Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / FontStretchConverter.cs / 1305600 / FontStretchConverter.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontStretch type converter. // // History: // 01/25/2005 mleonov - Created. // //--------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// FontStretchConverter class parses a font stretch string. /// public sealed class FontStretchConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFrom - attempt to convert to a FontStretch from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a FontStretch. /// public override object ConvertFrom(ITypeDescriptorContext td, CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } FontStretch fontStretch = new FontStretch(); if (!FontStretches.FontStretchStringToKnownStretch(s, ci, ref fontStretch)) throw new FormatException(SR.Get(SRID.Parsers_IllegalToken)); return fontStretch; } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a FontStretch, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for FontStretch.FromOpenTypeStretch, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is FontStretch) { if (destinationType == typeof(InstanceDescriptor)) { MethodInfo mi = typeof(FontStretch).GetMethod("FromOpenTypeStretch", new Type[]{typeof(int)}); FontStretch c = (FontStretch)value; return new InstanceDescriptor(mi, new object[]{c.ToOpenTypeStretch()}); } else if (destinationType == typeof(string)) { FontStretch c = (FontStretch)value; return ((IFormattable)c).ToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontStretch type converter. // // History: // 01/25/2005 mleonov - Created. // //--------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// FontStretchConverter class parses a font stretch string. /// public sealed class FontStretchConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFrom - attempt to convert to a FontStretch from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a FontStretch. /// public override object ConvertFrom(ITypeDescriptorContext td, CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } FontStretch fontStretch = new FontStretch(); if (!FontStretches.FontStretchStringToKnownStretch(s, ci, ref fontStretch)) throw new FormatException(SR.Get(SRID.Parsers_IllegalToken)); return fontStretch; } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a FontStretch, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for FontStretch.FromOpenTypeStretch, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is FontStretch) { if (destinationType == typeof(InstanceDescriptor)) { MethodInfo mi = typeof(FontStretch).GetMethod("FromOpenTypeStretch", new Type[]{typeof(int)}); FontStretch c = (FontStretch)value; return new InstanceDescriptor(mi, new object[]{c.ToOpenTypeStretch()}); } else if (destinationType == typeof(string)) { FontStretch c = (FontStretch)value; return ((IFormattable)c).ToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
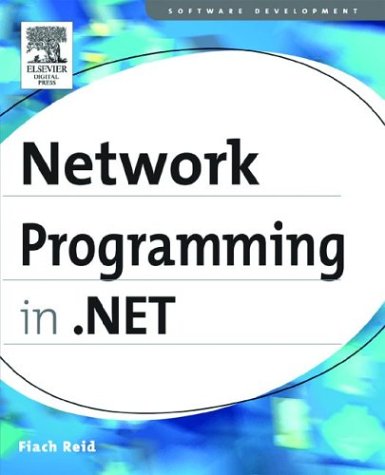
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IndexerNameAttribute.cs
- RelationshipEndCollection.cs
- EraserBehavior.cs
- CodeConstructor.cs
- SlipBehavior.cs
- GCHandleCookieTable.cs
- DesignerRegionCollection.cs
- figurelength.cs
- UrlMappingsModule.cs
- IMembershipProvider.cs
- AccessibleObject.cs
- ChangeNode.cs
- Geometry3D.cs
- XmlUtf8RawTextWriter.cs
- SectionUpdates.cs
- Stylus.cs
- DataGridViewTopLeftHeaderCell.cs
- Logging.cs
- SiteMapNode.cs
- OracleNumber.cs
- XmlTypeMapping.cs
- FrameAutomationPeer.cs
- PageContentCollection.cs
- CrossSiteScriptingValidation.cs
- LinqDataSourceStatusEventArgs.cs
- Invariant.cs
- GPRECT.cs
- ListMarkerLine.cs
- XmlReaderSettings.cs
- DataTemplateSelector.cs
- InstanceHandle.cs
- AjaxFrameworkAssemblyAttribute.cs
- initElementDictionary.cs
- __Filters.cs
- RuleAttributes.cs
- SyndicationContent.cs
- TimersDescriptionAttribute.cs
- DefaultBindingPropertyAttribute.cs
- DragCompletedEventArgs.cs
- SelectionChangedEventArgs.cs
- InputScopeManager.cs
- Axis.cs
- ToolStripRenderer.cs
- Evidence.cs
- ThrowHelper.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- DelegateTypeInfo.cs
- ConfigurationLocationCollection.cs
- DefaultValueMapping.cs
- DataTableMapping.cs
- LicenseException.cs
- EntityReference.cs
- TypeInitializationException.cs
- EditorPartDesigner.cs
- KeyedCollection.cs
- ServiceOperationListItemList.cs
- ConfigDefinitionUpdates.cs
- FocusChangedEventArgs.cs
- DataGridColumn.cs
- URLMembershipCondition.cs
- HtmlContainerControl.cs
- MenuItemCollectionEditor.cs
- CompModSwitches.cs
- ApplicationFileCodeDomTreeGenerator.cs
- VerificationException.cs
- ZipIOExtraField.cs
- DPTypeDescriptorContext.cs
- ByeMessageCD1.cs
- datacache.cs
- ConfigurationPermission.cs
- PageContentCollection.cs
- PackageRelationship.cs
- ObfuscationAttribute.cs
- Publisher.cs
- XmlElement.cs
- GeometryModel3D.cs
- Random.cs
- MetaDataInfo.cs
- securitycriticaldataClass.cs
- RemotingConfigParser.cs
- ColumnMapTranslator.cs
- basecomparevalidator.cs
- ServiceRouteHandler.cs
- SchemaTableOptionalColumn.cs
- ExtendedProtectionPolicyElement.cs
- DbParameterHelper.cs
- TextRangeEditLists.cs
- HebrewNumber.cs
- StreamWithDictionary.cs
- EndpointIdentity.cs
- SimplePropertyEntry.cs
- BrowserCapabilitiesFactory35.cs
- IdentityHolder.cs
- TransactionInterop.cs
- FixedSOMGroup.cs
- mactripleDES.cs
- TextServicesPropertyRanges.cs
- CalculatedColumn.cs
- Identity.cs
- AggregateNode.cs