Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / Dom / XmlDeclaration.cs / 1 / XmlDeclaration.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System.Text; using System.Diagnostics; // Represents the xml declaration nodes: public class XmlDeclaration : XmlLinkedNode { const string YES = "yes"; const string NO = "no"; const string VERNUM = "1.0"; private string encoding; private string standalone; protected internal XmlDeclaration( string version, string encoding, string standalone, XmlDocument doc ) : base( doc ) { if ( version != VERNUM ) throw new ArgumentException( Res.GetString( Res.Xdom_Version ) ); if( ( standalone != null ) && ( standalone.Length > 0 ) ) if ( ( standalone != YES ) && ( standalone != NO ) ) throw new ArgumentException( Res.GetString(Res.Xdom_standalone, standalone) ); this.Encoding = encoding; this.Standalone = standalone; } // The version attribute (1.0) for public string Version { get { return VERNUM; } } // Specifies the value of the encoding attribute, as for // public string Encoding { get { return this.encoding; } set { this.encoding = ( (value == null) ? String.Empty : value ); } } // Specifies the value of the standalone attribute. public string Standalone { get { return this.standalone; } set { if ( value == null ) this.standalone = String.Empty; else if ( value.Length == 0 || value == YES || value == NO ) this.standalone = value; else throw new ArgumentException( Res.GetString(Res.Xdom_standalone, value) ); } } public override String Value { get { return InnerText; } set { InnerText = value; } } // Gets or sets the concatenated values of the node and // all its children. public override string InnerText { get { StringBuilder strb = new StringBuilder("version=\"" + Version + "\""); if ( Encoding.Length > 0 ) { strb.Append(" encoding=\""); strb.Append(Encoding); strb.Append("\""); } if ( Standalone.Length > 0 ) { strb.Append(" standalone=\""); strb.Append(Standalone); strb.Append("\""); } return strb.ToString(); } set { string tempVersion = null; string tempEncoding = null; string tempStandalone = null; string orgEncoding = this.Encoding; string orgStandalone = this.Standalone; XmlLoader.ParseXmlDeclarationValue( value, out tempVersion, out tempEncoding, out tempStandalone ); try { if ( tempVersion != null && tempVersion != VERNUM ) throw new ArgumentException(Res.GetString(Res.Xdom_Version)); if ( tempEncoding != null ) Encoding = tempEncoding; if ( tempStandalone != null ) Standalone = tempStandalone; } catch { Encoding = orgEncoding; Standalone = orgStandalone; throw; } } } //override methods and properties from XmlNode // Gets the name of the node. public override String Name { get { return "xml"; } } // Gets the name of the current node without the namespace prefix. public override string LocalName { get { return Name;} } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.XmlDeclaration;} } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateXmlDeclaration( Version, Encoding, Standalone ); } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteProcessingInstruction(Name, InnerText); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing since the node doesn't have children. } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System.Text; using System.Diagnostics; // Represents the xml declaration nodes: public class XmlDeclaration : XmlLinkedNode { const string YES = "yes"; const string NO = "no"; const string VERNUM = "1.0"; private string encoding; private string standalone; protected internal XmlDeclaration( string version, string encoding, string standalone, XmlDocument doc ) : base( doc ) { if ( version != VERNUM ) throw new ArgumentException( Res.GetString( Res.Xdom_Version ) ); if( ( standalone != null ) && ( standalone.Length > 0 ) ) if ( ( standalone != YES ) && ( standalone != NO ) ) throw new ArgumentException( Res.GetString(Res.Xdom_standalone, standalone) ); this.Encoding = encoding; this.Standalone = standalone; } // The version attribute (1.0) for public string Version { get { return VERNUM; } } // Specifies the value of the encoding attribute, as for // public string Encoding { get { return this.encoding; } set { this.encoding = ( (value == null) ? String.Empty : value ); } } // Specifies the value of the standalone attribute. public string Standalone { get { return this.standalone; } set { if ( value == null ) this.standalone = String.Empty; else