Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Activities / Rules / RuleDefinitions.cs / 1305376 / RuleDefinitions.cs
// ---------------------------------------------------------------------------- // Copyright (C) 2006 Microsoft Corporation All Rights Reserved // --------------------------------------------------------------------------- #define CODE_ANALYSIS using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Workflow.ComponentModel; namespace System.Workflow.Activities.Rules { #region class RuleDefinitions public sealed class RuleDefinitions : IWorkflowChangeDiff { [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes")] public static readonly DependencyProperty RuleDefinitionsProperty = DependencyProperty.RegisterAttached("RuleDefinitions", typeof(RuleDefinitions), typeof(RuleDefinitions), new PropertyMetadata(null, DependencyPropertyOptions.Metadata, new GetValueOverride(OnGetRuleConditions), null, new Attribute[] { new DesignerSerializationVisibilityAttribute(DesignerSerializationVisibility.Hidden) })); private RuleConditionCollection conditions; private RuleSetCollection ruleSets; private bool runtimeInitialized; [NonSerialized] private object syncLock = new object(); [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public RuleConditionCollection Conditions { get { if (this.conditions == null) this.conditions = new RuleConditionCollection(); return conditions; } } [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public RuleSetCollection RuleSets { get { if (this.ruleSets == null) this.ruleSets = new RuleSetCollection(); return this.ruleSets; } } internal static object OnGetRuleConditions(DependencyObject dependencyObject) { if (dependencyObject == null) throw new ArgumentNullException("dependencyObject"); RuleDefinitions rules = dependencyObject.GetValueBase(RuleDefinitions.RuleDefinitionsProperty) as RuleDefinitions; if (rules != null) return rules; Activity rootActivity = dependencyObject as Activity; if (rootActivity.Parent == null) { rules = ConditionHelper.GetRuleDefinitionsFromManifest(rootActivity.GetType()); if (rules != null) dependencyObject.SetValue(RuleDefinitions.RuleDefinitionsProperty, rules); } return rules; } internal void OnRuntimeInitialized() { lock (syncLock) { if (runtimeInitialized) return; Conditions.OnRuntimeInitialized(); RuleSets.OnRuntimeInitialized(); runtimeInitialized = true; } } #region IWorkflowChangeDiff Members public IListDiff(object originalDefinition, object changedDefinition) { RuleDefinitions originalRules = originalDefinition as RuleDefinitions; RuleDefinitions changedRules = changedDefinition as RuleDefinitions; if ((originalRules == null) || (changedRules == null)) return new List (); IList cdiff = Conditions.Diff(originalRules.Conditions, changedRules.Conditions); IList rdiff = RuleSets.Diff(originalRules.RuleSets, changedRules.RuleSets); // quick optimization -- if no condition changes, simply return the ruleset changes if (cdiff.Count == 0) return rdiff; // merge ruleset changes into condition changes for (int i = 0; i < rdiff.Count; ++i) { cdiff.Add(rdiff[i]); } return cdiff; } #endregion internal RuleDefinitions Clone() { RuleDefinitions newRuleDefinitions = new RuleDefinitions(); if (this.ruleSets != null) { newRuleDefinitions.ruleSets = new RuleSetCollection(); foreach (RuleSet r in this.ruleSets) newRuleDefinitions.ruleSets.Add(r.Clone()); } if (this.conditions != null) { newRuleDefinitions.conditions = new RuleConditionCollection(); foreach (RuleCondition r in this.conditions) newRuleDefinitions.conditions.Add(r.Clone()); } return newRuleDefinitions; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
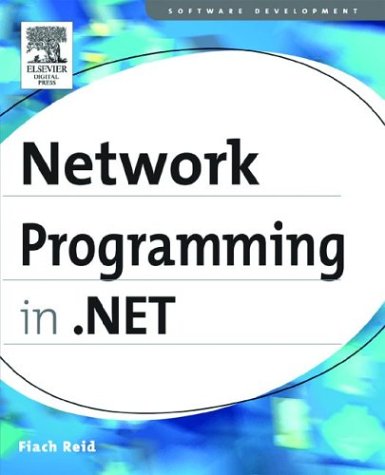
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemoryFailPoint.cs
- DecimalStorage.cs
- StructuralCache.cs
- ASCIIEncoding.cs
- PersonalizationProviderHelper.cs
- ButtonChrome.cs
- MetadataArtifactLoaderResource.cs
- NativeMethodsCLR.cs
- ExistsInCollection.cs
- ThreadAbortException.cs
- RemotingServices.cs
- HideDisabledControlAdapter.cs
- EventSourceCreationData.cs
- bindurihelper.cs
- Message.cs
- PersianCalendar.cs
- WebScriptEnablingBehavior.cs
- Authorization.cs
- SqlRowUpdatedEvent.cs
- SamlAssertionKeyIdentifierClause.cs
- Logging.cs
- BufferModesCollection.cs
- ParsedAttributeCollection.cs
- DataListGeneralPage.cs
- XmlReader.cs
- ListBoxDesigner.cs
- AccessedThroughPropertyAttribute.cs
- SymbolTable.cs
- TrueReadOnlyCollection.cs
- XamlTemplateSerializer.cs
- ValueUtilsSmi.cs
- ExpandedProjectionNode.cs
- NextPreviousPagerField.cs
- Parallel.cs
- BigInt.cs
- ContentDisposition.cs
- EntityCommandDefinition.cs
- HtmlControl.cs
- DateTimeValueSerializerContext.cs
- ProcessProtocolHandler.cs
- InputBindingCollection.cs
- SimpleBitVector32.cs
- baseaxisquery.cs
- DCSafeHandle.cs
- httpserverutility.cs
- CodeMemberProperty.cs
- ExpressionStringBuilder.cs
- TextBoxBase.cs
- LostFocusEventManager.cs
- EditorPart.cs
- IMembershipProvider.cs
- TrustManager.cs
- MetaModel.cs
- storepermission.cs
- CapabilitiesUse.cs
- MethodCallExpression.cs
- VideoDrawing.cs
- CodeMethodReturnStatement.cs
- processwaithandle.cs
- CheckPair.cs
- AppLevelCompilationSectionCache.cs
- PersonalizableAttribute.cs
- WindowsGraphicsCacheManager.cs
- MailSettingsSection.cs
- TextDecorationCollectionConverter.cs
- ScopelessEnumAttribute.cs
- DrawingContextWalker.cs
- FontWeights.cs
- NodeFunctions.cs
- JsonFormatWriterGenerator.cs
- ObjectHelper.cs
- DataGridColumnReorderingEventArgs.cs
- SafeEventLogReadHandle.cs
- DelimitedListTraceListener.cs
- SHA256Managed.cs
- ITextView.cs
- EntityContainer.cs
- DataGridColumnHeadersPresenter.cs
- ToolStripRendererSwitcher.cs
- ComponentCollection.cs
- ReferenceEqualityComparer.cs
- ProxyManager.cs
- JoinTreeNode.cs
- CultureTableRecord.cs
- BamlRecords.cs
- ArgumentValidation.cs
- WebPart.cs
- FragmentNavigationEventArgs.cs
- LoginView.cs
- XpsS0ValidatingLoader.cs
- XmlSchemaSimpleType.cs
- DesignerValidatorAdapter.cs
- ReferenceAssemblyAttribute.cs
- PanelContainerDesigner.cs
- RpcResponse.cs
- ByteKeyFrameCollection.cs
- DurableErrorHandler.cs
- DataColumnChangeEvent.cs
- SchemaImporter.cs
- Icon.cs