Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Advanced / Metafile.cs / 1305376 / Metafile.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Internal; using System.Drawing; using System.Drawing.Design; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Internal; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Runtime.Versioning; /** * Represent a metafile image */ ////// /// Defines a graphic metafile. A metafile /// contains records that describe a sequence of graphics operations that can be /// recorded and played back. /// [ Editor("System.Drawing.Design.MetafileEditor, " + AssemblyRef.SystemDrawingDesign, typeof(UITypeEditor)), ] [Serializable] public sealed class Metafile : Image { /* * Create a new metafile object from a metafile handle (WMF) */ ////// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr hmetafile, WmfPlaceableFileHeader wmfHeader) : this(hmetafile, wmfHeader, false) {} ////// Initializes a new instance of the ///class from the specified handle and /// . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr hmetafile, WmfPlaceableFileHeader wmfHeader, bool deleteWmf) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateMetafileFromWmf(new HandleRef(null, hmetafile), wmfHeader, deleteWmf, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } /* * Create a new metafile object from an enhanced metafile handle */ ////// Initializes a new instance of the ///class from the specified handle and /// . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr henhmetafile, bool deleteEmf) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateMetafileFromEmf(new HandleRef(null, henhmetafile), deleteEmf, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } /** * Create a new metafile object from a file */ ////// Initializes a new instance of the ///class from the /// specified handle and . /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string filename) { IntSecurity.DemandReadFileIO(filename); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateMetafileFromFile(filename, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } /** * Create a new metafile object from a stream */ ///class from the specified filename. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream) { if (stream == null) throw new ArgumentException(SR.GetString(SR.InvalidArgument, "stream", "null")); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateMetafileFromStream(new GPStream(stream), out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ///class from the specified stream. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, EmfType emfType) : this(referenceHdc, emfType, null) {} ///class from the specified handle to a /// device context. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, EmfType emfType, String description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipRecordMetafile(new HandleRef(null, referenceHdc), (int)emfType, NativeMethods.NullHandleRef, (int) MetafileFrameUnit.GdiCompatible, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class from the /// specified handle to a device context. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, RectangleF frameRect) : this(referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class from the specified device context, /// bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit) : this(referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class from the specified device context, /// bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type, String description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; GPRECTF rectf = new GPRECTF(frameRect); int status = SafeNativeMethods.Gdip.GdipRecordMetafile(new HandleRef(null, referenceHdc), (int)type, ref rectf, (int)frameUnit, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class from the specified device context, /// bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, Rectangle frameRect) : this(referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit) : this(referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type, string desc) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status; if (frameRect.IsEmpty) { status = SafeNativeMethods.Gdip.GdipRecordMetafile(new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int)MetafileFrameUnit.GdiCompatible, desc, out metafile); } else { GPRECT gprect = new GPRECT(frameRect); status = SafeNativeMethods.Gdip.GdipRecordMetafileI(new HandleRef(null, referenceHdc), (int)type, ref gprect, (int)frameUnit, desc, out metafile); } if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc) : this(fileName, referenceHdc, EmfType.EmfPlusDual, null) {} ///class with the specified /// filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, EmfType type) : this(fileName, referenceHdc, type, null) {} ////// Initializes a new instance of the ///class with the specified /// filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, EmfType type, String description) { IntSecurity.DemandReadFileIO(fileName); IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipRecordMetafileFileName(fileName, new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int) MetafileFrameUnit.GdiCompatible, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class with the specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect) : this(fileName, referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class with the specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit) : this(fileName, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class with the specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(fileName, referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class with the specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, string desc) : this(fileName, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual, desc) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type, String description) { IntSecurity.DemandReadFileIO(fileName); IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; GPRECTF rectf = new GPRECTF(frameRect); int status = SafeNativeMethods.Gdip.GdipRecordMetafileFileName(fileName, new HandleRef(null, referenceHdc), (int)type, ref rectf, (int)frameUnit, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect) : this(fileName, referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit) : this(fileName, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(fileName, referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, string description) : this(fileName, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual, description) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type, string description) { IntSecurity.DemandReadFileIO(fileName); IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status; if (frameRect.IsEmpty) { status = SafeNativeMethods.Gdip.GdipRecordMetafileFileName(fileName, new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int)frameUnit, description, out metafile); } else { GPRECT gprect = new GPRECT(frameRect); status = SafeNativeMethods.Gdip.GdipRecordMetafileFileNameI(fileName, new HandleRef(null, referenceHdc), (int)type, ref gprect, (int)frameUnit, description, out metafile); } if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc) : this(stream, referenceHdc, EmfType.EmfPlusDual, null) {} ////// Initializes a new instance of the ///class from the specified data /// stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, EmfType type) : this(stream, referenceHdc, type, null) {} ////// Initializes a new instance of the ///class from the specified data /// stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, EmfType type, string description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipRecordMetafileStream(new GPStream(stream), new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int)MetafileFrameUnit.GdiCompatible, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class from the specified data stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, RectangleF frameRect) : this(stream, referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class from the specified data stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit) : this(stream, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(stream, referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type, string description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; GPRECTF rectf = new GPRECTF(frameRect); int status = SafeNativeMethods.Gdip.GdipRecordMetafileStream(new GPStream(stream), new HandleRef(null, referenceHdc), (int)type, ref rectf, (int)frameUnit, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, Rectangle frameRect) : this(stream, referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class from the /// specified data stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit) : this(stream, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(stream, referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type, string description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status; if (frameRect.IsEmpty) { status = SafeNativeMethods.Gdip.GdipRecordMetafileStream(new GPStream(stream), new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int)frameUnit, description, out metafile); } else { GPRECT gprect = new GPRECT(frameRect); status = SafeNativeMethods.Gdip.GdipRecordMetafileStreamI(new GPStream(stream), new HandleRef(null, referenceHdc), (int)type, ref gprect, (int)frameUnit, description, out metafile); } if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } /** * Constructor used in deserialization */ [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] private Metafile(SerializationInfo info, StreamingContext context) : base(info, context) { } ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public static MetafileHeader GetMetafileHeader(IntPtr hmetafile, WmfPlaceableFileHeader wmfHeader) { IntSecurity.ObjectFromWin32Handle.Demand(); MetafileHeader header = new MetafileHeader(); header.wmf = new MetafileHeaderWmf(); int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromWmf(new HandleRef(null, hmetafile), wmfHeader, header.wmf); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return header; } ////// Returns the ///associated with the specified . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public static MetafileHeader GetMetafileHeader(IntPtr henhmetafile) { IntSecurity.ObjectFromWin32Handle.Demand(); MetafileHeader header = new MetafileHeader(); header.emf = new MetafileHeaderEmf(); int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromEmf(new HandleRef(null, henhmetafile), header.emf); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return header; } ////// Returns the ///associated with the specified . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public static MetafileHeader GetMetafileHeader(string fileName) { IntSecurity.DemandReadFileIO(fileName); MetafileHeader header = new MetafileHeader(); IntPtr memory = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(MetafileHeaderEmf))); try { int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromFile(fileName, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } int[] type = new int[] { 0}; Marshal.Copy(memory, type, 0, 1); MetafileType metafileType = (MetafileType) type[0]; if (metafileType == MetafileType.Wmf || metafileType == MetafileType.WmfPlaceable) { // WMF header header.wmf = (MetafileHeaderWmf) UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderWmf)); header.emf = null; } else { // EMF header header.wmf = null; header.emf = (MetafileHeaderEmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderEmf)); } } finally { Marshal.FreeHGlobal(memory); } return header; } ////// Returns the ///associated with the specified . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public static MetafileHeader GetMetafileHeader(Stream stream) { MetafileHeader header; IntPtr memory = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(MetafileHeaderEmf))); try { int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromStream(new GPStream(stream), memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } int[] type = new int[] { 0}; Marshal.Copy(memory, type, 0, 1); MetafileType metafileType = (MetafileType) type[0]; header = new MetafileHeader(); if (metafileType == MetafileType.Wmf || metafileType == MetafileType.WmfPlaceable) { // WMF header header.wmf = (MetafileHeaderWmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderWmf)); header.emf = null; } else { // EMF header header.wmf = null; header.emf = (MetafileHeaderEmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderEmf)); } } finally { Marshal.FreeHGlobal(memory); } return header; } ////// Returns the ///associated with the specified . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public MetafileHeader GetMetafileHeader() { MetafileHeader header; IntPtr memory = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(MetafileHeaderEmf))); try { int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromMetafile(new HandleRef(this, nativeImage), memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } int[] type = new int[] { 0}; Marshal.Copy(memory, type, 0, 1); MetafileType metafileType = (MetafileType) type[0]; header = new MetafileHeader(); if (metafileType == MetafileType.Wmf || metafileType == MetafileType.WmfPlaceable) { // WMF header header.wmf = (MetafileHeaderWmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderWmf)); header.emf = null; } else { // EMF header header.wmf = null; header.emf = (MetafileHeaderEmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderEmf)); } } finally { Marshal.FreeHGlobal(memory); } return header; } ////// Returns the ///associated with this . /// /// /// Returns a Windows handle to an enhanced /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public IntPtr GetHenhmetafile() { IntPtr hEmf = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipGetHemfFromMetafile(new HandleRef(this, nativeImage), out hEmf); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return hEmf; } ///. /// /// /// Plays an EMF+ file. /// public void PlayRecord(EmfPlusRecordType recordType, int flags, int dataSize, byte[] data) { // Used in conjunction with Graphics.EnumerateMetafile to play an EMF+ // The data must be DWORD aligned if it's an EMF or EMF+. It must be // WORD aligned if it's a WMF. int status = SafeNativeMethods.Gdip.GdipPlayMetafileRecord(new HandleRef(this, nativeImage), recordType, flags, dataSize, data); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } /* * Create a new metafile object from a native metafile handle. * This is only for internal purpose. */ internal static Metafile FromGDIplus(IntPtr nativeImage) { Metafile metafile = new Metafile(); metafile.SetNativeImage(nativeImage); return metafile; } private Metafile() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Internal; using System.Drawing; using System.Drawing.Design; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Internal; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Runtime.Versioning; /** * Represent a metafile image */ ////// /// Defines a graphic metafile. A metafile /// contains records that describe a sequence of graphics operations that can be /// recorded and played back. /// [ Editor("System.Drawing.Design.MetafileEditor, " + AssemblyRef.SystemDrawingDesign, typeof(UITypeEditor)), ] [Serializable] public sealed class Metafile : Image { /* * Create a new metafile object from a metafile handle (WMF) */ ////// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr hmetafile, WmfPlaceableFileHeader wmfHeader) : this(hmetafile, wmfHeader, false) {} ////// Initializes a new instance of the ///class from the specified handle and /// . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr hmetafile, WmfPlaceableFileHeader wmfHeader, bool deleteWmf) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateMetafileFromWmf(new HandleRef(null, hmetafile), wmfHeader, deleteWmf, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } /* * Create a new metafile object from an enhanced metafile handle */ ////// Initializes a new instance of the ///class from the specified handle and /// . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr henhmetafile, bool deleteEmf) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateMetafileFromEmf(new HandleRef(null, henhmetafile), deleteEmf, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } /** * Create a new metafile object from a file */ ////// Initializes a new instance of the ///class from the /// specified handle and . /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string filename) { IntSecurity.DemandReadFileIO(filename); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateMetafileFromFile(filename, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } /** * Create a new metafile object from a stream */ ///class from the specified filename. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream) { if (stream == null) throw new ArgumentException(SR.GetString(SR.InvalidArgument, "stream", "null")); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateMetafileFromStream(new GPStream(stream), out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ///class from the specified stream. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, EmfType emfType) : this(referenceHdc, emfType, null) {} ///class from the specified handle to a /// device context. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, EmfType emfType, String description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipRecordMetafile(new HandleRef(null, referenceHdc), (int)emfType, NativeMethods.NullHandleRef, (int) MetafileFrameUnit.GdiCompatible, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class from the /// specified handle to a device context. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, RectangleF frameRect) : this(referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class from the specified device context, /// bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit) : this(referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class from the specified device context, /// bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type, String description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; GPRECTF rectf = new GPRECTF(frameRect); int status = SafeNativeMethods.Gdip.GdipRecordMetafile(new HandleRef(null, referenceHdc), (int)type, ref rectf, (int)frameUnit, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class from the specified device context, /// bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, Rectangle frameRect) : this(referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit) : this(referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type, string desc) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status; if (frameRect.IsEmpty) { status = SafeNativeMethods.Gdip.GdipRecordMetafile(new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int)MetafileFrameUnit.GdiCompatible, desc, out metafile); } else { GPRECT gprect = new GPRECT(frameRect); status = SafeNativeMethods.Gdip.GdipRecordMetafileI(new HandleRef(null, referenceHdc), (int)type, ref gprect, (int)frameUnit, desc, out metafile); } if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class from the /// specified device context, bounded by the specified rectangle. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc) : this(fileName, referenceHdc, EmfType.EmfPlusDual, null) {} ///class with the specified /// filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, EmfType type) : this(fileName, referenceHdc, type, null) {} ////// Initializes a new instance of the ///class with the specified /// filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, EmfType type, String description) { IntSecurity.DemandReadFileIO(fileName); IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipRecordMetafileFileName(fileName, new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int) MetafileFrameUnit.GdiCompatible, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class with the specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect) : this(fileName, referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class with the specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit) : this(fileName, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class with the specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(fileName, referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class with the specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, string desc) : this(fileName, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual, desc) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type, String description) { IntSecurity.DemandReadFileIO(fileName); IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; GPRECTF rectf = new GPRECTF(frameRect); int status = SafeNativeMethods.Gdip.GdipRecordMetafileFileName(fileName, new HandleRef(null, referenceHdc), (int)type, ref rectf, (int)frameUnit, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect) : this(fileName, referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit) : this(fileName, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(fileName, referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, string description) : this(fileName, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual, description) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(string fileName, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type, string description) { IntSecurity.DemandReadFileIO(fileName); IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status; if (frameRect.IsEmpty) { status = SafeNativeMethods.Gdip.GdipRecordMetafileFileName(fileName, new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int)frameUnit, description, out