Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / State / SessionState.cs / 1 / SessionState.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HttpSessionState * * Copyright (c) 1998-1999, Microsoft Corporation * */ namespace System.Web.SessionState { using System.Threading; using System.Runtime.InteropServices; using System.Web; using System.Collections; using System.Collections.Specialized; using System.Text; using System.Globalization; using System.Security.Permissions; ////// public enum SessionStateMode { ///[To be supplied.] ////// Off = 0, ///[To be supplied.] ////// InProc = 1, ///[To be supplied.] ////// StateServer = 2, ///[To be supplied.] ////// SQLServer = 3, ///[To be supplied.] ////// Custom = 4 }; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public interface IHttpSessionState { string SessionID { get; } /* * The length of a session before it times out, in minutes. */ ///[To be supplied.] ////// int Timeout { get; set; } /* * Is this a new session? */ ///[To be supplied.] ////// bool IsNewSession { get; } /* * Is session state in a separate process */ ///[To be supplied.] ////// SessionStateMode Mode { get; } /* * Is session state cookieless? */ bool IsCookieless { get; } HttpCookieMode CookieMode { get; } /* * Abandon the session. * */ ///[To be supplied.] ////// void Abandon(); ///[To be supplied.] ////// int LCID { get; set; } ///[To be supplied.] ////// int CodePage { get; set; } ///[To be supplied.] ////// HttpStaticObjectsCollection StaticObjects { get; } ///[To be supplied.] ////// Object this[String name] { get; set; } ///[To be supplied.] ////// Object this[int index] { get; set; } ///[To be supplied.] ////// void Add(String name, Object value); ///[To be supplied.] ////// void Remove(String name); ///[To be supplied.] ////// void RemoveAt(int index); ///[To be supplied.] ////// void Clear(); ///[To be supplied.] ////// void RemoveAll(); ///[To be supplied.] ////// int Count { get; } ///[To be supplied.] ////// NameObjectCollectionBase.KeysCollection Keys { get; } ///[To be supplied.] ////// IEnumerator GetEnumerator(); ///[To be supplied.] ////// void CopyTo(Array array, int index); ///[To be supplied.] ////// Object SyncRoot { get; } ///[To be supplied.] ////// bool IsReadOnly { get; } ///[To be supplied.] ////// bool IsSynchronized { get; } } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpSessionState : ICollection { private IHttpSessionState _container; internal HttpSessionState(IHttpSessionState container) { _container = container; } internal IHttpSessionState Container { get { return _container; } } /* * The Id of the session. */ ///[To be supplied.] ////// public String SessionID { get {return _container.SessionID;} } /* * The length of a session before it times out, in minutes. */ ///[To be supplied.] ////// public int Timeout { get {return _container.Timeout;} set {_container.Timeout = value;} } /* * Is this a new session? */ ///[To be supplied.] ////// public bool IsNewSession { get {return _container.IsNewSession;} } /* * Is session state in a separate process */ ///[To be supplied.] ////// public SessionStateMode Mode { get {return _container.Mode;} } /* * Is session state cookieless? */ public bool IsCookieless { get {return _container.IsCookieless;} } public HttpCookieMode CookieMode { get {return _container.CookieMode; } } /* * Abandon the session. * */ ///[To be supplied.] ////// public void Abandon() { _container.Abandon(); } ///[To be supplied.] ////// public int LCID { get { return _container.LCID; } set { _container.LCID = value; } } ///[To be supplied.] ////// public int CodePage { get { return _container.CodePage; } set { _container.CodePage = value; } } ///[To be supplied.] ////// public HttpSessionState Contents { get {return this;} } ///[To be supplied.] ////// public HttpStaticObjectsCollection StaticObjects { get { return _container.StaticObjects;} } ///[To be supplied.] ////// public Object this[String name] { get { return _container[name]; } set { _container[name] = value; } } ///[To be supplied.] ////// public Object this[int index] { get {return _container[index];} set {_container[index] = value;} } ///[To be supplied.] ////// public void Add(String name, Object value) { _container[name] = value; } ///[To be supplied.] ////// public void Remove(String