Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / ByteStack.cs / 1305376 / ByteStack.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; namespace System.Xml { // This stack is designed to minimize object creation for the // objects being stored in the stack by allowing them to be // re-used over time. It basically pushes the objects creating // a high water mark then as Pop() is called they are not removed // so that next time Push() is called it simply returns the last // object that was already on the stack. internal class ByteStack { private byte[] stack; private int growthRate; private int top; private int size; public ByteStack(int growthRate) { this.growthRate = growthRate; top = 0; stack = new byte[growthRate]; size = growthRate; } public void Push(byte data) { if (size == top) { byte[] newstack = new byte[size + growthRate]; if (top > 0) { Buffer.BlockCopy(stack, 0, newstack, 0, top); } stack = newstack; size += growthRate; } stack[top++] = data; } public byte Pop() { if (top > 0) { return stack[--top]; } else { return 0; } } public byte Peek() { if (top > 0) { return stack[top - 1]; } else { return 0; } } public int Length { get { return top; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
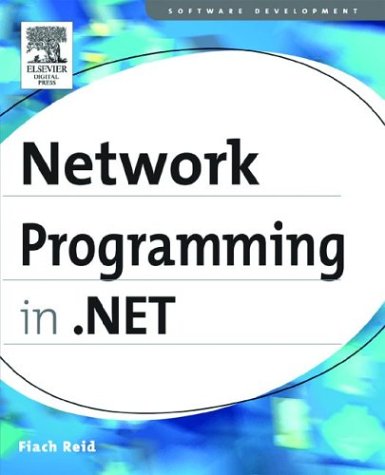
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Aggregates.cs
- EventHandlingScope.cs
- NumberFormatInfo.cs
- SafeLibraryHandle.cs
- SystemInfo.cs
- CommonObjectSecurity.cs
- DesignerLoader.cs
- TypeBuilderInstantiation.cs
- RootAction.cs
- EventLogPermissionHolder.cs
- RangeBase.cs
- SQLResource.cs
- Main.cs
- UriTemplateDispatchFormatter.cs
- ToolStripItemGlyph.cs
- FixedDSBuilder.cs
- Validator.cs
- PrinterResolution.cs
- TreePrinter.cs
- IndexingContentUnit.cs
- CodeDomConfigurationHandler.cs
- httpstaticobjectscollection.cs
- Bezier.cs
- StdValidatorsAndConverters.cs
- ClientTargetCollection.cs
- DataGridViewAutoSizeModeEventArgs.cs
- GAC.cs
- DataGridViewColumn.cs
- CompiledRegexRunnerFactory.cs
- GC.cs
- InputEventArgs.cs
- Baml6ConstructorInfo.cs
- ProfilePropertySettingsCollection.cs
- FunctionNode.cs
- _Semaphore.cs
- Ops.cs
- PngBitmapEncoder.cs
- XmlNamespaceMapping.cs
- RelatedPropertyManager.cs
- RemoteWebConfigurationHostServer.cs
- DataTableReaderListener.cs
- Figure.cs
- EntityType.cs
- DataGridViewAdvancedBorderStyle.cs
- RelationshipConstraintValidator.cs
- StaticExtension.cs
- CodeTypeMember.cs
- ToolStripDropDownMenu.cs
- EmptyStringExpandableObjectConverter.cs
- IgnoreSectionHandler.cs
- ADConnectionHelper.cs
- LocalClientSecuritySettingsElement.cs
- ManagementOperationWatcher.cs
- NoPersistScope.cs
- DataGridViewButtonColumn.cs
- WizardForm.cs
- SqlTriggerContext.cs
- BaseAddressElement.cs
- SevenBitStream.cs
- WsatExtendedInformation.cs
- FilterException.cs
- DesignerSerializerAttribute.cs
- RewritingValidator.cs
- userdatakeys.cs
- Vector3DCollectionValueSerializer.cs
- HwndSourceKeyboardInputSite.cs
- PageAsyncTaskManager.cs
- TrackBarRenderer.cs
- SqlDependencyListener.cs
- RenderDataDrawingContext.cs
- LineInfo.cs
- SqlStatistics.cs
- UriExt.cs
- TextPattern.cs
- SystemIPv4InterfaceProperties.cs
- DecoderExceptionFallback.cs
- ProcessModelSection.cs
- HtmlInputCheckBox.cs
- WebConfigurationHost.cs
- SimplePropertyEntry.cs
- MetadataSet.cs
- TypeBrowser.xaml.cs
- TreeNode.cs
- WebPartConnectionsCancelEventArgs.cs
- StorageRoot.cs
- StaticFileHandler.cs
- TaskExceptionHolder.cs
- QueueException.cs
- XmlNamedNodeMap.cs
- SafeNativeMemoryHandle.cs
- HandleCollector.cs
- HashCodeCombiner.cs
- XpsDocumentEvent.cs
- BooleanSwitch.cs
- WindowsFormsSectionHandler.cs
- ReadWriteObjectLock.cs
- FunctionImportMapping.cs
- ThousandthOfEmRealDoubles.cs
- ResourcesBuildProvider.cs
- StrokeNodeData.cs