Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / ConstantExpression.cs / 1305376 / ConstantExpression.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Diagnostics; using System.Dynamic.Utils; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ////// Represents an expression that has a constant value. /// #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.ConstantExpressionProxy))] #endif public class ConstantExpression : Expression { // Possible optimization: we could have a Constantsubclass that // stores the unboxed value. private readonly object _value; internal ConstantExpression(object value) { _value = value; } internal static ConstantExpression Make(object value, Type type) { if ((value == null && type == typeof(object)) || (value != null && value.GetType() == type)) { return new ConstantExpression(value); } else { return new TypedConstantExpression(value, type); } } /// /// Gets the static type of the expression that this ///represents. /// The public override Type Type { get { if (_value == null) { return typeof(object); } return _value.GetType(); } } ///that represents the static type of the expression. /// Returns the node type of this Expression. Extension nodes should return /// ExpressionType.Extension when overriding this method. /// ///The public sealed override ExpressionType NodeType { get { return ExpressionType.Constant; } } ///of the expression. /// Gets the value of the constant expression. /// public object Value { get { return _value; } } ////// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitConstant(this); } } internal class TypedConstantExpression : ConstantExpression { private readonly Type _type; internal TypedConstantExpression(object value, Type type) : base(value) { _type = type; } public sealed override Type Type { get { return _type; } } } public partial class Expression { ////// Creates a /// Anthat has the property set to the specified value. . /// to set the property equal to. /// /// A public static ConstantExpression Constant(object value) { return ConstantExpression.Make(value, value == null ? typeof(object) : value.GetType()); } ///that has the property equal to /// and the property set to the specified value. /// /// Creates a /// Anthat has the /// and properties set to the specified values. . /// to set the property equal to. /// A to set the property equal to. /// /// A public static ConstantExpression Constant(object value, Type type) { ContractUtils.RequiresNotNull(type, "type"); if (value == null && type.IsValueType && !TypeUtils.IsNullableType(type)) { throw Error.ArgumentTypesMustMatch(); } if (value != null && !type.IsAssignableFrom(value.GetType())) { throw Error.ArgumentTypesMustMatch(); } return ConstantExpression.Make(value, type); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Diagnostics; using System.Dynamic.Utils; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ///that has the property equal to /// and the and /// properties set to the specified values. /// /// Represents an expression that has a constant value. /// #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.ConstantExpressionProxy))] #endif public class ConstantExpression : Expression { // Possible optimization: we could have a Constantsubclass that // stores the unboxed value. private readonly object _value; internal ConstantExpression(object value) { _value = value; } internal static ConstantExpression Make(object value, Type type) { if ((value == null && type == typeof(object)) || (value != null && value.GetType() == type)) { return new ConstantExpression(value); } else { return new TypedConstantExpression(value, type); } } /// /// Gets the static type of the expression that this ///represents. /// The public override Type Type { get { if (_value == null) { return typeof(object); } return _value.GetType(); } } ///that represents the static type of the expression. /// Returns the node type of this Expression. Extension nodes should return /// ExpressionType.Extension when overriding this method. /// ///The public sealed override