Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / EntityDesignerUtils.cs / 1305376 / EntityDesignerUtils.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.IO; using System.Text; using System.Xml; using System.Data.Metadata.Edm; using System.Data.Mapping; namespace System.Data.Entity.Design { internal static class EntityDesignerUtils { private static readonly EFNamespaceSet v1Namespaces = new EFNamespaceSet { Edmx = "http://schemas.microsoft.com/ado/2007/06/edmx", Csdl = XmlConstants.ModelNamespace_1, Msl = StorageMslConstructs.NamespaceUriV1, Ssdl = XmlConstants.TargetNamespace_1 }; private static readonly EFNamespaceSet v2Namespaces = new EFNamespaceSet { Edmx = "http://schemas.microsoft.com/ado/2008/10/edmx", Csdl = XmlConstants.ModelNamespace_2, Msl = StorageMslConstructs.NamespaceUriV2, Ssdl = XmlConstants.TargetNamespace_2 }; internal static readonly string _edmxFileExtension = ".edmx"; ////// Extract the Conceptual, Mapping and Storage nodes from an EDMX input streams, and extract the value of the metadataArtifactProcessing property. /// /// /// /// /// /// internal static void ExtractConceptualMappingAndStorageNodes(StreamReader edmxInputStream, out XmlElement conceptualSchemaNode, out XmlElement mappingNode, out XmlElement storageSchemaNode, out string metadataArtifactProcessingValue) { // load up an XML document representing the edmx file XmlDocument xmlDocument = new XmlDocument(); xmlDocument.Load(edmxInputStream); EFNamespaceSet set = v2Namespaces; if (xmlDocument.DocumentElement.NamespaceURI == v1Namespaces.Edmx) { set = v1Namespaces; } XmlNamespaceManager nsMgr = new XmlNamespaceManager(xmlDocument.NameTable); nsMgr.AddNamespace("edmx", set.Edmx); nsMgr.AddNamespace("edm", set.Csdl); nsMgr.AddNamespace("ssdl", set.Ssdl); nsMgr.AddNamespace("map", set.Msl); // find the ConceptualModel Schema node conceptualSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:ConceptualModels/edm:Schema", nsMgr); // find the StorageModel Schema node storageSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:StorageModels/ssdl:Schema", nsMgr); // find the Mapping node mappingNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:Mappings/map:Mapping", nsMgr); // find the Connection node metadataArtifactProcessingValue = String.Empty; XmlNodeList connectionProperties = xmlDocument.SelectNodes( "/edmx:Edmx/edmx:Designer/edmx:Connection/edmx:DesignerInfoPropertySet/edmx:DesignerProperty", nsMgr); if (connectionProperties != null) { foreach (XmlNode propertyNode in connectionProperties) { foreach (XmlAttribute a in propertyNode.Attributes) { // treat attribute names case-sensitive (since it is xml), but attribute value case-insensitive to be accommodating . if (a.Name.Equals("Name", StringComparison.Ordinal) && a.Value.Equals("MetadataArtifactProcessing", StringComparison.OrdinalIgnoreCase)) { foreach (XmlAttribute a2 in propertyNode.Attributes) { if (a2.Name.Equals("Value", StringComparison.Ordinal)) { metadataArtifactProcessingValue = a2.Value; break; } } } } } } } // utility method to ensure an XmlElement (containing the C, M or S element // from the Edmx file) is sent out to a stream in the same format internal static void OutputXmlElementToStream(XmlElement xmlElement, Stream stream) { XmlWriterSettings settings = new XmlWriterSettings(); settings.Encoding = Encoding.UTF8; settings.Indent = true; // set