Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / Diagnostics / TraceListener.cs / 1 / TraceListener.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Diagnostics { using System; using System.Text; using System.Security.Permissions; using System.Collections.Specialized; using System.Globalization; using System.Runtime.InteropServices; using System.Collections; using System.Configuration; using System.Runtime.Versioning; ////// [HostProtection(Synchronization=true)] public abstract class TraceListener : MarshalByRefObject, IDisposable { int indentLevel; int indentSize = 4; TraceOptions traceOptions = TraceOptions.None; bool needIndent = true; string listenerName; TraceFilter filter = null; StringDictionary attributes; internal string initializeData; ///Provides the ///base class for the listeners who /// monitor trace and debug output. /// protected TraceListener () { } ///Initializes a new instance of the ///class. /// protected TraceListener(string name) { this.listenerName = name; } public StringDictionary Attributes { get { if (attributes == null) attributes = new StringDictionary(); return attributes; } } ///Initializes a new instance of the ///class using the specified name as the /// listener. /// public virtual string Name { get { return (listenerName == null) ? "" : listenerName; } set { listenerName = value; } } public virtual bool IsThreadSafe { get { return false; } } ///Gets or sets a name for this ///. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// protected virtual void Dispose(bool disposing) { return; } ////// public virtual void Close() { return; } ///When overridden in a derived class, closes the output stream /// so that it no longer receives tracing or debugging output. ////// public virtual void Flush() { return; } ///When overridden in a derived class, flushes the output buffer. ////// public int IndentLevel { get { return indentLevel; } set { indentLevel = (value < 0) ? 0 : value; } } ///Gets or sets the indent level. ////// public int IndentSize { get { return indentSize; } set { if (value < 0) throw new ArgumentOutOfRangeException("IndentSize", value, SR.GetString(SR.TraceListenerIndentSize)); indentSize = value; } } [ ComVisible(false) ] public TraceFilter Filter { get { return filter; } set { filter = value; } } ///Gets or sets the number of spaces in an indent. ////// protected bool NeedIndent { get { return needIndent; } set { needIndent = value; } } [ ComVisible(false) ] public TraceOptions TraceOutputOptions { get { return traceOptions; } set { if (( (int) value >> 6) != 0) { throw new ArgumentOutOfRangeException("value"); } traceOptions = value; } } internal void SetAttributes(Hashtable attribs) { TraceUtils.VerifyAttributes(attribs, GetSupportedAttributes(), this); attributes = new StringDictionary(); attributes.contents = attribs; } ///Gets or sets a value indicating whether an indent is needed. ////// public virtual void Fail(string message) { Fail(message, null); } ///Emits or displays a message for an assertion that always fails. ////// public virtual void Fail(string message, string detailMessage) { StringBuilder failMessage = new StringBuilder(); failMessage.Append(SR.GetString(SR.TraceListenerFail)); failMessage.Append(" "); failMessage.Append(message); if (detailMessage != null) { failMessage.Append(" "); failMessage.Append(detailMessage); } WriteLine(failMessage.ToString()); } virtual protected internal string[] GetSupportedAttributes() { return null; } ///Emits or displays messages for an assertion that always fails. ////// public abstract void Write(string message); ///When overridden in a derived class, writes the specified /// message to the listener you specify in the derived class. ////// public virtual void Write(object o) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, null, null, o)) return; if (o == null) return; Write(o.ToString()); } ///Writes the name of the ///parameter to the listener you specify when you inherit from the /// class. /// public virtual void Write(string message, string category) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, message)) return; if (category == null) Write(message); else Write(category + ": " + ((message == null) ? string.Empty : message)); } ///Writes a category name and a message to the listener you specify when you /// inherit from the ////// class. /// public virtual void Write(object o, string category) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, category, null, o)) return; if (category == null) Write(o); else Write(o == null ? "" : o.ToString(), category); } ///Writes a category name and the name of the ///parameter to the listener you /// specify when you inherit from the /// class. /// protected virtual void WriteIndent() { NeedIndent = false; for (int i = 0; i < indentLevel; i++) { if (indentSize == 4) Write(" "); else { for (int j = 0; j < indentSize; j++) { Write(" "); } } } } ///Writes the indent to the listener you specify when you /// inherit from the ////// class, and resets the property to . /// public abstract void WriteLine(string message); ///When