Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Design / PropertyValueUIItem.cs / 1 / PropertyValueUIItem.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing.Design { using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.Drawing; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class PropertyValueUIItem { ///Provides information about the property value UI including the invoke /// handler, tool tip, and the glyph icon to be displayed on the property /// browser. ////// /// The image to display for this. Must be 8x8 /// private Image itemImage; ////// /// The handler to fire if this item is double clicked. /// private PropertyValueUIItemInvokeHandler handler; ////// /// The tooltip for this item. /// private string tooltip; ////// /// public PropertyValueUIItem(Image uiItemImage, PropertyValueUIItemInvokeHandler handler, string tooltip){ this.itemImage = uiItemImage; this.handler = handler; if (itemImage == null) { throw new ArgumentNullException("uiItemImage"); } if (handler == null) { throw new ArgumentNullException("handler"); } this.tooltip = tooltip; } ///Initiailzes a new instance of the ///class. /// /// public virtual Image Image { get { return itemImage; } } ///Gets or sets /// the 8x8 pixel image that will be drawn on the properties window. ////// /// public virtual PropertyValueUIItemInvokeHandler InvokeHandler { get { return handler; } } ///Gets or sets the handler that will be raised when this item is double clicked. ////// /// public virtual string ToolTip { get { return tooltip; } } ///Gets or sets the /// tool tip to display for this item. ////// /// public virtual void Reset(){ } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Resets the UI item. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing.Design { using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.Drawing; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class PropertyValueUIItem { ///Provides information about the property value UI including the invoke /// handler, tool tip, and the glyph icon to be displayed on the property /// browser. ////// /// The image to display for this. Must be 8x8 /// private Image itemImage; ////// /// The handler to fire if this item is double clicked. /// private PropertyValueUIItemInvokeHandler handler; ////// /// The tooltip for this item. /// private string tooltip; ////// /// public PropertyValueUIItem(Image uiItemImage, PropertyValueUIItemInvokeHandler handler, string tooltip){ this.itemImage = uiItemImage; this.handler = handler; if (itemImage == null) { throw new ArgumentNullException("uiItemImage"); } if (handler == null) { throw new ArgumentNullException("handler"); } this.tooltip = tooltip; } ///Initiailzes a new instance of the ///class. /// /// public virtual Image Image { get { return itemImage; } } ///Gets or sets /// the 8x8 pixel image that will be drawn on the properties window. ////// /// public virtual PropertyValueUIItemInvokeHandler InvokeHandler { get { return handler; } } ///Gets or sets the handler that will be raised when this item is double clicked. ////// /// public virtual string ToolTip { get { return tooltip; } } ///Gets or sets the /// tool tip to display for this item. ////// /// public virtual void Reset(){ } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Resets the UI item. ///
Link Menu
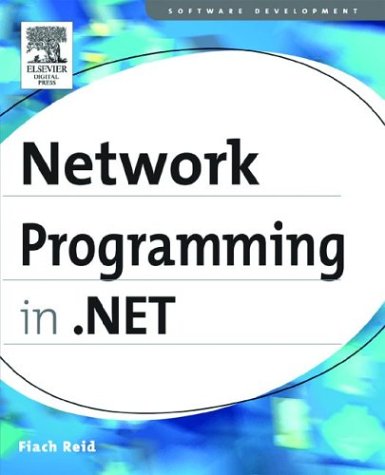
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebServiceBindingAttribute.cs
- AuthenticationSection.cs
- ProcessDesigner.cs
- ScriptResourceAttribute.cs
- BaseTransportHeaders.cs
- Interlocked.cs
- NamedPipeAppDomainProtocolHandler.cs
- SafeSecurityHandles.cs
- QualifiedCellIdBoolean.cs
- ReadOnlyDictionary.cs
- XmlSchemaSimpleType.cs
- SqlExpander.cs
- RemotingConfiguration.cs
- StylusPlugin.cs
- WinFormsUtils.cs
- SaveFileDialog.cs
- DragStartedEventArgs.cs
- FindSimilarActivitiesVerb.cs
- PersonalizationStateInfoCollection.cs
- PageTheme.cs
- DataGridItemCollection.cs
- NetWebProxyFinder.cs
- XmlBinaryReaderSession.cs
- HttpWriter.cs
- MULTI_QI.cs
- DocumentGridContextMenu.cs
- PresentationSource.cs
- SqlMultiplexer.cs
- SqlWebEventProvider.cs
- Native.cs
- _TimerThread.cs
- RNGCryptoServiceProvider.cs
- DataGridViewDataErrorEventArgs.cs
- StringPropertyBuilder.cs
- WebPartCatalogCloseVerb.cs
- shaperfactory.cs
- FindSimilarActivitiesVerb.cs
- ToolStripControlHost.cs
- RijndaelManagedTransform.cs
- DataGridViewCellStyleChangedEventArgs.cs
- StringAnimationBase.cs
- WindowsFont.cs
- SQLMoneyStorage.cs
- StringUtil.cs
- UserControlCodeDomTreeGenerator.cs
- WebPartEditorOkVerb.cs
- MultitargetUtil.cs
- tooltip.cs
- SchemaTableOptionalColumn.cs
- UnsupportedPolicyOptionsException.cs
- PageRequestManager.cs
- LoadWorkflowByInstanceKeyCommand.cs
- XsltSettings.cs
- Console.cs
- DesignerTransaction.cs
- ParseHttpDate.cs
- PrintPageEvent.cs
- DbDataReader.cs
- MatrixAnimationUsingPath.cs
- TokenBasedSetEnumerator.cs
- ThreadLocal.cs
- PasswordRecovery.cs
- DateTimeOffsetConverter.cs
- DoubleLinkList.cs
- Maps.cs
- VisemeEventArgs.cs
- ClrProviderManifest.cs
- SystemColors.cs
- HttpRuntime.cs
- DesignerTransactionCloseEvent.cs
- ZipIOLocalFileBlock.cs
- MonitoringDescriptionAttribute.cs
- BevelBitmapEffect.cs
- assemblycache.cs
- DbConnectionClosed.cs
- EntityContainerEmitter.cs
- SiteMapDataSource.cs
- DiagnosticStrings.cs
- RankException.cs
- StylusCaptureWithinProperty.cs
- ParserStack.cs
- XmlSerializerVersionAttribute.cs
- XPathMessageFilterElementCollection.cs
- XmlSchemaParticle.cs
- LineGeometry.cs
- DynamicILGenerator.cs
- NativeMethods.cs
- RotateTransform.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- PnrpPeerResolverBindingElement.cs
- ListDesigner.cs
- PersonalizationProviderHelper.cs
- FullTrustAssembly.cs
- UnaryNode.cs
- StylusEditingBehavior.cs
- WizardPanelChangingEventArgs.cs
- URLString.cs
- WMIInterop.cs
- CodeMemberEvent.cs
- DependencyObject.cs