Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / TextBoxDesigner.cs / 1 / TextBoxDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.TextBoxDesigner..ctor()")] namespace System.Windows.Forms.Design { using System; using System.Design; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms.Design.Behavior; using System.Diagnostics; ////// /// internal class TextBoxDesigner : TextBoxBaseDesigner { private DesignerActionListCollection _actionLists; public override DesignerActionListCollection ActionLists { get { if (_actionLists == null) { _actionLists = new DesignerActionListCollection(); _actionLists.Add(new TextBoxActionList(this)); } return _actionLists; } } ////// Provides a designer for TextBox. ////// Allows a designer to filter the set of properties /// the component it is designing will expose through the /// TypeDescriptor object. This method is called /// immediately before its corresponding "Post" method. /// If you are overriding this method you should call /// the base implementation before you perform your own /// filtering. /// protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); PropertyDescriptor prop; string[] shadowProps = new string[] { "PasswordChar" }; Attribute[] empty = new Attribute[0]; for (int i = 0; i < shadowProps.Length; i++) { prop = (PropertyDescriptor)properties[shadowProps[i]]; if (prop != null) { properties[shadowProps[i]] = TypeDescriptor.CreateProperty(typeof(TextBoxDesigner), prop, empty); } } } ////// Shadows the PasswordChar. UseSystemPasswordChar overrides PasswordChar so independent on the value /// of PasswordChar it will return the systemp password char. However, the value of PasswordChar is /// cached so if UseSystemPasswordChar is reset at design time the PasswordChar value can be restored. /// So in the case both properties are set, we need to serialize the real PasswordChar value as well. /// /// Note: This code was copied from MaskedTextBoxDesigner, if fixing a bug here it is probable that the /// same bug needs to be fixed there. /// private char PasswordChar { get { TextBox tb = this.Control as TextBox; Debug.Assert(tb != null, "Designed control is not a TextBox."); if (tb.UseSystemPasswordChar) { tb.UseSystemPasswordChar = false; char pwdChar = tb.PasswordChar; tb.UseSystemPasswordChar = true; return pwdChar; } else { return tb.PasswordChar; } } set { TextBox tb = this.Control as TextBox; Debug.Assert(tb != null, "Designed control is not a TextBox."); tb.PasswordChar = value; } } } internal class TextBoxActionList : DesignerActionList { public TextBoxActionList(TextBoxDesigner designer) : base(designer.Component) { } public bool Multiline { get { return ((TextBox)Component).Multiline; } set { TypeDescriptor.GetProperties(Component)["Multiline"].SetValue(Component, value); } } public override DesignerActionItemCollection GetSortedActionItems() { DesignerActionItemCollection items = new DesignerActionItemCollection(); items.Add(new DesignerActionPropertyItem("Multiline", SR.GetString(SR.MultiLineDisplayName), SR.GetString(SR.PropertiesCategoryName), SR.GetString(SR.MultiLineDescription))); return items; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
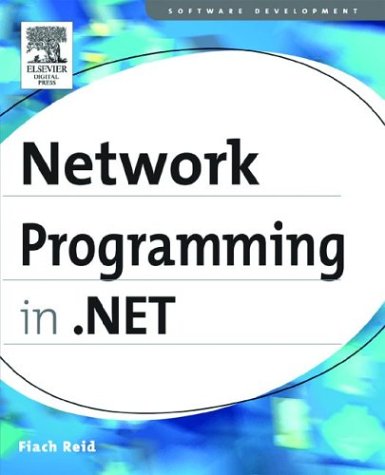
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeViewEvent.cs
- TypeGeneratedEventArgs.cs
- ImageButton.cs
- TypeElementCollection.cs
- PrintPreviewDialog.cs
- StringSorter.cs
- PropVariant.cs
- ManifestSignedXml.cs
- ExpressionBuilder.cs
- MethodBody.cs
- BasicDesignerLoader.cs
- WebRequestModuleElement.cs
- AttachedPropertyInfo.cs
- ColorPalette.cs
- PolyQuadraticBezierSegment.cs
- SerialStream.cs
- SqlPersonalizationProvider.cs
- HtmlFormAdapter.cs
- Transform3D.cs
- GroupAggregateExpr.cs
- InvalidDataException.cs
- WebPartVerbCollection.cs
- Int32CAMarshaler.cs
- Int32Converter.cs
- CompModSwitches.cs
- Vector3DCollection.cs
- Rfc4050KeyFormatter.cs
- StrongNameIdentityPermission.cs
- XmlDownloadManager.cs
- Overlapped.cs
- StagingAreaInputItem.cs
- DelegateSerializationHolder.cs
- DrawingBrush.cs
- EntitySet.cs
- ChannelManagerBase.cs
- XPathNodeIterator.cs
- Transform3DGroup.cs
- NoClickablePointException.cs
- _LazyAsyncResult.cs
- PerformanceCounterManager.cs
- TripleDESCryptoServiceProvider.cs
- DataBindingCollection.cs
- LongSumAggregationOperator.cs
- PropertyConverter.cs
- Size.cs
- InputScope.cs
- MethodAccessException.cs
- IOException.cs
- AbandonedMutexException.cs
- EarlyBoundInfo.cs
- ToolStripItemDesigner.cs
- DatasetMethodGenerator.cs
- Int32EqualityComparer.cs
- PropertyDescriptor.cs
- MaskDescriptors.cs
- OleDbConnection.cs
- RangeBaseAutomationPeer.cs
- PreProcessor.cs
- DataGridViewLayoutData.cs
- DataStreams.cs
- ProfileManager.cs
- WebPartHeaderCloseVerb.cs
- XmlConverter.cs
- GrammarBuilderWildcard.cs
- OlePropertyStructs.cs
- HitTestFilterBehavior.cs
- followingsibling.cs
- IsolatedStoragePermission.cs
- SrgsText.cs
- Msec.cs
- Viewport2DVisual3D.cs
- RequestQueue.cs
- CompositionTarget.cs
- Win32.cs
- StatusCommandUI.cs
- BaseCollection.cs
- NotSupportedException.cs
- MemoryMappedViewAccessor.cs
- RecipientInfo.cs
- ActivitySurrogateSelector.cs
- ListViewItem.cs
- BindingList.cs
- ScriptIgnoreAttribute.cs
- CqlParserHelpers.cs
- ChildTable.cs
- TextElementEnumerator.cs
- InputMethodStateTypeInfo.cs
- CachedCompositeFamily.cs
- XmlSchemaNotation.cs
- KeyedCollection.cs
- AssemblyNameProxy.cs
- Operator.cs
- SmtpNetworkElement.cs
- WizardStepBase.cs
- CodeRemoveEventStatement.cs
- ObjectResult.cs
- URIFormatException.cs
- Rfc2898DeriveBytes.cs
- TagPrefixInfo.cs
- Lasso.cs