Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / SpnEndpointIdentity.cs / 1 / SpnEndpointIdentity.cs
//---------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel { using System; using System.ServiceModel.Dispatcher; using System.Diagnostics; using System.DirectoryServices; using System.Runtime.InteropServices; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.Security.Cryptography; using System.Security.Principal; using System.ServiceModel.Diagnostics; using System.ServiceModel.Security; using System.Xml; using System.Xml.Serialization; public class SpnEndpointIdentity : EndpointIdentity { static TimeSpan spnLookupTime = TimeSpan.FromMinutes(1); SecurityIdentifier spnSid; bool hasSpnSidBeenComputed; Object thisLock = new Object(); static Object typeLock = new Object(); static DirectoryEntry directoryEntry; public static TimeSpan SpnLookupTime { get { return spnLookupTime; } set { if (value.Ticks < 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", value.Ticks, SR.GetString(SR.ValueMustBeNonNegative))); } spnLookupTime = value; } } public SpnEndpointIdentity(string spnName) { if (spnName == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("spnName"); base.Initialize(Claim.CreateSpnClaim(spnName)); } public SpnEndpointIdentity(Claim identity) { if (identity == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("identity"); // PreSharp Bug: Parameter 'identity.ResourceType' to this public method must be validated: A null-dereference can occur here. #pragma warning suppress 56506 // Claim.ClaimType will never return null if (!identity.ClaimType.Equals(ClaimTypes.Spn)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.UnrecognizedClaimTypeForIdentity, identity.ClaimType, ClaimTypes.Spn)); base.Initialize(identity); } internal override void WriteContentsTo(XmlDictionaryWriter writer) { if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); writer.WriteElementString(XD.AddressingDictionary.Spn, XD.AddressingDictionary.IdentityExtensionNamespace, (string)this.IdentityClaim.Resource); } internal SecurityIdentifier GetSpnSid() { DiagnosticUtility.DebugAssert(ClaimTypes.Spn.Equals(this.IdentityClaim.ClaimType) || ClaimTypes.Dns.Equals(this.IdentityClaim.ClaimType), ""); if (!hasSpnSidBeenComputed) { lock (thisLock) { if (!hasSpnSidBeenComputed) { string spn = null; try { if (ClaimTypes.Dns.Equals(this.IdentityClaim.ClaimType)) { spn = "host/" + (string)this.IdentityClaim.Resource; } else { spn = (string)this.IdentityClaim.Resource; } // canonicalize SPN for use in LDAP filter following RFC 1960: if (spn != null) { spn = spn.Replace("*",@"\*").Replace("(",@"\(").Replace(")",@"\)"); } DirectoryEntry de = GetDirectoryEntry(); using (DirectorySearcher searcher = new DirectorySearcher(de)) { searcher.CacheResults = true; searcher.ClientTimeout = SpnLookupTime; searcher.Filter = "(&(objectCategory=Computer)(objectClass=computer)(servicePrincipalName=" + spn + "))"; searcher.PropertiesToLoad.Add("objectSid"); SearchResult result = searcher.FindOne(); if (result != null) { byte[] sidBinaryForm = (byte[])result.Properties["objectSid"][0]; this.spnSid = new SecurityIdentifier(sidBinaryForm, 0); } else { SecurityTraceRecordHelper.TraceSpnToSidMappingFailure(spn, null); } } } #pragma