if ( value.Length == 0 || value == YES || value == NO ) this.standalone = value; else throw new ArgumentException( Res.GetString(Res.Xdom_standalone, value) ); } } public override String Value { get { return InnerText; } set { InnerText = value; } } // Gets or sets the concatenated values of the node and // all its children. public override string InnerText { get { StringBuilder strb = new StringBuilder("version=\"" + Version + "\""); if ( Encoding.Length > 0 ) { strb.Append(" encoding=\""); strb.Append(Encoding); strb.Append("\""); } if ( Standalone.Length > 0 ) { strb.Append(" standalone=\""); strb.Append(Standalone); strb.Append("\""); } return strb.ToString(); } set { string tempVersion = null; string tempEncoding = null; string tempStandalone = null; string orgEncoding = this.Encoding; string orgStandalone = this.Standalone; XmlLoader.ParseXmlDeclarationValue( value, out tempVersion, out tempEncoding, out tempStandalone ); try { if ( tempVersion != null && tempVersion != VERNUM ) throw new ArgumentException(Res.GetString(Res.Xdom_Version)); if ( tempEncoding != null ) Encoding = tempEncoding; if ( tempStandalone != null ) Standalone = tempStandalone; } catch { Encoding = orgEncoding; Standalone = orgStandalone; throw; } } } //override methods and properties from XmlNode // Gets the name of the node. public override String Name { get { return "xml"; } } // Gets the name of the current node without the namespace prefix. public override string LocalName { get { return Name;} } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.XmlDeclaration;} } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateXmlDeclaration( Version, Encoding, Standalone ); } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteProcessingInstruction(Name, InnerText); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing since the node doesn't have children. } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
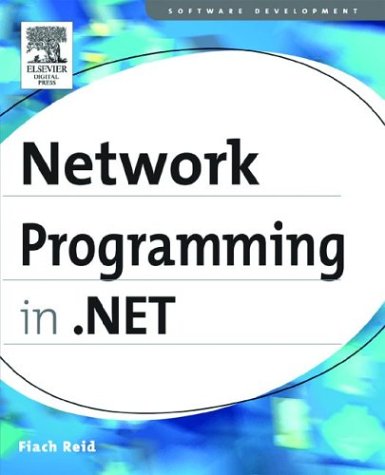
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeViewImageIndexConverter.cs
- Closure.cs
- ContentElementAutomationPeer.cs
- EmptyStringExpandableObjectConverter.cs
- fixedPageContentExtractor.cs
- ReliableChannelBinder.cs
- MultiDataTrigger.cs
- AnonymousIdentificationModule.cs
- OpCopier.cs
- OleDbCommand.cs
- TextPattern.cs
- DataServiceContext.cs
- FocusTracker.cs
- DataTableNewRowEvent.cs
- SinglePhaseEnlistment.cs
- CodeTypeDeclaration.cs
- ActivityInstance.cs
- UrlMappingsModule.cs
- Wildcard.cs
- BaseValidator.cs
- ToolboxItemWrapper.cs
- SafeNativeMethods.cs
- ApplicationInterop.cs
- FixUpCollection.cs
- ConnectionPointCookie.cs
- KeyEventArgs.cs
- FixedElement.cs
- DropDownList.cs
- ConfigLoader.cs
- MenuCommand.cs
- CompilerInfo.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- ExpressionBuilderContext.cs
- ShortcutKeysEditor.cs
- ObjectStateFormatter.cs
- InstancePersistenceContext.cs
- DoubleUtil.cs
- SqlRewriteScalarSubqueries.cs
- CounterCreationData.cs
- XmlEnumAttribute.cs
- Method.cs
- DocumentSchemaValidator.cs
- NameNode.cs
- CodeVariableReferenceExpression.cs
- BindingsCollection.cs
- DomainUpDown.cs
- HttpCachePolicyBase.cs
- NamespaceList.cs
- CodeDirectiveCollection.cs
- MaterialCollection.cs
- KeyboardNavigation.cs
- XmlSerializerFactory.cs
- BamlLocalizableResourceKey.cs
- Int64Storage.cs
- HttpHandlerActionCollection.cs
- StrongNamePublicKeyBlob.cs
- CodeThrowExceptionStatement.cs
- InputEventArgs.cs
- ContentPlaceHolder.cs
- ProvidersHelper.cs
- TextProviderWrapper.cs
- SequenceFullException.cs
- DataReceivedEventArgs.cs
- ContentType.cs
- BasePattern.cs
- Rotation3DAnimationBase.cs
- CultureInfoConverter.cs
- EventData.cs
- StreamInfo.cs
- InputReport.cs
- TimelineClockCollection.cs
- ParameterToken.cs
- DataBindEngine.cs
- DataGridRelationshipRow.cs
- PreservationFileWriter.cs
- MessageBox.cs
- CatalogZoneAutoFormat.cs
- Atom10FormatterFactory.cs
- WorkflowApplicationIdleEventArgs.cs
- WebPart.cs
- HttpCacheVaryByContentEncodings.cs
- AdRotator.cs
- StreamReader.cs
- DataStreams.cs
- OpCodes.cs
- ListDictionaryInternal.cs
- DataServiceBuildProvider.cs
- TypeHelpers.cs
- WindowCollection.cs
- SecondaryViewProvider.cs
- ListViewPagedDataSource.cs
- PrintingPermissionAttribute.cs
- MouseOverProperty.cs
- HtmlShimManager.cs
- RequiredAttributeAttribute.cs
- TdsEnums.cs
- XmlSchemaSimpleType.cs
- TextWriter.cs
- ServiceBehaviorElement.cs
- LicenseContext.cs