Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / InternalDuplexChannelListener.cs / 1 / InternalDuplexChannelListener.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.Runtime.Serialization; using System.ServiceModel.Diagnostics; sealed class InternalDuplexChannelListener : DelegatingChannelListener{ IChannelFactory innerChannelFactory; bool providesCorrelation; internal InternalDuplexChannelListener(InternalDuplexBindingElement bindingElement, BindingContext context) : base(context.Binding, context.Clone().BuildInnerChannelListener ()) { this.innerChannelFactory = context.BuildInnerChannelFactory (); this.providesCorrelation = bindingElement.ProvidesCorrelation; } IOutputChannel GetOutputChannel(Uri to, TimeoutHelper timeoutHelper) { IOutputChannel channel = this.innerChannelFactory.CreateChannel(new EndpointAddress(to)); channel.Open(timeoutHelper.RemainingTime()); return channel; } protected override void OnAbort() { try { this.innerChannelFactory.Abort(); } finally { base.OnAbort(); } } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedCloseAsyncResult(timeout, callback, state, base.OnBeginClose, base.OnEndClose, this.innerChannelFactory); } protected override void OnEndClose(IAsyncResult result) { ChainedCloseAsyncResult.End(result); } protected override void OnClose(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnClose(timeoutHelper.RemainingTime()); this.innerChannelFactory.Close(timeoutHelper.RemainingTime()); } protected override void OnOpening() { base.OnOpening(); this.Acceptor = (IChannelAcceptor )(object)new CompositeDuplexChannelAcceptor(this, (IChannelListener )this.InnerChannelListener); } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedOpenAsyncResult(timeout, callback, state, base.OnBeginOpen, base.OnEndOpen, this.innerChannelFactory); } protected override void OnEndOpen(IAsyncResult result) { ChainedOpenAsyncResult.End(result); } protected override void OnOpen(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnOpen(timeoutHelper.RemainingTime()); this.innerChannelFactory.Open(timeoutHelper.RemainingTime()); } public override T GetProperty () { if (typeof(T) == typeof(IChannelFactory)) { return (T)(object)innerChannelFactory; } if (typeof(T) == typeof(ISecurityCapabilities) && !this.providesCorrelation) { return InternalDuplexBindingElement.GetSecurityCapabilities (base.GetProperty ()); } T baseProperty = base.GetProperty (); if (baseProperty != null) { return baseProperty; } return this.innerChannelFactory.GetProperty (); } sealed class CompositeDuplexChannelAcceptor : LayeredChannelAcceptor { public CompositeDuplexChannelAcceptor(InternalDuplexChannelListener listener, IChannelListener innerListener) : base(listener, innerListener) { } protected override IDuplexChannel OnAcceptChannel(IInputChannel innerChannel) { return new ServerCompositeDuplexChannel((InternalDuplexChannelListener)ChannelManager, innerChannel); } } sealed class ServerCompositeDuplexChannel : ChannelBase, IDuplexChannel { IInputChannel innerInputChannel; TimeSpan sendTimeout; public ServerCompositeDuplexChannel(InternalDuplexChannelListener listener, IInputChannel innerInputChannel) : base(listener) { this.innerInputChannel = innerInputChannel; this.sendTimeout = listener.DefaultSendTimeout; } InternalDuplexChannelListener Listener { get { return (InternalDuplexChannelListener)base.Manager; } } public EndpointAddress LocalAddress { get { return this.innerInputChannel.LocalAddress; } } public EndpointAddress RemoteAddress { get { return null; } } public Uri Via { get { return null; } } public Message Receive() { return this.Receive(this.DefaultReceiveTimeout); } public Message Receive(TimeSpan timeout) { return InputChannel.HelpReceive(this, timeout); } public IAsyncResult BeginReceive(AsyncCallback callback, object state) { return this.BeginReceive(this.DefaultReceiveTimeout, callback, state); } public