new Size(width, width); } return GetNaturalSize(); } ////// Returns the final size of the shape and caches the bounds. /// protected override Size ArrangeOverride(Size finalSize) { // We construct the rectangle to fit finalSize with the appropriate Stretch mode. The rendering // transformation will thus be the identity. double penThickness = GetStrokeThickness(); double margin = penThickness / 2; _rect = new Rect( margin, // X margin, // Y Math.Max(0, finalSize.Width - penThickness), // Width Math.Max(0, finalSize.Height - penThickness)); // Height switch (Stretch) { case Stretch.None: // A 0 Rect.Width and Rect.Height rectangle _rect.Width = _rect.Height = 0; break; case Stretch.Fill: // The most common case: a rectangle that fills the box. // _rect has already been initialized for that. break; case Stretch.Uniform: // The maximal square that fits in the final box if (_rect.Width > _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width <= _rect.Height { _rect.Height = _rect.Width; } break; case Stretch.UniformToFill: // The minimal square that fills the final box if (_rect.Width < _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width >= _rect.Height { _rect.Height = _rect.Width; } break; } ResetRenderedGeometry(); return finalSize; } ////// Get the ellipse that defines this shape /// protected override Geometry DefiningGeometry { get { if (_rect.IsEmpty) { return Geometry.Empty; } return new EllipseGeometry(_rect); } } ////// Render callback. /// protected override void OnRender(DrawingContext drawingContext) { if (!_rect.IsEmpty) { Pen pen = GetPen(); drawingContext.DrawGeometry(Fill, pen, new EllipseGeometry(_rect)); } } #endregion Protected #region Internal Methods internal override void CacheDefiningGeometry() { double margin = GetStrokeThickness() / 2; _rect = new Rect(margin, margin, 0, 0); } ////// Get the natural size of the geometry that defines this shape /// internal override Size GetNaturalSize() { double strokeThickness = GetStrokeThickness(); return new Size(strokeThickness, strokeThickness); } ////// Get the bonds of the rectangle that defines this shape /// internal override Rect GetDefiningGeometryBounds() { return _rect; } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 13; } } #endregion Internal Methods #region Private Fields private Rect _rect = Rect.Empty; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // File: Ellipse.cs // // Description: // Implementation of Ellipse shape element. // // History: // 05/30/02 - [....] - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System.ComponentModel; using System; namespace System.Windows.Shapes { ////// The ellipse shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// ///public sealed class Ellipse : Shape { #region Constructors /// /// Instantiates a new instance of a Ellipse. /// ///public Ellipse() { } // The default stretch mode of Ellipse is Fill static Ellipse() { StretchProperty.OverrideMetadata(typeof(Ellipse), new FrameworkPropertyMetadata(Stretch.Fill)); } #endregion Constructors #region Dynamic Properties // For an Ellipse, RenderedGeometry = defining geometry and GeometryTransform = Identity /// /// The RenderedGeometry property returns the final rendered geometry /// public override Geometry RenderedGeometry { get { // RenderedGeometry = defining geometry return DefiningGeometry; } } ////// Return the transformation applied to the geometry before rendering /// public override Transform GeometryTransform { get { return Transform.Identity; } } #endregion Dynamic Properties #region Protected ////// Updates DesiredSize of the Ellipse. Called by parent UIElement. This is the first pass of layout. /// /// Constraint size is an "upper limit" that Ellipse should not exceed. ///Ellipse's desired size. protected override Size MeasureOverride(Size constraint) { if (Stretch == Stretch.UniformToFill) { double width = constraint.Width; double height = constraint.Height; if (Double.IsInfinity(width) && Double.IsInfinity(height)) { return GetNaturalSize(); } else if (Double.IsInfinity(width) || Double.IsInfinity(height)) { width = Math.Min(width, height); } else { width = Math.Max(width, height); } return new Size(width, width); } return GetNaturalSize(); } ////// Returns the final size of the shape and caches the bounds. /// protected override Size ArrangeOverride(Size finalSize) { // We construct the rectangle to fit finalSize with the appropriate Stretch mode. The rendering // transformation will thus be the identity. double penThickness = GetStrokeThickness(); double margin = penThickness / 2; _rect = new Rect( margin, // X margin, // Y Math.Max(0, finalSize.Width - penThickness), // Width Math.Max(0, finalSize.Height - penThickness)); // Height switch (Stretch) { case Stretch.None: // A 0 Rect.Width and Rect.Height rectangle _rect.Width = _rect.Height = 0; break; case Stretch.Fill: // The most common case: a rectangle that fills the box. // _rect has already been initialized for that. break; case Stretch.Uniform: // The maximal square that fits in the final box if (_rect.Width > _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width <= _rect.Height { _rect.Height = _rect.Width; } break; case Stretch.UniformToFill: // The minimal square that fills the final box if (_rect.Width < _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width >= _rect.Height { _rect.Height = _rect.Width; } break; } ResetRenderedGeometry(); return finalSize; } ////// Get the ellipse that defines this shape /// protected override Geometry DefiningGeometry { get { if (_rect.IsEmpty) { return Geometry.Empty; } return new EllipseGeometry(_rect); } } ////// Render callback. /// protected override void OnRender(DrawingContext drawingContext) { if (!_rect.IsEmpty) { Pen pen = GetPen(); drawingContext.DrawGeometry(Fill, pen, new EllipseGeometry(_rect)); } } #endregion Protected #region Internal Methods internal override void CacheDefiningGeometry() { double margin = GetStrokeThickness() / 2; _rect = new Rect(margin, margin, 0, 0); } ////// Get the natural size of the geometry that defines this shape /// internal override Size GetNaturalSize() { double strokeThickness = GetStrokeThickness(); return new Size(strokeThickness, strokeThickness); } ////// Get the bonds of the rectangle that defines this shape /// internal override Rect GetDefiningGeometryBounds() { return _rect; } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 13; } } #endregion Internal Methods #region Private Fields private Rect _rect = Rect.Empty; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
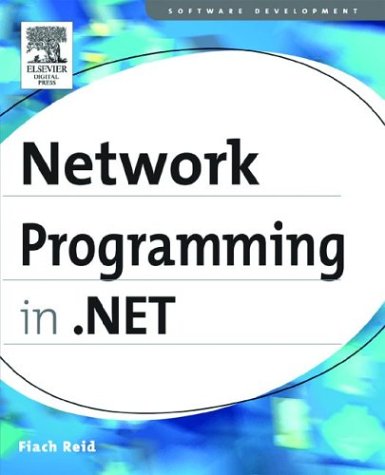
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AutoGeneratedFieldProperties.cs
- SimpleApplicationHost.cs
- XmlSchemaSequence.cs
- XmlArrayAttribute.cs
- WebPartExportVerb.cs
- DataContractAttribute.cs
- X509CertificateClaimSet.cs
- RecordsAffectedEventArgs.cs
- Config.cs
- NullRuntimeConfig.cs
- basevalidator.cs
- X509SecurityToken.cs
- CroppedBitmap.cs
- SqlDataSourceSelectingEventArgs.cs
- ProgressBarAutomationPeer.cs
- Pkcs7Signer.cs
- HttpServerUtilityBase.cs
- XmlSchemaElement.cs
- RefExpr.cs
- DataGridViewCellCancelEventArgs.cs
- SQLResource.cs
- BooleanStorage.cs
- GeneralTransformGroup.cs
- ParameterBuilder.cs
- RelationshipEntry.cs
- SineEase.cs
- SafeLibraryHandle.cs
- CheckBox.cs
- FileStream.cs
- CuspData.cs
- HighContrastHelper.cs
- DetailsViewCommandEventArgs.cs
- PageContentCollection.cs
- LicenseException.cs
- SafeFileMapViewHandle.cs
- XmlSignatureManifest.cs
- SignedInfo.cs
- X509RawDataKeyIdentifierClause.cs
- ObjectResult.cs
- RegexBoyerMoore.cs
- TableCellAutomationPeer.cs
- TypeSchema.cs
- TreeViewDesigner.cs
- RoleService.cs
- CallbackCorrelationInitializer.cs
- VectorAnimationBase.cs
- VideoDrawing.cs
- IBuiltInEvidence.cs
- OrderedDictionaryStateHelper.cs
- TokenizerHelper.cs
- SqlDelegatedTransaction.cs
- CreateBookmarkScope.cs
- ImageCollectionEditor.cs
- CommandField.cs
- StrongTypingException.cs
- CoreSwitches.cs
- CellIdBoolean.cs
- ToolBar.cs
- ClientRuntimeConfig.cs
- ListBoxAutomationPeer.cs
- AutoCompleteStringCollection.cs
- ValueTable.cs
- ClientRolePrincipal.cs
- AdPostCacheSubstitution.cs
- NetworkCredential.cs
- DSACryptoServiceProvider.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- RawStylusInputCustomDataList.cs
- HttpWebRequest.cs
- InProcStateClientManager.cs
- SingleObjectCollection.cs
- Serializer.cs
- MarkupCompilePass1.cs
- TranslateTransform.cs
- ModuleBuilderData.cs
- LocatorBase.cs
- Base64Stream.cs
- SqlDataSourceCommandEventArgs.cs
- ScopelessEnumAttribute.cs
- Image.cs
- WithStatement.cs
- ReflectionUtil.cs
- UDPClient.cs
- ParameterElement.cs
- ObjectCloneHelper.cs
- RequiredFieldValidator.cs
- CellParaClient.cs
- MailWebEventProvider.cs
- RangeValidator.cs
- MobileDeviceCapabilitiesSectionHandler.cs
- PeerDuplexChannelListener.cs
- CachedCompositeFamily.cs
- _SingleItemRequestCache.cs
- QueryResultOp.cs
- EditorPartChrome.cs
- BoolExpression.cs
- ExternalCalls.cs
- XmlExceptionHelper.cs
- DoubleUtil.cs
- StaticSiteMapProvider.cs