warning suppress 56500 // covered by FxCOP catch (Exception e) { // Always immediately rethrow fatal exceptions. if (DiagnosticUtility.IsFatal(e)) throw; if (e is NullReferenceException || e is SEHException) throw; SecurityTraceRecordHelper.TraceSpnToSidMappingFailure(spn, e); } finally { hasSpnSidBeenComputed = true; } } } } return this.spnSid; } static DirectoryEntry GetDirectoryEntry() { if (directoryEntry == null) { lock (typeLock) { if (directoryEntry == null) { DirectoryEntry tmp = new DirectoryEntry(@"LDAP://" + SecurityUtils.GetPrimaryDomain()); tmp.RefreshCache(new string[] { "name" }); System.Threading.Thread.MemoryBarrier(); directoryEntry = tmp; } } } return directoryEntry; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
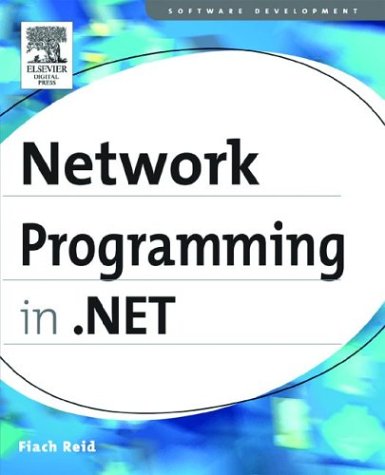
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BoundField.cs
- EventLogPermissionAttribute.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- StrongNameMembershipCondition.cs
- PropertyInformationCollection.cs
- EventMap.cs
- TimeoutConverter.cs
- Encoding.cs
- Model3DGroup.cs
- ToolStripRendererSwitcher.cs
- MaskedTextBoxTextEditorDropDown.cs
- CommandSet.cs
- CollectionChange.cs
- ReturnType.cs
- SpeechEvent.cs
- InvalidCastException.cs
- Compiler.cs
- ScrollEventArgs.cs
- DataListGeneralPage.cs
- PostBackTrigger.cs
- ScopelessEnumAttribute.cs
- BindingOperations.cs
- InternalSafeNativeMethods.cs
- TlsSspiNegotiation.cs
- DropShadowBitmapEffect.cs
- DataServiceException.cs
- NameScopePropertyAttribute.cs
- EmbeddedMailObject.cs
- ContextMenuService.cs
- BitmapCacheBrush.cs
- XmlDocumentFragment.cs
- AudienceUriMode.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- RealProxy.cs
- ElementsClipboardData.cs
- COAUTHINFO.cs
- DataSourceView.cs
- CacheHelper.cs
- DataGridViewRowPostPaintEventArgs.cs
- NetNamedPipeBindingElement.cs
- ActivationServices.cs
- Point3DKeyFrameCollection.cs
- URIFormatException.cs
- CharEnumerator.cs
- XmlQuerySequence.cs
- TabControlCancelEvent.cs
- ZipIOCentralDirectoryFileHeader.cs
- LinqDataSourceContextData.cs
- StringWriter.cs
- DayRenderEvent.cs
- ViewService.cs
- DesignerToolStripControlHost.cs
- DocumentPageTextView.cs
- SchemaImporterExtensionsSection.cs
- MultiSelectRootGridEntry.cs
- webeventbuffer.cs
- DataKeyCollection.cs
- ParenthesizePropertyNameAttribute.cs
- WhitespaceRuleLookup.cs
- PolicyStatement.cs
- SqlConnectionManager.cs
- IndexOutOfRangeException.cs
- NativeRecognizer.cs
- ToolBarButtonClickEvent.cs
- ConsumerConnectionPoint.cs
- SqlDataSourceView.cs
- CollectionBase.cs
- URI.cs
- BitmapFrameEncode.cs
- RightsManagementEncryptionTransform.cs
- MaskDesignerDialog.cs
- TrackingServices.cs
- Vector.cs
- Timer.cs
- HttpRawResponse.cs
- PopupControlService.cs
- Trace.cs
- Timeline.cs
- IPGlobalProperties.cs
- SqlDataRecord.cs
- EditBehavior.cs
- WrappedReader.cs
- Hash.cs
- Parser.cs
- IfAction.cs
- WeakReadOnlyCollection.cs
- ReachSerializableProperties.cs
- HtmlTableCell.cs
- SqlGatherProducedAliases.cs
- InkPresenterAutomationPeer.cs
- OleDbSchemaGuid.cs
- SkinIDTypeConverter.cs
- PageCache.cs
- SupportingTokenProviderSpecification.cs
- ExpressionVisitor.cs
- StorageComplexTypeMapping.cs
- GridViewRowCollection.cs
- Pts.cs
- __ConsoleStream.cs
- CustomError.cs