Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SamlAuthorityBinding.cs / 1305376 / SamlAuthorityBinding.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Xml; using System.Xml.Serialization; using System.Runtime.Serialization; using System.IdentityModel.Selectors; [DataContract] public class SamlAuthorityBinding { XmlQualifiedName authorityKind; string binding; string location; [DataMember] bool isReadOnly = false; public SamlAuthorityBinding(XmlQualifiedName authorityKind, string binding, string location) { this.AuthorityKind = authorityKind; this.Binding = binding; this.Location = location; CheckObjectValidity(); } public SamlAuthorityBinding() { } [DataMember] public XmlQualifiedName AuthorityKind { get {return this.authorityKind; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (value == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); if (String.IsNullOrEmpty(value.Name)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLAuthorityKindMissingName)); this.authorityKind = value; } } [DataMember] public string Binding { get {return this.binding; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (String.IsNullOrEmpty(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLAuthorityBindingRequiresBinding)); this.binding = value; } } [DataMember] public string Location { get {return this.location; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (String.IsNullOrEmpty(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLAuthorityBindingRequiresLocation)); this.location = value; } } public bool IsReadOnly { get { return this.isReadOnly; } } public void MakeReadOnly() { this.isReadOnly = true; } void CheckObjectValidity() { if (this.authorityKind == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingAuthorityKind))); if (String.IsNullOrEmpty(authorityKind.Name)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityKindMissingName))); if (String.IsNullOrEmpty(this.binding)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingRequiresBinding))); if (String.IsNullOrEmpty(this.location)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingRequiresLocation))); } public virtual void ReadXml(XmlDictionaryReader reader, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; string authKind = reader.GetAttribute(dictionary.AuthorityKind, null); if (String.IsNullOrEmpty(authKind)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingAuthorityKindOnRead))); string[] authKindParts = authKind.Split(':'); if (authKindParts.Length > 2) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingInvalidAuthorityKind))); string localName; string prefix; string nameSpace; if (authKindParts.Length == 2) { prefix = authKindParts[0]; localName = authKindParts[1]; } else { prefix = String.Empty; localName = authKindParts[0]; } nameSpace = reader.LookupNamespace(prefix); this.authorityKind = new XmlQualifiedName(localName, nameSpace); this.binding = reader.GetAttribute(dictionary.Binding, null); if (String.IsNullOrEmpty(this.binding)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingBindingOnRead))); this.location = reader.GetAttribute(dictionary.Location, null); if (String.IsNullOrEmpty(this.location)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingLocationOnRead))); if (reader.IsEmptyElement) { reader.MoveToContent(); reader.Read(); } else { reader.MoveToContent(); reader.Read(); reader.ReadEndElement(); } } public virtual void WriteXml(XmlDictionaryWriter writer, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer) { CheckObjectValidity(); if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; writer.WriteStartElement(dictionary.PreferredPrefix.Value, dictionary.AuthorityBinding, dictionary.Namespace); string prefix = null; if (!String.IsNullOrEmpty(this.authorityKind.Namespace)) { writer.WriteAttributeString(String.Empty, dictionary.NamespaceAttributePrefix.Value, null, this.authorityKind.Namespace); prefix = writer.LookupPrefix(this.authorityKind.Namespace); } writer.WriteStartAttribute(dictionary.AuthorityKind, null); if (String.IsNullOrEmpty(prefix)) writer.WriteString(this.authorityKind.Name); else writer.WriteString(prefix + ":" + this.authorityKind.Name); writer.WriteEndAttribute(); writer.WriteStartAttribute(dictionary.Location, null); writer.WriteString(this.location); writer.WriteEndAttribute(); writer.WriteStartAttribute(dictionary.Binding, null); writer.WriteString(this.binding); writer.WriteEndAttribute(); writer.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Xml; using System.Xml.Serialization; using System.Runtime.Serialization; using System.IdentityModel.Selectors; [DataContract] public class SamlAuthorityBinding { XmlQualifiedName authorityKind; string binding; string location; [DataMember] bool isReadOnly = false; public SamlAuthorityBinding(XmlQualifiedName authorityKind, string binding, string location) { this.AuthorityKind = authorityKind; this.Binding = binding; this.Location = location; CheckObjectValidity(); } public SamlAuthorityBinding() { } [DataMember] public XmlQualifiedName AuthorityKind { get {return this.authorityKind; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (value == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); if (String.IsNullOrEmpty(value.Name)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLAuthorityKindMissingName)); this.authorityKind = value; } } [DataMember] public string Binding { get {return this.binding; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (String.IsNullOrEmpty(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLAuthorityBindingRequiresBinding)); this.binding = value; } } [DataMember] public string Location { get {return this.location; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (String.IsNullOrEmpty(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLAuthorityBindingRequiresLocation)); this.location = value; } } public bool IsReadOnly { get { return this.isReadOnly; } } public void MakeReadOnly() { this.isReadOnly = true; } void CheckObjectValidity() { if (this.authorityKind == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingAuthorityKind))); if (String.IsNullOrEmpty(authorityKind.Name)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityKindMissingName))); if (String.IsNullOrEmpty(this.binding)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingRequiresBinding))); if (String.IsNullOrEmpty(this.location)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingRequiresLocation))); } public virtual void ReadXml(XmlDictionaryReader reader, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; string authKind = reader.GetAttribute(dictionary.AuthorityKind, null); if (String.IsNullOrEmpty(authKind)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingAuthorityKindOnRead))); string[] authKindParts = authKind.Split(':'); if (authKindParts.Length > 2) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingInvalidAuthorityKind))); string localName; string prefix; string nameSpace; if (authKindParts.Length == 2) { prefix = authKindParts[0]; localName = authKindParts[1]; } else { prefix = String.Empty; localName = authKindParts[0]; } nameSpace = reader.LookupNamespace(prefix); this.authorityKind = new XmlQualifiedName(localName, nameSpace); this.binding = reader.GetAttribute(dictionary.Binding, null); if (String.IsNullOrEmpty(this.binding)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingBindingOnRead))); this.location = reader.GetAttribute(dictionary.Location, null); if (String.IsNullOrEmpty(this.location)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingLocationOnRead))); if (reader.IsEmptyElement) { reader.MoveToContent(); reader.Read(); } else { reader.MoveToContent(); reader.Read(); reader.ReadEndElement(); } } public virtual void WriteXml(XmlDictionaryWriter writer, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer) { CheckObjectValidity(); if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; writer.WriteStartElement(dictionary.PreferredPrefix.Value, dictionary.AuthorityBinding, dictionary.Namespace); string prefix = null; if (!String.IsNullOrEmpty(this.authorityKind.Namespace)) { writer.WriteAttributeString(String.Empty, dictionary.NamespaceAttributePrefix.Value, null, this.authorityKind.Namespace); prefix = writer.LookupPrefix(this.authorityKind.Namespace); } writer.WriteStartAttribute(dictionary.AuthorityKind, null); if (String.IsNullOrEmpty(prefix)) writer.WriteString(this.authorityKind.Name); else writer.WriteString(prefix + ":" + this.authorityKind.Name); writer.WriteEndAttribute(); writer.WriteStartAttribute(dictionary.Location, null); writer.WriteString(this.location); writer.WriteEndAttribute(); writer.WriteStartAttribute(dictionary.Binding, null); writer.WriteString(this.binding); writer.WriteEndAttribute(); writer.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
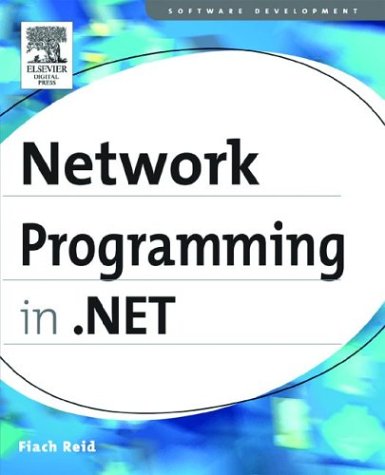
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- X509RecipientCertificateServiceElement.cs
- EnumerableRowCollection.cs
- XmlSecureResolver.cs
- Currency.cs
- HMACSHA1.cs
- InfiniteTimeSpanConverter.cs
- LOSFormatter.cs
- RsaKeyIdentifierClause.cs
- CSharpCodeProvider.cs
- HttpPostProtocolImporter.cs
- FileDialog.cs
- _UriSyntax.cs
- System.Data_BID.cs
- RangeBaseAutomationPeer.cs
- RawStylusActions.cs
- CategoryNameCollection.cs
- EncoderNLS.cs
- DesignerVerbCollection.cs
- ExpressionBindings.cs
- TailCallAnalyzer.cs
- CompilerTypeWithParams.cs
- LicenseException.cs
- SatelliteContractVersionAttribute.cs
- MatcherBuilder.cs
- DataGridAutoFormatDialog.cs
- ContourSegment.cs
- DebugView.cs
- EntityDataSourceQueryBuilder.cs
- CultureSpecificStringDictionary.cs
- mediaeventargs.cs
- SmiTypedGetterSetter.cs
- ImageSource.cs
- EventArgs.cs
- SEHException.cs
- Win32PrintDialog.cs
- SqlMethods.cs
- ArgumentOutOfRangeException.cs
- StringUtil.cs
- PropagatorResult.cs
- ContainerParagraph.cs
- EntityDataSourceDataSelection.cs
- WriteFileContext.cs
- StringAnimationUsingKeyFrames.cs
- CompilationSection.cs
- BamlRecordReader.cs
- ListBindableAttribute.cs
- OleDbDataReader.cs
- PersonalizationStateInfo.cs
- DataGridColumnHeaderAutomationPeer.cs
- ChannelDispatcherBase.cs
- OperatingSystem.cs
- AnimationClockResource.cs
- embossbitmapeffect.cs
- CodeDirectionExpression.cs
- XhtmlBasicLabelAdapter.cs
- BrowserDefinition.cs
- TextEffectResolver.cs
- ADMembershipProvider.cs
- ComplexPropertyEntry.cs
- GenericEnumerator.cs
- PersonalizationEntry.cs
- FontFaceLayoutInfo.cs
- ObjectStateFormatter.cs
- EntityDataSourceChangedEventArgs.cs
- ZipPackagePart.cs
- HttpProxyTransportBindingElement.cs
- BitmapMetadataBlob.cs
- DispatcherExceptionEventArgs.cs
- WebPartTransformer.cs
- HealthMonitoringSection.cs
- SynchronizationContext.cs
- MulticastIPAddressInformationCollection.cs
- TextRangeProviderWrapper.cs
- RepeatBehaviorConverter.cs
- URLMembershipCondition.cs
- Adorner.cs
- DragDeltaEventArgs.cs
- WebPartsPersonalizationAuthorization.cs
- FactoryGenerator.cs
- SymmetricSecurityBindingElement.cs
- BitmapScalingModeValidation.cs
- NameTable.cs
- TypographyProperties.cs
- DataSourceCache.cs
- ArrayElementGridEntry.cs
- WinCategoryAttribute.cs
- CatalogPart.cs
- BitmapCacheBrush.cs
- InternalConfigConfigurationFactory.cs
- OrderByQueryOptionExpression.cs
- SiteMapNode.cs
- MimeFormatExtensions.cs
- ConnectionProviderAttribute.cs
- PropertyChangedEventArgs.cs
- EmissiveMaterial.cs
- RuntimeEnvironment.cs
- RowBinding.cs
- DataControlPagerLinkButton.cs
- Logging.cs
- GridViewColumnCollectionChangedEventArgs.cs