Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Shared / MS / Win32 / UnsafeNativeMethodsCLR.cs / 2 / UnsafeNativeMethodsCLR.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace MS.Win32 { using Accessibility; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Runtime.ConstrainedExecution; using System; using System.Security.Permissions; using System.Collections; using System.IO; using System.Text; using System.Security; using System.Diagnostics; using System.ComponentModel; using MS.Win32 ; //The SecurityHelper class differs between assemblies and could not actually be // shared, so it is duplicated across namespaces to prevent name collision. #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use a class (duplicated across multiple namespaces) from an unknown assembly. #endif using IComDataObject = System.Runtime.InteropServices.ComTypes.IDataObject; internal partial class UnsafeNativeMethods { private struct POINTSTRUCT { public int x; public int y; public POINTSTRUCT(int x, int y) { this.x = x; this.y = y; } } // For some reason "PtrToStructure" requires super high permission. ////// Critical: The code below has a link demand for unmanaged code permission.This code can be used to /// get to data that a pointer points to which can lead to easier data reading. /// [SecurityCritical] public static object PtrToStructure(IntPtr lparam, Type cls) { return Marshal.PtrToStructure(lparam, cls); } // For some reason "StructureToPtr" requires super high permission. ////// Critical: The code below has a link demand for unmanaged code permission.This code can be used to /// write data to arbitrary memory. /// [SecurityCritical] public static void StructureToPtr(object structure, IntPtr ptr, bool fDeleteOld) { Marshal.StructureToPtr(structure, ptr, fDeleteOld); } #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int OleGetClipboard(ref IComDataObject data); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int OleSetClipboard(IComDataObject pDataObj); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int OleFlushClipboard(); #endif ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Uxtheme, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern int GetCurrentThemeName(StringBuilder pszThemeFileName, int dwMaxNameChars, StringBuilder pszColorBuff, int dwMaxColorChars, StringBuilder pszSizeBuff, int cchMaxSizeChars); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.DwmAPI, BestFitMapping = false)] public static extern int DwmIsCompositionEnabled(out Int32 enabled); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern IntPtr GetCurrentThread(); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false)] public static extern int RegisterWindowMessage(string msg); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, EntryPoint = "SetWindowPos", ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true)] public static extern bool SetWindowPos(HandleRef hWnd, HandleRef hWndInsertAfter, int x, int y, int cx, int cy, int flags); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true)] public static extern IntPtr GetWindow(HandleRef hWnd, int uCmd); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, SetLastError = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false)] public static extern int GetClassName(HandleRef hwnd, StringBuilder lpClassName, int nMaxCount); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, SetLastError = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false)] public static extern int MessageBox(HandleRef hWnd, string text, string caption, int type); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Uxtheme, CharSet = CharSet.Auto, BestFitMapping = false, EntryPoint = "SetWindowTheme")] public static extern int CriticalSetWindowTheme(HandleRef hWnd, string subAppName, string subIdList); [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, EntryPoint = "CreateCompatibleBitmap", CharSet = CharSet.Auto)] public static extern IntPtr CreateCompatibleBitmap(HandleRef hDC, int width, int height); ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, EntryPoint = "CreateCompatibleBitmap", CharSet = CharSet.Auto)] public static extern IntPtr CriticalCreateCompatibleBitmap(HandleRef hDC, int width, int height); ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, EntryPoint = "GetStockObject", SetLastError = true, CharSet = CharSet.Auto)] public static extern IntPtr CriticalGetStockObject(int stockObject); ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "FillRect", SetLastError = true, CharSet = CharSet.Auto)] public static extern int CriticalFillRect(IntPtr hdc, ref NativeMethods.RECT rcFill, IntPtr brush); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern int GetBitmapBits(HandleRef hbmp, int cbBuffer, byte[] lpvBits); ////// Critical: This code escalates to unmanaged code permission /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool ShowWindow(HandleRef hWnd, int nCmdShow); ////// Critical: This code escalates to unmanaged code permission /// [SecurityCritical] public static void DeleteObject(HandleRef hObject) { HandleCollector.Remove((IntPtr)hObject, NativeMethods.CommonHandles.GDI); if (!IntDeleteObject(hObject)) { throw new Win32Exception(); } } ////// Critical: This code escalates to unmanaged code permission via a call to IntDeleteObject /// [SecurityCritical] public static bool DeleteObjectNoThrow(HandleRef hObject) { HandleCollector.Remove((IntPtr)hObject, NativeMethods.CommonHandles.GDI); bool result = IntDeleteObject(hObject); int error = Marshal.GetLastWin32Error(); if(!result) { Debug.WriteLine("DeleteObject failed. Error = " + error); } return result; } ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Gdi32, SetLastError=true, ExactSpelling = true, EntryPoint="DeleteObject", CharSet=System.Runtime.InteropServices.CharSet.Auto)] public static extern bool IntDeleteObject(HandleRef hObject); [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern IntPtr SelectObject(HandleRef hdc, IntPtr obj); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Gdi32, EntryPoint="SelectObject", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern IntPtr CriticalSelectObject(HandleRef hdc, IntPtr obj); [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false, SetLastError = true)] public static extern int GetClipboardFormatName(int format, StringBuilder lpString, int cchMax); ////// This code elevates to unmanaged code permission /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false)] public static extern int RegisterClipboardFormat(string format); [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool BitBlt(HandleRef hDC, int x, int y, int nWidth, int nHeight, HandleRef hSrcDC, int xSrc, int ySrc, int dwRop); ////// This code elevates to unmanaged code permission /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="PrintWindow", SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool CriticalPrintWindow(HandleRef hWnd, HandleRef hDC, int flags); ////// This code elevates to unmanaged code permission /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="RedrawWindow", ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool CriticalRedrawWindow(HandleRef hWnd, IntPtr lprcUpdate, IntPtr hrgnUpdate, int flags); [DllImport(ExternDll.Shell32, CharSet=CharSet.Auto, BestFitMapping = false)] public static extern int DragQueryFile(HandleRef hDrop, int iFile, StringBuilder lpszFile, int cch); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Shell32, CharSet=CharSet.Auto, BestFitMapping = false)] public static extern IntPtr ShellExecute(HandleRef hwnd, string lpOperation, string lpFile, string lpParameters, string lpDirectory, int nShowCmd); [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Unicode)] internal class ShellExecuteInfo { public int cbSize; public ShellExecuteFlags fMask; public IntPtr hwnd; public string lpVerb; public string lpFile; public string lpParameters; public string lpDirectory; public int nShow; public IntPtr hInstApp; public IntPtr lpIDList; public string lpClass; public IntPtr hkeyClass; public int dwHotKey; public IntPtr hIcon; public IntPtr hProcess; } [Flags] internal enum ShellExecuteFlags { SEE_MASK_CLASSNAME = 0x00000001, SEE_MASK_CLASSKEY = 0x00000003, SEE_MASK_NOCLOSEPROCESS = 0x00000040, SEE_MASK_FLAG_DDEWAIT = 0x00000100, SEE_MASK_DOENVSUBST = 0x00000200, SEE_MASK_FLAG_NO_UI = 0x00000400, SEE_MASK_UNICODE = 0x00004000, SEE_MASK_NO_CONSOLE = 0x00008000, SEE_MASK_ASYNCOK = 0x00100000, SEE_MASK_HMONITOR = 0x00200000, SEE_MASK_NOZONECHECKS = 0x00800000, SEE_MASK_NOQUERYCLASSSTORE = 0x01000000, SEE_MASK_WAITFORINPUTIDLE = 0x02000000 }; ////// Critical - elevates via SUC. Starts a new process. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Shell32, CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool ShellExecuteEx([In, Out] ShellExecuteInfo lpExecInfo); public const int MB_PRECOMPOSED = 0x00000001; public const int MB_COMPOSITE = 0x00000002; public const int MB_USEGLYPHCHARS = 0x00000004; public const int MB_ERR_INVALID_CHARS = 0x00000008; ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Kernel32, ExactSpelling=true, CharSet=CharSet.Unicode, SetLastError=true)] public static extern int MultiByteToWideChar(int CodePage, int dwFlags, byte[] lpMultiByteStr, int cchMultiByte, [Out, MarshalAs(UnmanagedType.LPWStr)] StringBuilder lpWideCharStr, int cchWideChar); ////// Critical - elevates (via SuppressUnmanagedCodeSecurity). /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Kernel32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Unicode)] public static extern int WideCharToMultiByte(int codePage, int flags, [MarshalAs(UnmanagedType.LPWStr)]string wideStr, int chars, [In,Out]byte[] pOutBytes, int bufferBytes, IntPtr defaultChar, IntPtr pDefaultUsed); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling=true, EntryPoint="RtlMoveMemory", CharSet=CharSet.Unicode)] public static extern void CopyMemoryW(IntPtr pdst, string psrc, int cb); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, EntryPoint = "RtlMoveMemory", CharSet = CharSet.Unicode)] public static extern void CopyMemoryW(IntPtr pdst, char[] psrc, int cb); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling=true, EntryPoint="RtlMoveMemory")] public static extern void CopyMemory(IntPtr pdst, byte[] psrc, int cb); #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation due to an unmanaged code call. Also this /// information can be used to exploit the system. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetKeyboardState", CharSet=CharSet.Auto, SetLastError=true)] private static extern int IntGetKeyboardState(byte [] keystate); [SecurityCritical] public static void GetKeyboardState(byte [] keystate) { if(IntGetKeyboardState(keystate) == 0) { throw new Win32Exception(); } } #endif #if DRT_NATIVEMETHODS [DllImport(ExternDll.User32, ExactSpelling=true, EntryPoint="keybd_event", CharSet=CharSet.Auto)] public static extern void Keybd_event(byte vk, byte scan, int flags, IntPtr extrainfo); #endif ////// Critical - This code elevates to unmanaged code. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport("oleacc.dll")] internal static extern int ObjectFromLresult(IntPtr lResult, ref Guid iid, IntPtr wParam, [In, Out] ref IAccessible ppvObject); ////// Critical - This code elevates to unmanaged code. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport("user32.dll")] internal static extern bool IsWinEventHookInstalled(int winevent); internal static Guid IID_IAccessible = new Guid(0x618736e0, 0x3c3d, 0x11cf, 0x81, 0x0c, 0x00, 0xaa, 0x00, 0x38, 0x9b, 0x71); ////// Critical: This code elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, EntryPoint = "GetModuleFileName", CharSet = CharSet.Auto, BestFitMapping = false, SetLastError = true)] private static extern int IntGetModuleFileName(HandleRef hModule, StringBuilder buffer, int length); ////// Critical: This code elevates to unmanaged code permission by calling into IntGetModuleFileName /// [SecurityCritical] internal static int GetModuleFileName(HandleRef hModule, StringBuilder buffer, int length) { int size = IntGetModuleFileName(hModule, buffer, length); if (size == 0) { throw new Win32Exception(); } return size; } #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern bool TranslateMessage([In, Out] ref System.Windows.Interop.MSG msg); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet=CharSet.Auto)] public static extern IntPtr DispatchMessage([In] ref System.Windows.Interop.MSG msg); #endif #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet=CharSet.Auto, EntryPoint="PostThreadMessage", SetLastError=true)] private static extern int IntPostThreadMessage(int id, int msg, IntPtr wparam, IntPtr lparam); [SecurityCritical] public static void PostThreadMessage(int id, int msg, IntPtr wparam, IntPtr lparam) { if(IntPostThreadMessage(id, msg, wparam, lparam) == 0) { throw new Win32Exception(); } } #endif ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [return: MarshalAs(UnmanagedType.Interface)] [DllImport(ExternDll.Ole32, ExactSpelling = true, PreserveSig = false)] [SecurityCritical, SuppressUnmanagedCodeSecurity] public static extern object CoCreateInstance( [In] ref Guid clsid, [MarshalAs(UnmanagedType.Interface)] object punkOuter, int context, [In] ref Guid iid); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, EntryPoint="OleInitialize")] private static extern int IntOleInitialize(IntPtr val); [SecurityCritical] public static int OleInitialize() { return IntOleInitialize(IntPtr.Zero); } ////// Critical: SUC. Inherently unsafe. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32)] public static extern int CoRegisterPSClsid(ref Guid riid, ref Guid rclsid); [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public extern static bool EnumThreadWindows(int dwThreadId, NativeMethods.EnumThreadWindowsCallback lpfn, HandleRef lParam); ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto, SetLastError=true)] public static extern int OleUninitialize(); [DllImport(ExternDll.Kernel32, EntryPoint="CloseHandle", CharSet=CharSet.Auto, SetLastError=true)] private static extern bool IntCloseHandle(HandleRef handle); /* public static void CloseHandle(HandleRef handle) { HandleCollector.Remove((IntPtr)handle, NativeMethods.CommonHandles.Kernel); if(!IntCloseHandle(handle)) { throw new Win32Exception(); } } */ ////// Critical: Closes a passed in handle, LinkDemand on Marshal.GetLastWin32Error /// [SecurityCritical] public static bool CloseHandleNoThrow(HandleRef handle) { HandleCollector.Remove((IntPtr)handle, NativeMethods.CommonHandles.Kernel); bool result = IntCloseHandle(handle); int error = Marshal.GetLastWin32Error(); if(!result) { Debug.WriteLine("CloseHandle failed. Error = " + error); } return result; } ////// Critical as this code performs an UnmanagedCodeSecurity elevation. /// [SecurityCritical] [DllImport(ExternDll.Ole32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int CreateStreamOnHGlobal(IntPtr hGlobal, bool fDeleteOnRelease, ref System.Runtime.InteropServices.ComTypes.IStream istream); #if BASE_NATIVEMETHODS [DllImport(ExternDll.Gdi32, SetLastError=true, EntryPoint="CreateCompatibleDC", CharSet=CharSet.Auto)] private static extern IntPtr IntCreateCompatibleDC(HandleRef hDC); ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError=true, EntryPoint="CreateCompatibleDC", CharSet=CharSet.Auto)] public static extern IntPtr CriticalCreateCompatibleDC(HandleRef hDC); ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If SupressUnmanagedCodeSecurity attribute is ever added to IntCreateCompatibleDC, we need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] public static IntPtr CreateCompatibleDC(HandleRef hDC) { IntPtr h = IntCreateCompatibleDC(hDC); if(h == IntPtr.Zero) { throw new Win32Exception(); } return HandleCollector.Add(h, NativeMethods.CommonHandles.HDC); } #endif [DllImport(ExternDll.Kernel32, EntryPoint="UnmapViewOfFile", CharSet=CharSet.Auto, SetLastError=true)] private static extern bool IntUnmapViewOfFile(HandleRef pvBaseAddress); /* ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If SupressUnmanagedCodeSecurity attribute is ever added to IntUnmapViewOfFile, we need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] public static void UnmapViewOfFile(HandleRef pvBaseAddress) { HandleCollector.Remove((IntPtr)pvBaseAddress, NativeMethods.CommonHandles.Kernel); if(IntUnmapViewOfFile(pvBaseAddress) == 0) { throw new Win32Exception(); } } */ ////// Critical: Unmaps a file handle, LinkDemand on Marshal.GetLastWin32Error /// [SecurityCritical] public static bool UnmapViewOfFileNoThrow(HandleRef pvBaseAddress) { HandleCollector.Remove((IntPtr)pvBaseAddress, NativeMethods.CommonHandles.Kernel); bool result = IntUnmapViewOfFile(pvBaseAddress); int error = Marshal.GetLastWin32Error(); if(!result) { Debug.WriteLine("UnmapViewOfFile failed. Error = " + error); } return result; } ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical] public static bool EnableWindow(HandleRef hWnd, bool enable) { UnsafeNativeMethods.SetLastError(0); bool result = IntEnableWindow(hWnd, enable); if(!result) { int win32Err = Marshal.GetLastWin32Error(); if(win32Err != 0) { throw new Win32Exception(win32Err); } } return result; } ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical] public static bool EnableWindowNoThrow(HandleRef hWnd, bool enable) { // This method is not throwing because the caller don't want to fail after calling this. // If the window was not previously disabled, the return value is zero, else it is non-zero. return IntEnableWindowNoThrow(hWnd, enable); } ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="EnableWindow", SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool IntEnableWindow(HandleRef hWnd, bool enable); ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="EnableWindow", CharSet=System.Runtime.InteropServices.CharSet.Auto)] public static extern bool IntEnableWindowNoThrow(HandleRef hWnd, bool enable); // GetObject stuff [DllImport(ExternDll.Gdi32, SetLastError=true, CharSet=CharSet.Auto)] public static extern int GetObject(HandleRef hObject, int nSize, [In, Out] NativeMethods.BITMAP bm); ////// Critical: This code returns the window which has focus and elevates to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr GetFocus(); ////// Critical - this code elevates via SUC. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetCursorPos", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern bool IntGetCursorPos([In, Out] NativeMethods.POINT pt); ////// Critical - calls a critical function. /// [SecurityCritical] internal static bool GetCursorPos([In, Out] NativeMethods.POINT pt) { bool returnValue = IntGetCursorPos(pt); if (returnValue == false) { throw new Win32Exception(); } return returnValue; } ////// Critical - this code elevates via SUC. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetCursorPos", ExactSpelling = true, CharSet = CharSet.Auto)] private static extern bool IntTryGetCursorPos([In, Out] NativeMethods.POINT pt); ////// Critical - calls a critical function. /// [SecurityCritical] internal static bool TryGetCursorPos([In, Out] NativeMethods.POINT pt) { bool returnValue = IntTryGetCursorPos(pt); // Sometimes Win32 will fail this call, such as if you are // not running in the interactive desktop. For example, // a secure screen saver may be running. if (returnValue == false) { System.Diagnostics.Debug.WriteLine("GetCursorPos failed!"); pt.x = 0; pt.y = 0; } return returnValue; } #if BASE_NATIVEMETHODS || CORE_NATIVEMETHODS || FRAMEWORK_NATIVEMETHODS ////// Critical:Unmanaged code that gets the state of the keyboard keys /// This can be exploited to get keyboard state. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=System.Runtime.InteropServices.CharSet.Auto)] public static extern int GetWindowThreadProcessId(HandleRef hWnd, out int lpdwProcessId); ////// Critical:Unmanaged code that gets the state of the keyboard keys /// This can be exploited to get keyboard state. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern short GetKeyState(int keyCode); [DllImport(ExternDll.Ole32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, PreserveSig = false)] public static extern void DoDragDrop(IComDataObject dataObject, UnsafeNativeMethods.IOleDropSource dropSource, int allowedEffects, int[] finalEffect); ////// Critical - this code elevates via SUC. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=System.Runtime.InteropServices.CharSet.Auto)] public static extern bool InvalidateRect(HandleRef hWnd, IntPtr rect, bool erase); #endif ////// Critical - this code elevates via SUC. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetWindowText", CharSet=CharSet.Auto, BestFitMapping = false, SetLastError = true)] private static extern int IntGetWindowText(HandleRef hWnd, [Out] StringBuilder lpString, int nMaxCount); ////// SecurityCritical due to a call to SetLastError and calls GetWindowText /// [SecurityCritical] internal static int GetWindowText(HandleRef hWnd, [Out] StringBuilder lpString, int nMaxCount) { SetLastError(0); int returnValue = IntGetWindowText(hWnd, lpString, nMaxCount); if (returnValue == 0) { int win32Err = Marshal.GetLastWin32Error(); if (win32Err != 0) { throw new Win32Exception(win32Err); } } return returnValue; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetWindowTextLength", CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true)] private static extern int IntGetWindowTextLength(HandleRef hWnd); ////// SecurityCritical due to a call to SetLastError /// [SecurityCritical] internal static int GetWindowTextLength(HandleRef hWnd) { SetLastError(0); int returnValue = IntGetWindowTextLength(hWnd); if (returnValue == 0) { int win32Err = Marshal.GetLastWin32Error(); if (win32Err != 0) { throw new Win32Exception(win32Err); } } return returnValue; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalAlloc(int uFlags, IntPtr dwBytes); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalReAlloc(HandleRef handle, IntPtr bytes, int flags); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalLock(HandleRef handle); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern bool GlobalUnlock(HandleRef handle); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalFree(HandleRef handle); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalSize(HandleRef handle); #if BASE_NATIVEMETHODS || CORE_NATIVEMETHODS || FRAMEWORK_NATIVEMETHODS ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet=CharSet.Auto)] public static extern bool ImmSetConversionStatus(HandleRef hIMC, int conversion, int sentence); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet=CharSet.Auto)] public static extern bool ImmGetConversionStatus(HandleRef hIMC, ref int conversion, ref int sentence); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern IntPtr ImmGetContext(HandleRef hWnd); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern bool ImmReleaseContext(HandleRef hWnd, HandleRef hIMC); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet=CharSet.Auto)] public static extern IntPtr ImmAssociateContext(HandleRef hWnd, HandleRef hIMC); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern bool ImmSetOpenStatus(HandleRef hIMC, bool open); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern bool ImmGetOpenStatus(HandleRef hIMC); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern bool ImmNotifyIME(HandleRef hIMC, int dwAction, int dwIndex, int dwValue); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet=CharSet.Auto)] public static extern int ImmGetProperty(HandleRef hkl, int flags); // ImmGetCompositionString for result and composition strings ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmGetCompositionString(HandleRef hIMC, int dwIndex, char[] lpBuf, int dwBufLen); // ImmGetCompositionString for display attributes ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmGetCompositionString(HandleRef hIMC, int dwIndex, byte[] lpBuf, int dwBufLen); // ImmGetCompositionString for clause information ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmGetCompositionString(HandleRef hIMC, int dwIndex, int[] lpBuf, int dwBufLen); // ImmGetCompositionString for query information ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmGetCompositionString(HandleRef hIMC, int dwIndex, IntPtr lpBuf, int dwBufLen); //[DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] //public static extern int ImmSetCompositionFont(HandleRef hIMC, [In, Out] ref NativeMethods.LOGFONT lf); [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmConfigureIME(HandleRef hkl, HandleRef hwnd, int dwData, IntPtr pvoid); [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmConfigureIME(HandleRef hkl, HandleRef hwnd, int dwData, [In] ref NativeMethods.REGISTERWORD registerWord); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmSetCompositionWindow(HandleRef hIMC, [In, Out] ref NativeMethods.COMPOSITIONFORM compform); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmSetCandidateWindow(HandleRef hIMC, [In, Out] ref NativeMethods.CANDIDATEFORM candform); [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern IntPtr ImmGetDefaultIMEWnd(HandleRef hwnd); #endif ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetFocus", ExactSpelling=true, CharSet=CharSet.Auto, SetLastError=true)] private static extern IntPtr IntSetFocus(HandleRef hWnd); ////// Critical - calls IntSetFocus (the real PInvoke method) /// [SecurityCritical] internal static IntPtr SetFocus(HandleRef hWnd) { SetLastError(0); IntPtr retVal = IntSetFocus(hWnd); int errorCode = Marshal.GetLastWin32Error(); if (retVal == IntPtr.Zero) { if (errorCode != 0) { throw new Win32Exception(errorCode); } } return retVal; } ////// Critical - calls IntSetFocus (the real PInvoke method) /// [SecurityCritical] internal static bool TrySetFocus(HandleRef hWnd, ref IntPtr result) { SetLastError(0); result = IntSetFocus(hWnd); int errorCode = Marshal.GetLastWin32Error(); if (result == IntPtr.Zero) { if (errorCode != 0) { return false; } } return true; } ////// Critical: It calls methods with SuppressUnmanagedCodeSecurity attribute, Win32 call /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetParent", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern IntPtr IntGetParent(HandleRef hWnd); ////// Critical - it calls SetLastError, which is Critical. it returns an IntPtr that represents the parent of a given hwnd. /// [SecurityCritical] internal static IntPtr GetParent(HandleRef hWnd) { const int successErrorCode = 0; SetLastError(successErrorCode); IntPtr retVal = IntGetParent(hWnd); int errorCode = Marshal.GetLastWin32Error(); if (retVal == IntPtr.Zero) { if (errorCode != successErrorCode) { throw new Win32Exception(errorCode); } } return retVal; } ////// Critical - This code returns a critical resource and causes unmanaged code elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern IntPtr GetAncestor(HandleRef hWnd, int flags); ////// Critical - This code causes unmanaged code elevation. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern bool IsChild(HandleRef hWndParent, HandleRef hwnd); //***************** // // if you're thinking of enabling either of the functions below. // you should first take a look at SafeSecurityHelper.TransformGlobalRectToLocal & TransformLocalRectToScreen // they likely do what you typically use the function for - and it's safe to use. // if you use the function below - you will get exceptions in partial trust. // anyquestions - email avsee. // //****************** ////// Critical as this code performs an elevation. /// [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] [ SecurityCritical, SuppressUnmanagedCodeSecurity] public static extern IntPtr SetParent(HandleRef hWnd, HandleRef hWndParent); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, EntryPoint = "GetModuleHandle", CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true, SetLastError = true)] private static extern IntPtr IntGetModuleHandle(string modName); ////// Critical as this code performs an elevation. /// [SecurityCritical] internal static IntPtr GetModuleHandle(string modName) { IntPtr retVal = IntGetModuleHandle(modName); if (retVal == IntPtr.Zero) { throw new Win32Exception(); } return retVal; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet=CharSet.Auto)] public static extern IntPtr CallWindowProc(IntPtr wndProc, IntPtr hWnd, int msg, IntPtr wParam, IntPtr lParam); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Unicode, EntryPoint = "DefWindowProcW")] public static extern IntPtr DefWindowProc(IntPtr hWnd, Int32 Msg, IntPtr wParam, IntPtr lParam); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError=true, EntryPoint="GetProcAddress", CharSet=CharSet.Ansi, BestFitMapping=false)] public static extern IntPtr IntGetProcAddress(HandleRef hModule, string lpProcName); ////// Critical - calls IntGetProcAddress (the real PInvoke method) /// [SecurityCritical] public static IntPtr GetProcAddress(HandleRef hModule, string lpProcName) { IntPtr result = IntGetProcAddress(hModule, lpProcName); if(result == IntPtr.Zero) { throw new Win32Exception(); } return result; } // GetProcAddress Note : The lpProcName parameter can identify the DLL function by specifying an ordinal value associated // with the function in the EXPORTS statement. GetProcAddress verifies that the specified ordinal is in // the range 1 through the highest ordinal value exported in the .def file. The function then uses the // ordinal as an index to read the function's address from a function table. If the .def file does not number // the functions consecutively from 1 to N (where N is the number of exported functions), an error can // occur where GetProcAddress returns an invalid, non-NULL address, even though there is no function with the specified ordinal. ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, EntryPoint="GetProcAddress", CharSet=CharSet.Ansi, BestFitMapping=false)] public static extern IntPtr GetProcAddressNoThrow(HandleRef hModule, string lpProcName); ////// Critical: as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, CharSet = CharSet.Unicode)] public static extern IntPtr LoadLibrary(string lpFileName); #if BASE_NATIVEMETHODS || FRAMEWORK_NATIVEMETHODS || CORE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int GetSystemMetrics(int nIndex); #endif ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet=CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, ref NativeMethods.RECT rc, int nUpdate); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, ref int value, int ignore); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, ref bool value, int ignore); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, ref NativeMethods.HIGHCONTRAST_I rc, int nUpdate); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, [In, Out] NativeMethods.NONCLIENTMETRICS metrics, int nUpdate); ////// Critical as this code performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, CharSet = CharSet.Auto, ExactSpelling = true)] public static extern bool GetSystemPowerStatus(ref NativeMethods.SYSTEM_POWER_STATUS systemPowerStatus); ////// Critical - performs an elevation via SUC. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="ClientToScreen", SetLastError=true, ExactSpelling=true, CharSet=CharSet.Auto)] private static extern int IntClientToScreen(HandleRef hWnd, [In, Out] NativeMethods.POINT pt); ////// Critical calls critical code - IntClientToScreen /// [SecurityCritical] public static void ClientToScreen(HandleRef hWnd, [In, Out] NativeMethods.POINT pt) { if(IntClientToScreen(hWnd, pt) == 0) { throw new Win32Exception(); } } [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr GetDesktopWindow(); ////// Critical:Elevates to Unmanaged code permission and can be used to /// change the foreground window. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr GetForegroundWindow(); [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int RegisterDragDrop(HandleRef hwnd, UnsafeNativeMethods.IOleDropTarget target); [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int RevokeDragDrop(HandleRef hwnd); ////// Critical:Elevates to Unmanaged code permission and can be used to /// get information of messages in queues. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet=CharSet.Auto)] public static extern bool PeekMessage([In, Out] ref System.Windows.Interop.MSG msg, HandleRef hwnd, int msgMin, int msgMax, int remove); #if BASE_NATIVEMETHODS [DllImport(ExternDll.User32, BestFitMapping = false, CharSet=CharSet.Auto)] public static extern bool SetProp(HandleRef hWnd, string propName, HandleRef data); #endif ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "PostMessage", CharSet = CharSet.Auto, SetLastError = true)] private static extern bool IntPostMessage(HandleRef hwnd, int msg, IntPtr wparam, IntPtr lparam); ////// Critical as this code performs an elevation. /// [SecurityCritical] internal static void PostMessage(HandleRef hwnd, int msg, IntPtr wparam, IntPtr lparam) { if (!IntPostMessage(hwnd, msg, wparam, lparam)) { throw new Win32Exception(); } } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "PostMessage", CharSet = CharSet.Auto)] internal static extern bool TryPostMessage(HandleRef hwnd, int msg, IntPtr wparam, IntPtr lparam); #if BASE_NATIVEMETHODS || CORE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern void NotifyWinEvent(int winEvent, HandleRef hwnd, int objType, int objID); #endif ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, EntryPoint = "BeginPaint", CharSet = CharSet.Auto)] private static extern IntPtr IntBeginPaint(HandleRef hWnd, [In, Out] ref NativeMethods.PAINTSTRUCT lpPaint); ////// Critical as this code performs an elevation. via the call to IntBeginPaint /// [SecurityCritical] public static IntPtr BeginPaint(HandleRef hWnd, [In, Out, MarshalAs(UnmanagedType.LPStruct)] ref NativeMethods.PAINTSTRUCT lpPaint) { return HandleCollector.Add(IntBeginPaint(hWnd, ref lpPaint), NativeMethods.CommonHandles.HDC); } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, EntryPoint = "EndPaint", CharSet = CharSet.Auto)] private static extern bool IntEndPaint(HandleRef hWnd, ref NativeMethods.PAINTSTRUCT lpPaint); ////// Critical as this code performs an elevation via the call to IntEndPaint. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] public static bool EndPaint(HandleRef hWnd, [In, MarshalAs(UnmanagedType.LPStruct)] ref NativeMethods.PAINTSTRUCT lpPaint) { HandleCollector.Remove(lpPaint.hdc, NativeMethods.CommonHandles.HDC); return IntEndPaint(hWnd, ref lpPaint); } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling = true, EntryPoint = "GetDC", CharSet = CharSet.Auto)] private static extern IntPtr IntGetDC(HandleRef hWnd); ////// Critical as this code performs an elevation. The call to handle collector is /// by itself not dangerous because handle collector simply /// stores a count of the number of instances of a given /// handle and not the handle itself. /// [SecurityCritical] public static IntPtr GetDC(HandleRef hWnd) { IntPtr hDc = IntGetDC(hWnd); if(hDc == IntPtr.Zero) { throw new Win32Exception(); } return HandleCollector.Add(hDc, NativeMethods.CommonHandles.HDC); } ////// Critical as this code performs an elevation.The call to handle collector /// is by itself not dangerous because handle collector simply /// stores a count of the number of instances of a given handle and not the handle itself. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, EntryPoint = "ReleaseDC", CharSet = CharSet.Auto)] private static extern int IntReleaseDC(HandleRef hWnd, HandleRef hDC); ////// Critical as this code performs an elevation. /// [SecurityCritical] public static int ReleaseDC(HandleRef hWnd, HandleRef hDC) { HandleCollector.Remove((IntPtr)hDC, NativeMethods.CommonHandles.HDC); return IntReleaseDC(hWnd, hDC); } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int GetDeviceCaps(HandleRef hDC, int nIndex); ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr GetActiveWindow(); ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern bool SetForegroundWindow(HandleRef hWnd); // Begin API Additions to support common dialog controls ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Comdlg32, SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] internal static extern int CommDlgExtendedError(); ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Comdlg32, SetLastError = true, CharSet = CharSet.Unicode)] internal static extern bool GetOpenFileName([In, Out] NativeMethods.OPENFILENAME_I ofn); ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Comdlg32, SetLastError = true, CharSet = CharSet.Unicode)] internal static extern bool GetSaveFileName([In, Out] NativeMethods.OPENFILENAME_I ofn); // End Common Dialog API Additions ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [return:MarshalAs(UnmanagedType.Bool)] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto, SetLastError=true)] public static extern bool SetLayeredWindowAttributes(HandleRef hwnd, int crKey, byte bAlpha, int dwFlags); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [return: MarshalAs(UnmanagedType.Bool)] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern bool UpdateLayeredWindow(IntPtr hwnd, IntPtr hdcDst, NativeMethods.POINT pptDst, NativeMethods.POINT pSizeDst, IntPtr hdcSrc, NativeMethods.POINT pptSrc, int crKey, ref NativeMethods.BLENDFUNCTION pBlend, int dwFlags); [DllImport(ExternDll.User32, EntryPoint = "SetActiveWindow", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern IntPtr IntSetActiveWindow(HandleRef hWnd); ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If SupressUnmanagedCodeSecurity attribute is ever added to IntSetActiveWindow, we need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] internal static IntPtr SetActiveWindow(HandleRef hWnd) { IntPtr retVal = IntSetActiveWindow(hWnd); if (retVal == IntPtr.Zero) { throw new Win32Exception(); } return retVal; } // #if PBTCOMPILER [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr SetCursor(HandleRef hcursor); #endif ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, EntryPoint="DestroyCursor", CharSet=CharSet.Auto)] private static extern bool IntDestroyCursor(IntPtr hCurs); ////// Critical calls IntDestroyCursor /// [SecurityCritical] public static bool DestroyCursor(IntPtr hCurs) { return IntDestroyCursor(hCurs); } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="DestroyIcon", CharSet=System.Runtime.InteropServices.CharSet.Auto, SetLastError=true)] private static extern bool IntDestroyIcon(IntPtr hIcon); ////// Critical: calls a critical method (IntDestroyIcon) /// [SecurityCritical] public static bool DestroyIcon(IntPtr hIcon) { bool result = IntDestroyIcon(hIcon); int error = Marshal.GetLastWin32Error(); if(!result) { // To be consistent with out other PInvoke wrappers // we should "throw" here. But we don't want to // introduce new "throws" w/o time to follow up on any // new problems that causes. Debug.WriteLine("DestroyIcon failed. Error = " + error); //throw new Win32Exception(); } return result; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, EntryPoint="DeleteObject", CharSet=System.Runtime.InteropServices.CharSet.Auto, SetLastError=true)] private static extern bool IntDeleteObject(IntPtr hObject); ////// Critical: calls a critical method (IntDeleteObject) /// [SecurityCritical] public static bool DeleteObject(IntPtr hObject) { bool result = IntDeleteObject(hObject); int error = Marshal.GetLastWin32Error(); if(!result) { // To be consistent with out other PInvoke wrappers // we should "throw" here. But we don't want to // introduce new "throws" w/o time to follow up on any // new problems that causes. Debug.WriteLine("DeleteObject failed. Error = " + error); //throw new Win32Exception(); } return result; } ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto, EntryPoint = "CreateDIBSection")] private static extern NativeMethods.BitmapHandle PrivateCreateDIBSection(HandleRef hdc, ref NativeMethods.BITMAPINFO bitmapInfo, int iUsage, ref IntPtr ppvBits, IntPtr hSection, int dwOffset); ////// Critical - The method invokes PrivateCreateDIBSection. /// [SecurityCritical] internal static NativeMethods.BitmapHandle CreateDIBSection(HandleRef hdc, ref NativeMethods.BITMAPINFO bitmapInfo, int iUsage, ref IntPtr ppvBits, IntPtr hSection, int dwOffset) { NativeMethods.BitmapHandle hBitmap = PrivateCreateDIBSection(hdc, ref bitmapInfo, iUsage, ref ppvBits, hSection, dwOffset); int error = Marshal.GetLastWin32Error(); if ( hBitmap.IsInvalid ) { Debug.WriteLine("CreateDIBSection failed. Error = " + error); } return hBitmap; } ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto, EntryPoint = "CreateBitmap")] private static extern NativeMethods.BitmapHandle PrivateCreateBitmap(int width, int height, int planes, int bitsPerPixel, byte[] lpvBits); ////// Critical - The method invokes PrivateCreateBitmap. /// [SecurityCritical] internal static NativeMethods.BitmapHandle CreateBitmap(int width, int height, int planes, int bitsPerPixel, byte[] lpvBits) { NativeMethods.BitmapHandle hBitmap = PrivateCreateBitmap(width, height, planes, bitsPerPixel, lpvBits); int error = Marshal.GetLastWin32Error(); if ( hBitmap.IsInvalid ) { Debug.WriteLine("CreateBitmap failed. Error = " + error); } return hBitmap; } ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto, EntryPoint = "DestroyIcon")] private static extern bool PrivateDestroyIcon(HandleRef handle); ////// Critical - The method invokes PrivateDestroyIcon. /// [SecurityCritical] internal static bool DestroyIcon(HandleRef handle) { HandleCollector.Remove((IntPtr)handle, NativeMethods.CommonHandles.Icon); bool result = PrivateDestroyIcon(handle); int error = Marshal.GetLastWin32Error(); if ( !result ) { Debug.WriteLine("DestroyIcon failed. Error = " + error); } return result; } ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto, EntryPoint = "CreateIconIndirect")] private static extern NativeMethods.IconHandle PrivateCreateIconIndirect([In, MarshalAs(UnmanagedType.LPStruct)]NativeMethods.ICONINFO iconInfo); ////// Critical - The method invokes PrivateCreateIconIndirect. /// [SecurityCritical] internal static NativeMethods.IconHandle CreateIconIndirect([In, MarshalAs(UnmanagedType.LPStruct)]NativeMethods.ICONINFO iconInfo) { NativeMethods.IconHandle hIcon = PrivateCreateIconIndirect(iconInfo); int error = Marshal.GetLastWin32Error(); if ( hIcon.IsInvalid ) { Debug.WriteLine("CreateIconIndirect failed. Error = " + error); } return hIcon; } ////// Critical: This code elevates to unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern bool IsWindow(HandleRef hWnd); #if BASE_NATIVEMETHODS [DllImport(ExternDll.Gdi32, SetLastError=true, ExactSpelling=true, EntryPoint="DeleteDC", CharSet=CharSet.Auto)] private static extern bool IntDeleteDC(HandleRef hDC); ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If SupressUnmanagedCodeSecurity attribute is ever added to IntDeleteDC, we need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] public static void DeleteDC(HandleRef hDC) { HandleCollector.Remove((IntPtr)hDC, NativeMethods.CommonHandles.HDC); if(!IntDeleteDC(hDC)) { throw new Win32Exception(); } } ////// Critical: This code elevates to unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError=true, ExactSpelling=true, EntryPoint="DeleteDC", CharSet=CharSet.Auto)] private static extern bool IntCriticalDeleteDC(HandleRef hDC); ////// Critical: This code elevates to unmanaged code /// [SecurityCritical] public static void CriticalDeleteDC(HandleRef hDC) { HandleCollector.Remove((IntPtr)hDC, NativeMethods.CommonHandles.HDC); if(!IntCriticalDeleteDC(hDC)) { throw new Win32Exception(); } } #endif #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError=true, EntryPoint="GetMessageW", ExactSpelling=true, CharSet=CharSet.Unicode)] private static extern int IntGetMessageW([In, Out] ref System.Windows.Interop.MSG msg, HandleRef hWnd, int uMsgFilterMin, int uMsgFilterMax); ////// Critical - calls IntGetMessageW (the real PInvoke method) /// [SecurityCritical] public static bool GetMessageW([In, Out] ref System.Windows.Interop.MSG msg, HandleRef hWnd, int uMsgFilterMin, int uMsgFilterMax) { bool boolResult = false; int result = IntGetMessageW(ref msg, hWnd, uMsgFilterMin, uMsgFilterMax); if(result == -1) { throw new Win32Exception(); } else if(result == 0) { boolResult = false; } else { boolResult = true; } return boolResult; } #endif #if BASE_NATIVEMETHODS ////// Critical: This code elevates via a SUC to call into unmanaged Code and can get the HWND of windows at any arbitrary point on the screen /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="WindowFromPoint", ExactSpelling=true, CharSet=CharSet.Auto)] private static extern IntPtr IntWindowFromPoint(POINTSTRUCT pt); ////// Critical: This calls WindowFromPoint(POINTSTRUCT) which is marked SecurityCritical /// [SecurityCritical] public static IntPtr WindowFromPoint(int x, int y) { POINTSTRUCT ps = new POINTSTRUCT(x, y); return IntWindowFromPoint(ps); } #endif ////// Critical: This code elevates to call into unmanaged Code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="CreateWindowEx", CharSet=CharSet.Auto, BestFitMapping = false, SetLastError=true)] public static extern IntPtr IntCreateWindowEx(int dwExStyle, string lpszClassName, string lpszWindowName, int style, int x, int y, int width, int height, HandleRef hWndParent, HandleRef hMenu, HandleRef hInst, [MarshalAs(UnmanagedType.AsAny)] object pvParam); ////// Critical: This code elevates to call into unmanaged Code by calling IntCreateWindowEx /// [SecurityCritical] public static IntPtr CreateWindowEx(int dwExStyle, string lpszClassName, string lpszWindowName, int style, int x, int y, int width, int height, HandleRef hWndParent, HandleRef hMenu, HandleRef hInst, [MarshalAs(UnmanagedType.AsAny)]object pvParam) { IntPtr retVal = IntCreateWindowEx(dwExStyle, lpszClassName, lpszWindowName, style, x, y, width, height, hWndParent, hMenu, hInst, pvParam); if(retVal == IntPtr.Zero) { throw new Win32Exception(); } return retVal; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, EntryPoint="DestroyWindow", CharSet=CharSet.Auto)] public static extern bool IntDestroyWindow(HandleRef hWnd); ////// Critical - calls Security Critical method /// [SecurityCritical] public static void DestroyWindow(HandleRef hWnd) { if(!IntDestroyWindow(hWnd)) { throw new Win32Exception(); } } ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32)] internal static extern IntPtr SetWinEventHook(int eventMin, int eventMax, IntPtr hmodWinEventProc, NativeMethods.WinEventProcDef WinEventReentrancyFilter, uint idProcess, uint idThread, int dwFlags); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32)] internal static extern bool UnhookWinEvent(IntPtr winEventHook); // for GetUserNameEx public enum EXTENDED_NAME_FORMAT { NameUnknown = 0, NameFullyQualifiedDN = 1, NameSamCompatible = 2, NameDisplay = 3, NameUniqueId = 6, NameCanonical = 7, NameUserPrincipal = 8, NameCanonicalEx = 9, NameServicePrincipal = 10 } [ComImport(), Guid("00000122-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleDropTarget { [PreserveSig] int OleDragEnter( [In, MarshalAs(UnmanagedType.Interface)] object pDataObj, [In, MarshalAs(UnmanagedType.U4)] int grfKeyState, [In, MarshalAs(UnmanagedType.U8)] long pt, [In, Out] ref int pdwEffect); [PreserveSig] int OleDragOver( [In, MarshalAs(UnmanagedType.U4)] int grfKeyState, [In, MarshalAs(UnmanagedType.U8)] long pt, [In, Out] ref int pdwEffect); [PreserveSig] int OleDragLeave(); [PreserveSig] int OleDrop( [In, MarshalAs(UnmanagedType.Interface)] object pDataObj, [In, MarshalAs(UnmanagedType.U4)] int grfKeyState, [In, MarshalAs(UnmanagedType.U8)] long pt, [In, Out] ref int pdwEffect); } [ComImport(), Guid("00000121-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleDropSource { [PreserveSig] int OleQueryContinueDrag( int fEscapePressed, [In, MarshalAs(UnmanagedType.U4)] int grfKeyState); [PreserveSig] int OleGiveFeedback( [In, MarshalAs(UnmanagedType.U4)] int dwEffect); } [ ComImport(), Guid("B196B289-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown) ] public interface IOleControlSite { [PreserveSig] int OnControlInfoChanged(); [PreserveSig] int LockInPlaceActive(int fLock); [PreserveSig] int GetExtendedControl( [Out, MarshalAs(UnmanagedType.IDispatch)] out object ppDisp); [PreserveSig] int TransformCoords( [In, Out] NativeMethods.POINT pPtlHimetric, [In, Out] NativeMethods.POINTF pPtfContainer, [In, MarshalAs(UnmanagedType.U4)] int dwFlags); [PreserveSig] int TranslateAccelerator( [In] ref System.Windows.Interop.MSG pMsg, [In, MarshalAs(UnmanagedType.U4)] int grfModifiers); [PreserveSig] int OnFocus(int fGotFocus); [PreserveSig] int ShowPropertyFrame(); } [ComImport(), Guid("00000118-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleClientSite { [PreserveSig] int SaveObject(); [PreserveSig] int GetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwAssign, [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [Out, MarshalAs(UnmanagedType.Interface)] out object moniker); [PreserveSig] int GetContainer(out IOleContainer container); [PreserveSig] int ShowObject(); [PreserveSig] int OnShowWindow(int fShow); [PreserveSig] int RequestNewObjectLayout(); } [ComImport(), Guid("00000119-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceSite { IntPtr GetWindow(); [PreserveSig] int ContextSensitiveHelp(int fEnterMode); [PreserveSig] int CanInPlaceActivate(); [PreserveSig] int OnInPlaceActivate(); [PreserveSig] int OnUIActivate(); [PreserveSig] int GetWindowContext( [Out, MarshalAs(UnmanagedType.Interface)] out UnsafeNativeMethods.IOleInPlaceFrame ppFrame, [Out, MarshalAs(UnmanagedType.Interface)] out UnsafeNativeMethods.IOleInPlaceUIWindow ppDoc, [Out] NativeMethods.COMRECT lprcPosRect, [Out] NativeMethods.COMRECT lprcClipRect, [In, Out] NativeMethods.OLEINPLACEFRAMEINFO lpFrameInfo); [PreserveSig] int Scroll( NativeMethods.SIZE scrollExtant); [PreserveSig] int OnUIDeactivate( int fUndoable); [PreserveSig] int OnInPlaceDeactivate(); [PreserveSig] int DiscardUndoState(); [PreserveSig] int DeactivateAndUndo(); [PreserveSig] int OnPosRectChange( [In] NativeMethods.COMRECT lprcPosRect); } [ComImport(), Guid("9BFBBC02-EFF1-101A-84ED-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IPropertyNotifySink { void OnChanged(int dispID); [PreserveSig] int OnRequestEdit(int dispID); } [ComImport(), Guid("00000100-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IEnumUnknown { [PreserveSig] int Next( [In, MarshalAs(UnmanagedType.U4)] int celt, [Out] IntPtr rgelt, IntPtr pceltFetched); [PreserveSig] int Skip( [In, MarshalAs(UnmanagedType.U4)] int celt); void Reset(); void Clone( [Out] out IEnumUnknown ppenum); } [ComImport(), Guid("0000011B-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleContainer { [PreserveSig] int ParseDisplayName( [In, MarshalAs(UnmanagedType.Interface)] object pbc, [In, MarshalAs(UnmanagedType.BStr)] string pszDisplayName, [Out, MarshalAs(UnmanagedType.LPArray)] int[] pchEaten, [Out, MarshalAs(UnmanagedType.LPArray)] object[] ppmkOut); [PreserveSig] int EnumObjects( [In, MarshalAs(UnmanagedType.U4)] int grfFlags, [Out] out IEnumUnknown ppenum); [PreserveSig] int LockContainer( bool fLock); } [ComImport(), Guid("00000116-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceFrame { IntPtr GetWindow(); [PreserveSig] int ContextSensitiveHelp(int fEnterMode); [PreserveSig] int GetBorder( [Out] NativeMethods.COMRECT lprectBorder); [PreserveSig] int RequestBorderSpace( [In] NativeMethods.COMRECT pborderwidths); [PreserveSig] int SetBorderSpace( [In] NativeMethods.COMRECT pborderwidths); [PreserveSig] int SetActiveObject( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleInPlaceActiveObject pActiveObject, [In, MarshalAs(UnmanagedType.LPWStr)] string pszObjName); [PreserveSig] int InsertMenus( [In] IntPtr hmenuShared, [In, Out] NativeMethods.tagOleMenuGroupWidths lpMenuWidths); [PreserveSig] int SetMenu( [In] IntPtr hmenuShared, [In] IntPtr holemenu, [In] IntPtr hwndActiveObject); [PreserveSig] int RemoveMenus( [In] IntPtr hmenuShared); [PreserveSig] int SetStatusText( [In, MarshalAs(UnmanagedType.LPWStr)] string pszStatusText); [PreserveSig] int EnableModeless( bool fEnable); [PreserveSig] int TranslateAccelerator( [In] ref System.Windows.Interop.MSG lpmsg, [In, MarshalAs(UnmanagedType.U2)] short wID); } //IMPORTANT: Do not try to optimize perf here by changing the enum size to byte //instead of int since this is used in COM Interop for browser hosting scenarios // Enum for OLECMDIDs used by IOleCommandTarget in browser hosted scenarios // Imported from the published header - docobj.h, If you need to support more // than these OLECMDS, add it from that header file public enum OLECMDID { OLECMDID_SAVE = 3, OLECMDID_SAVEAS = 4, OLECMDID_PRINT = 6, OLECMDID_PRINTPREVIEW = 7, OLECMDID_PAGESETUP = 8, OLECMDID_PROPERTIES = 10, OLECMDID_CUT = 11, OLECMDID_COPY = 12, OLECMDID_PASTE = 13, OLECMDID_SELECTALL = 17, OLECMDID_REFRESH = 22, OLECMDID_STOP = 23, } public enum OLECMDEXECOPT { OLECMDEXECOPT_DODEFAULT = 0, OLECMDEXECOPT_PROMPTUSER = 1, OLECMDEXECOPT_DONTPROMPTUSER = 2, OLECMDEXECOPT_SHOWHELP = 3 } // OLECMDID Flags used by IOleCommandTarget to specify status of commands in browser hosted scenarios // Imported from the published header - docobj.h public enum OLECMDF { ////// The command is supported by this object /// OLECMDF_SUPPORTED = 0x1, ////// The command is available and enabled /// OLECMDF_ENABLED = 0x2, ////// The command is an on-off toggle and is currently on /// OLECMDF_LATCHED = 0x4, ////// Reserved for future use /// OLECMDF_NINCHED = 0x8, ////// Command is invisible /// OLECMDF_INVISIBLE = 0x10, ////// Command should not be displayed in the context menu /// OLECMDF_DEFHIDEONCTXTMENU = 0x20 } [ComImport(), Guid("00000115-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceUIWindow { IntPtr GetWindow(); [PreserveSig] int ContextSensitiveHelp( int fEnterMode); [PreserveSig] int GetBorder( [Out] NativeMethods.RECT lprectBorder); [PreserveSig] int RequestBorderSpace( [In] NativeMethods.RECT pborderwidths); [PreserveSig] int SetBorderSpace( [In] NativeMethods.RECT pborderwidths); void SetActiveObject( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleInPlaceActiveObject pActiveObject, [In, MarshalAs(UnmanagedType.LPWStr)] string pszObjName); } [ComImport(), Guid("00000117-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceActiveObject { ////// Critical: SUC. Exposes a native window handle. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [PreserveSig] int GetWindow(out IntPtr hwnd); void ContextSensitiveHelp( int fEnterMode); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [PreserveSig] int TranslateAccelerator( [In] ref System.Windows.Interop.MSG lpmsg); void OnFrameWindowActivate( int fActivate); void OnDocWindowActivate( int fActivate); void ResizeBorder( [In] NativeMethods.RECT prcBorder, [In] UnsafeNativeMethods.IOleInPlaceUIWindow pUIWindow, bool fFrameWindow); void EnableModeless( int fEnable); } [ComImport(), Guid("00000114-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleWindow { [PreserveSig] int GetWindow( [Out]out IntPtr hwnd ); void ContextSensitiveHelp( int fEnterMode); } ////// Critical - elevates via a SUC. /// [ SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("00000113-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceObject { [PreserveSig] int GetWindow( [Out]out IntPtr hwnd ); void ContextSensitiveHelp( int fEnterMode); void InPlaceDeactivate(); [PreserveSig] int UIDeactivate(); void SetObjectRects( [In] NativeMethods.COMRECT lprcPosRect, [In] NativeMethods.COMRECT lprcClipRect); void ReactivateAndUndo(); } ////// Critical - elevates via a SUC. /// [SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("00000112-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleObject { [PreserveSig] int SetClientSite( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleClientSite pClientSite); UnsafeNativeMethods.IOleClientSite GetClientSite(); [PreserveSig] int SetHostNames( [In, MarshalAs(UnmanagedType.LPWStr)] string szContainerApp, [In, MarshalAs(UnmanagedType.LPWStr)] string szContainerObj); [PreserveSig] int Close( int dwSaveOption); [PreserveSig] int SetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [In, MarshalAs(UnmanagedType.Interface)] object pmk); [PreserveSig] int GetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwAssign, [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [Out, MarshalAs(UnmanagedType.Interface)] out object moniker); [PreserveSig] int InitFromData( [In, MarshalAs(UnmanagedType.Interface)] IComDataObject pDataObject, int fCreation, [In, MarshalAs(UnmanagedType.U4)] int dwReserved); [PreserveSig] int GetClipboardData( [In, MarshalAs(UnmanagedType.U4)] int dwReserved, out IComDataObject data); [PreserveSig] int DoVerb( int iVerb, [In] IntPtr lpmsg, [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleClientSite pActiveSite, int lindex, IntPtr hwndParent, [In] NativeMethods.COMRECT lprcPosRect); [PreserveSig] int EnumVerbs(out UnsafeNativeMethods.IEnumOLEVERB e); [PreserveSig] int OleUpdate(); [PreserveSig] int IsUpToDate(); [PreserveSig] int GetUserClassID( [In, Out] ref Guid pClsid); [PreserveSig] int GetUserType( [In, MarshalAs(UnmanagedType.U4)] int dwFormOfType, [Out, MarshalAs(UnmanagedType.LPWStr)] out string userType); [PreserveSig] int SetExtent( [In, MarshalAs(UnmanagedType.U4)] int dwDrawAspect, [In] NativeMethods.SIZE pSizel); [PreserveSig] int GetExtent( [In, MarshalAs(UnmanagedType.U4)] int dwDrawAspect, [Out] NativeMethods.SIZE pSizel); [PreserveSig] int Advise( IAdviseSink pAdvSink, out int cookie); [PreserveSig] int Unadvise( [In, MarshalAs(UnmanagedType.U4)] int dwConnection); [PreserveSig] int EnumAdvise(out IEnumSTATDATA e); [PreserveSig] int GetMiscStatus( [In, MarshalAs(UnmanagedType.U4)] int dwAspect, out int misc); [PreserveSig] int SetColorScheme( [In] NativeMethods.tagLOGPALETTE pLogpal); } [ComImport(), Guid("1C2056CC-5EF4-101B-8BC8-00AA003E3B29"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceObjectWindowless { [PreserveSig] int SetClientSite( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleClientSite pClientSite); [PreserveSig] int GetClientSite(out UnsafeNativeMethods.IOleClientSite site); [PreserveSig] int SetHostNames( [In, MarshalAs(UnmanagedType.LPWStr)] string szContainerApp, [In, MarshalAs(UnmanagedType.LPWStr)] string szContainerObj); [PreserveSig] int Close( int dwSaveOption); [PreserveSig] int SetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [In, MarshalAs(UnmanagedType.Interface)] object pmk); [PreserveSig] int GetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwAssign, [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [Out, MarshalAs(UnmanagedType.Interface)] out object moniker); [PreserveSig] int InitFromData( [In, MarshalAs(UnmanagedType.Interface)] IComDataObject pDataObject, int fCreation, [In, MarshalAs(UnmanagedType.U4)] int dwReserved); [PreserveSig] int GetClipboardData( [In, MarshalAs(UnmanagedType.U4)] int dwReserved, out IComDataObject data); [PreserveSig] int DoVerb( int iVerb, [In] IntPtr lpmsg, [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleClientSite pActiveSite, int lindex, IntPtr hwndParent, [In] NativeMethods.RECT lprcPosRect); [PreserveSig] int EnumVerbs(out UnsafeNativeMethods.IEnumOLEVERB e); [PreserveSig] int OleUpdate(); [PreserveSig] int IsUpToDate(); [PreserveSig] int GetUserClassID( [In, Out] ref Guid pClsid); [PreserveSig] int GetUserType( [In, MarshalAs(UnmanagedType.U4)] int dwFormOfType, [Out, MarshalAs(UnmanagedType.LPWStr)] out string userType); [PreserveSig] int SetExtent( [In, MarshalAs(UnmanagedType.U4)] int dwDrawAspect, [In] NativeMethods.SIZE pSizel); [PreserveSig] int GetExtent( [In, MarshalAs(UnmanagedType.U4)] int dwDrawAspect, [Out] NativeMethods.SIZE pSizel); [PreserveSig] int Advise( [In, MarshalAs(UnmanagedType.Interface)] IAdviseSink pAdvSink, out int cookie); [PreserveSig] int Unadvise( [In, MarshalAs(UnmanagedType.U4)] int dwConnection); [PreserveSig] int EnumAdvise(out IEnumSTATDATA e); [PreserveSig] int GetMiscStatus( [In, MarshalAs(UnmanagedType.U4)] int dwAspect, out int misc); [PreserveSig] int SetColorScheme( [In] NativeMethods.tagLOGPALETTE pLogpal); [PreserveSig] int OnWindowMessage( [In, MarshalAs(UnmanagedType.U4)] int msg, [In, MarshalAs(UnmanagedType.U4)] int wParam, [In, MarshalAs(UnmanagedType.U4)] int lParam, [Out, MarshalAs(UnmanagedType.U4)] int plResult); [PreserveSig] int GetDropTarget( [Out, MarshalAs(UnmanagedType.Interface)] object ppDropTarget); }; ////// Critical - elevates via a SUC. /// [SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("B196B288-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleControl { [PreserveSig] int GetControlInfo( [Out] NativeMethods.tagCONTROLINFO pCI); [PreserveSig] int OnMnemonic( [In] ref System.Windows.Interop.MSG pMsg); [PreserveSig] int OnAmbientPropertyChange( int dispID); [PreserveSig] int FreezeEvents( int bFreeze); } ////// Critical - elevates via a SUC. /// [SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("B196B286-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IConnectionPoint { [PreserveSig] int GetConnectionInterface(out Guid iid); [PreserveSig] int GetConnectionPointContainer( [MarshalAs(UnmanagedType.Interface)] ref IConnectionPointContainer pContainer); [PreserveSig] int Advise( [In, MarshalAs(UnmanagedType.Interface)] object pUnkSink, ref int cookie); [PreserveSig] int Unadvise( int cookie); [PreserveSig] int EnumConnections(out object pEnum); } [ComImport(), Guid("00020404-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IEnumVariant { ////// Critical: This code elevates to call unmanaged code /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [PreserveSig] int Next( [In, MarshalAs(UnmanagedType.U4)] int celt, [In, Out] IntPtr rgvar, [Out, MarshalAs(UnmanagedType.LPArray)] int[] pceltFetched); void Skip( [In, MarshalAs(UnmanagedType.U4)] int celt); ////// Critical: This code elevates to call unmanaged code /// [SecurityCritical, SuppressUnmanagedCodeSecurity] void Reset(); void Clone( [Out, MarshalAs(UnmanagedType.LPArray)] UnsafeNativeMethods.IEnumVariant[] ppenum); } [ComImport(), Guid("00000104-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IEnumOLEVERB { [PreserveSig] int Next( [MarshalAs(UnmanagedType.U4)] int celt, [Out] NativeMethods.tagOLEVERB rgelt, [Out, MarshalAs(UnmanagedType.LPArray)] int[] pceltFetched); [PreserveSig] int Skip( [In, MarshalAs(UnmanagedType.U4)] int celt); void Reset(); void Clone( out IEnumOLEVERB ppenum); } // This interface has different parameter marshaling from System.Runtime.InteropServices.ComTypes.IStream. // They are incompatable. But type cast will succeed because they have the same guid. [ComImport(), Guid("0000000C-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IStream { int Read( IntPtr buf, int len); int Write( IntPtr buf, int len); [return: MarshalAs(UnmanagedType.I8)] long Seek( [In, MarshalAs(UnmanagedType.I8)] long dlibMove, int dwOrigin); void SetSize( [In, MarshalAs(UnmanagedType.I8)] long libNewSize); [return: MarshalAs(UnmanagedType.I8)] long CopyTo( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IStream pstm, [In, MarshalAs(UnmanagedType.I8)] long cb, [Out, MarshalAs(UnmanagedType.LPArray)] long[] pcbRead); void Commit( int grfCommitFlags); void Revert(); void LockRegion( [In, MarshalAs(UnmanagedType.I8)] long libOffset, [In, MarshalAs(UnmanagedType.I8)] long cb, int dwLockType); void UnlockRegion( [In, MarshalAs(UnmanagedType.I8)] long libOffset, [In, MarshalAs(UnmanagedType.I8)] long cb, int dwLockType); void Stat( [Out] NativeMethods.STATSTG pStatstg, int grfStatFlag); [return: MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IStream Clone(); } ////// Critical - elevates via a SUC. /// [SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("B196B284-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IConnectionPointContainer { [return: MarshalAs(UnmanagedType.Interface)] object EnumConnectionPoints(); [PreserveSig] int FindConnectionPoint([In] ref Guid guid, [Out, MarshalAs(UnmanagedType.Interface)]out IConnectionPoint ppCP); } [ComImport(), Guid("B196B285-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IEnumConnectionPoints { [PreserveSig] int Next(int cConnections, out IConnectionPoint pCp, out int pcFetched); [PreserveSig] int Skip(int cSkip); void Reset(); IEnumConnectionPoints Clone(); } [ComImport(), Guid("00020400-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IDispatch { int GetTypeInfoCount(); [return: MarshalAs(UnmanagedType.Interface)] ITypeInfo GetTypeInfo( [In, MarshalAs(UnmanagedType.U4)] int iTInfo, [In, MarshalAs(UnmanagedType.U4)] int lcid); ////// Critical elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] void GetIDsOfNames( [In] ref Guid riid, [In, MarshalAs(UnmanagedType.LPArray)] string[] rgszNames, [In, MarshalAs(UnmanagedType.U4)] int cNames, [In, MarshalAs(UnmanagedType.U4)] int lcid, [Out, MarshalAs(UnmanagedType.LPArray)] int[] rgDispId); ////// Critical elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] void Invoke( int dispIdMember, [In] ref Guid riid, [In, MarshalAs(UnmanagedType.U4)] int lcid, [In, MarshalAs(UnmanagedType.U4)] int dwFlags, [Out, In] NativeMethods.tagDISPPARAMS pDispParams, [Out, MarshalAs(UnmanagedType.LPArray)] object[] pVarResult, [Out, In] NativeMethods.tagEXCEPINFO pExcepInfo, [Out, MarshalAs(UnmanagedType.LPArray)] IntPtr [] pArgErr); } #region WebBrowser Related Definitions [ComImport(), Guid("D30C1661-CDAF-11d0-8A3E-00C04FC9E26E"), TypeLibType(TypeLibTypeFlags.FHidden | TypeLibTypeFlags.FDual | TypeLibTypeFlags.FOleAutomation)] public interface IWebBrowser2 { // // IWebBrowser members ////// Critical elevates via a SUC. /// [DispId(100)] [SuppressUnmanagedCodeSecurity, SecurityCritical] void GoBack(); ////// Critical elevates via a SUC. /// [DispId(101)] [SuppressUnmanagedCodeSecurity, SecurityCritical] void GoForward(); [DispId(102)] void GoHome(); [DispId(103)] void GoSearch(); [DispId(104)] void Navigate([In] string Url, [In] ref object flags, [In] ref object targetFrameName, [In] ref object postData, [In] ref object headers); ////// Critical elevates via a SUC. /// [DispId(-550)] [SuppressUnmanagedCodeSecurity, SecurityCritical] void Refresh(); ////// Critical elevates via a SUC. /// [DispId(105)] [SuppressUnmanagedCodeSecurity, SecurityCritical] void Refresh2([In] ref object level); [DispId(106)] void Stop(); [DispId(200)] object Application { [return: MarshalAs(UnmanagedType.IDispatch)]get;} [DispId(201)] object Parent { [return: MarshalAs(UnmanagedType.IDispatch)]get;} [DispId(202)] object Container { [return: MarshalAs(UnmanagedType.IDispatch)]get;} ////// Critical elevates via a SUC. /// [DispId(203)] object Document { [return: MarshalAs(UnmanagedType.IDispatch)] [SuppressUnmanagedCodeSecurity, SecurityCritical] get;} [DispId(204)] bool TopLevelContainer { get;} [DispId(205)] string Type { get;} [DispId(206)] int Left { get; set;} [DispId(207)] int Top { get; set;} [DispId(208)] int Width { get; set;} [DispId(209)] int Height { get; set;} [DispId(210)] string LocationName { get;} ////// Critical elevates via a SUC. /// [DispId(211)] string LocationURL { [SuppressUnmanagedCodeSecurity, SecurityCritical] get;} [DispId(212)] bool Busy { get;} // // IWebBrowserApp members [DispId(300)] void Quit(); [DispId(301)] void ClientToWindow([Out]out int pcx, [Out]out int pcy); [DispId(302)] void PutProperty([In] string property, [In] object vtValue); [DispId(303)] object GetProperty([In] string property); [DispId(0)] string Name { get;} [DispId(-515)] int HWND { get;} [DispId(400)] string FullName { get;} [DispId(401)] string Path { get;} [DispId(402)] bool Visible { get; set;} [DispId(403)] bool StatusBar { get; set;} [DispId(404)] string StatusText { get; set;} [DispId(405)] int ToolBar { get; set;} [DispId(406)] bool MenuBar { get; set;} [DispId(407)] bool FullScreen { get; set;} // // IWebBrowser2 members ////// Critical elevates via a SUC. /// [DispId(500)] [SuppressUnmanagedCodeSecurity, SecurityCritical ] void Navigate2([In] ref object URL, [In] ref object flags, [In] ref object targetFrameName, [In] ref object postData, [In] ref object headers); [DispId(501)] UnsafeNativeMethods.OLECMDF QueryStatusWB([In] UnsafeNativeMethods.OLECMDID cmdID); [DispId(502)] void ExecWB([In] UnsafeNativeMethods.OLECMDID cmdID, [In] UnsafeNativeMethods.OLECMDEXECOPT cmdexecopt, ref object pvaIn, IntPtr pvaOut); [DispId(503)] void ShowBrowserBar([In] ref object pvaClsid, [In] ref object pvarShow, [In] ref object pvarSize); [DispId(-525)] NativeMethods.WebBrowserReadyState ReadyState { get;} [DispId(550)] bool Offline { get; set;} [DispId(551)] bool Silent { get; set;} [DispId(552)] bool RegisterAsBrowser { get; set;} [DispId(553)] bool RegisterAsDropTarget { get; set;} [DispId(554)] bool TheaterMode { get; set;} [DispId(555)] bool AddressBar { get; set;} [DispId(556)] bool Resizable { get; set;} } [ComImport(), Guid("34A715A0-6587-11D0-924A-0020AFC7AC4D"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIDispatch), TypeLibType(TypeLibTypeFlags.FHidden)] public interface DWebBrowserEvents2 { [DispId(102)] void StatusTextChange([In] string text); [DispId(108)] void ProgressChange([In] int progress, [In] int progressMax); [DispId(105)] void CommandStateChange([In] long command, [In] bool enable); [DispId(106)] void DownloadBegin(); [DispId(104)] void DownloadComplete(); [DispId(113)] void TitleChange([In] string text); [DispId(112)] void PropertyChange([In] string szProperty); [DispId(225)] void PrintTemplateInstantiation([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp); [DispId(226)] void PrintTemplateTeardown([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp); [DispId(227)] void UpdatePageStatus([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object nPage, [In] ref object fDone); [DispId(250)] void BeforeNavigate2([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object URL, [In] ref object flags, [In] ref object targetFrameName, [In] ref object postData, [In] ref object headers, [In, Out] ref bool cancel); [DispId(251)] void NewWindow2([In, Out, MarshalAs(UnmanagedType.IDispatch)] ref object pDisp, [In, Out] ref bool cancel); [DispId(252)] void NavigateComplete2([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object URL); [DispId(259)] void DocumentComplete([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object URL); [DispId(253)] void OnQuit(); [DispId(254)] void OnVisible([In] bool visible); [DispId(255)] void OnToolBar([In] bool toolBar); [DispId(256)] void OnMenuBar([In] bool menuBar); [DispId(257)] void OnStatusBar([In] bool statusBar); [DispId(258)] void OnFullScreen([In] bool fullScreen); [DispId(260)] void OnTheaterMode([In] bool theaterMode); [DispId(262)] void WindowSetResizable([In] bool resizable); [DispId(264)] void WindowSetLeft([In] int left); [DispId(265)] void WindowSetTop([In] int top); [DispId(266)] void WindowSetWidth([In] int width); [DispId(267)] void WindowSetHeight([In] int height); [DispId(263)] void WindowClosing([In] bool isChildWindow, [In, Out] ref bool cancel); [DispId(268)] void ClientToHostWindow([In, Out] ref long cx, [In, Out] ref long cy); [DispId(269)] void SetSecureLockIcon([In] int secureLockIcon); [DispId(270)] void FileDownload([In, Out] ref bool ActiveDocument, [In, Out] ref bool cancel); [DispId(271)] void NavigateError([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object URL, [In] ref object frame, [In] ref object statusCode, [In, Out] ref bool cancel); [DispId(272)] void PrivacyImpactedStateChange([In] bool bImpacted); [DispId(282)] // IE 7+ void SetPhishingFilterStatus(uint phishingFilterStatus); [DispId(283)] // IE 7+ void WindowStateChanged(uint dwFlags, uint dwValidFlagsMask); } // Used to control the webbrowser appearance and provide DTE to script via window.external [ ComImport(), Guid("BD3F23C0-D43E-11CF-893B-00AA00BDCE1A"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] internal interface IDocHostUIHandler { [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int ShowContextMenu( [In, MarshalAs(UnmanagedType.U4)] int dwID, [In] NativeMethods.POINT pt, [In, MarshalAs(UnmanagedType.Interface)] object pcmdtReserved, [In, MarshalAs(UnmanagedType.Interface)] object pdispReserved); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int GetHostInfo( [In, Out] NativeMethods.DOCHOSTUIINFO info); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int ShowUI( [In, MarshalAs(UnmanagedType.I4)] int dwID, [In] UnsafeNativeMethods.IOleInPlaceActiveObject activeObject, [In] NativeMethods.IOleCommandTarget commandTarget, [In] UnsafeNativeMethods.IOleInPlaceFrame frame, [In] UnsafeNativeMethods.IOleInPlaceUIWindow doc); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int HideUI(); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int UpdateUI(); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int EnableModeless( [In, MarshalAs(UnmanagedType.Bool)] bool fEnable); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int OnDocWindowActivate( [In, MarshalAs(UnmanagedType.Bool)] bool fActivate); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int OnFrameWindowActivate( [In, MarshalAs(UnmanagedType.Bool)] bool fActivate); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int ResizeBorder( [In] NativeMethods.COMRECT rect, [In] UnsafeNativeMethods.IOleInPlaceUIWindow doc, bool fFrameWindow); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int TranslateAccelerator( [In] ref System.Windows.Interop.MSG msg, [In] ref Guid group, [In, MarshalAs(UnmanagedType.I4)] int nCmdID); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int GetOptionKeyPath( [Out, MarshalAs(UnmanagedType.LPArray)] String[] pbstrKey, [In, MarshalAs(UnmanagedType.U4)] int dw); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int GetDropTarget( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleDropTarget pDropTarget, [Out, MarshalAs(UnmanagedType.Interface)] out UnsafeNativeMethods.IOleDropTarget ppDropTarget); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int GetExternal( [Out, MarshalAs(UnmanagedType.IDispatch)] out object ppDispatch); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int TranslateUrl( [In, MarshalAs(UnmanagedType.U4)] int dwTranslate, [In, MarshalAs(UnmanagedType.LPWStr)] string strURLIn, [Out, MarshalAs(UnmanagedType.LPWStr)] out string pstrURLOut); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int FilterDataObject( IComDataObject pDO, out IComDataObject ppDORet); } [ComVisible(true), Guid("626FC520-A41E-11CF-A731-00A0C9082637"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsDual)] internal interface IHTMLDocument { ////// Critical elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [return: MarshalAs(UnmanagedType.IDispatch)] object GetScript(); } internal static class ArrayToVARIANTHelper { ////// Critical - Calls Marshal.OffsetOf(), which has a LinkDemand for unmanaged code. /// TreatAsSafe - This is not exploitable. /// [SecurityCritical, SecurityTreatAsSafe] static ArrayToVARIANTHelper() { VariantSize = (int)Marshal.OffsetOf(typeof(FindSizeOfVariant), "b"); } // Convert a object[] into an array of VARIANT, allocated with CoTask allocators. ////// Critical: Calls Marshal.GetNativeVariantForObject(), which has a LinkDemand for unmanaged code. /// [SecurityCritical] public unsafe static IntPtr ArrayToVARIANTVector(object[] args) { IntPtr mem = IntPtr.Zero; int i = 0; try { checked { int len = args.Length; mem = Marshal.AllocCoTaskMem(len * VariantSize); byte* a = (byte*)(void*)mem; for (i = 0; i < len; ++i) { Marshal.GetNativeVariantForObject(args[i], (IntPtr)(a + VariantSize * i)); } } } catch { if (mem != IntPtr.Zero) { FreeVARIANTVector(mem, i); } throw; } return mem; } // Free a Variant array created with the above function ////// Critical: Calls Marshal.FreeCoTaskMem(), which has a LinkDemand for unmanaged code. /// [SecurityCritical] /// The allocated memory to be freed. /// The length of the Variant vector to be cleared. public unsafe static void FreeVARIANTVector(IntPtr mem, int len) { int hr = NativeMethods.S_OK; byte* a = (byte*)(void*)mem; for (int i = 0; i < len; ++i) { int hrcurrent = NativeMethods.S_OK; checked { hrcurrent = UnsafeNativeMethods.VariantClear((IntPtr)(a + VariantSize * i)); } // save the first error and throw after we finish all VariantClear. if (NativeMethods.Succeeded(hr) && NativeMethods.Failed(hrcurrent)) { hr = hrcurrent; } } Marshal.FreeCoTaskMem(mem); if (NativeMethods.Failed(hr)) { Marshal.ThrowExceptionForHR(hr); } } [StructLayout(LayoutKind.Sequential, Pack = 1)] private struct FindSizeOfVariant { [MarshalAs(UnmanagedType.Struct)] public object var; public byte b; } private static readonly int VariantSize; } ////// Critical - This code causes unmanaged code elevation. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Oleaut32, PreserveSig=true)] private static extern int VariantClear(IntPtr pObject); [ComImport(), Guid("7FD52380-4E07-101B-AE2D-08002B2EC713"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] internal interface IPersistStreamInit { void GetClassID( [Out] out Guid pClassID); [PreserveSig] int IsDirty(); ////// Critical elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] void Load( [In, MarshalAs(UnmanagedType.Interface)] System.Runtime.InteropServices.ComTypes.IStream pstm); void Save( [In, MarshalAs(UnmanagedType.Interface)] IStream pstm, [In, MarshalAs(UnmanagedType.Bool)] bool fClearDirty); void GetSizeMax( [Out, MarshalAs(UnmanagedType.LPArray)] long pcbSize); void InitNew(); } [Flags] internal enum BrowserNavConstants : uint { OpenInNewWindow = 0x00000001, NoHistory = 0x00000002, NoReadFromCache = 0x00000004, NoWriteToCache = 0x00000008, AllowAutosearch = 0x00000010, BrowserBar = 0x00000020, Hyperlink = 0x00000040, EnforceRestricted = 0x00000080, NewWindowsManaged = 0x00000100, UntrustedForDownload = 0x00000200, TrustedForActiveX = 0x00000400, OpenInNewTab = 0x00000800, OpenInBackgroundTab = 0x00001000, KeepWordWheelText = 0x00002000 } #if never // // Used to control the webbrowser security [ComVisible(true), ComImport(), Guid("79eac9ee-baf9-11ce-8c82-00aa004ba90b"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown), CLSCompliant(false)] public interface IInternetSecurityManager { [PreserveSig] int SetSecuritySite(); [PreserveSig] int GetSecuritySite(); [PreserveSig] int MapUrlToZone(); [PreserveSig] int GetSecurityId(); [PreserveSig] int ProcessUrlAction(string url, int action, [Out, MarshalAs(UnmanagedType.LPArray, SizeParamIndex=3)] byte[] policy, int cbPolicy, ref byte context, int cbContext, int flags, int reserved); [PreserveSig] int QueryCustomPolicy(); [PreserveSig] int SetZoneMapping(); [PreserveSig] int GetZoneMappings(); } #endif #endregion WebBrowser Related Definitions ////// Critical: as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError=true, CharSet=CharSet.Auto)] public static extern uint GetRawInputDeviceList( [In, Out] NativeMethods.RAWINPUTDEVICELIST[] ridl, [In, Out] ref uint numDevices, uint sizeInBytes); ////// Critical: as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError=true, CharSet=CharSet.Auto)] public static extern uint GetRawInputDeviceInfo( IntPtr hDevice, uint command, [In] ref NativeMethods.RID_DEVICE_INFO ridInfo, ref uint sizeInBytes); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace MS.Win32 { using Accessibility; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Runtime.ConstrainedExecution; using System; using System.Security.Permissions; using System.Collections; using System.IO; using System.Text; using System.Security; using System.Diagnostics; using System.ComponentModel; using MS.Win32 ; //The SecurityHelper class differs between assemblies and could not actually be // shared, so it is duplicated across namespaces to prevent name collision. #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use a class (duplicated across multiple namespaces) from an unknown assembly. #endif using IComDataObject = System.Runtime.InteropServices.ComTypes.IDataObject; internal partial class UnsafeNativeMethods { private struct POINTSTRUCT { public int x; public int y; public POINTSTRUCT(int x, int y) { this.x = x; this.y = y; } } // For some reason "PtrToStructure" requires super high permission. ////// Critical: The code below has a link demand for unmanaged code permission.This code can be used to /// get to data that a pointer points to which can lead to easier data reading. /// [SecurityCritical] public static object PtrToStructure(IntPtr lparam, Type cls) { return Marshal.PtrToStructure(lparam, cls); } // For some reason "StructureToPtr" requires super high permission. ////// Critical: The code below has a link demand for unmanaged code permission.This code can be used to /// write data to arbitrary memory. /// [SecurityCritical] public static void StructureToPtr(object structure, IntPtr