overridden in a derived class, writes a message to the listener you specify in /// the derived class, followed by a line terminator. The default line terminator is a carriage return followed /// by a line feed (\r\n). ////// public virtual void WriteLine(object o) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, null, null, o)) return; WriteLine(o == null ? "" : o.ToString()); } ///Writes the name of the ///parameter to the listener you specify when you inherit from the class, followed by a line terminator. The default line terminator is a /// carriage return followed by a line feed /// (\r\n). /// public virtual void WriteLine(string message, string category) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, message)) return; if (category == null) WriteLine(message); else WriteLine(category + ": " + ((message == null) ? string.Empty : message)); } ///Writes a category name and a message to the listener you specify when you /// inherit from the ///class, /// followed by a line terminator. The default line terminator is a carriage return followed by a line feed (\r\n). /// public virtual void WriteLine(object o, string category) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, category, null, o)) return; WriteLine(o == null ? "" : o.ToString(), category); } // new write methods used by TraceSource [ ComVisible(false) ] public virtual void TraceData(TraceEventCache eventCache, String source, TraceEventType eventType, int id, object data) { if (Filter != null && !Filter.ShouldTrace(eventCache, source, eventType, id, null, null, data)) return; WriteHeader(source, eventType, id); string datastring = String.Empty; if (data != null) datastring = data.ToString(); WriteLine(datastring); WriteFooter(eventCache); } [ ComVisible(false) ] public virtual void TraceData(TraceEventCache eventCache, String source, TraceEventType eventType, int id, params object[] data) { if (Filter != null && !Filter.ShouldTrace(eventCache, source, eventType, id, null, null, null, data)) return; WriteHeader(source, eventType, id); StringBuilder sb = new StringBuilder(); if (data != null) { for (int i=0; i< data.Length; i++) { if (i != 0) sb.Append(", "); if (data[i] != null) sb.Append(data[i].ToString()); } } WriteLine(sb.ToString()); WriteFooter(eventCache); } [ ComVisible(false) ] public virtual void TraceEvent(TraceEventCache eventCache, String source, TraceEventType eventType, int id) { TraceEvent(eventCache, source, eventType, id, String.Empty); } // All other TraceEvent methods come through this one. [ ComVisible(false) ] public virtual void TraceEvent(TraceEventCache eventCache, String source, TraceEventType eventType, int id, string message) { if (Filter != null && !Filter.ShouldTrace(eventCache, source, eventType, id, message)) return; WriteHeader(source, eventType, id); WriteLine(message); WriteFooter(eventCache); } [ ComVisible(false) ] public virtual void TraceEvent(TraceEventCache eventCache, String source, TraceEventType eventType, int id, string format, params object[] args) { if (Filter != null && !Filter.ShouldTrace(eventCache, source, eventType, id, format, args)) return; WriteHeader(source, eventType, id); if (args != null) WriteLine(String.Format(CultureInfo.InvariantCulture, format, args)); else WriteLine(format); WriteFooter(eventCache); } [ ComVisible(false) ] public virtual void TraceTransfer(TraceEventCache eventCache, String source, int id, string message, Guid relatedActivityId) { TraceEvent(eventCache, source, TraceEventType.Transfer, id, message + ", relatedActivityId=" + relatedActivityId.ToString()); } private void WriteHeader(String source, TraceEventType eventType, int id) { Write(String.Format(CultureInfo.InvariantCulture, "{0} {1}: {2} : ", source, eventType.ToString(), id.ToString(CultureInfo.InvariantCulture))); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] private void WriteFooter(TraceEventCache eventCache) { if (eventCache == null) return; indentLevel++; if (IsEnabled(TraceOptions.ProcessId)) WriteLine("ProcessId=" + eventCache.ProcessId); if (IsEnabled(TraceOptions.LogicalOperationStack)) { Write("LogicalOperationStack="); Stack operationStack = eventCache.LogicalOperationStack; bool first = true; foreach (Object obj in operationStack) { if (!first) Write(", "); else first = false; Write(obj.ToString()); } WriteLine(String.Empty); } if (IsEnabled(TraceOptions.ThreadId)) WriteLine("ThreadId=" + eventCache.ThreadId); if (IsEnabled(TraceOptions.DateTime)) WriteLine("DateTime=" + eventCache.DateTime.ToString("o", CultureInfo.InvariantCulture)); if (IsEnabled(TraceOptions.Timestamp)) WriteLine("Timestamp=" + eventCache.Timestamp); if (IsEnabled(TraceOptions.Callstack)) WriteLine("Callstack=" + eventCache.Callstack); indentLevel--; } internal bool IsEnabled(TraceOptions opts) { return (opts & TraceOutputOptions) != 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Writes a category /// name and the name of the ///parameter to the listener you /// specify when you inherit from the /// class, followed by a line terminator. The default line terminator is a carriage /// return followed by a line feed (\r\n). // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Diagnostics { using System; using System.Text; using System.Security.Permissions; using System.Collections.Specialized; using System.Globalization; using System.Runtime.InteropServices; using System.Collections; using System.Configuration; using System.Runtime.Versioning; ////// [HostProtection(Synchronization=true)] public abstract class TraceListener : MarshalByRefObject, IDisposable { int indentLevel; int indentSize = 4; TraceOptions traceOptions = TraceOptions.None; bool needIndent = true; string listenerName; TraceFilter filter = null; StringDictionary attributes; internal string initializeData; ///Provides the ///base class for the listeners who /// monitor trace and debug output. /// protected TraceListener () { } ///Initializes a new instance of the ///class. /// protected TraceListener(string name) { this.listenerName = name; } public StringDictionary Attributes { get { if (attributes == null) attributes = new StringDictionary(); return attributes; } } ///Initializes a new instance of the ///class using the specified name as the /// listener. /// public virtual string Name { get { return (listenerName == null) ? "" : listenerName; } set { listenerName = value; } } public virtual bool IsThreadSafe { get { return false; } } ///Gets or sets a name for this ///. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// protected virtual void Dispose(bool disposing) { return; } ////// public virtual void Close() { return; } ///When overridden in a derived class, closes the output stream /// so that it no longer receives tracing or debugging output. ////// public virtual void Flush() { return; } ///When overridden in a derived class, flushes the output buffer. ////// public int IndentLevel { get { return indentLevel; } set { indentLevel = (value < 0) ? 0 : value; } } ///Gets or sets the indent level. ////// public int IndentSize { get { return indentSize; } set { if (value < 0) throw new ArgumentOutOfRangeException("IndentSize", value, SR.GetString(SR.TraceListenerIndentSize)); indentSize = value; } } [ ComVisible(false) ] public TraceFilter Filter { get { return filter; } set { filter = value; } } ///Gets or sets the number of spaces in an indent. ////// protected bool NeedIndent { get { return needIndent; } set { needIndent = value; } } [ ComVisible(false) ] public TraceOptions TraceOutputOptions { get { return traceOptions; } set { if (( (int) value >> 6) != 0) { throw new ArgumentOutOfRangeException("value"); } traceOptions = value; } } internal void SetAttributes(Hashtable attribs) { TraceUtils.VerifyAttributes(attribs, GetSupportedAttributes(), this); attributes = new StringDictionary(); attributes.contents = attribs; } ///Gets or sets a value indicating whether an indent is needed. ////// public virtual void Fail(string message) { Fail(message, null); } ///Emits or displays a message for an assertion that always fails. ////// public virtual void Fail(string message, string detailMessage) { StringBuilder failMessage = new StringBuilder(); failMessage.Append(SR.GetString(SR.TraceListenerFail)); failMessage.Append(" "); failMessage.Append(message); if (detailMessage != null) { failMessage.Append(" "); failMessage.Append(detailMessage); } WriteLine(failMessage.ToString()); } virtual protected internal string[] GetSupportedAttributes() { return null; } ///Emits or displays messages for an assertion that always fails. ////// public abstract void Write(string message); ///When overridden in a derived class, writes the specified /// message to the listener you specify in the derived class. ////// public virtual void Write(object o) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, null, null, o)) return; if (o == null) return; Write(o.ToString()); } ///Writes the name of the ///parameter to the listener you specify when you inherit from the /// class. /// public virtual void Write(string message, string category) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, message)) return; if (category == null) Write(message); else Write(category + ": " + ((message == null) ? string.Empty : message)); } ///Writes a category name and a message to the listener you specify when you /// inherit from the ////// class. /// public virtual void Write(object o, string category) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, category, null, o)) return; if (category == null) Write(o); else Write(o == null ? "" : o.ToString(), category); } ///Writes a category name and the name of the ///parameter to the listener you /// specify when you inherit from the /// class. /// protected virtual void WriteIndent() { NeedIndent = false; for (int i = 0; i < indentLevel; i++) { if (indentSize == 4) Write(" "); else { for (int j = 0; j < indentSize; j++) { Write(" "); } } } } ///Writes the indent to the listener you specify when you /// inherit from the ////// class, and resets the property to . /// public abstract void WriteLine(string message); ///When overridden in a derived class, writes a message to the listener you