Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Compiler / CodeGeneration / ActivityCodeGenerator.cs / 1305376 / ActivityCodeGenerator.cs
namespace System.Workflow.ComponentModel.Compiler { using System; using System.CodeDom; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Serialization; #region Class ActivityCodeGenerator public class ActivityCodeGenerator { public virtual void GenerateCode(CodeGenerationManager manager, object obj) { if (manager == null) throw new ArgumentNullException("manager"); if (obj == null) throw new ArgumentNullException("obj"); Activity activity = obj as Activity; if (activity == null) throw new ArgumentException(SR.GetString(SR.Error_UnexpectedArgumentType, typeof(Activity).FullName), "obj"); manager.Context.Push(activity); // Generate code for all the member Binds. Walker walker = new Walker(); walker.FoundProperty += delegate(Walker w, WalkerEventArgs args) { // ActivityBind bindBase = args.CurrentValue as ActivityBind; if (bindBase != null) { // push if (args.CurrentProperty != null) manager.Context.Push(args.CurrentProperty); manager.Context.Push(args.CurrentPropertyOwner); // call generate code foreach (ActivityCodeGenerator codeGenerator in manager.GetCodeGenerators(bindBase.GetType())) codeGenerator.GenerateCode(manager, args.CurrentValue); // pops manager.Context.Pop(); if (args.CurrentProperty != null) manager.Context.Pop(); } }; walker.WalkProperties(activity, obj); manager.Context.Pop(); } protected CodeTypeDeclaration GetCodeTypeDeclaration(CodeGenerationManager manager, string fullClassName) { if (manager == null) throw new ArgumentNullException("manager"); if (fullClassName == null) throw new ArgumentNullException("fullClassName"); string namespaceName; string className; Helpers.GetNamespaceAndClassName(fullClassName, out namespaceName, out className); CodeNamespaceCollection codeNamespaces = manager.Context[typeof(CodeNamespaceCollection)] as CodeNamespaceCollection; if (codeNamespaces == null) throw new InvalidOperationException(SR.GetString(SR.Error_ContextStackItemMissing, typeof(CodeNamespaceCollection).Name)); CodeNamespace codeNS = null; return Helpers.GetCodeNamespaceAndClass(codeNamespaces, namespaceName, className, out codeNS); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
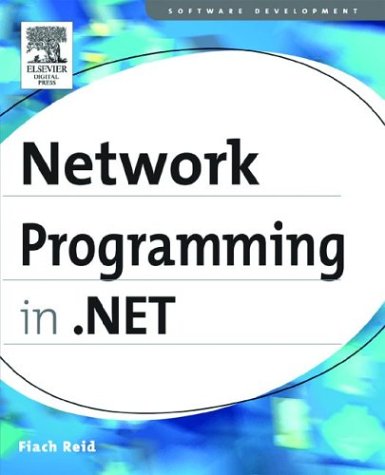
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LassoHelper.cs
- CreateUserWizard.cs
- DatagridviewDisplayedBandsData.cs
- KeyFrames.cs
- TargetInvocationException.cs
- InputLanguage.cs
- ContentValidator.cs
- TextRangeEditLists.cs
- AdCreatedEventArgs.cs
- EntityPropertyMappingAttribute.cs
- BrowserCapabilitiesFactoryBase.cs
- Vector3dCollection.cs
- QueryableDataSourceView.cs
- KeyEventArgs.cs
- WorkflowControlEndpoint.cs
- AssemblyContextControlItem.cs
- ThreadPool.cs
- DataSourceCache.cs
- Partitioner.cs
- CqlBlock.cs
- DrawingGroup.cs
- XNameTypeConverter.cs
- UrlRoutingHandler.cs
- TableLayout.cs
- TypeInfo.cs
- Errors.cs
- HtmlForm.cs
- ComboBoxRenderer.cs
- TemplatedMailWebEventProvider.cs
- PaperSource.cs
- MessagePropertyVariants.cs
- Int32AnimationUsingKeyFrames.cs
- TimeSpanSecondsConverter.cs
- UdpAnnouncementEndpoint.cs
- KeysConverter.cs
- UdpMessageProperty.cs
- IPHostEntry.cs
- WebHttpBehavior.cs
- TextEvent.cs
- DetailsViewDeletedEventArgs.cs
- ObjectContext.cs
- ClientSponsor.cs
- DelegatingConfigHost.cs
- TypeConverterValueSerializer.cs
- EntityStoreSchemaGenerator.cs
- ErrorHandlingReceiver.cs
- XmlNodeChangedEventManager.cs
- EntityDataSourceView.cs
- TextRangeProviderWrapper.cs
- FilterUserControlBase.cs
- ConstNode.cs
- LogicalTreeHelper.cs
- ImmutableAssemblyCacheEntry.cs
- CaseExpr.cs
- _NestedMultipleAsyncResult.cs
- PageThemeCodeDomTreeGenerator.cs
- TableCell.cs
- DataGridViewUtilities.cs
- DataBoundControl.cs
- Scanner.cs
- NetworkInformationException.cs
- LocalValueEnumerator.cs
- PrimitiveSchema.cs
- MouseGesture.cs
- ToolStripGripRenderEventArgs.cs
- EmbeddedMailObjectsCollection.cs
- CurrentChangingEventArgs.cs
- GlobalProxySelection.cs
- AutomationAttributeInfo.cs
- Profiler.cs
- SByteConverter.cs
- ParserHooks.cs
- DecimalMinMaxAggregationOperator.cs
- SqlServices.cs
- ObjectDataSourceMethodEventArgs.cs
- AccessControlList.cs
- OleDbRowUpdatingEvent.cs
- XPathArrayIterator.cs
- RequestCachePolicy.cs
- SafeNativeMethods.cs
- EmptyStringExpandableObjectConverter.cs
- CompositeActivityCodeGenerator.cs
- WhitespaceRuleLookup.cs
- HitTestParameters.cs
- HtmlWindow.cs
- Page.cs
- InfiniteIntConverter.cs
- StateRuntime.cs
- ItemTypeToolStripMenuItem.cs
- ResXBuildProvider.cs
- TextWriter.cs
- PopOutPanel.cs
- AnnotationService.cs
- StorageAssociationTypeMapping.cs
- CustomError.cs
- ParseHttpDate.cs
- ColumnResult.cs
- DetailsViewAutoFormat.cs
- _NegotiateClient.cs
- DifferencingCollection.cs