Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Web / WebChannelFactory.cs / 1305376 / WebChannelFactory.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Web { using System.IO; using System.Collections.Generic; using System.ServiceModel; using System.ServiceModel.Activation; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.Diagnostics.CodeAnalysis; using System.Configuration; using System.Net; using System.Globalization; public class WebChannelFactory: ChannelFactory where TChannel : class { public WebChannelFactory() : base() { } public WebChannelFactory(Binding binding) : base(binding) { } public WebChannelFactory(ServiceEndpoint endpoint) : base(endpoint) { } [SuppressMessage("Microsoft.Design", "CA1057:StringUriOverloadsCallSystemUriOverloads", Justification = "This is a configuration string and not a network location")] public WebChannelFactory(string endpointConfigurationName) : base(endpointConfigurationName) { } public WebChannelFactory(Type channelType) : base(channelType) { } public WebChannelFactory(Uri remoteAddress) : this(GetDefaultBinding(remoteAddress), remoteAddress) { } public WebChannelFactory(Binding binding, Uri remoteAddress) : base(binding, (remoteAddress != null) ? new EndpointAddress(remoteAddress) : null) { } public WebChannelFactory(string endpointConfigurationName, Uri remoteAddress) : base(endpointConfigurationName, (remoteAddress != null) ? new EndpointAddress(remoteAddress) : null) { } protected override void OnOpening() { if (this.Endpoint == null) { return; } // if the binding is missing, set up a default binding if (this.Endpoint.Binding == null && this.Endpoint.Address != null) { this.Endpoint.Binding = GetDefaultBinding(this.Endpoint.Address.Uri); } WebServiceHost.SetRawContentTypeMapperIfNecessary(this.Endpoint, false); if (this.Endpoint.Behaviors.Find () == null) { this.Endpoint.Behaviors.Add(new WebHttpBehavior()); } base.OnOpening(); } static Binding GetDefaultBinding(Uri remoteAddress) { if (remoteAddress == null || (remoteAddress.Scheme != Uri.UriSchemeHttp && remoteAddress.Scheme != Uri.UriSchemeHttps)) { return null; } if (remoteAddress.Scheme == Uri.UriSchemeHttp) { return new WebHttpBinding(); } else { WebHttpBinding result = new WebHttpBinding(); result.Security.Mode = WebHttpSecurityMode.Transport; result.Security.Transport.ClientCredentialType = HttpClientCredentialType.None; return result; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
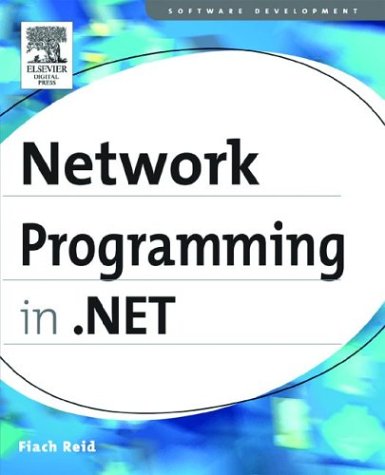
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateKey.cs
- StringKeyFrameCollection.cs
- ScriptingSectionGroup.cs
- ListViewCommandEventArgs.cs
- ListBoxAutomationPeer.cs
- FrameSecurityDescriptor.cs
- StateDesigner.LayoutSelectionGlyph.cs
- DoubleCollectionValueSerializer.cs
- DataGridViewSelectedRowCollection.cs
- _StreamFramer.cs
- AxisAngleRotation3D.cs
- AxDesigner.cs
- TextModifier.cs
- DataGridViewCellMouseEventArgs.cs
- CodeLabeledStatement.cs
- HostSecurityManager.cs
- EDesignUtil.cs
- UTF8Encoding.cs
- DocumentEventArgs.cs
- ConfigurationLocation.cs
- SqlConnectionManager.cs
- XmlIgnoreAttribute.cs
- SessionEndingEventArgs.cs
- AliasedSlot.cs
- followingsibling.cs
- LicFileLicenseProvider.cs
- NavigateUrlConverter.cs
- ExceptionWrapper.cs
- XPathDocumentIterator.cs
- DrawingImage.cs
- SettingsPropertyIsReadOnlyException.cs
- LinearGradientBrush.cs
- EventToken.cs
- TextTrailingWordEllipsis.cs
- EventListenerClientSide.cs
- SchemaElementDecl.cs
- FragmentQueryKB.cs
- TemplateBamlTreeBuilder.cs
- StatusBarItem.cs
- DataObject.cs
- MasterPageCodeDomTreeGenerator.cs
- ToolStripDropTargetManager.cs
- SqlXml.cs
- ActivityCodeDomSerializer.cs
- SvcMapFile.cs
- _SSPISessionCache.cs
- AppDomainFactory.cs
- TransportChannelFactory.cs
- StylusButton.cs
- ListParagraph.cs
- ThicknessConverter.cs
- ConnectionPointCookie.cs
- HttpHandlersSection.cs
- ListItemCollection.cs
- OdbcCommandBuilder.cs
- EnumerationRangeValidationUtil.cs
- InputMethod.cs
- TakeOrSkipWhileQueryOperator.cs
- WeakReferenceKey.cs
- SocketInformation.cs
- DBSchemaRow.cs
- TypeInitializationException.cs
- QueueProcessor.cs
- TypefaceCollection.cs
- PersonalizationEntry.cs
- AddIn.cs
- ValidatingReaderNodeData.cs
- VirtualPathUtility.cs
- XmlSerializerVersionAttribute.cs
- XPathException.cs
- EndOfStreamException.cs
- TextRangeAdaptor.cs
- mda.cs
- ResourceAssociationSetEnd.cs
- AddInController.cs
- GACMembershipCondition.cs
- httpapplicationstate.cs
- RuntimeIdentifierPropertyAttribute.cs
- WeakReferenceEnumerator.cs
- ObjectTag.cs
- SafeThemeHandle.cs
- ReturnValue.cs
- ViewGenerator.cs
- OptimalTextSource.cs
- Predicate.cs
- XsdBuilder.cs
- FixedSOMContainer.cs
- ChangePassword.cs
- CharStorage.cs
- WebSysDisplayNameAttribute.cs
- FormViewDesigner.cs
- XhtmlConformanceSection.cs
- Opcode.cs
- XmlSchemaComplexContent.cs
- TransactionChannel.cs
- TrackingServices.cs
- Propagator.cs
- EncoderParameters.cs
- WorkflowStateRollbackService.cs
- InheritanceAttribute.cs