up output document XmlDocument outputXmlDoc = new XmlDocument(); XmlNode importedElement = outputXmlDoc.ImportNode(xmlElement, true); outputXmlDoc.AppendChild(importedElement); // write out XmlDocument XmlWriter writer = null; try { writer = XmlWriter.Create(stream, settings); outputXmlDoc.WriteTo(writer); } finally { if (writer != null) { writer.Close(); } } } private struct EFNamespaceSet { public string Edmx; public string Csdl; public string Msl; public string Ssdl; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.IO; using System.Text; using System.Xml; using System.Data.Metadata.Edm; using System.Data.Mapping; namespace System.Data.Entity.Design { internal static class EntityDesignerUtils { private static readonly EFNamespaceSet v1Namespaces = new EFNamespaceSet { Edmx = "http://schemas.microsoft.com/ado/2007/06/edmx", Csdl = XmlConstants.ModelNamespace_1, Msl = StorageMslConstructs.NamespaceUriV1, Ssdl = XmlConstants.TargetNamespace_1 }; private static readonly EFNamespaceSet v2Namespaces = new EFNamespaceSet { Edmx = "http://schemas.microsoft.com/ado/2008/10/edmx", Csdl = XmlConstants.ModelNamespace_2, Msl = StorageMslConstructs.NamespaceUriV2, Ssdl = XmlConstants.TargetNamespace_2 }; internal static readonly string _edmxFileExtension = ".edmx"; ////// Extract the Conceptual, Mapping and Storage nodes from an EDMX input streams, and extract the value of the metadataArtifactProcessing property. /// /// /// /// /// /// internal static void ExtractConceptualMappingAndStorageNodes(StreamReader edmxInputStream, out XmlElement conceptualSchemaNode, out XmlElement mappingNode, out XmlElement storageSchemaNode, out string metadataArtifactProcessingValue) { // load up an XML document representing the edmx file XmlDocument xmlDocument = new XmlDocument(); xmlDocument.Load(edmxInputStream); EFNamespaceSet set = v2Namespaces; if (xmlDocument.DocumentElement.NamespaceURI == v1Namespaces.Edmx) { set = v1Namespaces; } XmlNamespaceManager nsMgr = new XmlNamespaceManager(xmlDocument.NameTable); nsMgr.AddNamespace("edmx", set.Edmx); nsMgr.AddNamespace("edm", set.Csdl); nsMgr.AddNamespace("ssdl", set.Ssdl); nsMgr.AddNamespace("map", set.Msl); // find the ConceptualModel Schema node conceptualSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:ConceptualModels/edm:Schema", nsMgr); // find the StorageModel Schema node storageSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:StorageModels/ssdl:Schema", nsMgr); // find the Mapping node mappingNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:Mappings/map:Mapping", nsMgr); // find the Connection node metadataArtifactProcessingValue = String.Empty; XmlNodeList connectionProperties = xmlDocument.SelectNodes( "/edmx:Edmx/edmx:Designer/edmx:Connection/edmx:DesignerInfoPropertySet/edmx:DesignerProperty", nsMgr); if (connectionProperties != null) { foreach (XmlNode propertyNode in connectionProperties) { foreach (XmlAttribute a in propertyNode.Attributes) { // treat attribute names case-sensitive (since it is xml), but attribute value case-insensitive to be accommodating . if (a.Name.Equals("Name", StringComparison.Ordinal) && a.Value.Equals("MetadataArtifactProcessing", StringComparison.OrdinalIgnoreCase)) { foreach (XmlAttribute a2 in propertyNode.Attributes) { if (a2.Name.Equals("Value", StringComparison.Ordinal)) { metadataArtifactProcessingValue = a2.Value; break; } } } } } } } // utility method to ensure an XmlElement (containing the C, M or S element // from the Edmx file) is sent out to a stream in the same format internal static void OutputXmlElementToStream(XmlElement xmlElement, Stream stream) { XmlWriterSettings settings = new XmlWriterSettings(); settings.Encoding = Encoding.UTF8; settings.Indent = true; // set up output document XmlDocument outputXmlDoc = new XmlDocument(); XmlNode importedElement = outputXmlDoc.ImportNode(xmlElement, true); outputXmlDoc.AppendChild(importedElement); // write out XmlDocument XmlWriter writer = null; try { writer = XmlWriter.Create(stream, settings); outputXmlDoc.WriteTo(writer); } finally { if (writer != null) { writer.Close(); } } } private struct EFNamespaceSet { public string Edmx; public string Csdl; public string Msl; public string Ssdl; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
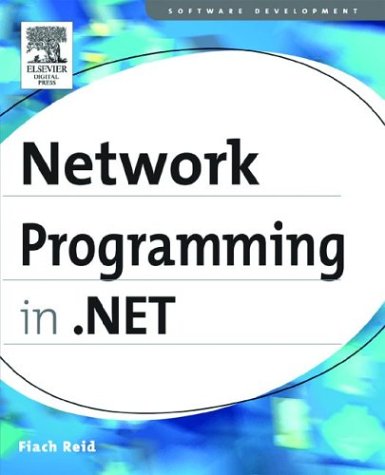
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WinFormsUtils.cs
- RemoteTokenFactory.cs
- ComponentDispatcherThread.cs
- UseLicense.cs
- FamilyMap.cs
- DbgCompiler.cs
- BitmapData.cs
- OutputScopeManager.cs
- TemplateManager.cs
- CodeCommentStatement.cs
- MsmqBindingBase.cs
- NumberFormatter.cs
- ChannelRequirements.cs
- ArcSegment.cs
- WsdlInspector.cs
- LinqDataSourceEditData.cs
- SecurityKeyUsage.cs
- PaintEvent.cs
- SharedStatics.cs
- ViewBox.cs
- _NestedMultipleAsyncResult.cs
- ImageListStreamer.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- ImageMapEventArgs.cs
- Expression.cs
- ImageListUtils.cs
- TextServicesCompartmentEventSink.cs
- ConstraintConverter.cs
- ExpressionQuoter.cs
- HtmlControlPersistable.cs
- DbProviderFactory.cs
- HtmlValidatorAdapter.cs
- DataView.cs
- SqlUtil.cs
- Compress.cs
- XPathParser.cs
- CheckBoxRenderer.cs
- StrongNameKeyPair.cs
- _LoggingObject.cs
- autovalidator.cs
- SqlDataSourceStatusEventArgs.cs
- RandomNumberGenerator.cs
- FieldTemplateUserControl.cs
- XmlSchemaAnyAttribute.cs
- TraceSwitch.cs
- WebService.cs
- PenLineJoinValidation.cs
- RawMouseInputReport.cs
- PageStatePersister.cs
- Marshal.cs
- ToolStripSplitStackLayout.cs
- AuthenticationSchemesHelper.cs
- StrokeNodeData.cs
- TracedNativeMethods.cs
- PropertyGrid.cs
- ByteStack.cs
- TextBoxBase.cs
- SafeThemeHandle.cs
- PageTheme.cs
- InheritanceContextHelper.cs
- PartialTrustVisibleAssembly.cs
- HttpProfileGroupBase.cs
- SolidColorBrush.cs
- UrlPropertyAttribute.cs
- MessageQueuePermissionAttribute.cs
- CheckBox.cs
- MsmqSecureHashAlgorithm.cs
- RegexMatch.cs
- UrlMappingsModule.cs
- JpegBitmapEncoder.cs
- TextServicesManager.cs
- entitydatasourceentitysetnameconverter.cs
- IPeerNeighbor.cs
- XmlSchemaAnnotated.cs
- ProfessionalColorTable.cs
- CharacterBufferReference.cs
- EdmRelationshipRoleAttribute.cs
- ResumeStoryboard.cs
- SymbolMethod.cs
- ExceptionUtility.cs
- IdentityManager.cs
- GridSplitterAutomationPeer.cs
- Panel.cs
- AndCondition.cs
- UseManagedPresentationBindingElementImporter.cs
- SynchronizationLockException.cs
- SmiEventSink.cs
- HorizontalAlignConverter.cs
- HttpCookiesSection.cs
- ToolboxComponentsCreatingEventArgs.cs
- ReaderOutput.cs
- SortDescriptionCollection.cs
- SoapServerMethod.cs
- Pair.cs
- IriParsingElement.cs
- InlinedAggregationOperatorEnumerator.cs
- ChtmlPageAdapter.cs
- BitVector32.cs
- ScriptBehaviorDescriptor.cs
- XmlCDATASection.cs