Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Byte.cs / 1 / Byte.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: Byte ** ** ** Purpose: This class will encapsulate a byte and provide an ** Object representation of it. ** ** ===========================================================*/ namespace System { using System; using System.Globalization; using System.Runtime.InteropServices; // The Byte class extends the Value class and // provides object representation of the byte primitive type. // [System.Runtime.InteropServices.ComVisible(true)] [Serializable, System.Runtime.InteropServices.StructLayout(LayoutKind.Sequential)] public struct Byte : IComparable, IFormattable, IConvertible #if GENERICS_WORK , IComparable, IEquatable #endif { private byte m_value; // The maximum value that a Byte may represent: 255. public const byte MaxValue = (byte)0xFF; // The minimum value that a Byte may represent: 0. public const byte MinValue = 0; // Compares this object to another object, returning an integer that // indicates the relationship. // Returns a value less than zero if this object // null is considered to be less than any instance. // If object is not of type byte, this method throws an ArgumentException. // public int CompareTo(Object value) { if (value == null) { return 1; } if (!(value is Byte)) { throw new ArgumentException(Environment.GetResourceString("Arg_MustBeByte")); } return m_value - (((Byte)value).m_value); } public int CompareTo(Byte value) { return m_value - value; } // Determines whether two Byte objects are equal. public override bool Equals(Object obj) { if (!(obj is Byte)) { return false; } return m_value == ((Byte)obj).m_value; } public bool Equals(Byte obj) { return m_value == obj; } // Gets a hash code for this instance. public override int GetHashCode() { return m_value; } public static byte Parse(String s) { return Parse(s, NumberStyles.Integer, NumberFormatInfo.CurrentInfo); } public static byte Parse(String s, NumberStyles style) { NumberFormatInfo.ValidateParseStyleInteger(style); return Parse(s, style, NumberFormatInfo.CurrentInfo); } public static byte Parse(String s, IFormatProvider provider) { return Parse(s, NumberStyles.Integer, NumberFormatInfo.GetInstance(provider)); } // Parses an unsigned byte from a String in the given style. If // a NumberFormatInfo isn't specified, the current culture's // NumberFormatInfo is assumed. public static byte Parse(String s, NumberStyles style, IFormatProvider provider) { NumberFormatInfo.ValidateParseStyleInteger(style); return Parse(s, style, NumberFormatInfo.GetInstance(provider)); } private static byte Parse(String s, NumberStyles style, NumberFormatInfo info) { int i = 0; try { i = Number.ParseInt32(s, style, info); } catch(OverflowException e) { throw new OverflowException(Environment.GetResourceString("Overflow_Byte"), e); } if (i < MinValue || i > MaxValue) throw new OverflowException(Environment.GetResourceString("Overflow_Byte")); return (byte)i; } public static bool TryParse(String s, out Byte result) { return TryParse(s, NumberStyles.Integer, NumberFormatInfo.CurrentInfo, out result); } public static bool TryParse(String s, NumberStyles style, IFormatProvider provider, out Byte result) { NumberFormatInfo.ValidateParseStyleInteger(style); return TryParse(s, style, NumberFormatInfo.GetInstance(provider), out result); } private static bool TryParse(String s, NumberStyles style, NumberFormatInfo info, out Byte result) { result = 0; int i; if (!Number.TryParseInt32(s, style, info, out i)) { return false; } if (i < MinValue || i > MaxValue) { return false; } result = (byte) i; return true; } public override String ToString() { return Number.FormatInt32(m_value, null, NumberFormatInfo.CurrentInfo); } public String ToString(String format) { return Number.FormatInt32(m_value, format, NumberFormatInfo.CurrentInfo); } public String ToString(IFormatProvider provider) { return Number.FormatInt32(m_value, null, NumberFormatInfo.GetInstance(provider)); } public String ToString(String format, IFormatProvider provider) { return