Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Monitoring / system / Diagnosticts / CounterSample.cs / 1305376 / CounterSample.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics { using System.Diagnostics; using System; ////// A struct holding the raw data for a performance counter. /// public struct CounterSample { private long rawValue; private long baseValue; private long timeStamp; private long counterFrequency; private PerformanceCounterType counterType; private long timeStamp100nSec; private long systemFrequency; private long counterTimeStamp; // Dummy holder for an empty sample ////// public static CounterSample Empty = new CounterSample(0, 0, 0, 0, 0, 0, PerformanceCounterType.NumberOfItems32); ///[To be supplied.] ////// public CounterSample(long rawValue, long baseValue, long counterFrequency, long systemFrequency, long timeStamp, long timeStamp100nSec, PerformanceCounterType counterType) { this.rawValue = rawValue; this.baseValue = baseValue; this.timeStamp = timeStamp; this.counterFrequency = counterFrequency; this.counterType = counterType; this.timeStamp100nSec = timeStamp100nSec; this.systemFrequency = systemFrequency; this.counterTimeStamp = 0; } ///[To be supplied.] ////// public CounterSample(long rawValue, long baseValue, long counterFrequency, long systemFrequency, long timeStamp, long timeStamp100nSec, PerformanceCounterType counterType, long counterTimeStamp) { this.rawValue = rawValue; this.baseValue = baseValue; this.timeStamp = timeStamp; this.counterFrequency = counterFrequency; this.counterType = counterType; this.timeStamp100nSec = timeStamp100nSec; this.systemFrequency = systemFrequency; this.counterTimeStamp = counterTimeStamp; } ///[To be supplied.] ////// Raw value of the counter. /// public long RawValue { get { return this.rawValue; } } internal ulong UnsignedRawValue { get { return (ulong)this.rawValue; } } ////// Optional base raw value for the counter (only used if multiple counter based). /// public long BaseValue { get { return this.baseValue; } } ////// Raw system frequency /// public long SystemFrequency { get { return this.systemFrequency; } } ////// Raw counter frequency /// public long CounterFrequency { get { return this.counterFrequency; } } ////// Raw counter frequency /// public long CounterTimeStamp { get { return this.counterTimeStamp; } } ////// Raw timestamp /// public long TimeStamp { get { return this.timeStamp; } } ////// Raw high fidelity timestamp /// public long TimeStamp100nSec { get { return this.timeStamp100nSec; } } ////// Counter type /// public PerformanceCounterType CounterType { get { return this.counterType; } } ////// Static functions to calculate the performance value off the sample /// public static float Calculate(CounterSample counterSample) { return CounterSampleCalculator.ComputeCounterValue(counterSample); } ////// Static functions to calculate the performance value off the samples /// public static float Calculate(CounterSample counterSample, CounterSample nextCounterSample) { return CounterSampleCalculator.ComputeCounterValue(counterSample, nextCounterSample); } public override bool Equals(Object o) { return ( o is CounterSample) && Equals((CounterSample)o); } public bool Equals(CounterSample sample) { return (rawValue == sample.rawValue) && (baseValue == sample.baseValue) && (timeStamp == sample.timeStamp) && (counterFrequency == sample.counterFrequency) && (counterType == sample.counterType) && (timeStamp100nSec == sample.timeStamp100nSec) && (systemFrequency == sample.systemFrequency) && (counterTimeStamp == sample.counterTimeStamp); } public override int GetHashCode() { return rawValue.GetHashCode(); } public static bool operator ==(CounterSample a, CounterSample b) { return a.Equals(b); } public static bool operator !=(CounterSample a, CounterSample b) { return !(a.Equals(b)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics { using System.Diagnostics; using System; ////// A struct holding the raw data for a performance counter. /// public struct CounterSample { private long rawValue; private long baseValue; private long timeStamp; private long counterFrequency; private PerformanceCounterType counterType; private long timeStamp100nSec; private long systemFrequency; private long counterTimeStamp; // Dummy holder for an empty sample ////// public static CounterSample Empty = new CounterSample(0, 0, 0, 0, 0, 0, PerformanceCounterType.NumberOfItems32); ///[To be supplied.] ////// public CounterSample(long rawValue, long baseValue, long counterFrequency, long systemFrequency, long timeStamp, long timeStamp100nSec, PerformanceCounterType counterType) { this.rawValue = rawValue; this.baseValue = baseValue; this.timeStamp = timeStamp; this.counterFrequency = counterFrequency; this.counterType = counterType; this.timeStamp100nSec = timeStamp100nSec; this.systemFrequency = systemFrequency; this.counterTimeStamp = 0; } ///[To be supplied.] ////// public CounterSample(long rawValue, long baseValue, long counterFrequency, long systemFrequency, long