Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Configuration / RegexWorker.cs / 1305376 / RegexWorker.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Base class for browser capabilities object: just a read-only dictionary * holder that supports Init() * * */ using System.Web.UI; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; using System.Text; using System.Text.RegularExpressions; using System.Web.RegularExpressions; using System.Web.Util; namespace System.Web.Configuration { // public class RegexWorker { internal static readonly Regex RefPat = new BrowserCapsRefRegex(); private Hashtable _groups; private HttpBrowserCapabilities _browserCaps; public RegexWorker(HttpBrowserCapabilities browserCaps) { _browserCaps = browserCaps; } private string Lookup(string from) { MatchCollection matches = RefPat.Matches(from); // shortcut for no reference case if (matches.Count == 0) { return from; } StringBuilder sb = new StringBuilder(); int startIndex = 0; foreach (Match match in matches) { int length = match.Index - startIndex; sb.Append(from.Substring(startIndex, length)); startIndex = match.Index + match.Length; string groupName = match.Groups["name"].Value; string result = null; if (_groups != null) { result = (String)_groups[groupName]; } if (result == null) { result = _browserCaps[groupName]; } sb.Append(result); } sb.Append(from, startIndex, from.Length - startIndex); string output = sb.ToString(); // Return null instead of empty string since empty string is used to override values. if (output.Length == 0) { return null; } return output; } public string this[string key] { get { return Lookup(key); } } public bool ProcessRegex(string target, string regexExpression) { if(target == null) { target = String.Empty; } Regex regex = new Regex(regexExpression, RegexOptions.ExplicitCapture); Match match = regex.Match(target); if(match.Success == false) { return false; } string[] groups = regex.GetGroupNames(); if (groups.Length > 0) { if (_groups == null) { _groups = new Hashtable(); } for (int i = 0; i < groups.Length; i++) { _groups[groups[i]] = match.Groups[i].Value; } } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Base class for browser capabilities object: just a read-only dictionary * holder that supports Init() * * */ using System.Web.UI; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; using System.Text; using System.Text.RegularExpressions; using System.Web.RegularExpressions; using System.Web.Util; namespace System.Web.Configuration { // public class RegexWorker { internal static readonly Regex RefPat = new BrowserCapsRefRegex(); private Hashtable _groups; private HttpBrowserCapabilities _browserCaps; public RegexWorker(HttpBrowserCapabilities browserCaps) { _browserCaps = browserCaps; } private string Lookup(string from) { MatchCollection matches = RefPat.Matches(from); // shortcut for no reference case if (matches.Count == 0) { return from; } StringBuilder sb = new StringBuilder(); int startIndex = 0; foreach (Match match in matches) { int length = match.Index - startIndex; sb.Append(from.Substring(startIndex, length)); startIndex = match.Index + match.Length; string groupName = match.Groups["name"].Value; string result = null; if (_groups != null) { result = (String)_groups[groupName]; } if (result == null) { result = _browserCaps[groupName]; } sb.Append(result); } sb.Append(from, startIndex, from.Length - startIndex); string output = sb.ToString(); // Return null instead of empty string since empty string is used to override values. if (output.Length == 0) { return null; } return output; } public string this[string key] { get { return Lookup(key); } } public bool ProcessRegex(string target, string regexExpression) { if(target == null) { target = String.Empty; } Regex regex = new Regex(regexExpression, RegexOptions.ExplicitCapture); Match match = regex.Match(target); if(match.Success == false) { return false; } string[] groups = regex.GetGroupNames(); if (groups.Length > 0) { if (_groups == null) { _groups = new Hashtable(); } for (int i = 0; i < groups.Length; i++) { _groups[groups[i]] = match.Groups[i].Value; } } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
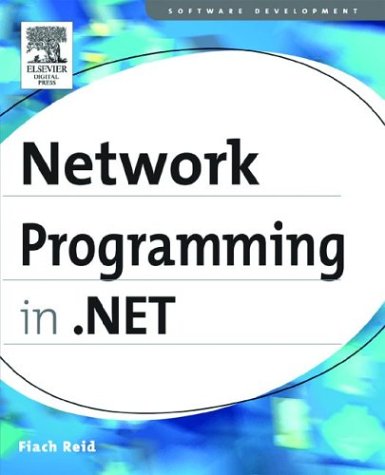
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIElementAutomationPeer.cs
- GridViewSelectEventArgs.cs
- ZipIOExtraField.cs
- HostedHttpRequestAsyncResult.cs
- _NtlmClient.cs
- FileSystemWatcher.cs
- Propagator.JoinPropagator.cs
- HwndSourceKeyboardInputSite.cs
- CompatibleIComparer.cs
- FullTrustAssembly.cs
- SeverityFilter.cs
- RoleGroupCollection.cs
- ServiceOperationInvoker.cs
- BitStack.cs
- ListViewAutomationPeer.cs
- DrawListViewColumnHeaderEventArgs.cs
- DataListItem.cs
- ClickablePoint.cs
- AgileSafeNativeMemoryHandle.cs
- RegexInterpreter.cs
- DuplexChannelBinder.cs
- CryptoHelper.cs
- Span.cs
- CompositionAdorner.cs
- ServiceEndpointCollection.cs
- BridgeDataReader.cs
- DataPagerFieldItem.cs
- OperationBehaviorAttribute.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- SerializationEventsCache.cs
- ParagraphResult.cs
- WebControlsSection.cs
- ExpandedWrapper.cs
- ProcessHostServerConfig.cs
- WorkflowDesigner.cs
- WebBrowsableAttribute.cs
- SessionPageStateSection.cs
- HTMLTagNameToTypeMapper.cs
- GeneralTransform3D.cs
- ConditionalBranch.cs
- FrameworkElementAutomationPeer.cs
- JpegBitmapEncoder.cs
- SspiWrapper.cs
- CustomError.cs
- WindowsAuthenticationEventArgs.cs
- TdsParameterSetter.cs
- TheQuery.cs
- SqlMethodAttribute.cs
- WaitHandleCannotBeOpenedException.cs
- InternalMappingException.cs
- Crypto.cs
- formatter.cs
- StringFormat.cs
- PropertyFilterAttribute.cs
- Operand.cs
- ScrollProviderWrapper.cs
- Set.cs
- HelpEvent.cs
- FlowThrottle.cs
- GridViewActionList.cs
- InvokeMethod.cs
- XmlSerializationReader.cs
- WinEventQueueItem.cs
- WpfWebRequestHelper.cs
- XmlSchemaAnnotation.cs
- RegexParser.cs
- DummyDataSource.cs
- PartBasedPackageProperties.cs
- File.cs
- DataExchangeServiceBinder.cs
- EntityDataSourceColumn.cs
- PagePropertiesChangingEventArgs.cs
- ChannelTraceRecord.cs
- SessionState.cs
- LogicalExpressionTypeConverter.cs
- AVElementHelper.cs
- DataTableNameHandler.cs
- IERequestCache.cs
- EventDescriptorCollection.cs
- RSAOAEPKeyExchangeDeformatter.cs
- SecureUICommand.cs
- Soap12ProtocolReflector.cs
- BaseTreeIterator.cs
- SignerInfo.cs
- InkCanvasInnerCanvas.cs
- ContextStaticAttribute.cs
- XmlNodeChangedEventArgs.cs
- MulticastDelegate.cs
- ImageListDesigner.cs
- PersonalizationProvider.cs
- SettingsPropertyValue.cs
- TranslateTransform.cs
- WaitForChangedResult.cs
- HostSecurityManager.cs
- CallbackDebugBehavior.cs
- QueryOutputWriter.cs
- DataControlFieldHeaderCell.cs
- StrongName.cs
- AppSettingsSection.cs
- InputLanguageCollection.cs