specify in /// the derived class, followed by a line terminator. The default line terminator is a carriage return followed /// by a line feed (\r\n). ////// public virtual void WriteLine(object o) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, null, null, o)) return; WriteLine(o == null ? "" : o.ToString()); } ///Writes the name of the ///parameter to the listener you specify when you inherit from the class, followed by a line terminator. The default line terminator is a /// carriage return followed by a line feed /// (\r\n). /// public virtual void WriteLine(string message, string category) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, message)) return; if (category == null) WriteLine(message); else WriteLine(category + ": " + ((message == null) ? string.Empty : message)); } ///Writes a category name and a message to the listener you specify when you /// inherit from the ///class, /// followed by a line terminator. The default line terminator is a carriage return followed by a line feed (\r\n). /// public virtual void WriteLine(object o, string category) { if (Filter != null && !Filter.ShouldTrace(null, "", TraceEventType.Verbose, 0, category, null, o)) return; WriteLine(o == null ? "" : o.ToString(), category); } // new write methods used by TraceSource [ ComVisible(false) ] public virtual void TraceData(TraceEventCache eventCache, String source, TraceEventType eventType, int id, object data) { if (Filter != null && !Filter.ShouldTrace(eventCache, source, eventType, id, null, null, data)) return; WriteHeader(source, eventType, id); string datastring = String.Empty; if (data != null) datastring = data.ToString(); WriteLine(datastring); WriteFooter(eventCache); } [ ComVisible(false) ] public virtual void TraceData(TraceEventCache eventCache, String source, TraceEventType eventType, int id, params object[] data) { if (Filter != null && !Filter.ShouldTrace(eventCache, source, eventType, id, null, null, null, data)) return; WriteHeader(source, eventType, id); StringBuilder sb = new StringBuilder(); if (data != null) { for (int i=0; i< data.Length; i++) { if (i != 0) sb.Append(", "); if (data[i] != null) sb.Append(data[i].ToString()); } } WriteLine(sb.ToString()); WriteFooter(eventCache); } [ ComVisible(false) ] public virtual void TraceEvent(TraceEventCache eventCache, String source, TraceEventType eventType, int id) { TraceEvent(eventCache, source, eventType, id, String.Empty); } // All other TraceEvent methods come through this one. [ ComVisible(false) ] public virtual void TraceEvent(TraceEventCache eventCache, String source, TraceEventType eventType, int id, string message) { if (Filter != null && !Filter.ShouldTrace(eventCache, source, eventType, id, message)) return; WriteHeader(source, eventType, id); WriteLine(message); WriteFooter(eventCache); } [ ComVisible(false) ] public virtual void TraceEvent(TraceEventCache eventCache, String source, TraceEventType eventType, int id, string format, params object[] args) { if (Filter != null && !Filter.ShouldTrace(eventCache, source, eventType, id, format, args)) return; WriteHeader(source, eventType, id); if (args != null) WriteLine(String.Format(CultureInfo.InvariantCulture, format, args)); else WriteLine(format); WriteFooter(eventCache); } [ ComVisible(false) ] public virtual void TraceTransfer(TraceEventCache eventCache, String source, int id, string message, Guid relatedActivityId) { TraceEvent(eventCache, source, TraceEventType.Transfer, id, message + ", relatedActivityId=" + relatedActivityId.ToString()); } private void WriteHeader(String source, TraceEventType eventType, int id) { Write(String.Format(CultureInfo.InvariantCulture, "{0} {1}: {2} : ", source, eventType.ToString(), id.ToString(CultureInfo.InvariantCulture))); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] private void WriteFooter(TraceEventCache eventCache) { if (eventCache == null) return; indentLevel++; if (IsEnabled(TraceOptions.ProcessId)) WriteLine("ProcessId=" + eventCache.ProcessId); if (IsEnabled(TraceOptions.LogicalOperationStack)) { Write("LogicalOperationStack="); Stack operationStack = eventCache.LogicalOperationStack; bool first = true; foreach (Object obj in operationStack) { if (!first) Write(", "); else first = false; Write(obj.ToString()); } WriteLine(String.Empty); } if (IsEnabled(TraceOptions.ThreadId)) WriteLine("ThreadId=" + eventCache.ThreadId); if (IsEnabled(TraceOptions.DateTime)) WriteLine("DateTime=" + eventCache.DateTime.ToString("o", CultureInfo.InvariantCulture)); if (IsEnabled(TraceOptions.Timestamp)) WriteLine("Timestamp=" + eventCache.Timestamp); if (IsEnabled(TraceOptions.Callstack)) WriteLine("Callstack=" + eventCache.Callstack); indentLevel--; } internal bool IsEnabled(TraceOptions opts) { return (opts & TraceOutputOptions) != 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Writes a category /// name and the name of the ///parameter to the listener you /// specify when you inherit from the /// class, followed by a line terminator. The default line terminator is a carriage /// return followed by a line feed (\r\n).
