Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / AddressUtility.cs / 1 / AddressUtility.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // A collection of utility functions for dealing with e-mail address // strings and Uri objects. // // History: // 10/26/05 - [....] created // //--------------------------------------------------------------------------- using System; using System.Globalization; using System.Text.RegularExpressions; using MS.Internal.WindowsBase; namespace MS.Internal.Documents { ////// A collection of utility functions for dealing with e-mail address /// strings and Uri objects. /// internal static class AddressUtility { #region Internal Methods //----------------------------------------------------- // Internal Methods //----------------------------------------------------- ////// Checks if the scheme of the URI given is of the mailto scheme. /// /// The URI to check ///Whether or not it is a mailto URI internal static bool IsMailtoUri(Uri mailtoUri) { if (mailtoUri != null) { return SecurityHelper.AreStringTypesEqual( mailtoUri.Scheme, Uri.UriSchemeMailto); } return false; } ////// Gets the e-mail address from the URI given. /// /// The URI ///The e-mail address internal static string GetEmailAddressFromMailtoUri(Uri mailtoUri) { string address = string.Empty; // Ensure it is a mailto: scheme URI if (IsMailtoUri(mailtoUri)) { // Create the address from the URI address = string.Format( CultureInfo.CurrentCulture, _addressTemplate, mailtoUri.GetComponents(UriComponents.UserInfo, UriFormat.Unescaped), mailtoUri.GetComponents(UriComponents.Host, UriFormat.Unescaped)); } return address; } ////// Generate a mailto: URI from the e-mail address given as an /// argument. /// /// The address to generate a URI from ///The generated URI internal static Uri GenerateMailtoUri(string address) { // Add the scheme to the e-mail address to form a URI object if (!string.IsNullOrEmpty(address)) { return new Uri(string.Format( CultureInfo.CurrentCulture, _mailtoUriTemplate, Uri.UriSchemeMailto, _mailtoSchemeDelimiter, address)); } return null; } #endregion Internal Methods #region Private Fields //-------------------------------------------------------------------------- // Private Fields //------------------------------------------------------------------------- ////// The template for an e-mail address with a user name and a host. /// private const string _addressTemplate = "{0}@{1}"; ////// The template for a mailto scheme URI with the mailto scheme and an /// address. /// private const string _mailtoUriTemplate = "{0}{1}{2}"; ////// The delimiter between the scheme and the address in a mailto URI. /// We unfortunately cannot use the Uri class SchemeDelimiter because /// it is by default set to "://", which makes mailto: URIs generated /// look like "mailto://user@host", whicn in turn cannot be parsed /// properly by this class. /// private const string _mailtoSchemeDelimiter = ":"; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
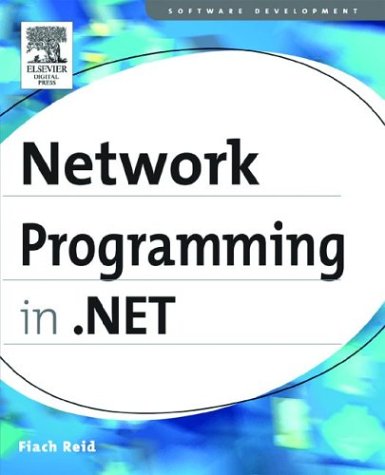
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventProviderBase.cs
- ServiceDescriptionContext.cs
- Stream.cs
- LineGeometry.cs
- DocumentAutomationPeer.cs
- ObservableDictionary.cs
- ActiveXSite.cs
- RequestQueue.cs
- EventSetter.cs
- Point3DCollection.cs
- XmlDocument.cs
- IndexingContentUnit.cs
- VisualTreeHelper.cs
- HostedAspNetEnvironment.cs
- CommandID.cs
- Stacktrace.cs
- ProcessModelInfo.cs
- CapabilitiesAssignment.cs
- SystemDropShadowChrome.cs
- UseLicense.cs
- HtmlControlAdapter.cs
- XamlSerializerUtil.cs
- TextParentUndoUnit.cs
- TextTreeInsertUndoUnit.cs
- HandlerBase.cs
- HtmlTextViewAdapter.cs
- GroupStyle.cs
- GuidelineSet.cs
- DurableInstance.cs
- CachingHintValidation.cs
- Win32.cs
- TypeDescriptor.cs
- OutputBuffer.cs
- Bezier.cs
- DataGridViewAutoSizeModeEventArgs.cs
- DecoderBestFitFallback.cs
- SmiEventStream.cs
- PermissionSet.cs
- ChannelFactoryBase.cs
- FlowNode.cs
- DataFormats.cs
- InstancePersistence.cs
- BindingElementExtensionElement.cs
- TraceSwitch.cs
- PropertyCollection.cs
- AppDomain.cs
- SqlConnectionFactory.cs
- SourceElementsCollection.cs
- ScriptingScriptResourceHandlerSection.cs
- BidirectionalDictionary.cs
- AccessDataSource.cs
- AttributedMetaModel.cs
- TreeNodeMouseHoverEvent.cs
- DataReaderContainer.cs
- BasePropertyDescriptor.cs
- CanonicalFontFamilyReference.cs
- XmlBinaryReader.cs
- TabItemAutomationPeer.cs
- Symbol.cs
- PipeSecurity.cs
- UnmanagedHandle.cs
- CodeValidator.cs
- CatalogZoneDesigner.cs
- Attribute.cs
- Command.cs
- OleDbCommand.cs
- DBCSCodePageEncoding.cs
- OracleLob.cs
- SharedPerformanceCounter.cs
- ProvideValueServiceProvider.cs
- LinkLabel.cs
- DbConnectionPoolCounters.cs
- FeatureSupport.cs
- HtmlHead.cs
- InputLangChangeEvent.cs
- AddInBase.cs
- QueryResponse.cs
- UrlParameterWriter.cs
- WindowsFormsEditorServiceHelper.cs
- XamlTemplateSerializer.cs
- ZipIOLocalFileHeader.cs
- COM2IDispatchConverter.cs
- SimpleType.cs
- TypeSource.cs
- XamlPointCollectionSerializer.cs
- WebResponse.cs
- ScriptResourceHandler.cs
- BitmapSizeOptions.cs
- HelpFileFileNameEditor.cs
- BitmapEffectDrawingContextState.cs
- AppDomain.cs
- connectionpool.cs
- ServerReliableChannelBinder.cs
- WebPart.cs
- Activator.cs
- FileRegion.cs
- ArrayElementGridEntry.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- TypeDependencyAttribute.cs
- DataBoundControl.cs