Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ToolStripItemGlyph.cs / 2 / ToolStripItemGlyph.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Design; using Accessibility; using System.Runtime.Serialization.Formatters; using System.Threading; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using Microsoft.Win32; using System.Windows.Forms.Design.Behavior; using System.Reflection; ////// The glyph we put over the items. Basically this sets the hit-testable area of the item itself. /// internal class ToolStripItemGlyph : ControlBodyGlyph{ private ToolStripItem _item; private Rectangle _bounds; private ToolStripItemDesigner _itemDesigner; public ToolStripItemGlyph(ToolStripItem item, ToolStripItemDesigner itemDesigner, Rectangle bounds, System.Windows.Forms.Design.Behavior.Behavior b) : base(bounds, Cursors.Default, item, b) { _item = item; _bounds = bounds; _itemDesigner = itemDesigner; } public ToolStripItem Item { get { return _item; } } public override Rectangle Bounds { get { return _bounds; } } public ToolStripItemDesigner ItemDesigner { get { return _itemDesigner; } } ////// /// Abstract method that forces Glyph implementations to provide /// hit test logic. Given any point - if the Glyph has decided to /// be involved with that location, the Glyph will need to return /// a valid Cursor. Otherwise, returning null will cause the /// the BehaviorService to simply ignore it. /// public override Cursor GetHitTest(Point p) { if (_item.Visible && _bounds.Contains(p)) { return Cursors.Default; } return null; } ////// /// Control host dont draw on Invalidation... /// public override void Paint(PaintEventArgs pe) { if (_item is ToolStripControlHost && _item.IsOnDropDown ) { if( _item is System.Windows.Forms.ToolStripComboBox && VisualStyles.VisualStyleRenderer.IsSupported) { // When processing WM_PAINT and the OS has a theme enabled, the native ComboBox sends a WM_PAINT // message to its parent when a theme is enabled in the OS forcing a repaint in the AdornerWindow // generating an infinite WM_PAINT message processing loop. We guard against this here. See DDB#99105. return; } _item.Invalidate(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
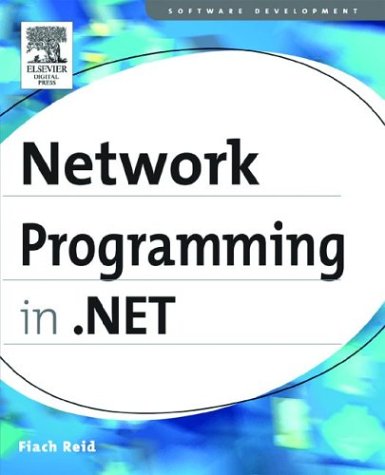
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssemblyBuilder.cs
- TrackingQueryElement.cs
- CssStyleCollection.cs
- TypeGeneratedEventArgs.cs
- InternalUserCancelledException.cs
- DecimalConstantAttribute.cs
- ProcessHostFactoryHelper.cs
- UnitySerializationHolder.cs
- XmlBoundElement.cs
- OrderByBuilder.cs
- SimpleExpression.cs
- XamlTypeMapper.cs
- WizardPanel.cs
- contentDescriptor.cs
- HttpModuleAction.cs
- ConnectionString.cs
- ExpressionVisitor.cs
- httpstaticobjectscollection.cs
- FullTextBreakpoint.cs
- SecurityTokenAuthenticator.cs
- TextSelection.cs
- CodeDomConfigurationHandler.cs
- QuaternionRotation3D.cs
- DetailsViewRow.cs
- DataGridViewCheckBoxColumn.cs
- ListItemCollection.cs
- ProfileSettingsCollection.cs
- CharStorage.cs
- WriteFileContext.cs
- WinCategoryAttribute.cs
- UseAttributeSetsAction.cs
- PixelShader.cs
- VirtualDirectoryMapping.cs
- CaseInsensitiveComparer.cs
- ExpressionPrefixAttribute.cs
- Part.cs
- AdornerHitTestResult.cs
- PreloadedPackages.cs
- DataFormat.cs
- RoutedEvent.cs
- PackageRelationshipCollection.cs
- PointLightBase.cs
- EventProviderTraceListener.cs
- ListBox.cs
- ClientProxyGenerator.cs
- NotCondition.cs
- TokenBasedSetEnumerator.cs
- ChangeDirector.cs
- PtsContext.cs
- FloatUtil.cs
- SmiEventStream.cs
- FlatButtonAppearance.cs
- ICspAsymmetricAlgorithm.cs
- HostExecutionContextManager.cs
- SourceExpressionException.cs
- TextServicesHost.cs
- SoapSchemaImporter.cs
- LocalizableResourceBuilder.cs
- BackgroundFormatInfo.cs
- RtfToXamlLexer.cs
- UInt16Converter.cs
- TableCellCollection.cs
- DetailsViewInsertEventArgs.cs
- XsltOutput.cs
- _PooledStream.cs
- IsolationInterop.cs
- SrgsOneOf.cs
- PageCatalogPartDesigner.cs
- MethodToken.cs
- ReceiveContext.cs
- ImmComposition.cs
- LogicalTreeHelper.cs
- PersistenceTask.cs
- ImpersonateTokenRef.cs
- TypeDelegator.cs
- Latin1Encoding.cs
- WsdlInspector.cs
- XmlSchemaRedefine.cs
- MiniModule.cs
- StrongNameIdentityPermission.cs
- StatusStrip.cs
- UTF8Encoding.cs
- LocalizedNameDescriptionPair.cs
- SimpleWorkerRequest.cs
- BigInt.cs
- DynamicDocumentPaginator.cs
- DesigntimeLicenseContext.cs
- BindingManagerDataErrorEventArgs.cs
- Vector3D.cs
- isolationinterop.cs
- WebResourceUtil.cs
- EventListenerClientSide.cs
- Processor.cs
- RequestDescription.cs
- StylusSystemGestureEventArgs.cs
- TextViewSelectionProcessor.cs
- UriSectionReader.cs
- ParserExtension.cs
- SQLConvert.cs
- CellIdBoolean.cs