Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Activities / LocalService / CorrelationTokenTypeConvertor.cs / 1305376 / CorrelationTokenTypeConvertor.cs
using System; using System.Xml; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Runtime.Serialization; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Serialization; using System.Workflow.Runtime; using System.Globalization; namespace System.Workflow.Activities { internal sealed class CorrelationTokenTypeConverter : ExpandableObjectConverter { public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { return (sourceType == typeof(string)); } public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { return (destinationType == typeof(string)); } public override object ConvertFrom(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value) { object convertedValue = null; string correlatorName = value as String; if (!String.IsNullOrEmpty(correlatorName)) { foreach (object obj in GetStandardValues(context)) { CorrelationToken correlator = obj as CorrelationToken; if (correlator != null && correlator.Name == correlatorName) { convertedValue = correlator; break; } } if (convertedValue == null) convertedValue = new CorrelationToken(correlatorName); } return convertedValue; } public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destinationType) { object convertedValue = null; CorrelationToken correlator = value as CorrelationToken; if (destinationType == typeof(string) && correlator != null) convertedValue = correlator.Name; return convertedValue; } public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { ArrayList values = new ArrayList(); Activity activity = context.Instance as Activity; if (activity != null) { foreach (Activity preceedingActivity in GetPreceedingActivities(activity)) { PropertyDescriptor correlatorProperty = TypeDescriptor.GetProperties(preceedingActivity)["CorrelationToken"] as PropertyDescriptor; if (correlatorProperty != null) { CorrelationToken correlator = correlatorProperty.GetValue(preceedingActivity) as CorrelationToken; if (correlator != null && !values.Contains(correlator)) values.Add(correlator); } } } return new StandardValuesCollection(values); } public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes) { PropertyDescriptorCollection properties = base.GetProperties(context, value, attributes); ArrayList props = new ArrayList(properties); return new PropertyDescriptorCollection((PropertyDescriptor[])props.ToArray(typeof(PropertyDescriptor))); } // private IEnumerable GetPreceedingActivities(Activity startActivity) { Activity currentActivity = null; StackactivityStack = new Stack (); activityStack.Push(startActivity); while ((currentActivity = activityStack.Pop()) != null) { if (currentActivity.Parent != null) { foreach (Activity siblingActivity in currentActivity.Parent.Activities) { if (siblingActivity == currentActivity) break; if (siblingActivity.Enabled) { if (siblingActivity is CompositeActivity) { foreach (Activity containedActivity in GetContainedActivities((CompositeActivity)siblingActivity)) yield return containedActivity; } else { yield return siblingActivity; } } } } activityStack.Push(currentActivity.Parent); } yield break; } private IEnumerable GetContainedActivities(CompositeActivity activity) { if (!activity.Enabled) yield break; foreach (Activity containedActivity in activity.Activities) { if (containedActivity is CompositeActivity) { foreach (Activity nestedActivity in GetContainedActivities((CompositeActivity)containedActivity)) { if (nestedActivity.Enabled) yield return nestedActivity; } } else { if (containedActivity.Enabled) yield return containedActivity; } } yield break; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Xml; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Runtime.Serialization; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Serialization; using System.Workflow.Runtime; using System.Globalization; namespace System.Workflow.Activities { internal sealed class CorrelationTokenTypeConverter : ExpandableObjectConverter { public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { return (sourceType == typeof(string)); } public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { return (destinationType == typeof(string)); } public override object ConvertFrom(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value) { object convertedValue = null; string correlatorName = value as String; if (!String.IsNullOrEmpty(correlatorName)) { foreach (object obj in GetStandardValues(context)) { CorrelationToken correlator = obj as CorrelationToken; if (correlator != null && correlator.Name == correlatorName) { convertedValue = correlator; break; } } if (convertedValue == null) convertedValue = new CorrelationToken(correlatorName); } return convertedValue; } public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destinationType) { object convertedValue = null; CorrelationToken