ptr, bool fDeleteOld) { Marshal.StructureToPtr(structure, ptr, fDeleteOld); } #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int OleGetClipboard(ref IComDataObject data); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int OleSetClipboard(IComDataObject pDataObj); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int OleFlushClipboard(); #endif ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Uxtheme, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern int GetCurrentThemeName(StringBuilder pszThemeFileName, int dwMaxNameChars, StringBuilder pszColorBuff, int dwMaxColorChars, StringBuilder pszSizeBuff, int cchMaxSizeChars); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.DwmAPI, BestFitMapping = false)] public static extern int DwmIsCompositionEnabled(out Int32 enabled); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern IntPtr GetCurrentThread(); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false)] public static extern int RegisterWindowMessage(string msg); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, EntryPoint = "SetWindowPos", ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true)] public static extern bool SetWindowPos(HandleRef hWnd, HandleRef hWndInsertAfter, int x, int y, int cx, int cy, int flags); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true)] public static extern IntPtr GetWindow(HandleRef hWnd, int uCmd); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, SetLastError = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false)] public static extern int GetClassName(HandleRef hwnd, StringBuilder lpClassName, int nMaxCount); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, SetLastError = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false)] public static extern int MessageBox(HandleRef hWnd, string text, string caption, int type); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Uxtheme, CharSet = CharSet.Auto, BestFitMapping = false, EntryPoint = "SetWindowTheme")] public static extern int CriticalSetWindowTheme(HandleRef hWnd, string subAppName, string subIdList); [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, EntryPoint = "CreateCompatibleBitmap", CharSet = CharSet.Auto)] public static extern IntPtr CreateCompatibleBitmap(HandleRef hDC, int width, int height); ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, EntryPoint = "CreateCompatibleBitmap", CharSet = CharSet.Auto)] public static extern IntPtr CriticalCreateCompatibleBitmap(HandleRef hDC, int width, int height); ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, EntryPoint = "GetStockObject", SetLastError = true, CharSet = CharSet.Auto)] public static extern IntPtr CriticalGetStockObject(int stockObject); ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "FillRect", SetLastError = true, CharSet = CharSet.Auto)] public static extern int CriticalFillRect(IntPtr hdc, ref NativeMethods.RECT rcFill, IntPtr brush); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern int GetBitmapBits(HandleRef hbmp, int cbBuffer, byte[] lpvBits); ////// Critical: This code escalates to unmanaged code permission /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool ShowWindow(HandleRef hWnd, int nCmdShow); ////// Critical: This code escalates to unmanaged code permission /// [SecurityCritical] public static void DeleteObject(HandleRef hObject) { HandleCollector.Remove((IntPtr)hObject, NativeMethods.CommonHandles.GDI); if (!IntDeleteObject(hObject)) { throw new Win32Exception(); } } ////// Critical: This code escalates to unmanaged code permission via a call to IntDeleteObject /// [SecurityCritical] public static bool DeleteObjectNoThrow(HandleRef hObject) { HandleCollector.Remove((IntPtr)hObject, NativeMethods.CommonHandles.GDI); bool result = IntDeleteObject(hObject); int error = Marshal.GetLastWin32Error(); if(!result) { Debug.WriteLine("DeleteObject failed. Error = " + error); } return result; } ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Gdi32, SetLastError=true, ExactSpelling = true, EntryPoint="DeleteObject", CharSet=System.Runtime.InteropServices.CharSet.Auto)] public static extern bool IntDeleteObject(HandleRef hObject); [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern IntPtr SelectObject(HandleRef hdc, IntPtr obj); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Gdi32, EntryPoint="SelectObject", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern IntPtr CriticalSelectObject(HandleRef hdc, IntPtr obj); [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false, SetLastError = true)] public static extern int GetClipboardFormatName(int format, StringBuilder lpString, int cchMax); ////// This code elevates to unmanaged code permission /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false)] public static extern int RegisterClipboardFormat(string format); [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool BitBlt(HandleRef hDC, int x, int y, int nWidth, int nHeight, HandleRef hSrcDC, int xSrc, int ySrc, int dwRop); ////// This code elevates to unmanaged code permission /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="PrintWindow", SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool CriticalPrintWindow(HandleRef hWnd, HandleRef hDC, int flags); ////// This code elevates to unmanaged code permission /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="RedrawWindow", ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool CriticalRedrawWindow(HandleRef hWnd, IntPtr lprcUpdate, IntPtr hrgnUpdate, int flags); [DllImport(ExternDll.Shell32, CharSet=CharSet.Auto, BestFitMapping = false)] public static extern int DragQueryFile(HandleRef hDrop, int iFile, StringBuilder lpszFile, int cch); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Shell32, CharSet=CharSet.Auto, BestFitMapping = false)] public static extern IntPtr ShellExecute(HandleRef hwnd, string lpOperation, string lpFile, string lpParameters, string lpDirectory, int nShowCmd); [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Unicode)] internal class ShellExecuteInfo { public int cbSize; public ShellExecuteFlags fMask; public IntPtr hwnd; public string lpVerb; public string lpFile; public string lpParameters; public string lpDirectory; public int nShow; public IntPtr hInstApp; public IntPtr lpIDList; public string lpClass; public IntPtr hkeyClass; public int dwHotKey; public IntPtr hIcon; public IntPtr hProcess; } [Flags] internal enum ShellExecuteFlags { SEE_MASK_CLASSNAME = 0x00000001, SEE_MASK_CLASSKEY = 0x00000003, SEE_MASK_NOCLOSEPROCESS = 0x00000040, SEE_MASK_FLAG_DDEWAIT = 0x00000100, SEE_MASK_DOENVSUBST = 0x00000200, SEE_MASK_FLAG_NO_UI = 0x00000400, SEE_MASK_UNICODE = 0x00004000, SEE_MASK_NO_CONSOLE = 0x00008000, SEE_MASK_ASYNCOK = 0x00100000, SEE_MASK_HMONITOR = 0x00200000, SEE_MASK_NOZONECHECKS = 0x00800000, SEE_MASK_NOQUERYCLASSSTORE = 0x01000000, SEE_MASK_WAITFORINPUTIDLE = 0x02000000 }; ////// Critical - elevates via SUC. Starts a new process. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Shell32, CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool ShellExecuteEx([In, Out] ShellExecuteInfo lpExecInfo); public const int MB_PRECOMPOSED = 0x00000001; public const int MB_COMPOSITE = 0x00000002; public const int MB_USEGLYPHCHARS = 0x00000004; public const int MB_ERR_INVALID_CHARS = 0x00000008; ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Kernel32, ExactSpelling=true, CharSet=CharSet.Unicode, SetLastError=true)] public static extern int MultiByteToWideChar(int CodePage, int dwFlags, byte[] lpMultiByteStr, int cchMultiByte, [Out, MarshalAs(UnmanagedType.LPWStr)] StringBuilder lpWideCharStr, int cchWideChar); ////// Critical - elevates (via SuppressUnmanagedCodeSecurity). /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Kernel32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Unicode)] public static extern int WideCharToMultiByte(int codePage, int flags, [MarshalAs(UnmanagedType.LPWStr)]string wideStr, int chars, [In,Out]byte[] pOutBytes, int bufferBytes, IntPtr defaultChar, IntPtr pDefaultUsed); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling=true, EntryPoint="RtlMoveMemory", CharSet=CharSet.Unicode)] public static extern void CopyMemoryW(IntPtr pdst, string psrc, int cb); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, EntryPoint = "RtlMoveMemory", CharSet = CharSet.Unicode)] public static extern void CopyMemoryW(IntPtr pdst, char[] psrc, int cb); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling=true, EntryPoint="RtlMoveMemory")] public static extern void CopyMemory(IntPtr pdst, byte[] psrc, int cb); #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation due to an unmanaged code call. Also this /// information can be used to exploit the system. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetKeyboardState", CharSet=CharSet.Auto, SetLastError=true)] private static extern int IntGetKeyboardState(byte [] keystate); [SecurityCritical] public static void GetKeyboardState(byte [] keystate) { if(IntGetKeyboardState(keystate) == 0) { throw new Win32Exception(); } } #endif #if DRT_NATIVEMETHODS [DllImport(ExternDll.User32, ExactSpelling=true, EntryPoint="keybd_event", CharSet=CharSet.Auto)] public static extern void Keybd_event(byte vk, byte scan, int flags, IntPtr extrainfo); #endif ////// Critical - This code elevates to unmanaged code. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport("oleacc.dll")] internal static extern int ObjectFromLresult(IntPtr lResult, ref Guid iid, IntPtr wParam, [In, Out] ref IAccessible ppvObject); ////// Critical - This code elevates to unmanaged code. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport("user32.dll")] internal static extern bool IsWinEventHookInstalled(int winevent); internal static Guid IID_IAccessible = new Guid(0x618736e0, 0x3c3d, 0x11cf, 0x81, 0x0c, 0x00, 0xaa, 0x00, 0x38, 0x9b, 0x71); ////// Critical: This code elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, EntryPoint = "GetModuleFileName", CharSet = CharSet.Auto, BestFitMapping = false, SetLastError = true)] private static extern int IntGetModuleFileName(HandleRef hModule, StringBuilder buffer, int length); ////// Critical: This code elevates to unmanaged code permission by calling into IntGetModuleFileName /// [SecurityCritical] internal static int GetModuleFileName(HandleRef hModule, StringBuilder buffer, int length) { int size = IntGetModuleFileName(hModule, buffer, length); if (size == 0) { throw new Win32Exception(); } return size; } #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern bool TranslateMessage([In, Out] ref System.Windows.Interop.MSG msg); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet=CharSet.Auto)] public static extern IntPtr DispatchMessage([In] ref System.Windows.Interop.MSG msg); #endif #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet=CharSet.Auto, EntryPoint="PostThreadMessage", SetLastError=true)] private static extern int IntPostThreadMessage(int id, int msg, IntPtr wparam, IntPtr lparam); [SecurityCritical] public static void PostThreadMessage(int id, int msg, IntPtr wparam, IntPtr lparam) { if(IntPostThreadMessage(id, msg, wparam, lparam) == 0) { throw new Win32Exception(); } } #endif ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [return: MarshalAs(UnmanagedType.Interface)] [DllImport(ExternDll.Ole32, ExactSpelling = true, PreserveSig = false)] [SecurityCritical, SuppressUnmanagedCodeSecurity] public static extern object CoCreateInstance( [In] ref Guid clsid, [MarshalAs(UnmanagedType.Interface)] object punkOuter, int context, [In] ref Guid iid); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, EntryPoint="OleInitialize")] private static extern int IntOleInitialize(IntPtr val); [SecurityCritical] public static int OleInitialize() { return IntOleInitialize(IntPtr.Zero); } ////// Critical: SUC. Inherently unsafe. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32)] public static extern int CoRegisterPSClsid(ref Guid riid, ref Guid rclsid); [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public extern static bool EnumThreadWindows(int dwThreadId, NativeMethods.EnumThreadWindowsCallback lpfn, HandleRef lParam); ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto, SetLastError=true)] public static extern int OleUninitialize(); [DllImport(ExternDll.Kernel32, EntryPoint="CloseHandle", CharSet=CharSet.Auto, SetLastError=true)] private static extern bool IntCloseHandle(HandleRef handle); /* public static void CloseHandle(HandleRef handle) { HandleCollector.Remove((IntPtr)handle, NativeMethods.CommonHandles.Kernel); if(!IntCloseHandle(handle)) { throw new Win32Exception(); } } */ ////// Critical: Closes a passed in handle, LinkDemand on Marshal.GetLastWin32Error /// [SecurityCritical] public static bool CloseHandleNoThrow(HandleRef handle) { HandleCollector.Remove((IntPtr)handle, NativeMethods.CommonHandles.Kernel); bool result = IntCloseHandle(handle); int error = Marshal.GetLastWin32Error(); if(!result) { Debug.WriteLine("CloseHandle failed. Error = " + error); } return result; } ////// Critical as this code performs an UnmanagedCodeSecurity elevation. /// [SecurityCritical] [DllImport(ExternDll.Ole32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int CreateStreamOnHGlobal(IntPtr hGlobal, bool fDeleteOnRelease, ref System.Runtime.InteropServices.ComTypes.IStream istream); #if BASE_NATIVEMETHODS [DllImport(ExternDll.Gdi32, SetLastError=true, EntryPoint="CreateCompatibleDC", CharSet=CharSet.Auto)] private static extern IntPtr IntCreateCompatibleDC(HandleRef hDC); ////// Critical - elevates via a SUC. Can be used to run arbitrary code. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError=true, EntryPoint="CreateCompatibleDC", CharSet=CharSet.Auto)] public static extern IntPtr CriticalCreateCompatibleDC(HandleRef hDC); ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If SupressUnmanagedCodeSecurity attribute is ever added to IntCreateCompatibleDC, we need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] public static IntPtr CreateCompatibleDC(HandleRef hDC) { IntPtr h = IntCreateCompatibleDC(hDC); if(h == IntPtr.Zero) { throw new Win32Exception(); } return HandleCollector.Add(h, NativeMethods.CommonHandles.HDC); } #endif [DllImport(ExternDll.Kernel32, EntryPoint="UnmapViewOfFile", CharSet=CharSet.Auto, SetLastError=true)] private static extern bool IntUnmapViewOfFile(HandleRef pvBaseAddress); /* ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If SupressUnmanagedCodeSecurity attribute is ever added to IntUnmapViewOfFile, we need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] public static void UnmapViewOfFile(HandleRef pvBaseAddress) { HandleCollector.Remove((IntPtr)pvBaseAddress, NativeMethods.CommonHandles.Kernel); if(IntUnmapViewOfFile(pvBaseAddress) == 0) { throw new Win32Exception(); } } */ ////// Critical: Unmaps a file handle, LinkDemand on Marshal.GetLastWin32Error /// [SecurityCritical] public static bool UnmapViewOfFileNoThrow(HandleRef pvBaseAddress) { HandleCollector.Remove((IntPtr)pvBaseAddress, NativeMethods.CommonHandles.Kernel); bool result = IntUnmapViewOfFile(pvBaseAddress); int error = Marshal.GetLastWin32Error(); if(!result) { Debug.WriteLine("UnmapViewOfFile failed. Error = " + error); } return result; } ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical] public static bool EnableWindow(HandleRef hWnd, bool enable) { UnsafeNativeMethods.SetLastError(0); bool result = IntEnableWindow(hWnd, enable); if(!result) { int win32Err = Marshal.GetLastWin32Error(); if(win32Err != 0) { throw new Win32Exception(win32Err); } } return result; } ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical] public static bool EnableWindowNoThrow(HandleRef hWnd, bool enable) { // This method is not throwing because the caller don't want to fail after calling this. // If the window was not previously disabled, the return value is zero, else it is non-zero. return IntEnableWindowNoThrow(hWnd, enable); } ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="EnableWindow", SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool IntEnableWindow(HandleRef hWnd, bool enable); ////// Critical: This code calls into unmanaged code which elevates /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="EnableWindow", CharSet=System.Runtime.InteropServices.CharSet.Auto)] public static extern bool IntEnableWindowNoThrow(HandleRef hWnd, bool enable); // GetObject stuff [DllImport(ExternDll.Gdi32, SetLastError=true, CharSet=CharSet.Auto)] public static extern int GetObject(HandleRef hObject, int nSize, [In, Out] NativeMethods.BITMAP bm); ////// Critical: This code returns the window which has focus and elevates to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr GetFocus(); ////// Critical - this code elevates via SUC. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetCursorPos", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern bool IntGetCursorPos([In, Out] NativeMethods.POINT pt); ////// Critical - calls a critical function. /// [SecurityCritical] internal static bool GetCursorPos([In, Out] NativeMethods.POINT pt) { bool returnValue = IntGetCursorPos(pt); if (returnValue == false) { throw new Win32Exception(); } return returnValue; } ////// Critical - this code elevates via SUC. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetCursorPos", ExactSpelling = true, CharSet = CharSet.Auto)] private static extern bool IntTryGetCursorPos([In, Out] NativeMethods.POINT pt); ////// Critical - calls a critical function. /// [SecurityCritical] internal static bool TryGetCursorPos([In, Out] NativeMethods.POINT pt) { bool returnValue = IntTryGetCursorPos(pt); // Sometimes Win32 will fail this call, such as if you are // not running in the interactive desktop. For example, // a secure screen saver may be running. if (returnValue == false) { System.Diagnostics.Debug.WriteLine("GetCursorPos failed!"); pt.x = 0; pt.y = 0; } return returnValue; } #if BASE_NATIVEMETHODS || CORE_NATIVEMETHODS || FRAMEWORK_NATIVEMETHODS ////// Critical:Unmanaged code that gets the state of the keyboard keys /// This can be exploited to get keyboard state. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=System.Runtime.InteropServices.CharSet.Auto)] public static extern int GetWindowThreadProcessId(HandleRef hWnd, out int lpdwProcessId); ////// Critical:Unmanaged code that gets the state of the keyboard keys /// This can be exploited to get keyboard state. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern short GetKeyState(int keyCode); [DllImport(ExternDll.Ole32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto, PreserveSig = false)] public static extern void DoDragDrop(IComDataObject dataObject, UnsafeNativeMethods.IOleDropSource dropSource, int allowedEffects, int[] finalEffect); ////// Critical - this code elevates via SUC. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=System.Runtime.InteropServices.CharSet.Auto)] public static extern bool InvalidateRect(HandleRef hWnd, IntPtr rect, bool erase); #endif ////// Critical - this code elevates via SUC. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetWindowText", CharSet=CharSet.Auto, BestFitMapping = false, SetLastError = true)] private static extern int IntGetWindowText(HandleRef hWnd, [Out] StringBuilder lpString, int nMaxCount); ////// SecurityCritical due to a call to SetLastError and calls GetWindowText /// [SecurityCritical] internal static int GetWindowText(HandleRef hWnd, [Out] StringBuilder lpString, int nMaxCount) { SetLastError(0); int returnValue = IntGetWindowText(hWnd, lpString, nMaxCount); if (returnValue == 0) { int win32Err = Marshal.GetLastWin32Error(); if (win32Err != 0) { throw new Win32Exception(win32Err); } } return returnValue; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetWindowTextLength", CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true)] private static extern int IntGetWindowTextLength(HandleRef hWnd); ////// SecurityCritical due to a call to SetLastError /// [SecurityCritical] internal static int GetWindowTextLength(HandleRef hWnd) { SetLastError(0); int returnValue = IntGetWindowTextLength(hWnd); if (returnValue == 0) { int win32Err = Marshal.GetLastWin32Error(); if (win32Err != 0) { throw new Win32Exception(win32Err); } } return returnValue; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalAlloc(int uFlags, IntPtr dwBytes); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalReAlloc(HandleRef handle, IntPtr bytes, int flags); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalLock(HandleRef handle); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern bool GlobalUnlock(HandleRef handle); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalFree(HandleRef handle); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern IntPtr GlobalSize(HandleRef handle); #if BASE_NATIVEMETHODS || CORE_NATIVEMETHODS || FRAMEWORK_NATIVEMETHODS ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet=CharSet.Auto)] public static extern bool ImmSetConversionStatus(HandleRef hIMC, int conversion, int sentence); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet=CharSet.Auto)] public static extern bool ImmGetConversionStatus(HandleRef hIMC, ref int conversion, ref int sentence); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern IntPtr ImmGetContext(HandleRef hWnd); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern bool ImmReleaseContext(HandleRef hWnd, HandleRef hIMC); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet=CharSet.Auto)] public static extern IntPtr ImmAssociateContext(HandleRef hWnd, HandleRef hIMC); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern bool ImmSetOpenStatus(HandleRef hIMC, bool open); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern bool ImmGetOpenStatus(HandleRef hIMC); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern bool ImmNotifyIME(HandleRef hIMC, int dwAction, int dwIndex, int dwValue); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet=CharSet.Auto)] public static extern int ImmGetProperty(HandleRef hkl, int flags); // ImmGetCompositionString for result and composition strings ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmGetCompositionString(HandleRef hIMC, int dwIndex, char[] lpBuf, int dwBufLen); // ImmGetCompositionString for display attributes ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmGetCompositionString(HandleRef hIMC, int dwIndex, byte[] lpBuf, int dwBufLen); // ImmGetCompositionString for clause information ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmGetCompositionString(HandleRef hIMC, int dwIndex, int[] lpBuf, int dwBufLen); // ImmGetCompositionString for query information ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmGetCompositionString(HandleRef hIMC, int dwIndex, IntPtr lpBuf, int dwBufLen); //[DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] //public static extern int ImmSetCompositionFont(HandleRef hIMC, [In, Out] ref NativeMethods.LOGFONT lf); [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmConfigureIME(HandleRef hkl, HandleRef hwnd, int dwData, IntPtr pvoid); [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmConfigureIME(HandleRef hkl, HandleRef hwnd, int dwData, [In] ref NativeMethods.REGISTERWORD registerWord); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmSetCompositionWindow(HandleRef hIMC, [In, Out] ref NativeMethods.COMPOSITIONFORM compform); ////// Critical:This code causes an elevation of privilige to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern int ImmSetCandidateWindow(HandleRef hIMC, [In, Out] ref NativeMethods.CANDIDATEFORM candform); [DllImport(ExternDll.Imm32, CharSet = CharSet.Auto)] public static extern IntPtr ImmGetDefaultIMEWnd(HandleRef hwnd); #endif ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetFocus", ExactSpelling=true, CharSet=CharSet.Auto, SetLastError=true)] private static extern IntPtr IntSetFocus(HandleRef hWnd); ////// Critical - calls IntSetFocus (the real PInvoke method) /// [SecurityCritical] internal static IntPtr SetFocus(HandleRef hWnd) { SetLastError(0); IntPtr retVal = IntSetFocus(hWnd); int errorCode = Marshal.GetLastWin32Error(); if (retVal == IntPtr.Zero) { if (errorCode != 0) { throw new Win32Exception(errorCode); } } return retVal; } ////// Critical - calls IntSetFocus (the real PInvoke method) /// [SecurityCritical] internal static bool TrySetFocus(HandleRef hWnd, ref IntPtr result) { SetLastError(0); result = IntSetFocus(hWnd); int errorCode = Marshal.GetLastWin32Error(); if (result == IntPtr.Zero) { if (errorCode != 0) { return false; } } return true; } ////// Critical: It calls methods with SuppressUnmanagedCodeSecurity attribute, Win32 call /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetParent", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern IntPtr IntGetParent(HandleRef hWnd); ////// Critical - it calls SetLastError, which is Critical. it returns an IntPtr that represents the parent of a given hwnd. /// [SecurityCritical] internal static IntPtr GetParent(HandleRef hWnd) { const int successErrorCode = 0; SetLastError(successErrorCode); IntPtr retVal = IntGetParent(hWnd); int errorCode = Marshal.GetLastWin32Error(); if (retVal == IntPtr.Zero) { if (errorCode != successErrorCode) { throw new Win32Exception(errorCode); } } return retVal; } ////// Critical - This code returns a critical resource and causes unmanaged code elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern IntPtr GetAncestor(HandleRef hWnd, int flags); ////// Critical - This code causes unmanaged code elevation. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern bool IsChild(HandleRef hWndParent, HandleRef hwnd); //***************** // // if you're thinking of enabling either of the functions below. // you should first take a look at SafeSecurityHelper.TransformGlobalRectToLocal & TransformLocalRectToScreen // they likely do what you typically use the function for - and it's safe to use. // if you use the function below - you will get exceptions in partial trust. // anyquestions - email avsee. // //****************** ////// Critical as this code performs an elevation. /// [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] [ SecurityCritical, SuppressUnmanagedCodeSecurity] public static extern IntPtr SetParent(HandleRef hWnd, HandleRef hWndParent); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, EntryPoint = "GetModuleHandle", CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true, SetLastError = true)] private static extern IntPtr IntGetModuleHandle(string modName); ////// Critical as this code performs an elevation. /// [SecurityCritical] internal static IntPtr GetModuleHandle(string modName) { IntPtr retVal = IntGetModuleHandle(modName); if (retVal == IntPtr.Zero) { throw new Win32Exception(); } return retVal; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet=CharSet.Auto)] public static extern IntPtr CallWindowProc(IntPtr wndProc, IntPtr hWnd, int msg, IntPtr wParam, IntPtr lParam); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Unicode, EntryPoint = "DefWindowProcW")] public static extern IntPtr DefWindowProc(IntPtr hWnd, Int32 Msg, IntPtr wParam, IntPtr lParam); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError=true, EntryPoint="GetProcAddress", CharSet=CharSet.Ansi, BestFitMapping=false)] public static extern IntPtr IntGetProcAddress(HandleRef hModule, string lpProcName); ////// Critical - calls IntGetProcAddress (the real PInvoke method) /// [SecurityCritical] public static IntPtr GetProcAddress(HandleRef hModule, string lpProcName) { IntPtr result = IntGetProcAddress(hModule, lpProcName); if(result == IntPtr.Zero) { throw new Win32Exception(); } return result; } // GetProcAddress Note : The lpProcName parameter can identify the DLL function by specifying an ordinal value associated // with the function in the EXPORTS statement. GetProcAddress verifies that the specified ordinal is in // the range 1 through the highest ordinal value exported in the .def file. The function then uses the // ordinal as an index to read the function's address from a function table. If the .def file does not number // the functions consecutively from 1 to N (where N is the number of exported functions), an error can // occur where GetProcAddress returns an invalid, non-NULL address, even though there is no function with the specified ordinal. ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, EntryPoint="GetProcAddress", CharSet=CharSet.Ansi, BestFitMapping=false)] public static extern IntPtr GetProcAddressNoThrow(HandleRef hModule, string lpProcName); ////// Critical: as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, CharSet = CharSet.Unicode)] public static extern IntPtr LoadLibrary(string lpFileName); #if BASE_NATIVEMETHODS || FRAMEWORK_NATIVEMETHODS || CORE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int GetSystemMetrics(int nIndex); #endif ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet=CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, ref NativeMethods.RECT rc, int nUpdate); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, ref int value, int ignore); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, ref bool value, int ignore); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, ref NativeMethods.HIGHCONTRAST_I rc, int nUpdate); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] public static extern bool SystemParametersInfo(int nAction, int nParam, [In, Out] NativeMethods.NONCLIENTMETRICS metrics, int nUpdate); ////// Critical as this code performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, CharSet = CharSet.Auto, ExactSpelling = true)] public static extern bool GetSystemPowerStatus(ref NativeMethods.SYSTEM_POWER_STATUS systemPowerStatus); ////// Critical - performs an elevation via SUC. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="ClientToScreen", SetLastError=true, ExactSpelling=true, CharSet=CharSet.Auto)] private static extern int IntClientToScreen(HandleRef hWnd, [In, Out] NativeMethods.POINT pt); ////// Critical calls critical code - IntClientToScreen /// [SecurityCritical] public static void ClientToScreen(HandleRef hWnd, [In, Out] NativeMethods.POINT pt) { if(IntClientToScreen(hWnd, pt) == 0) { throw new Win32Exception(); } } [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr GetDesktopWindow(); ////// Critical:Elevates to Unmanaged code permission and can be used to /// change the foreground window. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr GetForegroundWindow(); [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int RegisterDragDrop(HandleRef hwnd, UnsafeNativeMethods.IOleDropTarget target); [DllImport(ExternDll.Ole32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern int RevokeDragDrop(HandleRef hwnd); ////// Critical:Elevates to Unmanaged code permission and can be used to /// get information of messages in queues. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet=CharSet.Auto)] public static extern bool PeekMessage([In, Out] ref System.Windows.Interop.MSG msg, HandleRef hwnd, int msgMin, int msgMax, int remove); #if BASE_NATIVEMETHODS [DllImport(ExternDll.User32, BestFitMapping = false, CharSet=CharSet.Auto)] public static extern bool SetProp(HandleRef hWnd, string propName, HandleRef data); #endif ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "PostMessage", CharSet = CharSet.Auto, SetLastError = true)] private static extern bool IntPostMessage(HandleRef hwnd, int msg, IntPtr wparam, IntPtr lparam); ////// Critical as this code performs an elevation. /// [SecurityCritical] internal static void PostMessage(HandleRef hwnd, int msg, IntPtr wparam, IntPtr lparam) { if (!IntPostMessage(hwnd, msg, wparam, lparam)) { throw new Win32Exception(); } } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "PostMessage", CharSet = CharSet.Auto)] internal static extern bool TryPostMessage(HandleRef hwnd, int msg, IntPtr wparam, IntPtr lparam); #if BASE_NATIVEMETHODS || CORE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern void NotifyWinEvent(int winEvent, HandleRef hwnd, int objType, int objID); #endif ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, EntryPoint = "BeginPaint", CharSet = CharSet.Auto)] private static extern IntPtr IntBeginPaint(HandleRef hWnd, [In, Out] ref NativeMethods.PAINTSTRUCT lpPaint); ////// Critical as this code performs an elevation. via the call to IntBeginPaint /// [SecurityCritical] public static IntPtr BeginPaint(HandleRef hWnd, [In, Out, MarshalAs(UnmanagedType.LPStruct)] ref NativeMethods.PAINTSTRUCT lpPaint) { return HandleCollector.Add(IntBeginPaint(hWnd, ref lpPaint), NativeMethods.CommonHandles.HDC); } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, EntryPoint = "EndPaint", CharSet = CharSet.Auto)] private static extern bool IntEndPaint(HandleRef hWnd, ref NativeMethods.PAINTSTRUCT lpPaint); ////// Critical as this code performs an elevation via the call to IntEndPaint. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] public static bool EndPaint(HandleRef hWnd, [In, MarshalAs(UnmanagedType.LPStruct)] ref NativeMethods.PAINTSTRUCT lpPaint) { HandleCollector.Remove(lpPaint.hdc, NativeMethods.CommonHandles.HDC); return IntEndPaint(hWnd, ref lpPaint); } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling = true, EntryPoint = "GetDC", CharSet = CharSet.Auto)] private static extern IntPtr IntGetDC(HandleRef hWnd); ////// Critical as this code performs an elevation. The call to handle collector is /// by itself not dangerous because handle collector simply /// stores a count of the number of instances of a given /// handle and not the handle itself. /// [SecurityCritical] public static IntPtr GetDC(HandleRef hWnd) { IntPtr hDc = IntGetDC(hWnd); if(hDc == IntPtr.Zero) { throw new Win32Exception(); } return HandleCollector.Add(hDc, NativeMethods.CommonHandles.HDC); } ////// Critical as this code performs an elevation.The call to handle collector /// is by itself not dangerous because handle collector simply /// stores a count of the number of instances of a given handle and not the handle itself. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, EntryPoint = "ReleaseDC", CharSet = CharSet.Auto)] private static extern int IntReleaseDC(HandleRef hWnd, HandleRef hDC); ////// Critical as this code performs an elevation. /// [SecurityCritical] public static int ReleaseDC(HandleRef hWnd, HandleRef hDC) { HandleCollector.Remove((IntPtr)hDC, NativeMethods.CommonHandles.HDC); return IntReleaseDC(hWnd, hDC); } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int GetDeviceCaps(HandleRef hDC, int nIndex); ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr GetActiveWindow(); ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern bool SetForegroundWindow(HandleRef hWnd); // Begin API Additions to support common dialog controls ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Comdlg32, SetLastError = true, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] internal static extern int CommDlgExtendedError(); ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Comdlg32, SetLastError = true, CharSet = CharSet.Unicode)] internal static extern bool GetOpenFileName([In, Out] NativeMethods.OPENFILENAME_I ofn); ////// Critical as this code performs an elevation to unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Comdlg32, SetLastError = true, CharSet = CharSet.Unicode)] internal static extern bool GetSaveFileName([In, Out] NativeMethods.OPENFILENAME_I ofn); // End Common Dialog API Additions ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [return:MarshalAs(UnmanagedType.Bool)] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto, SetLastError=true)] public static extern bool SetLayeredWindowAttributes(HandleRef hwnd, int crKey, byte bAlpha, int dwFlags); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [return: MarshalAs(UnmanagedType.Bool)] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] public static extern bool UpdateLayeredWindow(IntPtr hwnd, IntPtr hdcDst, NativeMethods.POINT pptDst, NativeMethods.POINT pSizeDst, IntPtr hdcSrc, NativeMethods.POINT pptSrc, int crKey, ref NativeMethods.BLENDFUNCTION pBlend, int dwFlags); [DllImport(ExternDll.User32, EntryPoint = "SetActiveWindow", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern IntPtr IntSetActiveWindow(HandleRef hWnd); ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If SupressUnmanagedCodeSecurity attribute is ever added to IntSetActiveWindow, we need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] internal static IntPtr SetActiveWindow(HandleRef hWnd) { IntPtr retVal = IntSetActiveWindow(hWnd); if (retVal == IntPtr.Zero) { throw new Win32Exception(); } return retVal; } // #if PBTCOMPILER [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern IntPtr SetCursor(HandleRef hcursor); #endif ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, EntryPoint="DestroyCursor", CharSet=CharSet.Auto)] private static extern bool IntDestroyCursor(IntPtr hCurs); ////// Critical calls IntDestroyCursor /// [SecurityCritical] public static bool DestroyCursor(IntPtr hCurs) { return IntDestroyCursor(hCurs); } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="DestroyIcon", CharSet=System.Runtime.InteropServices.CharSet.Auto, SetLastError=true)] private static extern bool IntDestroyIcon(IntPtr hIcon); ////// Critical: calls a critical method (IntDestroyIcon) /// [SecurityCritical] public static bool DestroyIcon(IntPtr hIcon) { bool result = IntDestroyIcon(hIcon); int error = Marshal.GetLastWin32Error(); if(!result) { // To be consistent with out other PInvoke wrappers // we should "throw" here. But we don't want to // introduce new "throws" w/o time to follow up on any // new problems that causes. Debug.WriteLine("DestroyIcon failed. Error = " + error); //throw new Win32Exception(); } return result; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, EntryPoint="DeleteObject", CharSet=System.Runtime.InteropServices.CharSet.Auto, SetLastError=true)] private static extern bool IntDeleteObject(IntPtr hObject); ////// Critical: calls a critical method (IntDeleteObject) /// [SecurityCritical] public static bool DeleteObject(IntPtr hObject) { bool result = IntDeleteObject(hObject); int error = Marshal.GetLastWin32Error(); if(!result) { // To be consistent with out other PInvoke wrappers // we should "throw" here. But we don't want to // introduce new "throws" w/o time to follow up on any // new problems that causes. Debug.WriteLine("DeleteObject failed. Error = " + error); //throw new Win32Exception(); } return result; } ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto, EntryPoint = "CreateDIBSection")] private static extern NativeMethods.BitmapHandle PrivateCreateDIBSection(HandleRef hdc, ref NativeMethods.BITMAPINFO bitmapInfo, int iUsage, ref IntPtr ppvBits, IntPtr hSection, int dwOffset); ////// Critical - The method invokes PrivateCreateDIBSection. /// [SecurityCritical] internal static NativeMethods.BitmapHandle CreateDIBSection(HandleRef hdc, ref NativeMethods.BITMAPINFO bitmapInfo, int iUsage, ref IntPtr ppvBits, IntPtr hSection, int dwOffset) { NativeMethods.BitmapHandle hBitmap = PrivateCreateDIBSection(hdc, ref bitmapInfo, iUsage, ref ppvBits, hSection, dwOffset); int error = Marshal.GetLastWin32Error(); if ( hBitmap.IsInvalid ) { Debug.WriteLine("CreateDIBSection failed. Error = " + error); } return hBitmap; } ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto, EntryPoint = "CreateBitmap")] private static extern NativeMethods.BitmapHandle PrivateCreateBitmap(int width, int height, int planes, int bitsPerPixel, byte[] lpvBits); ////// Critical - The method invokes PrivateCreateBitmap. /// [SecurityCritical] internal static NativeMethods.BitmapHandle CreateBitmap(int width, int height, int planes, int bitsPerPixel, byte[] lpvBits) { NativeMethods.BitmapHandle hBitmap = PrivateCreateBitmap(width, height, planes, bitsPerPixel, lpvBits); int error = Marshal.GetLastWin32Error(); if ( hBitmap.IsInvalid ) { Debug.WriteLine("CreateBitmap failed. Error = " + error); } return hBitmap; } ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto, EntryPoint = "DestroyIcon")] private static extern bool PrivateDestroyIcon(HandleRef handle); ////// Critical - The method invokes PrivateDestroyIcon. /// [SecurityCritical] internal static bool DestroyIcon(HandleRef handle) { HandleCollector.Remove((IntPtr)handle, NativeMethods.CommonHandles.Icon); bool result = PrivateDestroyIcon(handle); int error = Marshal.GetLastWin32Error(); if ( !result ) { Debug.WriteLine("DestroyIcon failed. Error = " + error); } return result; } ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto, EntryPoint = "CreateIconIndirect")] private static extern NativeMethods.IconHandle PrivateCreateIconIndirect([In, MarshalAs(UnmanagedType.LPStruct)]NativeMethods.ICONINFO iconInfo); ////// Critical - The method invokes PrivateCreateIconIndirect. /// [SecurityCritical] internal static NativeMethods.IconHandle CreateIconIndirect([In, MarshalAs(UnmanagedType.LPStruct)]NativeMethods.ICONINFO iconInfo) { NativeMethods.IconHandle hIcon = PrivateCreateIconIndirect(iconInfo); int error = Marshal.GetLastWin32Error(); if ( hIcon.IsInvalid ) { Debug.WriteLine("CreateIconIndirect failed. Error = " + error); } return hIcon; } ////// Critical: This code elevates to unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling=true, CharSet=CharSet.Auto)] public static extern bool IsWindow(HandleRef hWnd); #if BASE_NATIVEMETHODS [DllImport(ExternDll.Gdi32, SetLastError=true, ExactSpelling=true, EntryPoint="DeleteDC", CharSet=CharSet.Auto)] private static extern bool IntDeleteDC(HandleRef hDC); ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If SupressUnmanagedCodeSecurity attribute is ever added to IntDeleteDC, we need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] public static void DeleteDC(HandleRef hDC) { HandleCollector.Remove((IntPtr)hDC, NativeMethods.CommonHandles.HDC); if(!IntDeleteDC(hDC)) { throw new Win32Exception(); } } ////// Critical: This code elevates to unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Gdi32, SetLastError=true, ExactSpelling=true, EntryPoint="DeleteDC", CharSet=CharSet.Auto)] private static extern bool IntCriticalDeleteDC(HandleRef hDC); ////// Critical: This code elevates to unmanaged code /// [SecurityCritical] public static void CriticalDeleteDC(HandleRef hDC) { HandleCollector.Remove((IntPtr)hDC, NativeMethods.CommonHandles.HDC); if(!IntCriticalDeleteDC(hDC)) { throw new Win32Exception(); } } #endif #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError=true, EntryPoint="GetMessageW", ExactSpelling=true, CharSet=CharSet.Unicode)] private static extern int IntGetMessageW([In, Out] ref System.Windows.Interop.MSG msg, HandleRef hWnd, int uMsgFilterMin, int uMsgFilterMax); ////// Critical - calls IntGetMessageW (the real PInvoke method) /// [SecurityCritical] public static bool GetMessageW([In, Out] ref System.Windows.Interop.MSG msg, HandleRef hWnd, int uMsgFilterMin, int uMsgFilterMax) { bool boolResult = false; int result = IntGetMessageW(ref msg, hWnd, uMsgFilterMin, uMsgFilterMax); if(result == -1) { throw new Win32Exception(); } else if(result == 0) { boolResult = false; } else { boolResult = true; } return boolResult; } #endif #if BASE_NATIVEMETHODS ////// Critical: This code elevates via a SUC to call into unmanaged Code and can get the HWND of windows at any arbitrary point on the screen /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="WindowFromPoint", ExactSpelling=true, CharSet=CharSet.Auto)] private static extern IntPtr IntWindowFromPoint(POINTSTRUCT pt); ////// Critical: This calls WindowFromPoint(POINTSTRUCT) which is marked SecurityCritical /// [SecurityCritical] public static IntPtr WindowFromPoint(int x, int y) { POINTSTRUCT ps = new POINTSTRUCT(x, y); return IntWindowFromPoint(ps); } #endif ////// Critical: This code elevates to call into unmanaged Code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="CreateWindowEx", CharSet=CharSet.Auto, BestFitMapping = false, SetLastError=true)] public static extern IntPtr IntCreateWindowEx(int dwExStyle, string lpszClassName, string lpszWindowName, int style, int x, int y, int width, int height, HandleRef hWndParent, HandleRef hMenu, HandleRef hInst, [MarshalAs(UnmanagedType.AsAny)] object pvParam); ////// Critical: This code elevates to call into unmanaged Code by calling IntCreateWindowEx /// [SecurityCritical] public static IntPtr CreateWindowEx(int dwExStyle, string lpszClassName, string lpszWindowName, int style, int x, int y, int width, int height, HandleRef hWndParent, HandleRef hMenu, HandleRef hInst, [MarshalAs(UnmanagedType.AsAny)]object pvParam) { IntPtr retVal = IntCreateWindowEx(dwExStyle, lpszClassName, lpszWindowName, style, x, y, width, height, hWndParent, hMenu, hInst, pvParam); if(retVal == IntPtr.Zero) { throw new Win32Exception(); } return retVal; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, EntryPoint="DestroyWindow", CharSet=CharSet.Auto)] public static extern bool IntDestroyWindow(HandleRef hWnd); ////// Critical - calls Security Critical method /// [SecurityCritical] public static void DestroyWindow(HandleRef hWnd) { if(!IntDestroyWindow(hWnd)) { throw new Win32Exception(); } } ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32)] internal static extern IntPtr SetWinEventHook(int eventMin, int eventMax, IntPtr hmodWinEventProc, NativeMethods.WinEventProcDef WinEventReentrancyFilter, uint idProcess, uint idThread, int dwFlags); ////// Critical - elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.User32)] internal static extern bool UnhookWinEvent(IntPtr winEventHook); // for GetUserNameEx public enum EXTENDED_NAME_FORMAT { NameUnknown = 0, NameFullyQualifiedDN = 1, NameSamCompatible = 2, NameDisplay = 3, NameUniqueId = 6, NameCanonical = 7, NameUserPrincipal = 8, NameCanonicalEx = 9, NameServicePrincipal = 10 } [ComImport(), Guid("00000122-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleDropTarget { [PreserveSig] int OleDragEnter( [In, MarshalAs(UnmanagedType.Interface)] object pDataObj, [In, MarshalAs(UnmanagedType.U4)] int grfKeyState, [In, MarshalAs(UnmanagedType.U8)] long pt, [In, Out] ref int pdwEffect); [PreserveSig] int OleDragOver( [In, MarshalAs(UnmanagedType.U4)] int grfKeyState, [In, MarshalAs(UnmanagedType.U8)] long pt, [In, Out] ref int pdwEffect); [PreserveSig] int OleDragLeave(); [PreserveSig] int OleDrop( [In, MarshalAs(UnmanagedType.Interface)] object pDataObj, [In, MarshalAs(UnmanagedType.U4)] int grfKeyState, [In, MarshalAs(UnmanagedType.U8)] long pt, [In, Out] ref int pdwEffect); } [ComImport(), Guid("00000121-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleDropSource { [PreserveSig] int OleQueryContinueDrag( int fEscapePressed, [In, MarshalAs(UnmanagedType.U4)] int grfKeyState); [PreserveSig] int OleGiveFeedback( [In, MarshalAs(UnmanagedType.U4)] int dwEffect); } [ ComImport(), Guid("B196B289-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown) ] public interface IOleControlSite { [PreserveSig] int OnControlInfoChanged(); [PreserveSig] int LockInPlaceActive(int fLock); [PreserveSig] int GetExtendedControl( [Out, MarshalAs(UnmanagedType.IDispatch)] out object ppDisp); [PreserveSig] int TransformCoords( [In, Out] NativeMethods.POINT pPtlHimetric, [In, Out] NativeMethods.POINTF pPtfContainer, [In, MarshalAs(UnmanagedType.U4)] int dwFlags); [PreserveSig] int TranslateAccelerator( [In] ref System.Windows.Interop.MSG pMsg, [In, MarshalAs(UnmanagedType.U4)] int grfModifiers); [PreserveSig] int OnFocus(int fGotFocus); [PreserveSig] int ShowPropertyFrame(); } [ComImport(), Guid("00000118-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleClientSite { [PreserveSig] int SaveObject(); [PreserveSig] int GetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwAssign, [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [Out, MarshalAs(UnmanagedType.Interface)] out object moniker); [PreserveSig] int GetContainer(out IOleContainer container); [PreserveSig] int ShowObject(); [PreserveSig] int OnShowWindow(int fShow); [PreserveSig] int RequestNewObjectLayout(); } [ComImport(), Guid("00000119-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceSite { IntPtr GetWindow(); [PreserveSig] int ContextSensitiveHelp(int fEnterMode); [PreserveSig] int CanInPlaceActivate(); [PreserveSig] int OnInPlaceActivate(); [PreserveSig] int OnUIActivate(); [PreserveSig] int GetWindowContext( [Out, MarshalAs(UnmanagedType.Interface)] out UnsafeNativeMethods.IOleInPlaceFrame ppFrame, [Out, MarshalAs(UnmanagedType.Interface)] out UnsafeNativeMethods.IOleInPlaceUIWindow ppDoc, [Out] NativeMethods.COMRECT lprcPosRect, [Out] NativeMethods.COMRECT lprcClipRect, [In, Out] NativeMethods.OLEINPLACEFRAMEINFO lpFrameInfo); [PreserveSig] int Scroll( NativeMethods.SIZE scrollExtant); [PreserveSig] int OnUIDeactivate( int fUndoable); [PreserveSig] int OnInPlaceDeactivate(); [PreserveSig] int DiscardUndoState(); [PreserveSig] int DeactivateAndUndo(); [PreserveSig] int OnPosRectChange( [In] NativeMethods.COMRECT lprcPosRect); } [ComImport(), Guid("9BFBBC02-EFF1-101A-84ED-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IPropertyNotifySink { void OnChanged(int dispID); [PreserveSig] int OnRequestEdit(int dispID); } [ComImport(), Guid("00000100-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IEnumUnknown { [PreserveSig] int Next( [In, MarshalAs(UnmanagedType.U4)] int celt, [Out] IntPtr rgelt, IntPtr pceltFetched); [PreserveSig] int Skip( [In, MarshalAs(UnmanagedType.U4)] int celt); void Reset(); void Clone( [Out] out IEnumUnknown ppenum); } [ComImport(), Guid("0000011B-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleContainer { [PreserveSig] int ParseDisplayName( [In, MarshalAs(UnmanagedType.Interface)] object pbc, [In, MarshalAs(UnmanagedType.BStr)] string pszDisplayName, [Out, MarshalAs(UnmanagedType.LPArray)] int[] pchEaten, [Out, MarshalAs(UnmanagedType.LPArray)] object[] ppmkOut); [PreserveSig] int EnumObjects( [In, MarshalAs(UnmanagedType.U4)] int grfFlags, [Out] out IEnumUnknown ppenum); [PreserveSig] int LockContainer( bool fLock); } [ComImport(), Guid("00000116-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceFrame { IntPtr GetWindow(); [PreserveSig] int ContextSensitiveHelp(int fEnterMode); [PreserveSig] int GetBorder( [Out] NativeMethods.COMRECT lprectBorder); [PreserveSig] int RequestBorderSpace( [In] NativeMethods.COMRECT pborderwidths); [PreserveSig] int SetBorderSpace( [In] NativeMethods.COMRECT pborderwidths); [PreserveSig] int SetActiveObject( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleInPlaceActiveObject pActiveObject, [In, MarshalAs(UnmanagedType.LPWStr)] string pszObjName); [PreserveSig] int InsertMenus( [In] IntPtr hmenuShared, [In, Out] NativeMethods.tagOleMenuGroupWidths lpMenuWidths); [PreserveSig] int SetMenu( [In] IntPtr hmenuShared, [In] IntPtr holemenu, [In] IntPtr hwndActiveObject); [PreserveSig] int RemoveMenus( [In] IntPtr hmenuShared); [PreserveSig] int SetStatusText( [In, MarshalAs(UnmanagedType.LPWStr)] string pszStatusText); [PreserveSig] int EnableModeless( bool fEnable); [PreserveSig] int TranslateAccelerator( [In] ref System.Windows.Interop.MSG lpmsg, [In, MarshalAs(UnmanagedType.U2)] short wID); } //IMPORTANT: Do not try to optimize perf here by changing the enum size to byte //instead of int since this is used in COM Interop for browser hosting scenarios // Enum for OLECMDIDs used by IOleCommandTarget in browser hosted scenarios // Imported from the published header - docobj.h, If you need to support more // than these OLECMDS, add it from that header file public enum OLECMDID { OLECMDID_SAVE = 3, OLECMDID_SAVEAS = 4, OLECMDID_PRINT = 6, OLECMDID_PRINTPREVIEW = 7, OLECMDID_PAGESETUP = 8, OLECMDID_PROPERTIES = 10, OLECMDID_CUT = 11, OLECMDID_COPY = 12, OLECMDID_PASTE = 13, OLECMDID_SELECTALL = 17, OLECMDID_REFRESH = 22, OLECMDID_STOP = 23, } public enum OLECMDEXECOPT { OLECMDEXECOPT_DODEFAULT = 0, OLECMDEXECOPT_PROMPTUSER = 1, OLECMDEXECOPT_DONTPROMPTUSER = 2, OLECMDEXECOPT_SHOWHELP = 3 } // OLECMDID Flags used by IOleCommandTarget to specify status of commands in browser hosted scenarios // Imported from the published header - docobj.h public enum OLECMDF { ////// The command is supported by this object /// OLECMDF_SUPPORTED = 0x1, ////// The command is available and enabled /// OLECMDF_ENABLED = 0x2, ////// The command is an on-off toggle and is currently on /// OLECMDF_LATCHED = 0x4, ////// Reserved for future use /// OLECMDF_NINCHED = 0x8, ////// Command is invisible /// OLECMDF_INVISIBLE = 0x10, ////// Command should not be displayed in the context menu /// OLECMDF_DEFHIDEONCTXTMENU = 0x20 } [ComImport(), Guid("00000115-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceUIWindow { IntPtr GetWindow(); [PreserveSig] int ContextSensitiveHelp( int fEnterMode); [PreserveSig] int GetBorder( [Out] NativeMethods.RECT lprectBorder); [PreserveSig] int RequestBorderSpace( [In] NativeMethods.RECT pborderwidths); [PreserveSig] int SetBorderSpace( [In] NativeMethods.RECT pborderwidths); void SetActiveObject( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleInPlaceActiveObject pActiveObject, [In, MarshalAs(UnmanagedType.LPWStr)] string pszObjName); } [ComImport(), Guid("00000117-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceActiveObject { ////// Critical: SUC. Exposes a native window handle. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [PreserveSig] int GetWindow(out IntPtr hwnd); void ContextSensitiveHelp( int fEnterMode); ////// Critical: This code escalates to unmanaged code permission /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [PreserveSig] int TranslateAccelerator( [In] ref System.Windows.Interop.MSG lpmsg); void OnFrameWindowActivate( int fActivate); void OnDocWindowActivate( int fActivate); void ResizeBorder( [In] NativeMethods.RECT prcBorder, [In] UnsafeNativeMethods.IOleInPlaceUIWindow pUIWindow, bool fFrameWindow); void EnableModeless( int fEnable); } [ComImport(), Guid("00000114-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleWindow { [PreserveSig] int GetWindow( [Out]out IntPtr hwnd ); void ContextSensitiveHelp( int fEnterMode); } ////// Critical - elevates via a SUC. /// [ SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("00000113-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceObject { [PreserveSig] int GetWindow( [Out]out IntPtr hwnd ); void ContextSensitiveHelp( int fEnterMode); void InPlaceDeactivate(); [PreserveSig] int UIDeactivate(); void SetObjectRects( [In] NativeMethods.COMRECT lprcPosRect, [In] NativeMethods.COMRECT lprcClipRect); void ReactivateAndUndo(); } ////// Critical - elevates via a SUC. /// [SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("00000112-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleObject { [PreserveSig] int SetClientSite( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleClientSite pClientSite); UnsafeNativeMethods.IOleClientSite GetClientSite(); [PreserveSig] int SetHostNames( [In, MarshalAs(UnmanagedType.LPWStr)] string szContainerApp, [In, MarshalAs(UnmanagedType.LPWStr)] string szContainerObj); [PreserveSig] int Close( int dwSaveOption); [PreserveSig] int SetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [In, MarshalAs(UnmanagedType.Interface)] object pmk); [PreserveSig] int GetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwAssign, [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [Out, MarshalAs(UnmanagedType.Interface)] out object moniker); [PreserveSig] int InitFromData( [In, MarshalAs(UnmanagedType.Interface)] IComDataObject pDataObject, int fCreation, [In, MarshalAs(UnmanagedType.U4)] int dwReserved); [PreserveSig] int GetClipboardData( [In, MarshalAs(UnmanagedType.U4)] int dwReserved, out IComDataObject data); [PreserveSig] int DoVerb( int iVerb, [In] IntPtr lpmsg, [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleClientSite pActiveSite, int lindex, IntPtr hwndParent, [In] NativeMethods.COMRECT lprcPosRect); [PreserveSig] int EnumVerbs(out UnsafeNativeMethods.IEnumOLEVERB e); [PreserveSig] int OleUpdate(); [PreserveSig] int IsUpToDate(); [PreserveSig] int GetUserClassID( [In, Out] ref Guid pClsid); [PreserveSig] int GetUserType( [In, MarshalAs(UnmanagedType.U4)] int dwFormOfType, [Out, MarshalAs(UnmanagedType.LPWStr)] out string userType); [PreserveSig] int SetExtent( [In, MarshalAs(UnmanagedType.U4)] int dwDrawAspect, [In] NativeMethods.SIZE pSizel); [PreserveSig] int GetExtent( [In, MarshalAs(UnmanagedType.U4)] int dwDrawAspect, [Out] NativeMethods.SIZE pSizel); [PreserveSig] int Advise( IAdviseSink pAdvSink, out int cookie); [PreserveSig] int Unadvise( [In, MarshalAs(UnmanagedType.U4)] int dwConnection); [PreserveSig] int EnumAdvise(out IEnumSTATDATA e); [PreserveSig] int GetMiscStatus( [In, MarshalAs(UnmanagedType.U4)] int dwAspect, out int misc); [PreserveSig] int SetColorScheme( [In] NativeMethods.tagLOGPALETTE pLogpal); } [ComImport(), Guid("1C2056CC-5EF4-101B-8BC8-00AA003E3B29"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleInPlaceObjectWindowless { [PreserveSig] int SetClientSite( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleClientSite pClientSite); [PreserveSig] int GetClientSite(out UnsafeNativeMethods.IOleClientSite site); [PreserveSig] int SetHostNames( [In, MarshalAs(UnmanagedType.LPWStr)] string szContainerApp, [In, MarshalAs(UnmanagedType.LPWStr)] string szContainerObj); [PreserveSig] int Close( int dwSaveOption); [PreserveSig] int SetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [In, MarshalAs(UnmanagedType.Interface)] object pmk); [PreserveSig] int GetMoniker( [In, MarshalAs(UnmanagedType.U4)] int dwAssign, [In, MarshalAs(UnmanagedType.U4)] int dwWhichMoniker, [Out, MarshalAs(UnmanagedType.Interface)] out object moniker); [PreserveSig] int InitFromData( [In, MarshalAs(UnmanagedType.Interface)] IComDataObject pDataObject, int fCreation, [In, MarshalAs(UnmanagedType.U4)] int dwReserved); [PreserveSig] int GetClipboardData( [In, MarshalAs(UnmanagedType.U4)] int dwReserved, out IComDataObject data); [PreserveSig] int DoVerb( int iVerb, [In] IntPtr lpmsg, [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleClientSite pActiveSite, int lindex, IntPtr hwndParent, [In] NativeMethods.RECT lprcPosRect); [PreserveSig] int EnumVerbs(out UnsafeNativeMethods.IEnumOLEVERB e); [PreserveSig] int OleUpdate(); [PreserveSig] int IsUpToDate(); [PreserveSig] int GetUserClassID( [In, Out] ref Guid pClsid); [PreserveSig] int GetUserType( [In, MarshalAs(UnmanagedType.U4)] int dwFormOfType, [Out, MarshalAs(UnmanagedType.LPWStr)] out string userType); [PreserveSig] int SetExtent( [In, MarshalAs(UnmanagedType.U4)] int dwDrawAspect, [In] NativeMethods.SIZE pSizel); [PreserveSig] int GetExtent( [In, MarshalAs(UnmanagedType.U4)] int dwDrawAspect, [Out] NativeMethods.SIZE pSizel); [PreserveSig] int Advise( [In, MarshalAs(UnmanagedType.Interface)] IAdviseSink pAdvSink, out int cookie); [PreserveSig] int Unadvise( [In, MarshalAs(UnmanagedType.U4)] int dwConnection); [PreserveSig] int EnumAdvise(out IEnumSTATDATA e); [PreserveSig] int GetMiscStatus( [In, MarshalAs(UnmanagedType.U4)] int dwAspect, out int misc); [PreserveSig] int SetColorScheme( [In] NativeMethods.tagLOGPALETTE pLogpal); [PreserveSig] int OnWindowMessage( [In, MarshalAs(UnmanagedType.U4)] int msg, [In, MarshalAs(UnmanagedType.U4)] int wParam, [In, MarshalAs(UnmanagedType.U4)] int lParam, [Out, MarshalAs(UnmanagedType.U4)] int plResult); [PreserveSig] int GetDropTarget( [Out, MarshalAs(UnmanagedType.Interface)] object ppDropTarget); }; ////// Critical - elevates via a SUC. /// [SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("B196B288-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IOleControl { [PreserveSig] int GetControlInfo( [Out] NativeMethods.tagCONTROLINFO pCI); [PreserveSig] int OnMnemonic( [In] ref System.Windows.Interop.MSG pMsg); [PreserveSig] int OnAmbientPropertyChange( int dispID); [PreserveSig] int FreezeEvents( int bFreeze); } ////// Critical - elevates via a SUC. /// [SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("B196B286-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IConnectionPoint { [PreserveSig] int GetConnectionInterface(out Guid iid); [PreserveSig] int GetConnectionPointContainer( [MarshalAs(UnmanagedType.Interface)] ref IConnectionPointContainer pContainer); [PreserveSig] int Advise( [In, MarshalAs(UnmanagedType.Interface)] object pUnkSink, ref int cookie); [PreserveSig] int Unadvise( int cookie); [PreserveSig] int EnumConnections(out object pEnum); } [ComImport(), Guid("00020404-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IEnumVariant { ////// Critical: This code elevates to call unmanaged code /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [PreserveSig] int