timeStamp, long timeStamp100nSec, PerformanceCounterType counterType, long counterTimeStamp) { this.rawValue = rawValue; this.baseValue = baseValue; this.timeStamp = timeStamp; this.counterFrequency = counterFrequency; this.counterType = counterType; this.timeStamp100nSec = timeStamp100nSec; this.systemFrequency = systemFrequency; this.counterTimeStamp = counterTimeStamp; } ///[To be supplied.] ////// Raw value of the counter. /// public long RawValue { get { return this.rawValue; } } internal ulong UnsignedRawValue { get { return (ulong)this.rawValue; } } ////// Optional base raw value for the counter (only used if multiple counter based). /// public long BaseValue { get { return this.baseValue; } } ////// Raw system frequency /// public long SystemFrequency { get { return this.systemFrequency; } } ////// Raw counter frequency /// public long CounterFrequency { get { return this.counterFrequency; } } ////// Raw counter frequency /// public long CounterTimeStamp { get { return this.counterTimeStamp; } } ////// Raw timestamp /// public long TimeStamp { get { return this.timeStamp; } } ////// Raw high fidelity timestamp /// public long TimeStamp100nSec { get { return this.timeStamp100nSec; } } ////// Counter type /// public PerformanceCounterType CounterType { get { return this.counterType; } } ////// Static functions to calculate the performance value off the sample /// public static float Calculate(CounterSample counterSample) { return CounterSampleCalculator.ComputeCounterValue(counterSample); } ////// Static functions to calculate the performance value off the samples /// public static float Calculate(CounterSample counterSample, CounterSample nextCounterSample) { return CounterSampleCalculator.ComputeCounterValue(counterSample, nextCounterSample); } public override bool Equals(Object o) { return ( o is CounterSample) && Equals((CounterSample)o); } public bool Equals(CounterSample sample) { return (rawValue == sample.rawValue) && (baseValue == sample.baseValue) && (timeStamp == sample.timeStamp) && (counterFrequency == sample.counterFrequency) && (counterType == sample.counterType) && (timeStamp100nSec == sample.timeStamp100nSec) && (systemFrequency == sample.systemFrequency) && (counterTimeStamp == sample.counterTimeStamp); } public override int GetHashCode() { return rawValue.GetHashCode(); } public static bool operator ==(CounterSample a, CounterSample b) { return a.Equals(b); } public static bool operator !=(CounterSample a, CounterSample b) { return !(a.Equals(b)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
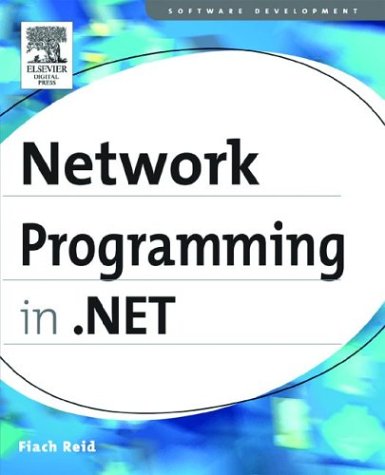
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- datacache.cs
- Size3DValueSerializer.cs
- ColorAnimationUsingKeyFrames.cs
- KnownTypes.cs
- CodeAttributeArgument.cs
- UpdatePanelControlTrigger.cs
- Geometry.cs
- ISessionStateStore.cs
- ApplicationHost.cs
- ObjectListCommandsPage.cs
- PointCollectionConverter.cs
- RepeatInfo.cs
- Table.cs
- BindToObject.cs
- GenericWebPart.cs
- DispatchOperation.cs
- ProjectionPathSegment.cs
- Assign.cs
- HostedHttpContext.cs
- RelatedCurrencyManager.cs
- XmlLinkedNode.cs
- BuildManagerHost.cs
- EndpointReference.cs
- errorpatternmatcher.cs
- RequestCachePolicy.cs
- ButtonBase.cs
- AuthenticationModuleElementCollection.cs
- DataTableCollection.cs
- FormatConvertedBitmap.cs
- FaultHandlingFilter.cs
- KnownBoxes.cs
- PartitionResolver.cs
- storepermissionattribute.cs
- InfoCardSchemas.cs
- DbUpdateCommandTree.cs
- CertificateManager.cs
- DataGridCellAutomationPeer.cs
- DocumentGridPage.cs
- ClientBuildManager.cs
- UIElementPropertyUndoUnit.cs
- XmlSchemaComplexContent.cs
- IdnElement.cs
- DocumentCollection.cs
- HandleCollector.cs
- BezierSegment.cs
- CompositeCollectionView.cs
- MimeTextImporter.cs
- URLMembershipCondition.cs
- Int32KeyFrameCollection.cs
- ExecutionEngineException.cs
- DataView.cs
- ApplicationSecurityInfo.cs
- __FastResourceComparer.cs
- MetafileHeader.cs
- TitleStyle.cs
- StringFunctions.cs
- TagMapInfo.cs
- sqlser.cs
- TabControlDesigner.cs
- SessionPageStatePersister.cs
- UnknownBitmapDecoder.cs
- FullTextBreakpoint.cs
- ScriptHandlerFactory.cs
- ReturnType.cs
- FixedTextView.cs
- SessionKeyExpiredException.cs
- LinqExpressionNormalizer.cs
- StringResourceManager.cs
- ExceptionHelpers.cs
- Misc.cs
- FormViewPagerRow.cs
- DataGridItemAutomationPeer.cs
- EventRoute.cs
- KeyboardNavigation.cs
- MobileErrorInfo.cs
- PnrpPermission.cs
- XPathSelectionIterator.cs
- XmlSignificantWhitespace.cs
- ElementUtil.cs
- DataColumnMapping.cs
- AddInDeploymentState.cs
- XmlIterators.cs
- WorkflowTransactionService.cs
- UpDownBase.cs
- InternalTypeHelper.cs
- HtmlTableRowCollection.cs
- KoreanLunisolarCalendar.cs
- ConfigurationManager.cs
- FileRecordSequence.cs
- CodeGen.cs
- DSGeneratorProblem.cs
- DbConnectionPoolCounters.cs
- InputLangChangeEvent.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- XmlIncludeAttribute.cs
- ImageList.cs
- ProcessHostFactoryHelper.cs
- CodeGeneratorOptions.cs
- ConstrainedDataObject.cs
- CapabilitiesAssignment.cs