Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / NegatedConstant.cs / 1305376 / NegatedConstant.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common.Utils; using System.Text; using System.Diagnostics; using System.Data.Mapping.ViewGeneration.Utils; using System.Data.Entity; namespace System.Data.Mapping.ViewGeneration.Structures { // A class that represents NOT(elements), e.g., NOT(1, 2, NULL), i.e., // all values other than null, 1 and 2 internal class NegatedConstant : Constant { #region Constructors // effects: Creates NOT(NULL} internal static NegatedConstant CreateNotNull() { NegatedConstant result = new NegatedConstant(); result.m_negatedDomain.Add(Constant.Null); return result; } // effects: Creates an empty domain private NegatedConstant() { m_negatedDomain = new Set(Constant.EqualityComparer); } // requires: values has no NegatedCellConstant // effects: Creates a negated constant with the values in it internal NegatedConstant(IEnumerable values) { m_negatedDomain = new Set (values, Constant.EqualityComparer); } #endregion #region Fields private Set m_negatedDomain; // e.g., NOT(1, 2, Undefined) #endregion #region Properties internal IEnumerable Elements { get { return m_negatedDomain; } } #endregion #region Methods // effects: Returns true if this contains "constant" internal bool Contains(Constant constant) { return m_negatedDomain.Contains(constant); } // effects: Returns true if this represents "NotNull" precisely internal override bool IsNotNull() { return m_negatedDomain.Count == 1 && m_negatedDomain.Contains(Constant.Null); } // effects: Returns true if this contains "NotNull" internal override bool HasNotNull() { return m_negatedDomain.Contains(Constant.Null); } // effects: Returns a hash value for this protected override int GetHash() { int result = 0; foreach (Constant constant in m_negatedDomain) { result ^= Constant.EqualityComparer.GetHashCode(constant); } return result; } // effects: Returns true if right has the same contents as this protected override bool IsEqualTo(Constant right) { NegatedConstant rightNegatedConstant = right as NegatedConstant; if (rightNegatedConstant == null) { return false; } return m_negatedDomain.SetEquals(rightNegatedConstant.m_negatedDomain); } // effects: Given a set of positive constants (constants) and this, // generates a Cql expression, e.g., 7, NOT(7, NULL) means NOT NULL // 7, 8, NOT(7, 8, 9, 10) Means NOT(9, 10) // Note: NOT(9, NULL) is NOT (a is 9 OR a is NULL) = // A IS NOT 9 AND A IS NOT NULL internal StringBuilder AsCql(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, constants, path, canSkipIsNotNull, false); } // effects: Given a set of constants, generates "Slot = X AND Slot = Y" or "Slot <> X AND Slot <> Y" (depending ifGenerateEquals) private static void GenerateAndsForSlot(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool anyAdded, bool userString) { foreach (Constant constant in constants) { if (anyAdded) { builder.Append(" AND "); } anyAdded = true; if (userString) { path.ToCompactString(builder, blockAlias); builder.Append(" <>"); constant.ToCompactString(builder); } else { path.AsCql(builder, blockAlias); builder.Append(" <>"); constant.AsCql(builder, path, blockAlias); } } } private StringBuilder ToStringHelper(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull, bool userString) { bool isNullable = path.IsNullable; // Remove all the constants from negated and then print NOT // .. AND NOT .. AND NOT ... Set negatedConstants = new Set (Elements, Constant.EqualityComparer); foreach (Constant constant in constants) { if (constant.Equals(this)) { continue; } Debug.Assert(negatedConstants.Contains(constant), "Negated constant must contain all positive constants"); negatedConstants.Remove(constant); } if (negatedConstants.Count == 0) { // All constants cancel out builder.Append("true"); return builder; } bool hasNull = negatedConstants.Contains(Constant.Null); negatedConstants.Remove(Constant.Null); // We always add IS NOT NULL if the property is nullable (and we // cannot skip IS NOT NULL). Also, if the domain contains NOT // NULL, we must add it bool anyAdded = false; if (hasNull || (isNullable && canSkipIsNotNull == false)) { if (userString) { path.ToCompactString(builder, blockAlias); builder.Append(" is not NULL"); } else { path.AsCql(builder, blockAlias); builder.Append(" IS NOT NULL"); } anyAdded = true; } GenerateAndsForSlot(builder, blockAlias, negatedConstants, path, anyAdded, userString); return