Link Menu
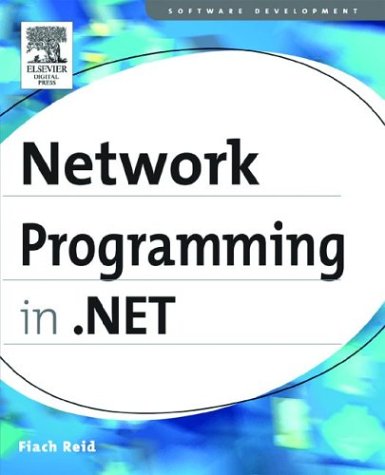
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CFStream.cs
- ConfigurationStrings.cs
- ListComponentEditor.cs
- WsatServiceAddress.cs
- TextUtf8RawTextWriter.cs
- Point.cs
- LoadedOrUnloadedOperation.cs
- RuntimeHandles.cs
- HttpServerVarsCollection.cs
- DataProtection.cs
- WarningException.cs
- RadioButtonFlatAdapter.cs
- HttpProfileBase.cs
- ServiceBehaviorAttribute.cs
- CustomLineCap.cs
- WebRequestModulesSection.cs
- MailDefinition.cs
- TextTreeTextElementNode.cs
- StylusPointPropertyUnit.cs
- TransactionManagerProxy.cs
- WebPartPersonalization.cs
- DataGridRowDetailsEventArgs.cs
- UxThemeWrapper.cs
- WindowsListViewSubItem.cs
- IItemContainerGenerator.cs
- TextEditorTyping.cs
- XmlILIndex.cs
- SafeSecurityHandles.cs
- SourceChangedEventArgs.cs
- FixedPageStructure.cs
- StorageEntityTypeMapping.cs
- AnnotationAuthorChangedEventArgs.cs
- MgmtConfigurationRecord.cs
- XmlAttributeOverrides.cs
- CodeAttributeArgument.cs
- ObjectReaderCompiler.cs
- ProfileGroupSettings.cs
- MouseDevice.cs
- SectionVisual.cs
- Subtree.cs
- PrivilegedConfigurationManager.cs
- Application.cs
- XmlUrlResolver.cs
- DataShape.cs
- SapiAttributeParser.cs
- ImageListUtils.cs
- WindowsPrincipal.cs
- OleDbInfoMessageEvent.cs
- NavigationWindow.cs
- HttpFileCollection.cs
- ButtonStandardAdapter.cs
- ZoneButton.cs
- NameNode.cs
- HtmlForm.cs
- Vector.cs
- MenuItem.cs
- Selector.cs
- Int32RectValueSerializer.cs
- HttpHandlersSection.cs
- NameValueConfigurationCollection.cs
- ToolboxItemFilterAttribute.cs
- FilterEventArgs.cs
- AutomationIdentifierGuids.cs
- documentsequencetextcontainer.cs
- StrongName.cs
- SystemNetHelpers.cs
- Win32.cs
- StylusLogic.cs
- HttpCacheVary.cs
- TaiwanLunisolarCalendar.cs
- LingerOption.cs
- Event.cs
- CustomError.cs
- CallContext.cs
- SystemResourceHost.cs
- DataControlFieldHeaderCell.cs
- BitArray.cs
- SizeAnimation.cs
- HTMLTagNameToTypeMapper.cs
- MSAAWinEventWrap.cs
- SessionEndedEventArgs.cs
- QilNode.cs
- DynamicResourceExtensionConverter.cs
- LogEntrySerializer.cs
- DependencyObjectType.cs
- Predicate.cs
- DataServiceResponse.cs
- IPAddress.cs
- StoreItemCollection.cs
- ToolStripItemRenderEventArgs.cs
- AutoGeneratedFieldProperties.cs
- TextServicesLoader.cs
- XmlSchemaNotation.cs
- ContainerSelectorBehavior.cs
- DataTableMappingCollection.cs
- DataGridViewSelectedRowCollection.cs
- SortQuery.cs
- SoapMessage.cs
- PersonalizationDictionary.cs
- PreservationFileWriter.cs