name) { _container.Remove(name); } ///[To be supplied.] ////// public void RemoveAt(int index) { _container.RemoveAt(index); } ///[To be supplied.] ////// public void Clear() { _container.Clear(); } ///[To be supplied.] ////// public void RemoveAll() { Clear(); } ///[To be supplied.] ////// public int Count { get {return _container.Count;} } ///[To be supplied.] ////// public NameObjectCollectionBase.KeysCollection Keys { get {return _container.Keys;} } ///[To be supplied.] ////// public IEnumerator GetEnumerator() { return _container.GetEnumerator(); } ///[To be supplied.] ////// public void CopyTo(Array array, int index) { _container.CopyTo(array, index); } ///[To be supplied.] ////// public Object SyncRoot { get { return _container.SyncRoot;} } ///[To be supplied.] ////// public bool IsReadOnly { get { return _container.IsReadOnly;} } ///[To be supplied.] ////// public bool IsSynchronized { get { return _container.IsSynchronized;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HttpSessionState * * Copyright (c) 1998-1999, Microsoft Corporation * */ namespace System.Web.SessionState { using System.Threading; using System.Runtime.InteropServices; using System.Web; using System.Collections; using System.Collections.Specialized; using System.Text; using System.Globalization; using System.Security.Permissions; ////// public enum SessionStateMode { ///[To be supplied.] ////// Off = 0, ///[To be supplied.] ////// InProc = 1, ///[To be supplied.] ////// StateServer = 2, ///[To be supplied.] ////// SQLServer = 3, ///[To be supplied.] ////// Custom = 4 }; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public interface IHttpSessionState { string SessionID { get; } /* * The length of a session before it times out, in minutes. */ ///[To be supplied.] ////// int Timeout { get; set; } /* * Is this a new session? */ ///[To be supplied.] ////// bool IsNewSession { get; } /* * Is session state in a separate process */ ///[To be supplied.] ////// SessionStateMode Mode { get; } /* * Is session state cookieless? */ bool IsCookieless { get; } HttpCookieMode CookieMode { get; } /* * Abandon the session. * */ ///[To be supplied.] ////// void Abandon(); ///[To be supplied.] ////// int LCID { get; set; } ///[To be supplied.] ////// int CodePage { get; set; } ///[To be supplied.] ////// HttpStaticObjectsCollection StaticObjects { get; } ///[To be supplied.] ////// Object this[String name] { get; set; } ///[To be supplied.] ////// Object this[int index] { get; set; } ///[To be supplied.] ////// void Add(String name, Object value); ///[To be supplied.] ////// void Remove(String name); ///[To be supplied.] ////// void RemoveAt(int index); ///[To be supplied.] ////// void Clear(); ///[To be supplied.] ////// void RemoveAll(); ///[To be supplied.] ////// int Count { get; } ///[To be supplied.] ////// NameObjectCollectionBase.KeysCollection Keys { get; } ///[To be supplied.] ////// IEnumerator GetEnumerator(); ///[To be supplied.] ////// void CopyTo(Array array, int index); ///[To be supplied.] ////// Object SyncRoot { get; } ///[To be supplied.] ////// bool IsReadOnly { get; } ///[To be supplied.] ////// bool IsSynchronized { get; } } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpSessionState : ICollection { private IHttpSessionState _container; internal HttpSessionState(IHttpSessionState container) { _container = container; } internal IHttpSessionState Container { get { return _container; } } /* * The Id of the session. */ ///[To be supplied.] ////// public String SessionID { get {return _container.SessionID;} } /* * The length of a session before it times out, in minutes. */ ///[To be supplied.] ////// public int Timeout { get {return _container.Timeout;} set {_container.Timeout = value;} } /* * Is this a new session? */ ///[To be supplied.] ////// public bool IsNewSession { get {return _container.IsNewSession;} } /* * Is session state in a separate process */ ///[To be supplied.] ////// public SessionStateMode Mode { get {return _container.Mode;} } /* * Is session state cookieless? */ public bool IsCookieless { get {return _container.IsCookieless;} } public HttpCookieMode CookieMode { get {return _container.CookieMode; } } /* * Abandon the session. * */ ///[To be supplied.] ////// public void Abandon() { _container.Abandon(); } ///[To