IAsyncResult BeginReceive(TimeSpan timeout, AsyncCallback callback, object state) { return InputChannel.HelpBeginReceive(this, timeout, callback, state); } public Message EndReceive(IAsyncResult result) { return InputChannel.HelpEndReceive(result); } public IAsyncResult BeginTryReceive(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerInputChannel.BeginTryReceive(timeout, callback, state); } public IAsyncResult BeginSend(Message message, AsyncCallback callback, object state) { return this.BeginSend(message, this.DefaultSendTimeout, callback, state); } public IAsyncResult BeginSend(Message message, TimeSpan timeout, AsyncCallback callback, object state) { return new SendAsyncResult(this, message, timeout, callback, state); } public bool EndTryReceive(IAsyncResult result, out Message message) { return this.innerInputChannel.EndTryReceive(result, out message); } public void EndSend(IAsyncResult result) { SendAsyncResult.End(result); } protected override void OnAbort() { this.innerInputChannel.Abort(); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerInputChannel.BeginClose(timeout, callback, state); } protected override void OnEndClose(IAsyncResult result) { this.innerInputChannel.EndClose(result); } protected override void OnClose(TimeSpan timeout) { if (this.innerInputChannel.State == CommunicationState.Opened) this.innerInputChannel.Close(timeout); } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerInputChannel.BeginOpen(callback, state); } protected override void OnEndOpen(IAsyncResult result) { this.innerInputChannel.EndOpen(result); } protected override void OnOpen(TimeSpan timeout) { this.innerInputChannel.Open(timeout); } public bool TryReceive(TimeSpan timeout, out Message message) { return this.innerInputChannel.TryReceive(timeout, out message); } public void Send(Message message) { this.Send(message, this.DefaultSendTimeout); } public void Send(Message message, TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); IOutputChannel outputChannel = ValidateStateAndGetOutputChannel(message, timeoutHelper); try { outputChannel.Send(message, timeoutHelper.RemainingTime()); outputChannel.Close(timeoutHelper.RemainingTime()); } finally { outputChannel.Abort(); } } IOutputChannel ValidateStateAndGetOutputChannel(Message message, TimeoutHelper timeoutHelper) { ThrowIfDisposedOrNotOpen(); if (message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("message"); } Uri to = message.Properties.Via; if (to == null) { to = message.Headers.To; if (to == null) { throw TraceUtility.ThrowHelperError(new CommunicationException(SR.GetString(SR.MessageMustHaveViaOrToSetForSendingOnServerSideCompositeDuplexChannels)), message); } //Check for EndpointAddress.AnonymousUri is just redundant else if (to.Equals(EndpointAddress.AnonymousUri) || to.Equals(message.Version.Addressing.AnonymousUri)) { throw TraceUtility.ThrowHelperError(new CommunicationException(SR.GetString(SR.MessageToCannotBeAddressedToAnonymousOnServerSideCompositeDuplexChannels, to)), message); } } //Check for EndpointAddress.AnonymousUri is just redundant else if (to.Equals(EndpointAddress.AnonymousUri) || to.Equals(message.Version.Addressing.AnonymousUri)) { throw TraceUtility.ThrowHelperError(new CommunicationException(SR.GetString(SR.MessageViaCannotBeAddressedToAnonymousOnServerSideCompositeDuplexChannels, to)), message); } return this.Listener.GetOutputChannel(to, timeoutHelper); } class SendAsyncResult : AsyncResult { IOutputChannel outputChannel; static AsyncCallback sendCompleteCallback = DiagnosticUtility.ThunkAsyncCallback(new AsyncCallback(SendCompleteCallback)); TimeoutHelper timeoutHelper; public SendAsyncResult(ServerCompositeDuplexChannel outer, Message message, TimeSpan timeout, AsyncCallback callback, object state) : base(callback, state) { this.timeoutHelper = new TimeoutHelper(timeout); this.outputChannel = outer.ValidateStateAndGetOutputChannel(message, timeoutHelper); bool success = false; try { IAsyncResult result = outputChannel.BeginSend(message, timeoutHelper.RemainingTime(), sendCompleteCallback, this); if (result.CompletedSynchronously) { CompleteSend(result); this.Complete(true); } success = true; } finally { if (!success) this.outputChannel.Abort(); } } void CompleteSend(IAsyncResult result) { try { outputChannel.EndSend(result); outputChannel.Close(); } finally { outputChannel.Abort(); } } internal static void End(IAsyncResult result) { AsyncResult.End (result); } static void SendCompleteCallback(IAsyncResult result) { if (result.CompletedSynchronously) { return; } SendAsyncResult thisPtr = (SendAsyncResult)result.AsyncState; Exception completionException = null; try { thisPtr.CompleteSend(result); } #pragma warning suppress 56500 // [....], transferring exception to another thread catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } completionException = e; } thisPtr.Complete(false, completionException); } } public bool WaitForMessage(TimeSpan timeout) { return innerInputChannel.WaitForMessage(timeout); } public IAsyncResult BeginWaitForMessage(TimeSpan timeout, AsyncCallback callback, object state) { return innerInputChannel.BeginWaitForMessage(timeout, callback, state); } public bool EndWaitForMessage(IAsyncResult result) { return innerInputChannel.EndWaitForMessage(result); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
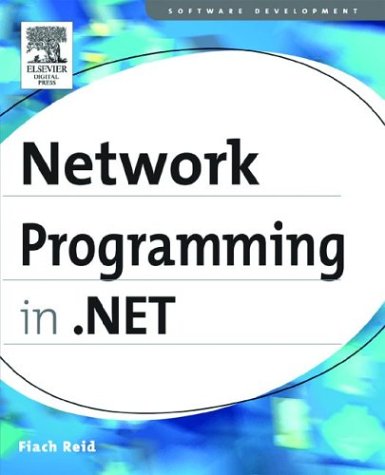
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataSourceDesigner.cs
- BCryptHashAlgorithm.cs
- DefaultEventAttribute.cs
- XmlIterators.cs
- BitmapFrame.cs
- CellQuery.cs
- ListenerElementsCollection.cs
- StructuralType.cs
- RuntimeConfigLKG.cs
- Trace.cs
- HttpDigestClientCredential.cs
- SoapAttributeAttribute.cs
- BStrWrapper.cs
- PackWebRequestFactory.cs
- _BufferOffsetSize.cs
- UIPermission.cs
- PropertyPathWorker.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- ProcessModuleCollection.cs
- BoundColumn.cs
- WebControlAdapter.cs
- COM2FontConverter.cs
- ObjectView.cs
- VBIdentifierTrimConverter.cs
- COM2IPerPropertyBrowsingHandler.cs
- BitmapMetadataEnumerator.cs
- XmlSchemaIdentityConstraint.cs
- AmbientProperties.cs
- MSG.cs
- UserControl.cs
- TextEndOfLine.cs
- ListViewDataItem.cs
- InvalidProgramException.cs
- XmlLinkedNode.cs
- BooleanFunctions.cs
- BaseParser.cs
- RadioButtonFlatAdapter.cs
- GridViewEditEventArgs.cs
- RepeaterItemCollection.cs
- StreamAsIStream.cs
- XmlSchemaInclude.cs
- EtwTrace.cs
- ByteArrayHelperWithString.cs
- FixedStringLookup.cs
- ToolStripSeparator.cs
- IntSecurity.cs
- Table.cs
- IxmlLineInfo.cs
- DataBinding.cs
- SafeNativeMethods.cs
- SolidColorBrush.cs
- ProfileGroupSettings.cs
- PrintPreviewControl.cs
- BaseDataList.cs
- FontUnit.cs
- ToggleButtonAutomationPeer.cs
- Schema.cs
- PartialClassGenerationTask.cs
- CommandBindingCollection.cs
- Label.cs
- CalendarDay.cs
- DbgCompiler.cs
- BitmapMetadataBlob.cs
- ExpressionEvaluator.cs
- HttpAsyncResult.cs
- MappingItemCollection.cs
- ScriptRef.cs
- WebPart.cs
- SerializableAttribute.cs
- IconBitmapDecoder.cs
- SQLConvert.cs
- AutoCompleteStringCollection.cs
- Behavior.cs
- ScrollViewer.cs
- ChannelTracker.cs
- DocumentPageView.cs
- COM2ColorConverter.cs
- Scene3D.cs
- DynamicPropertyHolder.cs
- DependentList.cs
- BehaviorService.cs
- HttpStreamFormatter.cs
- Int64AnimationBase.cs
- StagingAreaInputItem.cs
- WebPartAddingEventArgs.cs
- ForeignKeyFactory.cs
- CrossAppDomainChannel.cs
- DetailsViewDeleteEventArgs.cs
- SchemaImporterExtension.cs
- CodeParameterDeclarationExpression.cs
- Array.cs
- CompilerGlobalScopeAttribute.cs
- DecimalAnimationBase.cs
- EndpointIdentityExtension.cs
- ClientRolePrincipal.cs
- PersonalizationAdministration.cs
- Rect3DValueSerializer.cs
- FixedTextContainer.cs
- Rect3DConverter.cs
- AutomationPeer.cs