correlator = value as CorrelationToken; if (destinationType == typeof(string) && correlator != null) convertedValue = correlator.Name; return convertedValue; } public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { ArrayList values = new ArrayList(); Activity activity = context.Instance as Activity; if (activity != null) { foreach (Activity preceedingActivity in GetPreceedingActivities(activity)) { PropertyDescriptor correlatorProperty = TypeDescriptor.GetProperties(preceedingActivity)["CorrelationToken"] as PropertyDescriptor; if (correlatorProperty != null) { CorrelationToken correlator = correlatorProperty.GetValue(preceedingActivity) as CorrelationToken; if (correlator != null && !values.Contains(correlator)) values.Add(correlator); } } } return new StandardValuesCollection(values); } public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes) { PropertyDescriptorCollection properties = base.GetProperties(context, value, attributes); ArrayList props = new ArrayList(properties); return new PropertyDescriptorCollection((PropertyDescriptor[])props.ToArray(typeof(PropertyDescriptor))); } // private IEnumerable GetPreceedingActivities(Activity startActivity) { Activity currentActivity = null; Stack activityStack = new Stack (); activityStack.Push(startActivity); while ((currentActivity = activityStack.Pop()) != null) { if (currentActivity.Parent != null) { foreach (Activity siblingActivity in currentActivity.Parent.Activities) { if (siblingActivity == currentActivity) break; if (siblingActivity.Enabled) { if (siblingActivity is CompositeActivity) { foreach (Activity containedActivity in GetContainedActivities((CompositeActivity)siblingActivity)) yield return containedActivity; } else { yield return siblingActivity; } } } } activityStack.Push(currentActivity.Parent); } yield break; } private IEnumerable GetContainedActivities(CompositeActivity activity) { if (!activity.Enabled) yield break; foreach (Activity containedActivity in activity.Activities) { if (containedActivity is CompositeActivity) { foreach (Activity nestedActivity in GetContainedActivities((CompositeActivity)containedActivity)) { if (nestedActivity.Enabled) yield return nestedActivity; } } else { if (containedActivity.Enabled) yield return containedActivity; } } yield break; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
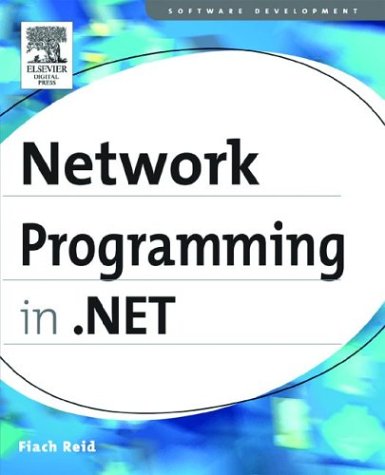
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Scalars.cs
- TableColumnCollection.cs
- ButtonFieldBase.cs
- ThumbAutomationPeer.cs
- activationcontext.cs
- WebBaseEventKeyComparer.cs
- SQLDoubleStorage.cs
- StdValidatorsAndConverters.cs
- UpdatePanelControlTrigger.cs
- AsymmetricKeyExchangeFormatter.cs
- TextSelectionHighlightLayer.cs
- WindowsGraphics.cs
- OdbcUtils.cs
- TypeUnloadedException.cs
- ConfigurationSettings.cs
- DynamicRenderer.cs
- TableLayoutSettings.cs
- Point4DValueSerializer.cs
- WebPartDisplayModeEventArgs.cs
- Pair.cs
- ObjectTypeMapping.cs
- Error.cs
- TypedTableHandler.cs
- SmtpSection.cs
- DataTable.cs
- Vector3DCollection.cs
- ObjectDataSourceStatusEventArgs.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- RemoteWebConfigurationHostStream.cs
- EventToken.cs
- SpecularMaterial.cs
- BrowserInteropHelper.cs
- HttpGetProtocolImporter.cs
- DuplicateWaitObjectException.cs
- SqlBulkCopyColumnMappingCollection.cs
- UInt32.cs
- OneOfScalarConst.cs
- ControlBindingsCollection.cs
- Registry.cs
- XmlDataProvider.cs
- SafeCancelMibChangeNotify.cs
- PartialTrustHelpers.cs
- RequestCacheManager.cs
- HelpInfo.cs
- SafeLocalMemHandle.cs
- GridPattern.cs
- ViewGenResults.cs
- XmlSubtreeReader.cs
- RSAPKCS1SignatureFormatter.cs
- FunctionUpdateCommand.cs
- ClientCultureInfo.cs
- DataGridViewColumnConverter.cs
- MsdtcClusterUtils.cs
- UriScheme.cs
- KoreanCalendar.cs
- DateTimeConverter2.cs
- InstanceDataCollection.cs
- ListViewItemSelectionChangedEvent.cs
- AdapterUtil.cs
- CodeGenerator.cs
- SystemFonts.cs
- EpmSyndicationContentDeSerializer.cs
- DesigntimeLicenseContext.cs
- ServiceOperationParameter.cs
- SqlClientPermission.cs
- ZoneLinkButton.cs
- CollectionEditorDialog.cs
- MarkupCompilePass2.cs
- WmpBitmapEncoder.cs
- ErrorHandlingReceiver.cs
- TextBreakpoint.cs
- TouchFrameEventArgs.cs
- GridView.cs
- Matrix.cs
- ObjectDataSourceView.cs
- HtmlUtf8RawTextWriter.cs
- ZipQueryOperator.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- DbParameterHelper.cs
- ReadOnlyDictionary.cs
- PopupControlService.cs
- Decoder.cs
- ObjectSecurityT.cs
- DoubleKeyFrameCollection.cs
- SerializerProvider.cs
- ThaiBuddhistCalendar.cs
- ZipIOExtraFieldElement.cs
- HtmlTableCell.cs
- TaskCanceledException.cs
- UnmanagedMarshal.cs
- DesignerForm.cs
- ControlDesigner.cs
- ContextMarshalException.cs
- BaseResourcesBuildProvider.cs
- MimeBasePart.cs
- RuleSettingsCollection.cs
- ContextDataSource.cs
- ClientConfigurationHost.cs
- AsyncWaitHandle.cs
- CodeObjectCreateExpression.cs