Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / ElementAction.cs / 1305376 / ElementAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ElementAction : ContainerAction { private const int NameDone = 2; private Avt nameAvt; private Avt nsAvt; private bool empty; private InputScopeManager manager; // Compile time precalculated AVTs private string name; private string nsUri; private PrefixQName qname; // When we not have AVTs at all we can do this. null otherwise. internal ElementAction() {} private static PrefixQName CreateElementQName(string name, string nsUri, InputScopeManager manager) { if (nsUri == XmlReservedNs.NsXmlNs) { throw XsltException.Create(Res.Xslt_ReservedNS, nsUri); } PrefixQName qname = new PrefixQName(); qname.SetQName(name); if (nsUri == null) { qname.Namespace = manager.ResolveXmlNamespace(qname.Prefix); } else { qname.Namespace = nsUri; } return qname; } internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.nameAvt, "name"); this.name = PrecalculateAvt(ref this.nameAvt); this.nsUri = PrecalculateAvt(ref this.nsAvt ); // if both name and ns are not AVT we can calculate qname at compile time and will not need namespace manager anymore if (this.nameAvt == null && this.nsAvt == null) { if(this.name != "xmlns") { this.qname = CreateElementQName(this.name, this.nsUri, compiler.CloneScopeManager()); } } else { this.manager = compiler.CloneScopeManager(); } if (compiler.Recurse()) { Debug.Assert(this.empty == false); CompileTemplate(compiler); compiler.ToParent(); } this.empty = (this.containedActions == null) ; } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Ref.Equal(name, compiler.Atoms.Name)) { this.nameAvt = Avt.CompileAvt(compiler, value); } else if (Ref.Equal(name, compiler.Atoms.Namespace)) { this.nsAvt = Avt.CompileAvt(compiler, value); } else if (Ref.Equal(name, compiler.Atoms.UseAttributeSets)) { AddAction(compiler.CreateUseAttributeSetsAction()); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: if(this.qname != null) { frame.CalulatedName = this.qname; } else { frame.CalulatedName = CreateElementQName( this.nameAvt == null ? this.name : this.nameAvt.Evaluate(processor, frame), this.nsAvt == null ? this.nsUri : this.nsAvt .Evaluate(processor, frame), this.manager ); } goto case NameDone; case NameDone: { PrefixQName qname = frame.CalulatedName; if (processor.BeginEvent(XPathNodeType.Element, qname.Prefix, qname.Name, qname.Namespace, this.empty) == false) { // Come back later frame.State = NameDone; break; } if (! this.empty) { processor.PushActionFrame(frame); frame.State = ProcessingChildren; break; // Allow children to run } else { goto case ProcessingChildren; } } case ProcessingChildren: if (processor.EndEvent(XPathNodeType.Element) == false) { frame.State = ProcessingChildren; break; } frame.Finished(); break; default: Debug.Fail("Invalid ElementAction execution state"); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
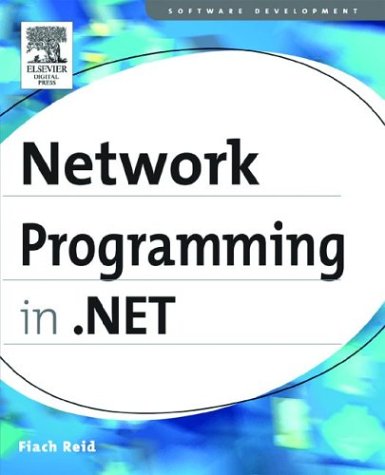
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlInfoMessageEvent.cs
- RemotingServices.cs
- OleDbRowUpdatingEvent.cs
- XmlReaderSettings.cs
- ColumnCollection.cs
- CodeVariableReferenceExpression.cs
- ScanQueryOperator.cs
- ColumnResizeAdorner.cs
- Line.cs
- PreservationFileWriter.cs
- GlyphingCache.cs
- SequenceDesigner.cs
- DataGridRelationshipRow.cs
- EventProviderWriter.cs
- PropertyRecord.cs
- TextElement.cs
- LinkLabelLinkClickedEvent.cs
- Border.cs
- SerializationEventsCache.cs
- Trigger.cs
- SoapExtension.cs
- HtmlTableRowCollection.cs
- Brushes.cs
- FixedTextSelectionProcessor.cs
- NavigateEvent.cs
- PocoEntityKeyStrategy.cs
- DoubleAnimationUsingPath.cs
- CodeDirectiveCollection.cs
- DataPager.cs
- ServiceThrottle.cs
- HtmlTableRow.cs
- DbConnectionOptions.cs
- HtmlGenericControl.cs
- NumericUpDownAcceleration.cs
- CommandField.cs
- BoundField.cs
- InvalidContentTypeException.cs
- XAMLParseException.cs
- ClientRolePrincipal.cs
- Nodes.cs
- BounceEase.cs
- SortAction.cs
- RawStylusSystemGestureInputReport.cs
- CompositeDataBoundControl.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- EntityDataSourceUtil.cs
- DoubleAnimationUsingKeyFrames.cs
- Focus.cs
- SchemaInfo.cs
- AnonymousIdentificationModule.cs
- TextBoxDesigner.cs
- DetailsViewDesigner.cs
- UrlAuthorizationModule.cs
- Console.cs
- RemotingServices.cs
- PenThreadWorker.cs
- DtrList.cs
- XmlBaseWriter.cs
- MergeLocalizationDirectives.cs
- NetworkInformationException.cs
- DockPattern.cs
- SystemDropShadowChrome.cs
- panel.cs
- ListViewPagedDataSource.cs
- SqlUtil.cs
- Tokenizer.cs
- FileDialog_Vista.cs
- TemplateFactory.cs
- ColorConvertedBitmapExtension.cs
- RtfControls.cs
- XamlTemplateSerializer.cs
- MouseActionConverter.cs
- InputLangChangeEvent.cs
- SqlProviderServices.cs
- EntitySqlQueryBuilder.cs
- EnumBuilder.cs
- SqlFileStream.cs
- ConfigurationElementCollection.cs
- ImageMetadata.cs
- DataSourceControl.cs
- TextElement.cs
- UserMapPath.cs
- DataGridViewCellStateChangedEventArgs.cs
- PenContexts.cs
- XmlNode.cs
- ReadOnlyPropertyMetadata.cs
- TextLine.cs
- HMACRIPEMD160.cs
- WebEvents.cs
- XslTransform.cs
- ImageBrush.cs
- ListChangedEventArgs.cs
- SoapSchemaMember.cs
- HtmlFormParameterWriter.cs
- SectionRecord.cs
- ConfigPathUtility.cs
- WindowsPrincipal.cs
- XmlBinaryReaderSession.cs
- OdbcRowUpdatingEvent.cs
- UnmanagedMarshal.cs