be supplied.] ////// public int LCID { get { return _container.LCID; } set { _container.LCID = value; } } ///[To be supplied.] ////// public int CodePage { get { return _container.CodePage; } set { _container.CodePage = value; } } ///[To be supplied.] ////// public HttpSessionState Contents { get {return this;} } ///[To be supplied.] ////// public HttpStaticObjectsCollection StaticObjects { get { return _container.StaticObjects;} } ///[To be supplied.] ////// public Object this[String name] { get { return _container[name]; } set { _container[name] = value; } } ///[To be supplied.] ////// public Object this[int index] { get {return _container[index];} set {_container[index] = value;} } ///[To be supplied.] ////// public void Add(String name, Object value) { _container[name] = value; } ///[To be supplied.] ////// public void Remove(String name) { _container.Remove(name); } ///[To be supplied.] ////// public void RemoveAt(int index) { _container.RemoveAt(index); } ///[To be supplied.] ////// public void Clear() { _container.Clear(); } ///[To be supplied.] ////// public void RemoveAll() { Clear(); } ///[To be supplied.] ////// public int Count { get {return _container.Count;} } ///[To be supplied.] ////// public NameObjectCollectionBase.KeysCollection Keys { get {return _container.Keys;} } ///[To be supplied.] ////// public IEnumerator GetEnumerator() { return _container.GetEnumerator(); } ///[To be supplied.] ////// public void CopyTo(Array array, int index) { _container.CopyTo(array, index); } ///[To be supplied.] ////// public Object SyncRoot { get { return _container.SyncRoot;} } ///[To be supplied.] ////// public bool IsReadOnly { get { return _container.IsReadOnly;} } ///[To be supplied.] ////// public bool IsSynchronized { get { return _container.IsSynchronized;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
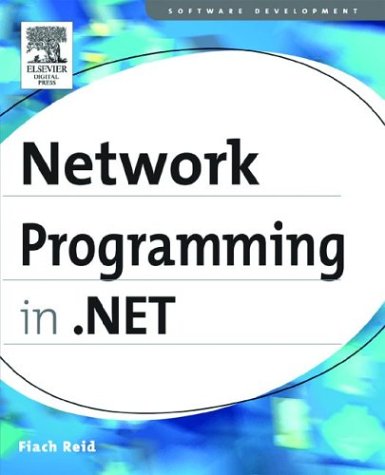
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StrokeNodeOperations.cs
- ToolStripItemClickedEventArgs.cs
- CommentAction.cs
- ParsedAttributeCollection.cs
- PreProcessor.cs
- BaseServiceProvider.cs
- PersistNameAttribute.cs
- EventToken.cs
- MinimizableAttributeTypeConverter.cs
- HttpStreamXmlDictionaryWriter.cs
- XPathExpr.cs
- TextElementCollectionHelper.cs
- RpcResponse.cs
- CodeDirectoryCompiler.cs
- LinqDataSource.cs
- remotingproxy.cs
- ClassData.cs
- PlacementWorkspace.cs
- PreviewKeyDownEventArgs.cs
- XamlRtfConverter.cs
- SqlUDTStorage.cs
- Highlights.cs
- HttpValueCollection.cs
- RoutedEventConverter.cs
- Page.cs
- CustomCategoryAttribute.cs
- FormatException.cs
- XmlDocumentType.cs
- xmlglyphRunInfo.cs
- FieldToken.cs
- XPathSelectionIterator.cs
- RunWorkerCompletedEventArgs.cs
- PerformanceCounterLib.cs
- Cursor.cs
- WebScriptMetadataMessageEncoderFactory.cs
- BaseValidator.cs
- TreeView.cs
- XpsFilter.cs
- EdmEntityTypeAttribute.cs
- ZipIOBlockManager.cs
- Nullable.cs
- ProcessModelInfo.cs
- ConnectionManagementSection.cs
- NativeMethods.cs
- GridViewPageEventArgs.cs
- XPathNavigatorReader.cs
- Privilege.cs
- MailBnfHelper.cs
- SortDescriptionCollection.cs
- SafeNativeMethods.cs
- WebBrowserPermission.cs
- DefaultBinder.cs
- StateDesigner.CommentLayoutGlyph.cs
- StructuralCache.cs
- PrinterSettings.cs
- HtmlInputControl.cs
- EmbeddedMailObject.cs
- OperatingSystem.cs
- JsonFormatReaderGenerator.cs
- DodSequenceMerge.cs
- LabelTarget.cs
- ComponentResourceKeyConverter.cs
- OrderByBuilder.cs
- Stackframe.cs
- CryptoApi.cs
- MetadataCollection.cs
- GPRECTF.cs
- SiteMapPath.cs
- VirtualPathUtility.cs
- ExplicitDiscriminatorMap.cs
- SHA1Managed.cs
- EditorReuseAttribute.cs
- ListView.cs
- UniqueIdentifierService.cs
- DependencyPropertyDescriptor.cs
- Guid.cs
- CngUIPolicy.cs
- XmlILStorageConverter.cs
- CFStream.cs
- DispatchProxy.cs
- TreeViewHitTestInfo.cs
- OpCodes.cs
- _SingleItemRequestCache.cs
- RepeatBehaviorConverter.cs
- WaitForChangedResult.cs
- wgx_sdk_version.cs
- SecurityTimestamp.cs
- SerializationInfoEnumerator.cs
- ListBoxItemWrapperAutomationPeer.cs
- ValueTypeFixupInfo.cs
- SubqueryRules.cs
- ControlIdConverter.cs
- EncryptedPackageFilter.cs
- SQLDoubleStorage.cs
- KnownTypesProvider.cs
- ColorTransformHelper.cs
- PassportAuthenticationModule.cs
- EntryPointNotFoundException.cs
- CodeGeneratorAttribute.cs
- WebPartConnectionsEventArgs.cs