ExpressionType NodeType { get { return ExpressionType.Constant; } } ///of the expression. /// Gets the value of the constant expression. /// public object Value { get { return _value; } } ////// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitConstant(this); } } internal class TypedConstantExpression : ConstantExpression { private readonly Type _type; internal TypedConstantExpression(object value, Type type) : base(value) { _type = type; } public sealed override Type Type { get { return _type; } } } public partial class Expression { ////// Creates a /// Anthat has the property set to the specified value. . /// to set the property equal to. /// /// A public static ConstantExpression Constant(object value) { return ConstantExpression.Make(value, value == null ? typeof(object) : value.GetType()); } ///that has the property equal to /// and the property set to the specified value. /// /// Creates a /// Anthat has the /// and properties set to the specified values. . /// to set the property equal to. /// A to set the property equal to. /// /// A public static ConstantExpression Constant(object value, Type type) { ContractUtils.RequiresNotNull(type, "type"); if (value == null && type.IsValueType && !TypeUtils.IsNullableType(type)) { throw Error.ArgumentTypesMustMatch(); } if (value != null && !type.IsAssignableFrom(value.GetType())) { throw Error.ArgumentTypesMustMatch(); } return ConstantExpression.Make(value, type); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.that has the property equal to /// and the and /// properties set to the specified values. ///
Link Menu
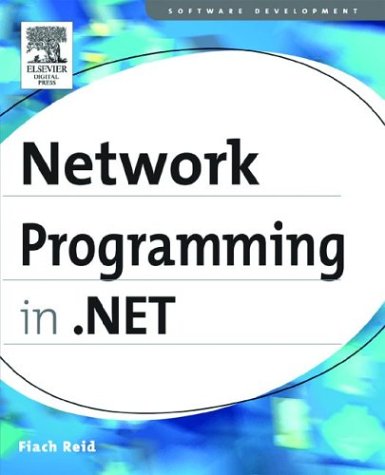
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebServiceTypeData.cs
- ButtonStandardAdapter.cs
- TargetInvocationException.cs
- FileLogRecord.cs
- GetImportedCardRequest.cs
- XhtmlBasicValidationSummaryAdapter.cs
- TraceEventCache.cs
- KerberosRequestorSecurityToken.cs
- PolicyLevel.cs
- PolicyValidationException.cs
- UriSectionReader.cs
- ShapingWorkspace.cs
- MsmqIntegrationReceiveParameters.cs
- EntityDataSourceContainerNameItem.cs
- ClientSettingsProvider.cs
- SignedInfo.cs
- Base64Stream.cs
- _TimerThread.cs
- HttpResponse.cs
- ToolboxBitmapAttribute.cs
- FormatPage.cs
- XamlParser.cs
- WebPartVerb.cs
- RuntimeIdentifierPropertyAttribute.cs
- Metadata.cs
- XPathSelectionIterator.cs
- TextReader.cs
- SatelliteContractVersionAttribute.cs
- RenderDataDrawingContext.cs
- ZoomingMessageFilter.cs
- StringInfo.cs
- Version.cs
- TemplateNameScope.cs
- GenericUI.cs
- FullTrustAssemblyCollection.cs
- CodeTypeConstructor.cs
- OperandQuery.cs
- ComponentDispatcher.cs
- RtfFormatStack.cs
- ApplicationFileParser.cs
- DataFormats.cs
- IsolatedStorageFilePermission.cs
- DataGridViewComboBoxColumn.cs
- WebServicesSection.cs
- TypeExtension.cs
- RegistrySecurity.cs
- TrustManagerMoreInformation.cs
- DateTimeStorage.cs
- Input.cs
- StreamProxy.cs
- TextTreeRootNode.cs
- RepeatBehaviorConverter.cs
- DayRenderEvent.cs
- EditCommandColumn.cs
- HandlerBase.cs
- ScrollItemPattern.cs
- SqlWriter.cs
- DesignTimeDataBinding.cs
- ReachSerializableProperties.cs
- DXD.cs
- BooleanKeyFrameCollection.cs
- CTreeGenerator.cs
- XmlSchemaNotation.cs
- Membership.cs
- versioninfo.cs
- Span.cs
- SqlUtil.cs
- CalculatedColumn.cs
- VariableAction.cs
- PropertyValueUIItem.cs
- WebExceptionStatus.cs
- RSAPKCS1KeyExchangeFormatter.cs
- InternalPolicyElement.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- DataTablePropertyDescriptor.cs
- EnumValidator.cs
- PropertiesTab.cs
- WebPartConnectionsCloseVerb.cs
- DataGridViewComboBoxColumnDesigner.cs
- HtmlImage.cs
- StringFreezingAttribute.cs
- XPathSelfQuery.cs
- MetadataPropertyCollection.cs
- SmiMetaData.cs
- ResourceDictionary.cs
- Track.cs
- QilXmlReader.cs
- JsonWriterDelegator.cs
- ResourceDefaultValueAttribute.cs
- CultureTableRecord.cs
- Char.cs
- CheckBoxRenderer.cs
- AttributeCollection.cs
- M3DUtil.cs
- SHA1CryptoServiceProvider.cs
- HtmlValidationSummaryAdapter.cs
- ToolBarButtonClickEvent.cs
- cookieexception.cs
- Avt.cs
- MdImport.cs