builder; } internal StringBuilder AsUserString(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, constants, path, canSkipIsNotNull, true); } internal override string ToUserString() { if (IsNotNull()) { return System.Data.Entity.Strings.ViewGen_NotNull; } else { StringBuilder builder = new StringBuilder(); bool isFirst = true; foreach (Constant constant in m_negatedDomain) { // Skip printing out Null if m_negatedDomain has other values if (m_negatedDomain.Count > 1 && constant.IsNull()) { continue; } if (isFirst == false) { builder.Append(System.Data.Entity.Strings.ViewGen_CommaBlank); } isFirst = false; builder.Append(constant.ToUserString()); } StringBuilder result = new StringBuilder(); result.Append(Strings.ViewGen_NegatedCellConstant_0(builder.ToString())); return result.ToString(); } } internal override void ToCompactString(StringBuilder builder) { // Special code for NOT NULL if (IsNotNull()) { builder.Append("NOT_NULL"); } else { builder.Append("NOT("); StringUtil.ToCommaSeparatedStringSorted(builder, m_negatedDomain); builder.Append(")"); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common.Utils; using System.Text; using System.Diagnostics; using System.Data.Mapping.ViewGeneration.Utils; using System.Data.Entity; namespace System.Data.Mapping.ViewGeneration.Structures { // A class that represents NOT(elements), e.g., NOT(1, 2, NULL), i.e., // all values other than null, 1 and 2 internal class NegatedConstant : Constant { #region Constructors // effects: Creates NOT(NULL} internal static NegatedConstant CreateNotNull() { NegatedConstant result = new NegatedConstant(); result.m_negatedDomain.Add(Constant.Null); return result; } // effects: Creates an empty domain private NegatedConstant() { m_negatedDomain = new Set(Constant.EqualityComparer); } // requires: values has no NegatedCellConstant // effects: Creates a negated constant with the values in it internal NegatedConstant(IEnumerable values) { m_negatedDomain = new Set (values, Constant.EqualityComparer); } #endregion #region Fields private Set m_negatedDomain; // e.g., NOT(1, 2, Undefined) #endregion #region Properties internal IEnumerable Elements { get { return m_negatedDomain; } } #endregion #region Methods // effects: Returns true if this contains "constant" internal bool Contains(Constant constant) { return m_negatedDomain.Contains(constant); } // effects: Returns true if this represents "NotNull" precisely internal override bool IsNotNull() { return m_negatedDomain.Count == 1 && m_negatedDomain.Contains(Constant.Null); } // effects: Returns true if this contains "NotNull" internal override bool HasNotNull() { return m_negatedDomain.Contains(Constant.Null); } // effects: Returns a hash value for this protected override int GetHash() { int result = 0; foreach (Constant constant in m_negatedDomain) { result ^= Constant.EqualityComparer.GetHashCode(constant); } return result; } // effects: Returns true if right has the same contents as this protected override bool IsEqualTo(Constant right) { NegatedConstant rightNegatedConstant = right as NegatedConstant; if (rightNegatedConstant == null) { return false; } return m_negatedDomain.SetEquals(rightNegatedConstant.m_negatedDomain); } // effects: Given a set of positive constants (constants) and this, // generates a Cql expression, e.g., 7, NOT(7, NULL) means NOT NULL // 7, 8, NOT(7, 8, 9, 10) Means NOT(9, 10) // Note: NOT(9, NULL) is NOT (a is 9 OR a is NULL) = // A IS NOT 9 AND A IS NOT NULL internal StringBuilder AsCql(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, constants, path, canSkipIsNotNull, false); } // effects: Given a set of constants, generates "Slot = X AND Slot = Y" or "Slot <> X AND Slot <> Y" (depending ifGenerateEquals) private static void GenerateAndsForSlot(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool anyAdded, bool userString) { foreach (Constant constant in constants) { if (anyAdded) { builder.Append(" AND "); } anyAdded = true; if (userString) { path.ToCompactString(builder, blockAlias); builder.Append(" <>"); constant.ToCompactString(builder); } else { path.AsCql(builder, blockAlias); builder.Append(" <>"); constant.AsCql(builder, path, blockAlias); } } } private StringBuilder ToStringHelper(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull, bool userString) { bool isNullable = path.IsNullable; // Remove all the constants from negated and then print NOT // .. AND NOT .. AND NOT ... Set