Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Effects / Generated / BevelBitmapEffect.cs / 1305600 / BevelBitmapEffect.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Effects { sealed partial class BevelBitmapEffect : BitmapEffect { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new BevelBitmapEffect Clone() { return (BevelBitmapEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new BevelBitmapEffect CloneCurrentValue() { return (BevelBitmapEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void BevelWidthPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { BevelBitmapEffect target = ((BevelBitmapEffect) d); target.PropertyChanged(BevelWidthProperty); } private static void ReliefPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { BevelBitmapEffect target = ((BevelBitmapEffect) d); target.PropertyChanged(ReliefProperty); } private static void LightAnglePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { BevelBitmapEffect target = ((BevelBitmapEffect) d); target.PropertyChanged(LightAngleProperty); } private static void SmoothnessPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { BevelBitmapEffect target = ((BevelBitmapEffect) d); target.PropertyChanged(SmoothnessProperty); } #region Public Properties ////// BevelWidth - double. Default value is 5.0. /// public double BevelWidth { get { return (double) GetValue(BevelWidthProperty); } set { SetValueInternal(BevelWidthProperty, value); } } ////// Relief - double. Default value is 0.3. /// public double Relief { get { return (double) GetValue(ReliefProperty); } set { SetValueInternal(ReliefProperty, value); } } ////// LightAngle - double. Default value is 135.0. /// public double LightAngle { get { return (double) GetValue(LightAngleProperty); } set { SetValueInternal(LightAngleProperty, value); } } ////// Smoothness - double. Default value is 0.2. /// public double Smoothness { get { return (double) GetValue(SmoothnessProperty); } set { SetValueInternal(SmoothnessProperty, value); } } ////// EdgeProfile - EdgeProfile. Default value is EdgeProfile.Linear. /// public EdgeProfile EdgeProfile { get { return (EdgeProfile) GetValue(EdgeProfileProperty); } set { SetValueInternal(EdgeProfileProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new BevelBitmapEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the BevelBitmapEffect.BevelWidth property. /// public static readonly DependencyProperty BevelWidthProperty; ////// The DependencyProperty for the BevelBitmapEffect.Relief property. /// public static readonly DependencyProperty ReliefProperty; ////// The DependencyProperty for the BevelBitmapEffect.LightAngle property. /// public static readonly DependencyProperty LightAngleProperty; ////// The DependencyProperty for the BevelBitmapEffect.Smoothness property. /// public static readonly DependencyProperty SmoothnessProperty; ////// The DependencyProperty for the BevelBitmapEffect.EdgeProfile property. /// public static readonly DependencyProperty EdgeProfileProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_BevelWidth = 5.0; internal const double c_Relief = 0.3; internal const double c_LightAngle = 135.0; internal const double c_Smoothness = 0.2; internal const EdgeProfile c_EdgeProfile = EdgeProfile.Linear; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static BevelBitmapEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(BevelBitmapEffect); BevelWidthProperty = RegisterProperty("BevelWidth", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(BevelWidthPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ReliefProperty = RegisterProperty("Relief", typeof(double), typeofThis, 0.3, new PropertyChangedCallback(ReliefPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); LightAngleProperty = RegisterProperty("LightAngle", typeof(double), typeofThis, 135.0, new PropertyChangedCallback(LightAnglePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); SmoothnessProperty = RegisterProperty("Smoothness", typeof(double), typeofThis, 0.2, new PropertyChangedCallback(SmoothnessPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); EdgeProfileProperty = RegisterProperty("EdgeProfile", typeof(EdgeProfile), typeofThis, EdgeProfile.Linear, null, new ValidateValueCallback(System.Windows.Media.Effects.ValidateEnums.IsEdgeProfileValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Effects { sealed partial class BevelBitmapEffect : BitmapEffect { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new BevelBitmapEffect Clone() { return (BevelBitmapEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new BevelBitmapEffect CloneCurrentValue() { return (BevelBitmapEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void BevelWidthPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { BevelBitmapEffect target = ((BevelBitmapEffect) d); target.PropertyChanged(BevelWidthProperty); } private static void ReliefPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { BevelBitmapEffect target = ((BevelBitmapEffect) d); target.PropertyChanged(ReliefProperty); } private static void LightAnglePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { BevelBitmapEffect target = ((BevelBitmapEffect) d); target.PropertyChanged(LightAngleProperty); } private static void SmoothnessPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { BevelBitmapEffect target = ((BevelBitmapEffect) d); target.PropertyChanged(SmoothnessProperty); } #region Public Properties ////// BevelWidth - double. Default value is 5.0. /// public double BevelWidth { get { return (double) GetValue(BevelWidthProperty); } set { SetValueInternal(BevelWidthProperty, value); } } ////// Relief - double. Default value is 0.3. /// public double Relief { get { return (double) GetValue(ReliefProperty); } set { SetValueInternal(ReliefProperty, value); } } ////// LightAngle - double. Default value is 135.0. /// public double LightAngle { get { return (double) GetValue(LightAngleProperty); } set { SetValueInternal(LightAngleProperty, value); } } ////// Smoothness - double. Default value is 0.2. /// public double Smoothness { get { return (double) GetValue(SmoothnessProperty); } set { SetValueInternal(SmoothnessProperty, value); } } ////// EdgeProfile - EdgeProfile. Default value is EdgeProfile.Linear. /// public EdgeProfile EdgeProfile { get { return (EdgeProfile) GetValue(EdgeProfileProperty); } set { SetValueInternal(EdgeProfileProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new BevelBitmapEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the BevelBitmapEffect.BevelWidth property. /// public static readonly DependencyProperty BevelWidthProperty; ////// The DependencyProperty for the BevelBitmapEffect.Relief property. /// public static readonly DependencyProperty ReliefProperty; ////// The DependencyProperty for the BevelBitmapEffect.LightAngle property. /// public static readonly DependencyProperty LightAngleProperty; ////// The DependencyProperty for the BevelBitmapEffect.Smoothness property. /// public static readonly DependencyProperty SmoothnessProperty; ////// The DependencyProperty for the BevelBitmapEffect.EdgeProfile property. /// public static readonly DependencyProperty EdgeProfileProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_BevelWidth = 5.0; internal const double c_Relief = 0.3; internal const double c_LightAngle = 135.0; internal const double c_Smoothness = 0.2; internal const EdgeProfile c_EdgeProfile = EdgeProfile.Linear; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static BevelBitmapEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(BevelBitmapEffect); BevelWidthProperty = RegisterProperty("BevelWidth", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(BevelWidthPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ReliefProperty = RegisterProperty("Relief", typeof(double), typeofThis, 0.3, new PropertyChangedCallback(ReliefPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); LightAngleProperty = RegisterProperty("LightAngle", typeof(double), typeofThis, 135.0, new PropertyChangedCallback(LightAnglePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); SmoothnessProperty = RegisterProperty("Smoothness", typeof(double), typeofThis, 0.2, new PropertyChangedCallback(SmoothnessPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); EdgeProfileProperty = RegisterProperty("EdgeProfile", typeof(EdgeProfile), typeofThis, EdgeProfile.Linear, null, new ValidateValueCallback(System.Windows.Media.Effects.ValidateEnums.IsEdgeProfileValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
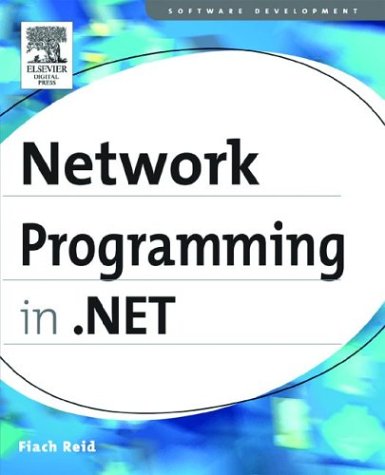
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OrderedHashRepartitionStream.cs
- SQLDateTimeStorage.cs
- LoadRetryStrategyFactory.cs
- XmlReflectionImporter.cs
- Util.cs
- SessionIDManager.cs
- ScaleTransform.cs
- IssuedTokenServiceElement.cs
- AuthStoreRoleProvider.cs
- ListParaClient.cs
- ListItem.cs
- CryptoKeySecurity.cs
- AtomParser.cs
- MultipartIdentifier.cs
- Glyph.cs
- Table.cs
- GridViewEditEventArgs.cs
- UnicastIPAddressInformationCollection.cs
- PanelDesigner.cs
- HandlerFactoryCache.cs
- CryptoSession.cs
- ComponentCache.cs
- WebMessageEncodingElement.cs
- DecimalAnimationUsingKeyFrames.cs
- DateTimeAutomationPeer.cs
- SingleSelectRootGridEntry.cs
- XmlCustomFormatter.cs
- SafeNativeMethods.cs
- BindingCollection.cs
- JsonObjectDataContract.cs
- WindowsAltTab.cs
- InputBinding.cs
- InputElement.cs
- RoutedEventConverter.cs
- filewebrequest.cs
- DataGridViewCellEventArgs.cs
- DynamicMetaObjectBinder.cs
- MatrixKeyFrameCollection.cs
- Utils.cs
- CompiledRegexRunnerFactory.cs
- LassoSelectionBehavior.cs
- HttpModuleAction.cs
- sqlstateclientmanager.cs
- EndpointNameMessageFilter.cs
- BamlRecords.cs
- XmlIlTypeHelper.cs
- GeometryGroup.cs
- NullExtension.cs
- ProviderConnectionPoint.cs
- PartialArray.cs
- EventHandlerList.cs
- EntityDataSourceReferenceGroup.cs
- PointValueSerializer.cs
- OracleEncoding.cs
- ParserStreamGeometryContext.cs
- SettingsContext.cs
- DBCommand.cs
- MultiTrigger.cs
- CodeVariableReferenceExpression.cs
- QuaternionRotation3D.cs
- ComPlusTypeLoader.cs
- BinHexEncoder.cs
- LineGeometry.cs
- XhtmlBasicTextViewAdapter.cs
- Sequence.cs
- ScriptServiceAttribute.cs
- ConfigurationStrings.cs
- TrayIconDesigner.cs
- AdPostCacheSubstitution.cs
- DrawingContextDrawingContextWalker.cs
- WebBrowserEvent.cs
- IgnoreSectionHandler.cs
- SettingsAttributes.cs
- PeerContact.cs
- TreeWalkHelper.cs
- CrossContextChannel.cs
- Int32RectConverter.cs
- AxWrapperGen.cs
- AutoFocusStyle.xaml.cs
- EmbeddedMailObjectsCollection.cs
- ReachObjectContext.cs
- MenuItem.cs
- DesignerOptionService.cs
- FilterException.cs
- DataGridViewCellValueEventArgs.cs
- SafeUserTokenHandle.cs
- CustomTypeDescriptor.cs
- SecurityPermission.cs
- UndoEngine.cs
- DataSourceDesigner.cs
- Int32AnimationUsingKeyFrames.cs
- ConnectionStringsSection.cs
- DataViewManager.cs
- ClientConfigurationSystem.cs
- BitVector32.cs
- ApplicationId.cs
- InternalConfigRoot.cs
- ProfilePropertyNameValidator.cs
- XmlNavigatorFilter.cs
- OperatingSystemVersionCheck.cs