Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Configuration / XPathMessageFilterElementCollection.cs / 1 / XPathMessageFilterElementCollection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Configuration { using System; using System.ServiceModel.Dispatcher; using System.Configuration; using System.Globalization; using System.ServiceModel; [ConfigurationCollection(typeof(XPathMessageFilterElement))] public sealed class XPathMessageFilterElementCollection : ServiceModelConfigurationElementCollection{ public XPathMessageFilterElementCollection() : base(ConfigurationElementCollectionType.AddRemoveClearMap, null, new XPathMessageFilterElementComparer()) { } public override bool ContainsKey(object key) { if (key == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("key"); } string newKey = string.Empty; if (key.GetType().IsAssignableFrom(typeof(XPathMessageFilter))) { newKey = XPathMessageFilterElementComparer.ParseXPathString((XPathMessageFilter)key); } else if (key.GetType().IsAssignableFrom(typeof(string))) { newKey = (string)key; } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException( SR.GetString(SR.ConfigInvalidKeyType, "XPathMessageFilterElement", typeof(XPathMessageFilter).AssemblyQualifiedName, key.GetType().AssemblyQualifiedName))); } return base.ContainsKey(newKey); } protected override Object GetElementKey(ConfigurationElement element) { if (element == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("element"); } XPathMessageFilterElement configElement = (XPathMessageFilterElement)element; if (configElement.Filter == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("element", SR.GetString(SR.ConfigXPathFilterIsNull)); } return XPathMessageFilterElementComparer.ParseXPathString(configElement.Filter); } protected override ConfigurationPropertyCollection Properties { get { return new ConfigurationPropertyCollection(); } } public override XPathMessageFilterElement this[object key] { get { if (key == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("key"); } if (!key.GetType().IsAssignableFrom(typeof(XPathMessageFilter))) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException( SR.GetString(SR.ConfigInvalidKeyType, "XPathMessageFilterElement", typeof(XPathMessageFilter).AssemblyQualifiedName, key.GetType().AssemblyQualifiedName))); } XPathMessageFilterElement retval = (XPathMessageFilterElement)this.BaseGet(key); if (retval == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new System.Collections.Generic.KeyNotFoundException( SR.GetString(SR.ConfigKeyNotFoundInElementCollection, key.ToString()))); } return retval; } set { if (this.IsReadOnly()) { Add(value); } if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } if (key == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("key"); } if (!key.GetType().IsAssignableFrom(typeof(XPathMessageFilter))) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException( SR.GetString(SR.ConfigInvalidKeyType, "XPathMessageFilterElement", typeof(XPathMessageFilter).AssemblyQualifiedName, key.GetType().AssemblyQualifiedName))); } string oldKey = XPathMessageFilterElementComparer.ParseXPathString((XPathMessageFilter)key); string newKey = (string)this.GetElementKey(value); if (String.Equals(oldKey, newKey, StringComparison.Ordinal)) { if (BaseGet(key) != null) { BaseRemove(key); } Add(value); } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.ConfigKeysDoNotMatch, this.GetElementKey(value).ToString(), key.ToString())); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
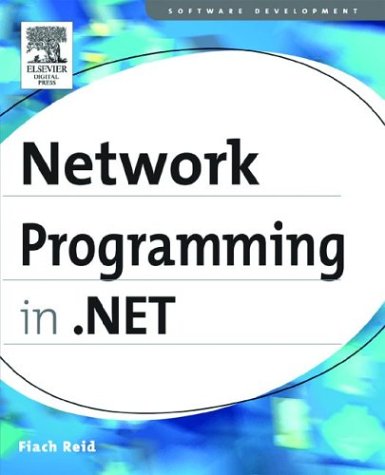
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AdjustableArrowCap.cs
- DataGridParentRows.cs
- ContentPlaceHolder.cs
- TableSectionStyle.cs
- TemplateXamlParser.cs
- HandlerBase.cs
- DefaultDialogButtons.cs
- DocumentPageView.cs
- QueryCreatedEventArgs.cs
- validationstate.cs
- ItemMap.cs
- AnnotationHighlightLayer.cs
- MarshalByRefObject.cs
- VirtualPathUtility.cs
- CodeGotoStatement.cs
- DataGridHeaderBorder.cs
- OdbcConnectionHandle.cs
- AttributeCollection.cs
- BoolExpression.cs
- ControlBindingsCollection.cs
- XmlConverter.cs
- ConfigXmlComment.cs
- ApplicationBuildProvider.cs
- StringBuilder.cs
- MouseCaptureWithinProperty.cs
- SupportingTokenChannel.cs
- LastQueryOperator.cs
- MenuItem.cs
- DES.cs
- TextInfo.cs
- ClientBuildManagerCallback.cs
- regiisutil.cs
- XmlSchemaGroupRef.cs
- Pts.cs
- SocketElement.cs
- LinqDataSource.cs
- CompiledAction.cs
- _SecureChannel.cs
- ConvertEvent.cs
- RestHandler.cs
- WebBrowserBase.cs
- TraceProvider.cs
- RangeBaseAutomationPeer.cs
- DataTableNewRowEvent.cs
- SharedRuntimeState.cs
- AspCompat.cs
- FormsAuthenticationEventArgs.cs
- Visual.cs
- ManagementClass.cs
- MemberDescriptor.cs
- UInt64.cs
- SqlRemoveConstantOrderBy.cs
- FileInfo.cs
- GraphicsPathIterator.cs
- SoapMessage.cs
- ErrorTableItemStyle.cs
- LinkedResourceCollection.cs
- EventListenerClientSide.cs
- CompiledQuery.cs
- DataSpaceManager.cs
- WebPartTransformerCollection.cs
- Figure.cs
- DataGridRelationshipRow.cs
- SqlClientWrapperSmiStreamChars.cs
- DefaultHttpHandler.cs
- BitmapEffectDrawing.cs
- RC2.cs
- EngineSiteSapi.cs
- StickyNoteContentControl.cs
- Group.cs
- IntSecurity.cs
- DynamicPhysicalDiscoSearcher.cs
- EffectiveValueEntry.cs
- DataConnectionHelper.cs
- DropShadowEffect.cs
- PopOutPanel.cs
- WebPartEditorApplyVerb.cs
- RawStylusInput.cs
- Button.cs
- ZipIOLocalFileHeader.cs
- ToolboxItemLoader.cs
- RowUpdatingEventArgs.cs
- ComponentManagerBroker.cs
- BaseUriHelper.cs
- PingOptions.cs
- CommonGetThemePartSize.cs
- FragmentQueryProcessor.cs
- ActivatableWorkflowsQueryResult.cs
- PopupRootAutomationPeer.cs
- TextBox.cs
- OdbcException.cs
- DataSourceHelper.cs
- OdbcPermission.cs
- WindowsSysHeader.cs
- MessageDecoder.cs
- SubMenuStyle.cs
- CodeFieldReferenceExpression.cs
- TypedTableBase.cs
- BindingNavigator.cs
- RtfToXamlLexer.cs