negatedConstants = new Set (Elements, Constant.EqualityComparer); foreach (Constant constant in constants) { if (constant.Equals(this)) { continue; } Debug.Assert(negatedConstants.Contains(constant), "Negated constant must contain all positive constants"); negatedConstants.Remove(constant); } if (negatedConstants.Count == 0) { // All constants cancel out builder.Append("true"); return builder; } bool hasNull = negatedConstants.Contains(Constant.Null); negatedConstants.Remove(Constant.Null); // We always add IS NOT NULL if the property is nullable (and we // cannot skip IS NOT NULL). Also, if the domain contains NOT // NULL, we must add it bool anyAdded = false; if (hasNull || (isNullable && canSkipIsNotNull == false)) { if (userString) { path.ToCompactString(builder, blockAlias); builder.Append(" is not NULL"); } else { path.AsCql(builder, blockAlias); builder.Append(" IS NOT NULL"); } anyAdded = true; } GenerateAndsForSlot(builder, blockAlias, negatedConstants, path, anyAdded, userString); return builder; } internal StringBuilder AsUserString(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, constants, path, canSkipIsNotNull, true); } internal override string ToUserString() { if (IsNotNull()) { return System.Data.Entity.Strings.ViewGen_NotNull; } else { StringBuilder builder = new StringBuilder(); bool isFirst = true; foreach (Constant constant in m_negatedDomain) { // Skip printing out Null if m_negatedDomain has other values if (m_negatedDomain.Count > 1 && constant.IsNull()) { continue; } if (isFirst == false) { builder.Append(System.Data.Entity.Strings.ViewGen_CommaBlank); } isFirst = false; builder.Append(constant.ToUserString()); } StringBuilder result = new StringBuilder(); result.Append(Strings.ViewGen_NegatedCellConstant_0(builder.ToString())); return result.ToString(); } } internal override void ToCompactString(StringBuilder builder) { // Special code for NOT NULL if (IsNotNull()) { builder.Append("NOT_NULL"); } else { builder.Append("NOT("); StringUtil.ToCommaSeparatedStringSorted(builder, m_negatedDomain); builder.Append(")"); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
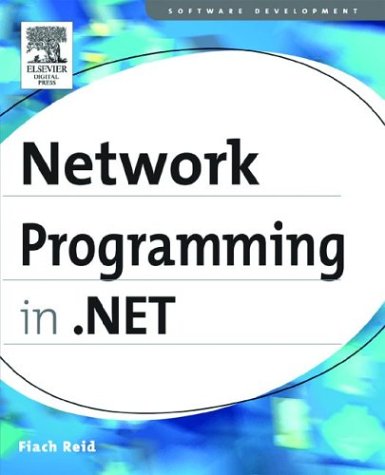
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FlowPanelDesigner.cs
- StringExpressionSet.cs
- SchemaCollectionCompiler.cs
- CommandEventArgs.cs
- RemotingServices.cs
- XmlReturnWriter.cs
- TemplatedWizardStep.cs
- TypefaceMetricsCache.cs
- FlowDocumentPaginator.cs
- WindowsScroll.cs
- UncommonField.cs
- BindingOperations.cs
- ScaleTransform.cs
- IndicFontClient.cs
- GridEntry.cs
- SortedDictionary.cs
- Rectangle.cs
- querybuilder.cs
- OracleParameter.cs
- GroupedContextMenuStrip.cs
- MimeBasePart.cs
- DataMember.cs
- Property.cs
- PrivilegeNotHeldException.cs
- SubpageParagraph.cs
- SQLBytes.cs
- ArcSegment.cs
- FontEmbeddingManager.cs
- WsatConfiguration.cs
- SizeConverter.cs
- UidManager.cs
- CryptographicAttribute.cs
- CodeTypeOfExpression.cs
- Lasso.cs
- ExtentJoinTreeNode.cs
- SoapSchemaImporter.cs
- KeyValueInternalCollection.cs
- ImageUrlEditor.cs
- DataTemplateKey.cs
- XsdValidatingReader.cs
- CapabilitiesUse.cs
- CustomError.cs
- DataGridTablesFactory.cs
- shaper.cs
- OleDbParameterCollection.cs
- OleDbRowUpdatedEvent.cs
- PenLineJoinValidation.cs
- DesignerActionListCollection.cs
- KeyboardEventArgs.cs
- Solver.cs
- IDReferencePropertyAttribute.cs
- RowUpdatingEventArgs.cs
- WarningException.cs
- PenThread.cs
- HttpWriter.cs
- FilteredXmlReader.cs
- MarginCollapsingState.cs
- InternalException.cs
- IdnElement.cs
- XmlSchemaProviderAttribute.cs
- ScriptReference.cs
- Misc.cs
- ClientSponsor.cs
- CopyCodeAction.cs
- TypeBuilderInstantiation.cs
- RulePatternOps.cs
- CompModSwitches.cs
- SubclassTypeValidator.cs
- OracleNumber.cs
- DataGridCommandEventArgs.cs
- ImageField.cs
- ValueProviderWrapper.cs
- PaintValueEventArgs.cs
- SchemaTableColumn.cs
- QilReplaceVisitor.cs
- XmlWellformedWriter.cs
- PasswordTextContainer.cs
- ThreadInterruptedException.cs
- WebBrowserNavigatingEventHandler.cs
- Wildcard.cs
- EntityProxyTypeInfo.cs
- Parser.cs
- DataGridViewImageCell.cs
- QilSortKey.cs
- HttpCacheVaryByContentEncodings.cs
- DataGridRowDetailsEventArgs.cs
- PageParser.cs
- RegexCapture.cs
- DesignerActionUI.cs
- WindowsFormsSectionHandler.cs
- ActiveDocumentEvent.cs
- VisualProxy.cs
- SiteMapProvider.cs
- Soap.cs
- ConditionCollection.cs
- TreeViewEvent.cs
- Exceptions.cs
- MasterPageBuildProvider.cs
- Bits.cs
- ResourceExpressionBuilder.cs