metafile); } else { GPRECT gprect = new GPRECT(frameRect); status = SafeNativeMethods.Gdip.GdipRecordMetafileFileNameI(fileName, new HandleRef(null, referenceHdc), (int)type, ref gprect, (int)frameUnit, description, out metafile); } if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc) : this(stream, referenceHdc, EmfType.EmfPlusDual, null) {} ////// Initializes a new instance of the ///class from the specified data /// stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, EmfType type) : this(stream, referenceHdc, type, null) {} ////// Initializes a new instance of the ///class from the specified data /// stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, EmfType type, string description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipRecordMetafileStream(new GPStream(stream), new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int)MetafileFrameUnit.GdiCompatible, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class from the specified data stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, RectangleF frameRect) : this(stream, referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class from the specified data stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit) : this(stream, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(stream, referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, RectangleF frameRect, MetafileFrameUnit frameUnit, EmfType type, string description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; GPRECTF rectf = new GPRECTF(frameRect); int status = SafeNativeMethods.Gdip.GdipRecordMetafileStream(new GPStream(stream), new HandleRef(null, referenceHdc), (int)type, ref rectf, (int)frameUnit, description, out metafile); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, Rectangle frameRect) : this(stream, referenceHdc, frameRect, MetafileFrameUnit.GdiCompatible) {} ////// Initializes a new instance of the ///class from the /// specified data stream. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit) : this(stream, referenceHdc, frameRect, frameUnit, EmfType.EmfPlusDual) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type) : this(stream, referenceHdc, frameRect, frameUnit, type, null) {} ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Metafile(Stream stream, IntPtr referenceHdc, Rectangle frameRect, MetafileFrameUnit frameUnit, EmfType type, string description) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr metafile = IntPtr.Zero; int status; if (frameRect.IsEmpty) { status = SafeNativeMethods.Gdip.GdipRecordMetafileStream(new GPStream(stream), new HandleRef(null, referenceHdc), (int)type, NativeMethods.NullHandleRef, (int)frameUnit, description, out metafile); } else { GPRECT gprect = new GPRECT(frameRect); status = SafeNativeMethods.Gdip.GdipRecordMetafileStreamI(new GPStream(stream), new HandleRef(null, referenceHdc), (int)type, ref gprect, (int)frameUnit, description, out metafile); } if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImage(metafile); } /** * Constructor used in deserialization */ [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] private Metafile(SerializationInfo info, StreamingContext context) : base(info, context) { } ////// Initializes a new instance of the ///class with the /// specified filename. /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public static MetafileHeader GetMetafileHeader(IntPtr hmetafile, WmfPlaceableFileHeader wmfHeader) { IntSecurity.ObjectFromWin32Handle.Demand(); MetafileHeader header = new MetafileHeader(); header.wmf = new MetafileHeaderWmf(); int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromWmf(new HandleRef(null, hmetafile), wmfHeader, header.wmf); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return header; } ////// Returns the ///associated with the specified . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public static MetafileHeader GetMetafileHeader(IntPtr henhmetafile) { IntSecurity.ObjectFromWin32Handle.Demand(); MetafileHeader header = new MetafileHeader(); header.emf = new MetafileHeaderEmf(); int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromEmf(new HandleRef(null, henhmetafile), header.emf); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return header; } ////// Returns the ///associated with the specified . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public static MetafileHeader GetMetafileHeader(string fileName) { IntSecurity.DemandReadFileIO(fileName); MetafileHeader header = new MetafileHeader(); IntPtr memory = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(MetafileHeaderEmf))); try { int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromFile(fileName, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } int[] type = new int[] { 0}; Marshal.Copy(memory, type, 0, 1); MetafileType metafileType = (MetafileType) type[0]; if (metafileType == MetafileType.Wmf || metafileType == MetafileType.WmfPlaceable) { // WMF header header.wmf = (MetafileHeaderWmf) UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderWmf)); header.emf = null; } else { // EMF header header.wmf = null; header.emf = (MetafileHeaderEmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderEmf)); } } finally { Marshal.FreeHGlobal(memory); } return header; } ////// Returns the ///associated with the specified . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public static MetafileHeader GetMetafileHeader(Stream stream) { MetafileHeader header; IntPtr memory = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(MetafileHeaderEmf))); try { int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromStream(new GPStream(stream), memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } int[] type = new int[] { 0}; Marshal.Copy(memory, type, 0, 1); MetafileType metafileType = (MetafileType) type[0]; header = new MetafileHeader(); if (metafileType == MetafileType.Wmf || metafileType == MetafileType.WmfPlaceable) { // WMF header header.wmf = (MetafileHeaderWmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderWmf)); header.emf = null; } else { // EMF header header.wmf = null; header.emf = (MetafileHeaderEmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderEmf)); } } finally { Marshal.FreeHGlobal(memory); } return header; } ////// Returns the ///associated with the specified . /// /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public MetafileHeader GetMetafileHeader() { MetafileHeader header; IntPtr memory = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(MetafileHeaderEmf))); try { int status = SafeNativeMethods.Gdip.GdipGetMetafileHeaderFromMetafile(new HandleRef(this, nativeImage), memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } int[] type = new int[] { 0}; Marshal.Copy(memory, type, 0, 1); MetafileType metafileType = (MetafileType) type[0]; header = new MetafileHeader(); if (metafileType == MetafileType.Wmf || metafileType == MetafileType.WmfPlaceable) { // WMF header header.wmf = (MetafileHeaderWmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderWmf)); header.emf = null; } else { // EMF header header.wmf = null; header.emf = (MetafileHeaderEmf)UnsafeNativeMethods.PtrToStructure(memory, typeof(MetafileHeaderEmf)); } } finally { Marshal.FreeHGlobal(memory); } return header; } ////// Returns the ///associated with this . /// /// /// Returns a Windows handle to an enhanced /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public IntPtr GetHenhmetafile() { IntPtr hEmf = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipGetHemfFromMetafile(new HandleRef(this, nativeImage), out hEmf); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return hEmf; } ///. /// /// /// Plays an EMF+ file. /// public void PlayRecord(EmfPlusRecordType recordType, int flags, int dataSize, byte[] data) { // Used in conjunction with Graphics.EnumerateMetafile to play an EMF+ // The data must be DWORD aligned if it's an EMF or EMF+. It must be // WORD aligned if it's a WMF. int status = SafeNativeMethods.Gdip.GdipPlayMetafileRecord(new HandleRef(this, nativeImage), recordType, flags, dataSize, data); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } /* * Create a new metafile object from a native metafile handle. * This is only for internal purpose. */ internal static Metafile FromGDIplus(IntPtr nativeImage) { Metafile metafile = new Metafile(); metafile.SetNativeImage(nativeImage); return metafile; } private Metafile() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
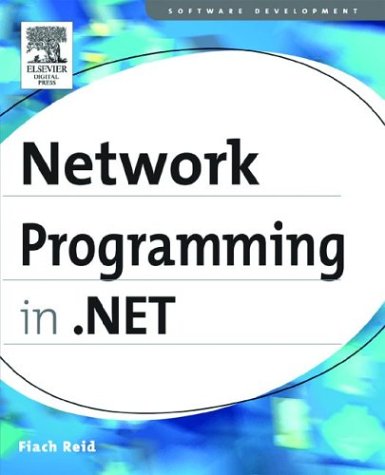
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PolyQuadraticBezierSegment.cs
- arabicshape.cs
- SafeHandle.cs
- XmlSchemaInferenceException.cs
- PageAdapter.cs
- InvalidComObjectException.cs
- ClientUtils.cs
- FileLevelControlBuilderAttribute.cs
- SqlEnums.cs
- BridgeDataRecord.cs
- ObjectTag.cs
- GeneralTransform3DCollection.cs
- AssemblyBuilderData.cs
- ReferencedCollectionType.cs
- LicenseContext.cs
- HtmlInputControl.cs
- ControlParameter.cs
- CharConverter.cs
- RequestCachePolicy.cs
- panel.cs
- IImplicitResourceProvider.cs
- TextRenderingModeValidation.cs
- TimeSpanStorage.cs
- PageBuildProvider.cs
- EntityDataSourceWizardForm.cs
- Config.cs
- WebPartDescription.cs
- NetworkAddressChange.cs
- HandlerBase.cs
- Journaling.cs
- QueryOptionExpression.cs
- UInt64Converter.cs
- ElapsedEventArgs.cs
- ConnectionStringSettings.cs
- XPathChildIterator.cs
- DataServiceSaveChangesEventArgs.cs
- StylusShape.cs
- GridViewDeleteEventArgs.cs
- ReadContentAsBinaryHelper.cs
- BaseParaClient.cs
- MenuItemCollection.cs
- AVElementHelper.cs
- FullTextLine.cs
- DynamicResourceExtension.cs
- SymbolMethod.cs
- infer.cs
- LostFocusEventManager.cs
- CuspData.cs
- WindowsContainer.cs
- DrawingVisualDrawingContext.cs
- ImageListStreamer.cs
- OdbcFactory.cs
- NCryptSafeHandles.cs
- InvalidOperationException.cs
- ControlIdConverter.cs
- XmlCharacterData.cs
- BuildProviderUtils.cs
- IpcChannel.cs
- RecipientServiceModelSecurityTokenRequirement.cs
- SecurityRuntime.cs
- RegistryPermission.cs
- PolyLineSegment.cs
- DataContractSerializer.cs
- PatternMatcher.cs
- ImageInfo.cs
- InstanceKeyView.cs
- FontCollection.cs
- XsdDuration.cs
- VideoDrawing.cs
- ColumnWidthChangingEvent.cs
- CellTreeSimplifier.cs
- WebPartMinimizeVerb.cs
- EdmSchemaAttribute.cs
- PropertyIDSet.cs
- SendOperation.cs
- OlePropertyStructs.cs
- FlowDocumentReader.cs
- mediapermission.cs
- DbParameterCollectionHelper.cs
- AppDomainGrammarProxy.cs
- ScriptResourceInfo.cs
- DocumentPageView.cs
- InfoCardKeyedHashAlgorithm.cs
- SortKey.cs
- TreeWalkHelper.cs
- SizeLimitedCache.cs
- RijndaelManaged.cs
- WorkflowCompensationBehavior.cs
- ExpressionBinding.cs
- ThreadSafeList.cs
- HttpVersion.cs
- SqlClientFactory.cs
- SystemTcpStatistics.cs
- ReceiveMessageRecord.cs
- PartialCachingAttribute.cs
- CalendarDay.cs
- URLMembershipCondition.cs
- AssemblyLoader.cs
- PreviewKeyDownEventArgs.cs
- ServiceSecurityAuditElement.cs