Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ServiceThrottle.cs / 1 / ServiceThrottle.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel; using System.Collections.Generic; using System.Globalization; using System.Threading; using System.Runtime.Serialization; interface ISessionThrottleNotification { void ThrottleAcquired(); } public sealed class ServiceThrottle { internal const int DefaultMaxConcurrentCalls = 16; internal const int DefaultMaxConcurrentSessions = 10; FlowThrottle calls; FlowThrottle sessions; QuotaThrottle dynamic; FlowThrottle instanceContexts; ServiceHostBase host; bool isActive; object thisLock = new object(); internal ServiceThrottle(ServiceHostBase host) { if (!((host != null))) { DiagnosticUtility.DebugAssert("ServiceThrottle.ServiceThrottle: (host != null)"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("host"); } this.host = host; this.MaxConcurrentCalls = ServiceThrottle.DefaultMaxConcurrentCalls; this.MaxConcurrentSessions = ServiceThrottle.DefaultMaxConcurrentSessions; this.isActive = true; } FlowThrottle Calls { get { lock (this.ThisLock) { if (this.calls == null) { this.calls = new FlowThrottle(this.GotCall, ServiceThrottle.DefaultMaxConcurrentCalls, ServiceThrottle.MaxConcurrentCallsPropertyName, ServiceThrottle.MaxConcurrentCallsConfigName); } return this.calls; } } } FlowThrottle Sessions { get { lock (this.ThisLock) { if (this.sessions == null) { this.sessions = new FlowThrottle(this.GotSession, ServiceThrottle.DefaultMaxConcurrentSessions, ServiceThrottle.MaxConcurrentSessionsPropertyName, ServiceThrottle.MaxConcurrentSessionsConfigName); } return this.sessions; } } } QuotaThrottle Dynamic { get { lock (this.ThisLock) { if (this.dynamic == null) { this.dynamic = new QuotaThrottle(this.GotDynamic, new object()); this.dynamic.Owner = "ServiceHost"; } this.UpdateIsActive(); return this.dynamic; } } } internal int ManualFlowControlLimit { get { return this.Dynamic.Limit; } set { this.Dynamic.SetLimit(value); } } const string MaxConcurrentCallsPropertyName = "MaxConcurrentCalls"; const string MaxConcurrentCallsConfigName = "maxConcurrentCalls"; public int MaxConcurrentCalls { get { return this.Calls.Capacity; } set { this.ThrowIfClosedOrOpened(MaxConcurrentCallsPropertyName); this.Calls.Capacity = value; this.UpdateIsActive(); } } const string MaxConcurrentSessionsPropertyName = "MaxConcurrentSessions"; const string MaxConcurrentSessionsConfigName = "maxConcurrentSessions"; public int MaxConcurrentSessions { get { return this.Sessions.Capacity; } set { this.ThrowIfClosedOrOpened(MaxConcurrentSessionsPropertyName); this.Sessions.Capacity = value; this.UpdateIsActive(); } } const string MaxConcurrentInstancesPropertyName = "MaxConcurrentInstances"; const string MaxConcurrentInstancesConfigName = "maxConcurrentInstances"; public int MaxConcurrentInstances { get { return this.InstanceContexts.Capacity; } set { this.ThrowIfClosedOrOpened(MaxConcurrentInstancesPropertyName); this.InstanceContexts.Capacity = value; this.UpdateIsActive(); } } FlowThrottle InstanceContexts { get { lock (this.ThisLock) { if (this.instanceContexts == null) { this.instanceContexts = new FlowThrottle(this.GotInstanceContext, Int32.MaxValue, ServiceThrottle.MaxConcurrentInstancesPropertyName, ServiceThrottle.MaxConcurrentInstancesConfigName); } return this.instanceContexts; } } } internal bool IsActive { get { return this.isActive; } } internal object ThisLock { get { return this.thisLock; } } bool PrivateAcquireCall(ChannelHandler channel) { return (this.calls == null) || this.calls.Acquire(channel); } bool PrivateAcquireSessionListenerHandler(ListenerHandler listener) { if ((this.sessions != null) && (listener.Channel != null) && (listener.Channel.Throttle == null)) { listener.Channel.Throttle = this; return this.sessions.Acquire(listener); } else { return true; } } bool PrivateAcquireSession(ISessionThrottleNotification source) { return (this.sessions == null || this.sessions.Acquire(source)); } bool PrivateAcquireDynamic(ChannelHandler channel) { return (this.dynamic == null) || this.dynamic.Acquire(channel); } bool PrivateAcquireInstanceContext(ChannelHandler channel) { if ((this.instanceContexts != null) && (channel.InstanceContext == null)) { channel.InstanceContextServiceThrottle = this; return this.instanceContexts.Acquire(channel); } else { return true; } } internal bool AcquireCall(ChannelHandler channel) { lock (this.ThisLock) { return (this.PrivateAcquireCall(channel)); } } internal bool AcquireInstanceContextAndDynamic(ChannelHandler channel, bool