Number.FormatInt32(m_value, format, NumberFormatInfo.GetInstance(provider)); } // // IValue implementation // public TypeCode GetTypeCode() { return TypeCode.Byte; } /// bool IConvertible.ToBoolean(IFormatProvider provider) { return Convert.ToBoolean(m_value); } /// char IConvertible.ToChar(IFormatProvider provider) { return Convert.ToChar(m_value); } /// sbyte IConvertible.ToSByte(IFormatProvider provider) { return Convert.ToSByte(m_value); } /// byte IConvertible.ToByte(IFormatProvider provider) { return m_value; } /// short IConvertible.ToInt16(IFormatProvider provider) { return Convert.ToInt16(m_value); } /// ushort IConvertible.ToUInt16(IFormatProvider provider) { return Convert.ToUInt16(m_value); } /// int IConvertible.ToInt32(IFormatProvider provider) { return Convert.ToInt32(m_value); } /// uint IConvertible.ToUInt32(IFormatProvider provider) { return Convert.ToUInt32(m_value); } /// long IConvertible.ToInt64(IFormatProvider provider) { return Convert.ToInt64(m_value); } /// ulong IConvertible.ToUInt64(IFormatProvider provider) { return Convert.ToUInt64(m_value); } /// float IConvertible.ToSingle(IFormatProvider provider) { return Convert.ToSingle(m_value); } /// double IConvertible.ToDouble(IFormatProvider provider) { return Convert.ToDouble(m_value); } /// Decimal IConvertible.ToDecimal(IFormatProvider provider) { return Convert.ToDecimal(m_value); } /// DateTime IConvertible.ToDateTime(IFormatProvider provider) { throw new InvalidCastException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("InvalidCast_FromTo"), "Byte", "DateTime")); } /// Object IConvertible.ToType(Type type, IFormatProvider provider) { return Convert.DefaultToType((IConvertible)this, type, provider); } } }
Link Menu
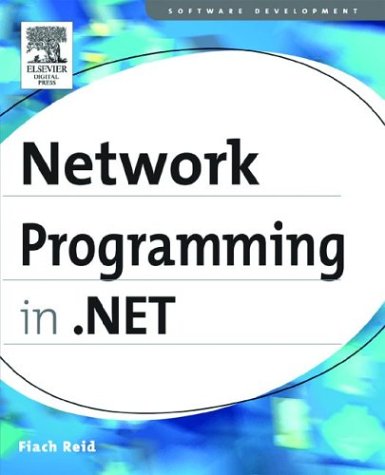
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ellipse.cs
- ClientBuildManager.cs
- TraceLevelStore.cs
- CallbackValidatorAttribute.cs
- OleDbInfoMessageEvent.cs
- ModelUIElement3D.cs
- X509ChainElement.cs
- HttpProfileBase.cs
- LicFileLicenseProvider.cs
- HttpModuleCollection.cs
- BaseTemplateParser.cs
- WebPartUserCapability.cs
- UIInitializationException.cs
- smtpconnection.cs
- NavigationExpr.cs
- DataViewSetting.cs
- EntityConnection.cs
- InstancePersistence.cs
- SmtpMail.cs
- XPathAncestorQuery.cs
- AuthenticationModulesSection.cs
- Latin1Encoding.cs
- UniqueSet.cs
- ToolStripSettings.cs
- AnimatedTypeHelpers.cs
- XmlStreamStore.cs
- Nullable.cs
- MultiByteCodec.cs
- DocumentGridPage.cs
- PingReply.cs
- XmlObjectSerializerReadContextComplexJson.cs
- WebPartHelpVerb.cs
- Item.cs
- InputGestureCollection.cs
- mactripleDES.cs
- DataSourceHelper.cs
- ObjectDataSourceStatusEventArgs.cs
- MouseCaptureWithinProperty.cs
- DataRecordInternal.cs
- TypeContext.cs
- ISO2022Encoding.cs
- MultiView.cs
- HtmlTableCell.cs
- DataSourceComponent.cs
- CSharpCodeProvider.cs
- Operator.cs
- XmlSchemaComplexContentRestriction.cs
- SiteMapDataSource.cs
- MimeMapping.cs
- ToolStripComboBox.cs
- Span.cs
- Convert.cs
- MulticastDelegate.cs
- ComboBoxItem.cs
- DataGridView.cs
- TagMapInfo.cs
- newitemfactory.cs
- TextBlockAutomationPeer.cs
- ThreadPool.cs
- ApplicationGesture.cs
- _RequestCacheProtocol.cs
- SqlCacheDependencySection.cs
- UnsafeNativeMethods.cs
- AuthenticationModuleElement.cs
- PeerToPeerException.cs
- HtmlTableRow.cs
- RowUpdatingEventArgs.cs
- RawMouseInputReport.cs
- PageThemeCodeDomTreeGenerator.cs
- ReflectionServiceProvider.cs
- TemplateField.cs
- Typeface.cs
- FileChangesMonitor.cs
- DataSpaceManager.cs
- MemberPath.cs
- InputManager.cs
- CodeMemberEvent.cs
- CellQuery.cs
- SslSecurityTokenParameters.cs
- ProgressBar.cs
- Line.cs
- Rfc2898DeriveBytes.cs
- StructuralObject.cs
- MissingMemberException.cs
- DocumentViewer.cs
- ValidationSummary.cs
- BaseServiceProvider.cs
- SvcMapFileSerializer.cs
- UpdateEventArgs.cs
- IntSecurity.cs
- DataViewListener.cs
- AddressUtility.cs
- _SecureChannel.cs
- RelationshipEndCollection.cs
- PeerCollaborationPermission.cs
- WebBrowserContainer.cs
- UnSafeCharBuffer.cs
- SafeNativeMethods.cs
- DispatcherHooks.cs
- dbenumerator.cs