Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SamlDoNotCacheCondition.cs / 1305376 / SamlDoNotCacheCondition.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Xml; using System.IdentityModel.Selectors; public class SamlDoNotCacheCondition : SamlCondition { bool isReadOnly = false; public SamlDoNotCacheCondition() { } public override bool IsReadOnly { get { return this.isReadOnly; } } public override void MakeReadOnly() { this.isReadOnly = true; } public override void ReadXml(XmlDictionaryReader reader, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; if (!reader.IsStartElement(dictionary.DoNotCacheCondition, dictionary.Namespace)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLBadSchema, dictionary.DoNotCacheCondition.Value))); // saml:DoNotCacheCondition is a empty element. So just issue a read for // the empty element. if (reader.IsEmptyElement) { reader.MoveToContent(); reader.Read(); return; } reader.MoveToContent(); reader.Read(); reader.ReadEndElement(); } public override void WriteXml(XmlDictionaryWriter writer, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer) { if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; writer.WriteStartElement(dictionary.PreferredPrefix.Value, dictionary.DoNotCacheCondition, dictionary.Namespace); writer.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Xml; using System.IdentityModel.Selectors; public class SamlDoNotCacheCondition : SamlCondition { bool isReadOnly = false; public SamlDoNotCacheCondition() { } public override bool IsReadOnly { get { return this.isReadOnly; } } public override void MakeReadOnly() { this.isReadOnly = true; } public override void ReadXml(XmlDictionaryReader reader, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; if (!reader.IsStartElement(dictionary.DoNotCacheCondition, dictionary.Namespace)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLBadSchema, dictionary.DoNotCacheCondition.Value))); // saml:DoNotCacheCondition is a empty element. So just issue a read for // the empty element. if (reader.IsEmptyElement) { reader.MoveToContent(); reader.Read(); return; } reader.MoveToContent(); reader.Read(); reader.ReadEndElement(); } public override void WriteXml(XmlDictionaryWriter writer, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer) { if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; writer.WriteStartElement(dictionary.PreferredPrefix.Value, dictionary.DoNotCacheCondition, dictionary.Namespace); writer.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
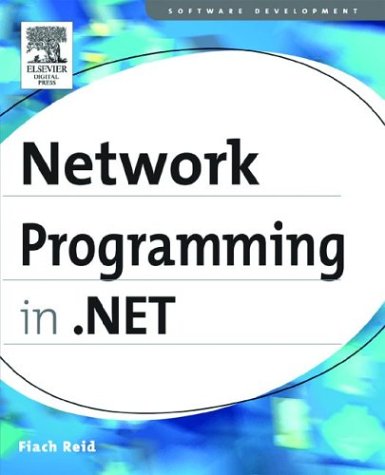
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataBindEngine.cs
- Automation.cs
- MarkupExtensionParser.cs
- DetailsViewInsertEventArgs.cs
- CounterSampleCalculator.cs
- ThrowOnMultipleAssignment.cs
- TransformConverter.cs
- EventLogPermissionEntryCollection.cs
- ViewManager.cs
- AtomMaterializer.cs
- FontUnit.cs
- GlyphTypeface.cs
- SerialReceived.cs
- ScrollPattern.cs
- HttpCacheVary.cs
- DataControlHelper.cs
- SqlNotificationRequest.cs
- RSACryptoServiceProvider.cs
- SessionStateSection.cs
- Attributes.cs
- InputDevice.cs
- SecurityTokenException.cs
- DefaultTypeArgumentAttribute.cs
- InternalConfigRoot.cs
- HttpApplication.cs
- ValidatingReaderNodeData.cs
- CommandDesigner.cs
- WebPart.cs
- HotSpotCollection.cs
- BindingExpression.cs
- MsmqException.cs
- AQNBuilder.cs
- DecoderFallback.cs
- UserControlParser.cs
- StorageMappingItemLoader.cs
- DbProviderFactories.cs
- FlowDocumentView.cs
- WebResourceUtil.cs
- FactoryId.cs
- WindowsGrip.cs
- SystemDiagnosticsSection.cs
- RuleDefinitions.cs
- RectKeyFrameCollection.cs
- TextEncodedRawTextWriter.cs
- CompoundFileStorageReference.cs
- Types.cs
- OracleDateTime.cs
- FlowPosition.cs
- HashHelper.cs
- SynchronousChannel.cs
- GridViewSelectEventArgs.cs
- DesignTimeVisibleAttribute.cs
- BitmapEffectCollection.cs
- RSAPKCS1SignatureDeformatter.cs
- MsmqReceiveHelper.cs
- UnsafeNativeMethodsMilCoreApi.cs
- CompositeFontInfo.cs
- EnglishPluralizationService.cs
- WindowsStatic.cs
- PrivateFontCollection.cs
- Stackframe.cs
- DetailsViewRow.cs
- HtmlHistory.cs
- UndoManager.cs
- SwitchElementsCollection.cs
- UInt32Storage.cs
- ProcessingInstructionAction.cs
- ImmutablePropertyDescriptorGridEntry.cs
- ToolStripItemEventArgs.cs
- ReaderWriterLockWrapper.cs
- RoleGroup.cs
- Pointer.cs
- RotateTransform3D.cs
- NativeMethods.cs
- BufferBuilder.cs
- BooleanToVisibilityConverter.cs
- ArraySet.cs
- GroupBox.cs
- ExecutionEngineException.cs
- TableCellCollection.cs
- CodeCommentStatementCollection.cs
- Pts.cs
- WindowsListViewItem.cs
- SortFieldComparer.cs
- SystemParameters.cs
- ConfigurationCollectionAttribute.cs
- TableAutomationPeer.cs
- WebBrowsableAttribute.cs
- SoapCodeExporter.cs
- AttachedPropertyMethodSelector.cs
- ProcessModule.cs
- SchemaMerger.cs
- Style.cs
- ExpressionEvaluator.cs
- OleDbPropertySetGuid.cs
- ToolStripOverflowButton.cs
- InternalConfigConfigurationFactory.cs
- DataMisalignedException.cs
- TimelineGroup.cs
- DiscoveryExceptionDictionary.cs