Next( [In, MarshalAs(UnmanagedType.U4)] int celt, [In, Out] IntPtr rgvar, [Out, MarshalAs(UnmanagedType.LPArray)] int[] pceltFetched); void Skip( [In, MarshalAs(UnmanagedType.U4)] int celt); ////// Critical: This code elevates to call unmanaged code /// [SecurityCritical, SuppressUnmanagedCodeSecurity] void Reset(); void Clone( [Out, MarshalAs(UnmanagedType.LPArray)] UnsafeNativeMethods.IEnumVariant[] ppenum); } [ComImport(), Guid("00000104-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IEnumOLEVERB { [PreserveSig] int Next( [MarshalAs(UnmanagedType.U4)] int celt, [Out] NativeMethods.tagOLEVERB rgelt, [Out, MarshalAs(UnmanagedType.LPArray)] int[] pceltFetched); [PreserveSig] int Skip( [In, MarshalAs(UnmanagedType.U4)] int celt); void Reset(); void Clone( out IEnumOLEVERB ppenum); } // This interface has different parameter marshaling from System.Runtime.InteropServices.ComTypes.IStream. // They are incompatable. But type cast will succeed because they have the same guid. [ComImport(), Guid("0000000C-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IStream { int Read( IntPtr buf, int len); int Write( IntPtr buf, int len); [return: MarshalAs(UnmanagedType.I8)] long Seek( [In, MarshalAs(UnmanagedType.I8)] long dlibMove, int dwOrigin); void SetSize( [In, MarshalAs(UnmanagedType.I8)] long libNewSize); [return: MarshalAs(UnmanagedType.I8)] long CopyTo( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IStream pstm, [In, MarshalAs(UnmanagedType.I8)] long cb, [Out, MarshalAs(UnmanagedType.LPArray)] long[] pcbRead); void Commit( int grfCommitFlags); void Revert(); void LockRegion( [In, MarshalAs(UnmanagedType.I8)] long libOffset, [In, MarshalAs(UnmanagedType.I8)] long cb, int dwLockType); void UnlockRegion( [In, MarshalAs(UnmanagedType.I8)] long libOffset, [In, MarshalAs(UnmanagedType.I8)] long cb, int dwLockType); void Stat( [Out] NativeMethods.STATSTG pStatstg, int grfStatFlag); [return: MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IStream Clone(); } ////// Critical - elevates via a SUC. /// [SecurityCritical( SecurityCriticalScope.Everything ) , SuppressUnmanagedCodeSecurity ] [ComImport(), Guid("B196B284-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IConnectionPointContainer { [return: MarshalAs(UnmanagedType.Interface)] object EnumConnectionPoints(); [PreserveSig] int FindConnectionPoint([In] ref Guid guid, [Out, MarshalAs(UnmanagedType.Interface)]out IConnectionPoint ppCP); } [ComImport(), Guid("B196B285-BAB4-101A-B69C-00AA00341D07"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IEnumConnectionPoints { [PreserveSig] int Next(int cConnections, out IConnectionPoint pCp, out int pcFetched); [PreserveSig] int Skip(int cSkip); void Reset(); IEnumConnectionPoints Clone(); } [ComImport(), Guid("00020400-0000-0000-C000-000000000046"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] public interface IDispatch { int GetTypeInfoCount(); [return: MarshalAs(UnmanagedType.Interface)] ITypeInfo GetTypeInfo( [In, MarshalAs(UnmanagedType.U4)] int iTInfo, [In, MarshalAs(UnmanagedType.U4)] int lcid); ////// Critical elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] void GetIDsOfNames( [In] ref Guid riid, [In, MarshalAs(UnmanagedType.LPArray)] string[] rgszNames, [In, MarshalAs(UnmanagedType.U4)] int cNames, [In, MarshalAs(UnmanagedType.U4)] int lcid, [Out, MarshalAs(UnmanagedType.LPArray)] int[] rgDispId); ////// Critical elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] void Invoke( int dispIdMember, [In] ref Guid riid, [In, MarshalAs(UnmanagedType.U4)] int lcid, [In, MarshalAs(UnmanagedType.U4)] int dwFlags, [Out, In] NativeMethods.tagDISPPARAMS pDispParams, [Out, MarshalAs(UnmanagedType.LPArray)] object[] pVarResult, [Out, In] NativeMethods.tagEXCEPINFO pExcepInfo, [Out, MarshalAs(UnmanagedType.LPArray)] IntPtr [] pArgErr); } #region WebBrowser Related Definitions [ComImport(), Guid("D30C1661-CDAF-11d0-8A3E-00C04FC9E26E"), TypeLibType(TypeLibTypeFlags.FHidden | TypeLibTypeFlags.FDual | TypeLibTypeFlags.FOleAutomation)] public interface IWebBrowser2 { // // IWebBrowser members ////// Critical elevates via a SUC. /// [DispId(100)] [SuppressUnmanagedCodeSecurity, SecurityCritical] void GoBack(); ////// Critical elevates via a SUC. /// [DispId(101)] [SuppressUnmanagedCodeSecurity, SecurityCritical] void GoForward(); [DispId(102)] void GoHome(); [DispId(103)] void GoSearch(); [DispId(104)] void Navigate([In] string Url, [In] ref object flags, [In] ref object targetFrameName, [In] ref object postData, [In] ref object headers); ////// Critical elevates via a SUC. /// [DispId(-550)] [SuppressUnmanagedCodeSecurity, SecurityCritical] void Refresh(); ////// Critical elevates via a SUC. /// [DispId(105)] [SuppressUnmanagedCodeSecurity, SecurityCritical] void Refresh2([In] ref object level); [DispId(106)] void Stop(); [DispId(200)] object Application { [return: MarshalAs(UnmanagedType.IDispatch)]get;} [DispId(201)] object Parent { [return: MarshalAs(UnmanagedType.IDispatch)]get;} [DispId(202)] object Container { [return: MarshalAs(UnmanagedType.IDispatch)]get;} ////// Critical elevates via a SUC. /// [DispId(203)] object Document { [return: MarshalAs(UnmanagedType.IDispatch)] [SuppressUnmanagedCodeSecurity, SecurityCritical] get;} [DispId(204)] bool TopLevelContainer { get;} [DispId(205)] string Type { get;} [DispId(206)] int Left { get; set;} [DispId(207)] int Top { get; set;} [DispId(208)] int Width { get; set;} [DispId(209)] int Height { get; set;} [DispId(210)] string LocationName { get;} ////// Critical elevates via a SUC. /// [DispId(211)] string LocationURL { [SuppressUnmanagedCodeSecurity, SecurityCritical] get;} [DispId(212)] bool Busy { get;} // // IWebBrowserApp members [DispId(300)] void Quit(); [DispId(301)] void ClientToWindow([Out]out int pcx, [Out]out int pcy); [DispId(302)] void PutProperty([In] string property, [In] object vtValue); [DispId(303)] object GetProperty([In] string property); [DispId(0)] string Name { get;} [DispId(-515)] int HWND { get;} [DispId(400)] string FullName { get;} [DispId(401)] string Path { get;} [DispId(402)] bool Visible { get; set;} [DispId(403)] bool StatusBar { get; set;} [DispId(404)] string StatusText { get; set;} [DispId(405)] int ToolBar { get; set;} [DispId(406)] bool MenuBar { get; set;} [DispId(407)] bool FullScreen { get; set;} // // IWebBrowser2 members ////// Critical elevates via a SUC. /// [DispId(500)] [SuppressUnmanagedCodeSecurity, SecurityCritical ] void Navigate2([In] ref object URL, [In] ref object flags, [In] ref object targetFrameName, [In] ref object postData, [In] ref object headers); [DispId(501)] UnsafeNativeMethods.OLECMDF QueryStatusWB([In] UnsafeNativeMethods.OLECMDID cmdID); [DispId(502)] void ExecWB([In] UnsafeNativeMethods.OLECMDID cmdID, [In] UnsafeNativeMethods.OLECMDEXECOPT cmdexecopt, ref object pvaIn, IntPtr pvaOut); [DispId(503)] void ShowBrowserBar([In] ref object pvaClsid, [In] ref object pvarShow, [In] ref object pvarSize); [DispId(-525)] NativeMethods.WebBrowserReadyState ReadyState { get;} [DispId(550)] bool Offline { get; set;} [DispId(551)] bool Silent { get; set;} [DispId(552)] bool RegisterAsBrowser { get; set;} [DispId(553)] bool RegisterAsDropTarget { get; set;} [DispId(554)] bool TheaterMode { get; set;} [DispId(555)] bool AddressBar { get; set;} [DispId(556)] bool Resizable { get; set;} } [ComImport(), Guid("34A715A0-6587-11D0-924A-0020AFC7AC4D"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIDispatch), TypeLibType(TypeLibTypeFlags.FHidden)] public interface DWebBrowserEvents2 { [DispId(102)] void StatusTextChange([In] string text); [DispId(108)] void ProgressChange([In] int progress, [In] int progressMax); [DispId(105)] void CommandStateChange([In] long command, [In] bool enable); [DispId(106)] void DownloadBegin(); [DispId(104)] void DownloadComplete(); [DispId(113)] void TitleChange([In] string text); [DispId(112)] void PropertyChange([In] string szProperty); [DispId(225)] void PrintTemplateInstantiation([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp); [DispId(226)] void PrintTemplateTeardown([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp); [DispId(227)] void UpdatePageStatus([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object nPage, [In] ref object fDone); [DispId(250)] void BeforeNavigate2([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object URL, [In] ref object flags, [In] ref object targetFrameName, [In] ref object postData, [In] ref object headers, [In, Out] ref bool cancel); [DispId(251)] void NewWindow2([In, Out, MarshalAs(UnmanagedType.IDispatch)] ref object pDisp, [In, Out] ref bool cancel); [DispId(252)] void NavigateComplete2([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object URL); [DispId(259)] void DocumentComplete([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object URL); [DispId(253)] void OnQuit(); [DispId(254)] void OnVisible([In] bool visible); [DispId(255)] void OnToolBar([In] bool toolBar); [DispId(256)] void OnMenuBar([In] bool menuBar); [DispId(257)] void OnStatusBar([In] bool statusBar); [DispId(258)] void OnFullScreen([In] bool fullScreen); [DispId(260)] void OnTheaterMode([In] bool theaterMode); [DispId(262)] void WindowSetResizable([In] bool resizable); [DispId(264)] void WindowSetLeft([In] int left); [DispId(265)] void WindowSetTop([In] int top); [DispId(266)] void WindowSetWidth([In] int width); [DispId(267)] void WindowSetHeight([In] int height); [DispId(263)] void WindowClosing([In] bool isChildWindow, [In, Out] ref bool cancel); [DispId(268)] void ClientToHostWindow([In, Out] ref long cx, [In, Out] ref long cy); [DispId(269)] void SetSecureLockIcon([In] int secureLockIcon); [DispId(270)] void FileDownload([In, Out] ref bool ActiveDocument, [In, Out] ref bool cancel); [DispId(271)] void NavigateError([In, MarshalAs(UnmanagedType.IDispatch)] object pDisp, [In] ref object URL, [In] ref object frame, [In] ref object statusCode, [In, Out] ref bool cancel); [DispId(272)] void PrivacyImpactedStateChange([In] bool bImpacted); [DispId(282)] // IE 7+ void SetPhishingFilterStatus(uint phishingFilterStatus); [DispId(283)] // IE 7+ void WindowStateChanged(uint dwFlags, uint dwValidFlagsMask); } // Used to control the webbrowser appearance and provide DTE to script via window.external [ ComImport(), Guid("BD3F23C0-D43E-11CF-893B-00AA00BDCE1A"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] internal interface IDocHostUIHandler { [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int ShowContextMenu( [In, MarshalAs(UnmanagedType.U4)] int dwID, [In] NativeMethods.POINT pt, [In, MarshalAs(UnmanagedType.Interface)] object pcmdtReserved, [In, MarshalAs(UnmanagedType.Interface)] object pdispReserved); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int GetHostInfo( [In, Out] NativeMethods.DOCHOSTUIINFO info); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int ShowUI( [In, MarshalAs(UnmanagedType.I4)] int dwID, [In] UnsafeNativeMethods.IOleInPlaceActiveObject activeObject, [In] NativeMethods.IOleCommandTarget commandTarget, [In] UnsafeNativeMethods.IOleInPlaceFrame frame, [In] UnsafeNativeMethods.IOleInPlaceUIWindow doc); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int HideUI(); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int UpdateUI(); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int EnableModeless( [In, MarshalAs(UnmanagedType.Bool)] bool fEnable); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int OnDocWindowActivate( [In, MarshalAs(UnmanagedType.Bool)] bool fActivate); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int OnFrameWindowActivate( [In, MarshalAs(UnmanagedType.Bool)] bool fActivate); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int ResizeBorder( [In] NativeMethods.COMRECT rect, [In] UnsafeNativeMethods.IOleInPlaceUIWindow doc, bool fFrameWindow); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int TranslateAccelerator( [In] ref System.Windows.Interop.MSG msg, [In] ref Guid group, [In, MarshalAs(UnmanagedType.I4)] int nCmdID); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int GetOptionKeyPath( [Out, MarshalAs(UnmanagedType.LPArray)] String[] pbstrKey, [In, MarshalAs(UnmanagedType.U4)] int dw); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int GetDropTarget( [In, MarshalAs(UnmanagedType.Interface)] UnsafeNativeMethods.IOleDropTarget pDropTarget, [Out, MarshalAs(UnmanagedType.Interface)] out UnsafeNativeMethods.IOleDropTarget ppDropTarget); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int GetExternal( [Out, MarshalAs(UnmanagedType.IDispatch)] out object ppDispatch); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int TranslateUrl( [In, MarshalAs(UnmanagedType.U4)] int dwTranslate, [In, MarshalAs(UnmanagedType.LPWStr)] string strURLIn, [Out, MarshalAs(UnmanagedType.LPWStr)] out string pstrURLOut); [return: MarshalAs(UnmanagedType.I4)] [PreserveSig] int FilterDataObject( IComDataObject pDO, out IComDataObject ppDORet); } [ComVisible(true), Guid("626FC520-A41E-11CF-A731-00A0C9082637"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsDual)] internal interface IHTMLDocument { ////// Critical elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [return: MarshalAs(UnmanagedType.IDispatch)] object GetScript(); } internal static class ArrayToVARIANTHelper { ////// Critical - Calls Marshal.OffsetOf(), which has a LinkDemand for unmanaged code. /// TreatAsSafe - This is not exploitable. /// [SecurityCritical, SecurityTreatAsSafe] static ArrayToVARIANTHelper() { VariantSize = (int)Marshal.OffsetOf(typeof(FindSizeOfVariant), "b"); } // Convert a object[] into an array of VARIANT, allocated with CoTask allocators. ////// Critical: Calls Marshal.GetNativeVariantForObject(), which has a LinkDemand for unmanaged code. /// [SecurityCritical] public unsafe static IntPtr ArrayToVARIANTVector(object[] args) { IntPtr mem = IntPtr.Zero; int i = 0; try { checked { int len = args.Length; mem = Marshal.AllocCoTaskMem(len * VariantSize); byte* a = (byte*)(void*)mem; for (i = 0; i < len; ++i) { Marshal.GetNativeVariantForObject(args[i], (IntPtr)(a + VariantSize * i)); } } } catch { if (mem != IntPtr.Zero) { FreeVARIANTVector(mem, i); } throw; } return mem; } // Free a Variant array created with the above function ////// Critical: Calls Marshal.FreeCoTaskMem(), which has a LinkDemand for unmanaged code. /// [SecurityCritical] /// The allocated memory to be freed. /// The length of the Variant vector to be cleared. public unsafe static void FreeVARIANTVector(IntPtr mem, int len) { int hr = NativeMethods.S_OK; byte* a = (byte*)(void*)mem; for (int i = 0; i < len; ++i) { int hrcurrent = NativeMethods.S_OK; checked { hrcurrent = UnsafeNativeMethods.VariantClear((IntPtr)(a + VariantSize * i)); } // save the first error and throw after we finish all VariantClear. if (NativeMethods.Succeeded(hr) && NativeMethods.Failed(hrcurrent)) { hr = hrcurrent; } } Marshal.FreeCoTaskMem(mem); if (NativeMethods.Failed(hr)) { Marshal.ThrowExceptionForHR(hr); } } [StructLayout(LayoutKind.Sequential, Pack = 1)] private struct FindSizeOfVariant { [MarshalAs(UnmanagedType.Struct)] public object var; public byte b; } private static readonly int VariantSize; } ////// Critical - This code causes unmanaged code elevation. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport(ExternDll.Oleaut32, PreserveSig=true)] private static extern int VariantClear(IntPtr pObject); [ComImport(), Guid("7FD52380-4E07-101B-AE2D-08002B2EC713"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown)] internal interface IPersistStreamInit { void GetClassID( [Out] out Guid pClassID); [PreserveSig] int IsDirty(); ////// Critical elevates via a SUC. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] void Load( [In, MarshalAs(UnmanagedType.Interface)] System.Runtime.InteropServices.ComTypes.IStream pstm); void Save( [In, MarshalAs(UnmanagedType.Interface)] IStream pstm, [In, MarshalAs(UnmanagedType.Bool)] bool fClearDirty); void GetSizeMax( [Out, MarshalAs(UnmanagedType.LPArray)] long pcbSize); void InitNew(); } [Flags] internal enum BrowserNavConstants : uint { OpenInNewWindow = 0x00000001, NoHistory = 0x00000002, NoReadFromCache = 0x00000004, NoWriteToCache = 0x00000008, AllowAutosearch = 0x00000010, BrowserBar = 0x00000020, Hyperlink = 0x00000040, EnforceRestricted = 0x00000080, NewWindowsManaged = 0x00000100, UntrustedForDownload = 0x00000200, TrustedForActiveX = 0x00000400, OpenInNewTab = 0x00000800, OpenInBackgroundTab = 0x00001000, KeepWordWheelText = 0x00002000 } #if never // // Used to control the webbrowser security [ComVisible(true), ComImport(), Guid("79eac9ee-baf9-11ce-8c82-00aa004ba90b"), InterfaceTypeAttribute(ComInterfaceType.InterfaceIsIUnknown), CLSCompliant(false)] public interface IInternetSecurityManager { [PreserveSig] int SetSecuritySite(); [PreserveSig] int GetSecuritySite(); [PreserveSig] int MapUrlToZone(); [PreserveSig] int GetSecurityId(); [PreserveSig] int ProcessUrlAction(string url, int action, [Out, MarshalAs(UnmanagedType.LPArray, SizeParamIndex=3)] byte[] policy, int cbPolicy, ref byte context, int cbContext, int flags, int reserved); [PreserveSig] int QueryCustomPolicy(); [PreserveSig] int SetZoneMapping(); [PreserveSig] int GetZoneMappings(); } #endif #endregion WebBrowser Related Definitions ////// Critical: as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError=true, CharSet=CharSet.Auto)] public static extern uint GetRawInputDeviceList( [In, Out] NativeMethods.RAWINPUTDEVICELIST[] ridl, [In, Out] ref uint numDevices, uint sizeInBytes); ////// Critical: as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError=true, CharSet=CharSet.Auto)] public static extern uint GetRawInputDeviceInfo( IntPtr hDevice, uint command, [In] ref NativeMethods.RID_DEVICE_INFO ridInfo, ref uint sizeInBytes); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
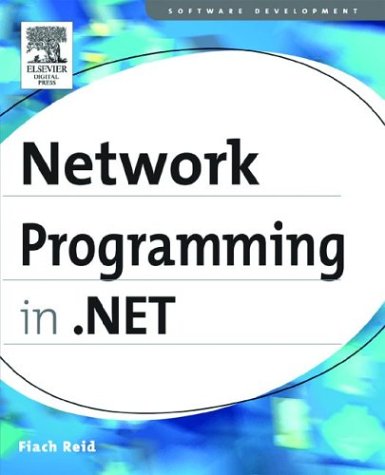
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinHexEncoding.cs
- WebBrowserProgressChangedEventHandler.cs
- ZipIOExtraFieldPaddingElement.cs
- HyperLinkField.cs
- AdPostCacheSubstitution.cs
- TreeSet.cs
- BitmapEffectDrawing.cs
- DynamicQueryableWrapper.cs
- WebBrowserUriTypeConverter.cs
- SqlConnectionFactory.cs
- ChangeBlockUndoRecord.cs
- Exceptions.cs
- QueryCoreOp.cs
- Tablet.cs
- TextOnlyOutput.cs
- RbTree.cs
- ThreadAbortException.cs
- ErrorHandlingReceiver.cs
- TrackingStringDictionary.cs
- InkSerializer.cs
- GroupItem.cs
- LinkConverter.cs
- ObjectHandle.cs
- PartBasedPackageProperties.cs
- CodeTypeParameter.cs
- TripleDES.cs
- XmlSchemaObjectCollection.cs
- View.cs
- JsonCollectionDataContract.cs
- WebChannelFactory.cs
- SqlDataSourceConfigureFilterForm.cs
- SmtpAuthenticationManager.cs
- DescendantBaseQuery.cs
- xamlnodes.cs
- HtmlElement.cs
- Environment.cs
- DeferredElementTreeState.cs
- SqlConnectionHelper.cs
- DisableDpiAwarenessAttribute.cs
- DiscoveryClientProtocol.cs
- ContentPosition.cs
- CallbackDebugBehavior.cs
- CssClassPropertyAttribute.cs
- PresentationTraceSources.cs
- InputMethodStateTypeInfo.cs
- XPathScanner.cs
- RoutedEventHandlerInfo.cs
- SynchronizationLockException.cs
- LinqDataSourceValidationException.cs
- DbUpdateCommandTree.cs
- Pair.cs
- KeyPressEvent.cs
- SynchronizationContext.cs
- TextEditorMouse.cs
- Zone.cs
- pingexception.cs
- LayoutDump.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- QilName.cs
- HybridCollection.cs
- FontSource.cs
- CommandField.cs
- DataPager.cs
- ManualWorkflowSchedulerService.cs
- BezierSegment.cs
- IpcPort.cs
- DataGridViewColumnEventArgs.cs
- DependencyObjectType.cs
- XmlAttributeCollection.cs
- EventBuilder.cs
- ResourceExpression.cs
- CrossSiteScriptingValidation.cs
- PropertiesTab.cs
- NullReferenceException.cs
- ContainerControl.cs
- OpenTypeCommon.cs
- TextAction.cs
- HtmlElementErrorEventArgs.cs
- HtmlTableRowCollection.cs
- _UncName.cs
- SourceElementsCollection.cs
- Handle.cs
- SystemFonts.cs
- SoundPlayerAction.cs
- ControlUtil.cs
- TextBlockAutomationPeer.cs
- ColorContextHelper.cs
- StickyNote.cs
- ExpressionEditorAttribute.cs
- BamlBinaryReader.cs
- AttachedPropertyMethodSelector.cs
- DockProviderWrapper.cs
- XmlSchemaComplexContent.cs
- StreamWriter.cs
- ConfigXmlAttribute.cs
- ExtentKey.cs
- EditingMode.cs
- OleDbCommand.cs
- LinkDesigner.cs
- PresentationTraceSources.cs