acquireInstanceContextThrottle) { lock(this.ThisLock) { if (!acquireInstanceContextThrottle) { return this.PrivateAcquireDynamic(channel); } else { return (this.PrivateAcquireInstanceContext(channel) && this.PrivateAcquireDynamic(channel)); } } } internal bool AcquireSession(ISessionThrottleNotification source) { lock (this.ThisLock) { return this.PrivateAcquireSession(source); } } internal bool AcquireSession(ListenerHandler listener) { lock (this.ThisLock) { return this.PrivateAcquireSessionListenerHandler(listener); } } void GotCall(object state) { ChannelHandler channel = (ChannelHandler)state; lock (this.ThisLock) { channel.ThrottleAcquiredForCall(); } } void GotDynamic(object state) { ((ChannelHandler)state).ThrottleAcquired(); } void GotInstanceContext(object state) { ChannelHandler channel = (ChannelHandler)state; lock (this.ThisLock) { if (this.PrivateAcquireDynamic(channel)) channel.ThrottleAcquired(); } } void GotSession(object state) { ((ISessionThrottleNotification)state).ThrottleAcquired(); } internal void DeactivateChannel() { if (this.isActive) { if (this.sessions != null) this.sessions.Release(); } } internal void DeactivateCall() { if (this.isActive) { if (this.calls != null) this.calls.Release(); } } internal void DeactivateInstanceContext() { if (this.isActive) { if (this.instanceContexts != null) { this.instanceContexts.Release(); } } } internal int IncrementManualFlowControlLimit(int incrementBy) { return this.Dynamic.IncrementLimit(incrementBy); } void ThrowIfClosedOrOpened(string memberName) { if (this.host.State == CommunicationState.Opened) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SFxImmutableThrottle1, memberName))); } else { this.host.ThrowIfClosedOrOpened(); } } void UpdateIsActive() { this.isActive = ((this.dynamic != null) || ((this.calls != null) && (this.calls.Capacity != Int32.MaxValue)) || ((this.sessions != null) && (this.sessions.Capacity != Int32.MaxValue)) || ((this.instanceContexts != null) && (this.instanceContexts.Capacity != Int32.MaxValue))); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
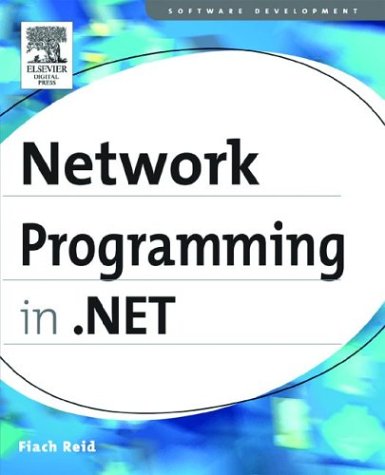
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OutputCacheSettings.cs
- Cursors.cs
- CloseCollectionAsyncResult.cs
- Polygon.cs
- InkCanvasSelectionAdorner.cs
- SafeCertificateContext.cs
- TextInfo.cs
- FilteredDataSetHelper.cs
- SpeakProgressEventArgs.cs
- XPathNodePointer.cs
- ReadOnlyNameValueCollection.cs
- IApplicationTrustManager.cs
- EventSinkHelperWriter.cs
- BrushMappingModeValidation.cs
- HighlightComponent.cs
- HttpWebRequest.cs
- CodeMemberMethod.cs
- APCustomTypeDescriptor.cs
- CodeGenHelper.cs
- Decorator.cs
- TransactionChannel.cs
- MemberCollection.cs
- FormViewInsertedEventArgs.cs
- DataGridTableCollection.cs
- TableLayoutStyleCollection.cs
- DataGridSortCommandEventArgs.cs
- bidPrivateBase.cs
- FormsAuthenticationUserCollection.cs
- AttachedAnnotation.cs
- CollaborationHelperFunctions.cs
- ProfileModule.cs
- UnsafeNativeMethods.cs
- Codec.cs
- DataGridRelationshipRow.cs
- SolidColorBrush.cs
- Page.cs
- TypeValidationEventArgs.cs
- BaseDataBoundControl.cs
- CommandLineParser.cs
- ApplicationTrust.cs
- XamlClipboardData.cs
- BidOverLoads.cs
- ConstructorArgumentAttribute.cs
- BitmapSource.cs
- StylusPointPropertyUnit.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- InteropExecutor.cs
- UnsafeNativeMethodsCLR.cs
- DynamicILGenerator.cs
- HttpHostedTransportConfiguration.cs
- ExtensionCollection.cs
- EndpointInfo.cs
- AnimationStorage.cs
- XmlChildNodes.cs
- PointAnimationUsingPath.cs
- IMembershipProvider.cs
- Formatter.cs
- SQLConvert.cs
- RemotingServices.cs
- SelectionProviderWrapper.cs
- SessionStateSection.cs
- Delegate.cs
- DataError.cs
- MetadataArtifactLoaderFile.cs
- ToolStripItemBehavior.cs
- InternalTypeHelper.cs
- recordstatescratchpad.cs
- CodeIterationStatement.cs
- _ConnectionGroup.cs
- AstNode.cs
- URLMembershipCondition.cs
- KeyboardDevice.cs
- CommandSet.cs
- EncodingDataItem.cs
- SourceLineInfo.cs
- ListDictionaryInternal.cs
- MenuItemCollectionEditor.cs
- Matrix3DValueSerializer.cs
- DataDocumentXPathNavigator.cs
- TablePattern.cs
- ButtonDesigner.cs
- IndependentAnimationStorage.cs
- XmlCollation.cs
- StylusPointCollection.cs
- KnownAssemblyEntry.cs
- XmlSchemaSet.cs
- WorkflowOwnershipException.cs
- ModelTreeEnumerator.cs
- ErrorWrapper.cs
- JsonClassDataContract.cs
- ConfigXmlCDataSection.cs
- FormsAuthenticationConfiguration.cs
- XmlSchemaFacet.cs
- RelationshipSet.cs
- DataGridViewAutoSizeModeEventArgs.cs
- XmlHierarchyData.cs
- TextDecorations.cs
- serverconfig.cs
- EventArgs.cs
- HtmlShimManager.cs