Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Primitives / ItemsChangedEventArgs.cs / 1305600 / ItemsChangedEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Delegate and args for the ItemsChanged event. // // Specs: http://avalon/connecteddata/M5%20General%20Docs/Data%20Styling.mht // //--------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.ComponentModel; namespace System.Windows.Controls.Primitives { ////// The ItemsChanged event is raised by an ItemContainerGenerator to inform /// layouts that the items collection has changed. /// public class ItemsChangedEventArgs : EventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- internal ItemsChangedEventArgs(NotifyCollectionChangedAction action, GeneratorPosition position, GeneratorPosition oldPosition, int itemCount, int itemUICount) { _action = action; _position = position; _oldPosition = oldPosition; _itemCount = itemCount; _itemUICount = itemUICount; } internal ItemsChangedEventArgs(NotifyCollectionChangedAction action, GeneratorPosition position, int itemCount, int itemUICount) : this(action, position, new GeneratorPosition(-1, 0), itemCount, itemUICount) { } //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ///What happened public NotifyCollectionChangedAction Action { get { return _action; } } ///Where it happened public GeneratorPosition Position { get { return _position; } } ///Where it happened public GeneratorPosition OldPosition { get { return _oldPosition; } } ///How many items were involved public int ItemCount { get { return _itemCount; } } ///How many UI elements were involved public int ItemUICount { get { return _itemUICount; } } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ NotifyCollectionChangedAction _action; GeneratorPosition _position; GeneratorPosition _oldPosition; int _itemCount; int _itemUICount; } ////// The delegate to use for handlers that receive ItemsChangedEventArgs. /// public delegate void ItemsChangedEventHandler(object sender, ItemsChangedEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
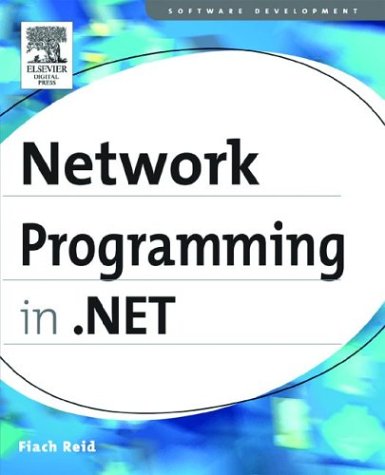
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FontWeights.cs
- DataColumnPropertyDescriptor.cs
- WebPartActionVerb.cs
- WriteFileContext.cs
- Token.cs
- ParameterCollection.cs
- HostVisual.cs
- ParameterModifier.cs
- XamlSerializationHelper.cs
- DllHostedComPlusServiceHost.cs
- ResourceReferenceExpression.cs
- TablePattern.cs
- SystemException.cs
- ConstantExpression.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- LinkedList.cs
- StaticResourceExtension.cs
- SoapProtocolImporter.cs
- ColumnMapTranslator.cs
- RTLAwareMessageBox.cs
- CompoundFileDeflateTransform.cs
- Variable.cs
- ThrowHelper.cs
- PostBackOptions.cs
- ProfileSettings.cs
- ExpressionBuilderContext.cs
- DataMemberFieldEditor.cs
- SoapHeaderAttribute.cs
- PagePropertiesChangingEventArgs.cs
- TextProviderWrapper.cs
- MetaForeignKeyColumn.cs
- UniqueEventHelper.cs
- ToolStripContentPanelDesigner.cs
- LongTypeConverter.cs
- AppDomainShutdownMonitor.cs
- X509Utils.cs
- ClientConfigPaths.cs
- PublisherMembershipCondition.cs
- BeginStoryboard.cs
- UnsafeNativeMethods.cs
- cookie.cs
- UpdateEventArgs.cs
- SuppressedPackageProperties.cs
- TextRangeProviderWrapper.cs
- ButtonField.cs
- validation.cs
- SamlDoNotCacheCondition.cs
- MatrixTransform.cs
- DiscoveryClientDocuments.cs
- XmlArrayItemAttribute.cs
- SocketCache.cs
- DirectoryNotFoundException.cs
- BitmapEffectInput.cs
- DataPagerFieldCollection.cs
- ContactManager.cs
- HtmlInputControl.cs
- Properties.cs
- MultiPropertyDescriptorGridEntry.cs
- ConfigXmlText.cs
- ScopelessEnumAttribute.cs
- OrderToken.cs
- Opcode.cs
- SiteMapNodeCollection.cs
- CollaborationHelperFunctions.cs
- EntitySetRetriever.cs
- TextTreeRootNode.cs
- StatementContext.cs
- Material.cs
- VirtualPathData.cs
- LocalizedNameDescriptionPair.cs
- SiteMapPath.cs
- ConnectionManagementSection.cs
- Calendar.cs
- AnchoredBlock.cs
- InvokeGenerator.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- IIS7UserPrincipal.cs
- Source.cs
- TransactionException.cs
- FormatterServices.cs
- AxDesigner.cs
- ItemCheckedEvent.cs
- HttpStreamFormatter.cs
- CodeDomSerializerBase.cs
- EntityContainerEmitter.cs
- LicenseContext.cs
- ServiceOperation.cs
- DbQueryCommandTree.cs
- ManualResetEvent.cs
- OdbcUtils.cs
- FrameworkPropertyMetadata.cs
- ExpandedWrapper.cs
- RadioButtonPopupAdapter.cs
- FontUnitConverter.cs
- XamlTemplateSerializer.cs
- Int16KeyFrameCollection.cs
- BatchServiceHost.cs
- NotCondition.cs
- RequestCacheEntry.cs
- TextTreeRootTextBlock.cs