Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / Source.cs / 1 / Source.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design { using System; using System.CodeDom; using System.Collections; using System.ComponentModel; using System.Data; using System.Windows.Forms; using System.Diagnostics; ////// internal abstract class Source: DataSourceComponent, IDataSourceNamedObject, ICloneable { protected string name; private MemberAttributes modifier; protected DataSourceComponent owner; private bool webMethod; private string webMethodDescription; private string userSourceName = null; private string generatorSourceName = null; private string generatorGetMethodName = null; private string generatorSourceNameForPaging = null; private string generatorGetMethodNameForPaging = null; internal Source() { modifier = MemberAttributes.Public; } [ DataSourceXmlAttribute(), DefaultValue(false) ] public bool EnableWebMethods { get { return this.webMethod; } set { this.webMethod = value; } } internal bool IsMainSource { get { DesignTable table = Owner as DesignTable; return (table != null && table.MainSource == this); } } [ DefaultValue(MemberAttributes.Public), DataSourceXmlAttribute() ] public MemberAttributes Modifier { get { return modifier; } set { modifier = value; } } ////// [ DefaultValue(""), DataSourceXmlAttribute(), MergableProperty(false) ] public virtual string Name{ get{ return name; } set{ if (name != value) { if (this.CollectionParent != null) { this.CollectionParent.ValidateUniqueName (this, value); } name = value; } } } ////// Displayed in the Table Control with signature, different with that in the property grid /// internal virtual string DisplayName { get { return Name; } set { } } ////// This is the reference to a DesignTable or DesignDataSource that owns this source. /// Only one DataSourceComponent at a time can own a given source. /// [Browsable(false)] internal DataSourceComponent Owner { get { if (this.owner == null) { // check to see we can find the owner from parent if (this.CollectionParent != null) { SourceCollection collection = this.CollectionParent as SourceCollection; Debug.Assert (collection != null, " Source is not in a SourceCollection?"); if (collection != null) { this.owner = (DataSourceComponent)collection.CollectionHost; } } } return this.owner; } set { Debug.Assert( value == null || value is DesignTable || value is DesignDataSource ); this.owner = value; } } [Browsable(false)] public virtual string PublicTypeName { get { return "Function"; } } [ DataSourceXmlAttribute(), DefaultValue("") ] public string WebMethodDescription { get{ return this.webMethodDescription; } set { this.webMethodDescription = value; } } public abstract object Clone(); ////// Compare the source by name /// /// ///internal virtual bool NameExist(string nameToCheck) { Debug.Assert(StringUtil.NotEmptyAfterTrim(nameToCheck)); return StringUtil.EqualValue(this.Name, nameToCheck, true /*CaseInsensitive*/); } /// /// IDataSourceCollectionMember implementation /// public override void SetCollection(DataSourceCollectionBase collection) { base.SetCollection(collection); if (collection != null) { Debug.Assert(collection is SourceCollection); this.Owner = (DataSourceComponent)collection.CollectionHost; // Might be null } else { this.Owner = null; } } public override string ToString() { return this.PublicTypeName + " " + this.DisplayName; } [DataSourceXmlAttribute(), Browsable (false), DefaultValue(null)] public string UserSourceName { get { return userSourceName; } set { userSourceName = value; } } [DataSourceXmlAttribute(), Browsable (false), DefaultValue(null)] public string GeneratorSourceName { get { return generatorSourceName; } set { generatorSourceName = value; } } [DataSourceXmlAttribute(), Browsable (false), DefaultValue(null)] public string GeneratorGetMethodName { get { return generatorGetMethodName; } set { generatorGetMethodName = value; } } [DataSourceXmlAttribute(), Browsable (false), DefaultValue(null)] public string GeneratorSourceNameForPaging { get { return generatorSourceNameForPaging; } set { generatorSourceNameForPaging = value; } } [DataSourceXmlAttribute(), Browsable (false), DefaultValue(null)] public string GeneratorGetMethodNameForPaging { get { return generatorGetMethodNameForPaging; } set { generatorGetMethodNameForPaging = value; } } // IDataSourceRenamableObject implementation [Browsable(false)] public override string GeneratorName { get { return GeneratorSourceName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
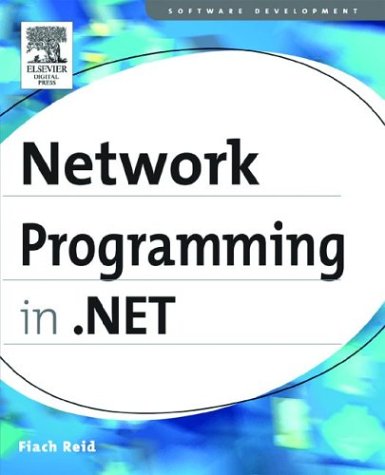
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DockPanel.cs
- WebServiceMethodData.cs
- RuntimeIdentifierPropertyAttribute.cs
- EntryIndex.cs
- OrderPreservingMergeHelper.cs
- OuterGlowBitmapEffect.cs
- SwitchLevelAttribute.cs
- UIElement3D.cs
- WCFBuildProvider.cs
- AnimationException.cs
- DataGridViewColumnHeaderCell.cs
- OracleCommand.cs
- LeftCellWrapper.cs
- LogicalExpressionTypeConverter.cs
- _HeaderInfo.cs
- WindowsGraphicsCacheManager.cs
- HttpCachePolicyBase.cs
- XmlSchemaGroup.cs
- SqlDataSourceCache.cs
- TransportConfigurationTypeElement.cs
- webproxy.cs
- SapiRecoContext.cs
- PingReply.cs
- ConvertEvent.cs
- SQLCharsStorage.cs
- ItemCheckEvent.cs
- DetailsViewUpdatedEventArgs.cs
- ClientTargetCollection.cs
- RoleGroup.cs
- ConfigUtil.cs
- ZoomPercentageConverter.cs
- ProxyHwnd.cs
- EntityContainerEmitter.cs
- FileFormatException.cs
- Clipboard.cs
- CustomValidator.cs
- WinInetCache.cs
- XPathNodeList.cs
- ContentDefinition.cs
- ReflectTypeDescriptionProvider.cs
- RuntimeCompatibilityAttribute.cs
- DirectoryGroupQuery.cs
- DataGridViewUtilities.cs
- ComboBox.cs
- ISFClipboardData.cs
- ByteStreamGeometryContext.cs
- BinHexEncoder.cs
- TableLayoutCellPaintEventArgs.cs
- FactoryId.cs
- StringWriter.cs
- LogReservationCollection.cs
- SecUtil.cs
- WizardForm.cs
- ManageRequest.cs
- SplineQuaternionKeyFrame.cs
- DesignTimeTemplateParser.cs
- TemplateBindingExpression.cs
- PersonalizableAttribute.cs
- DBConnectionString.cs
- DbProviderFactories.cs
- TcpTransportElement.cs
- LastQueryOperator.cs
- EnvelopeVersion.cs
- VectorAnimationUsingKeyFrames.cs
- GridViewColumn.cs
- ActionItem.cs
- PasswordPropertyTextAttribute.cs
- ConnectionPoolManager.cs
- StaticExtensionConverter.cs
- AmbiguousMatchException.cs
- MenuItem.cs
- SelectionProcessor.cs
- MsmqPoisonMessageException.cs
- WaitForChangedResult.cs
- ComponentCache.cs
- XamlVector3DCollectionSerializer.cs
- DbUpdateCommandTree.cs
- XmlUTF8TextReader.cs
- XmlProcessingInstruction.cs
- TextServicesHost.cs
- MetaForeignKeyColumn.cs
- CallTemplateAction.cs
- StyleHelper.cs
- ColorConverter.cs
- TimeoutException.cs
- Positioning.cs
- StatusBarItemAutomationPeer.cs
- SchemaType.cs
- ProfileManager.cs
- EntityContainer.cs
- PathFigureCollectionValueSerializer.cs
- RepeatButtonAutomationPeer.cs
- XmlSerializerOperationGenerator.cs
- AnonymousIdentificationSection.cs
- AutomationPattern.cs
- ListBase.cs
- DataContractSerializerOperationFormatter.cs
- PartialTrustHelpers.cs
- DataChangedEventManager.cs
- Propagator.JoinPropagator.cs