Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / CustomDictionarySources.cs / 1305600 / CustomDictionarySources.cs
//---------------------------------------------------------------------------- // // File: CustomDictionarySources.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Collection of Uri objects used to specify location of custom dictionaries. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Text; using System.Windows.Markup; using System.Windows.Navigation; using System.Windows.Controls.Primitives; using System.Windows.Documents; namespace System.Windows.Controls { ////// Collection of ///objects representing local file system or UNC paths. /// Methods that modify the collection also call into Speller callbakcs to notify about the change, so Speller /// could load/unload appropriate dicitonary files. /// /// Implements IList interface with 1 restriction - does not allow double addition of same item to /// the collection. /// For all methods (except Inser) that modify the collection behavior is to ignore add/set request, /// but still notify the Speller. /// In case of Insert an exception is thrown if the item already exists in the collection. /// /// There is a restriction on kinds of supported Uri objects, which is enforced in internal class CustomDictionarySources : IListmethod. /// Refer to this method for supported types of URIs. /// , IList { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region constructors internal CustomDictionarySources(TextBoxBase owner) { _owner = owner; _uriList = new List (); } #endregion constructors //------------------------------------------------------ // // Interface implementations // //----------------------------------------------------- #region Interface implementations #region IEnumerable Members public IEnumerator GetEnumerator() { return _uriList.GetEnumerator(); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return _uriList.GetEnumerator(); } #endregion #endregion Interface implementations #region IList Members int IList .IndexOf(Uri item) { return _uriList.IndexOf(item); } /// /// Implementation of Insert method. A restriction added by this implementation is that /// it will throw exception if the item was already added previously. /// This is to avoid ambiguity in respect to the expected position (index) of the inserted item. /// Caller will have to check first if item was already added. /// /// /// void IList.Insert(int index, Uri item) { if (_uriList.Contains(item)) { throw new ArgumentException(SR.Get(SRID.CustomDictionaryItemAlreadyExists), "item"); } ValidateUri(item); _uriList.Insert(index, item); if (Speller != null) { Speller.OnDictionaryUriAdded(item); } } void IList .RemoveAt(int index) { Uri uri = _uriList[index]; _uriList.RemoveAt(index); if (Speller != null) { Speller.OnDictionaryUriRemoved(uri); } } /// /// Sets value at specified index. /// Speller is notified that value at the index is being replaced, which means /// current value at given offset is removed, and new value is added at the same index. /// /// ///Uri IList .this[int index] { get { return _uriList[index]; } set { ValidateUri(value); Uri oldUri = _uriList[index]; if (Speller != null) { Speller.OnDictionaryUriRemoved(oldUri); } _uriList[index] = value; if (Speller != null) { Speller.OnDictionaryUriAdded(value); } } } #endregion #region ICollection Members /// /// Adds new item to the internal collection. /// Duplicate items ARE NOT added, but Speller is still notified. /// /// void ICollection.Add(Uri item) { ValidateUri(item); if (!_uriList.Contains(item)) { _uriList.Add(item); } if (Speller != null) { Speller.OnDictionaryUriAdded(item); } } void ICollection .Clear() { _uriList.Clear(); if (Speller != null) { Speller.OnDictionaryUriCollectionCleared(); } } bool ICollection .Contains(Uri item) { return _uriList.Contains(item); } void ICollection .CopyTo(Uri[] array, int arrayIndex) { _uriList.CopyTo(array, arrayIndex); } int ICollection .Count { get { return _uriList.Count; } } bool ICollection .IsReadOnly { get { return ((ICollection )_uriList).IsReadOnly; } } bool ICollection .Remove(Uri item) { bool removed = _uriList.Remove(item); if (removed && (Speller != null)) { Speller.OnDictionaryUriRemoved(item); } return removed; } #endregion #region IList Members /// /// See generic IList'Uri implementation notes. /// /// ///int IList.Add(object value) { ((IList )this).Add((Uri)value); return _uriList.IndexOf((Uri)value); } void IList.Clear() { ((IList )this).Clear(); } bool IList.Contains(object value) { return ((IList)_uriList).Contains(value); } int IList.IndexOf(object value) { return ((IList)_uriList).IndexOf(value); } void IList.Insert(int index, object value) { ((IList )this).Insert(index, (Uri)value); } bool IList.IsFixedSize { get { return ((IList)_uriList).IsFixedSize; } } bool IList.IsReadOnly { get { return ((IList)_uriList).IsReadOnly; } } void IList.Remove(object value) { ((IList )this).Remove((Uri)value); } void IList.RemoveAt(int index) { ((IList )this).RemoveAt(index); } object IList.this[int index] { get { return _uriList[index]; } set { ((IList )this)[index] = (Uri)value; } } #endregion #region ICollection Members void ICollection.CopyTo(Array array, int index) { ((ICollection)_uriList).CopyTo(array, index); } int ICollection.Count { get { return ((IList )this).Count; } } bool ICollection.IsSynchronized { get { return ((ICollection)_uriList).IsSynchronized; } } object ICollection.SyncRoot { get { return ((ICollection)_uriList).SyncRoot; } } #endregion //------------------------------------------------------ // // Private Properties // //------------------------------------------------------ #region Private Properties private Speller Speller { get { if (_owner.TextEditor == null) { return null; } return _owner.TextEditor.Speller; } } #endregion Private Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods /// /// Makes sure Uri is of supported kind. Throws exception for unsupported URI type. /// Supported URI types [please keep this list updated] /// 1. Local path /// 2. Relative path /// 3. UNC path /// 4. Pack URI /// /// private static void ValidateUri(Uri item) { if (item == null) { throw new ArgumentException(SR.Get(SRID.CustomDictionaryNullItem)); } if (item.IsAbsoluteUri) { if (!(item.IsUnc || item.IsFile || System.IO.Packaging.PackUriHelper.IsPackUri(item))) { throw new NotSupportedException(SR.Get(SRID.CustomDictionarySourcesUnsupportedURI)); } } } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private readonly List_uriList; private readonly TextBoxBase _owner; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: CustomDictionarySources.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Collection of Uri objects used to specify location of custom dictionaries. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Text; using System.Windows.Markup; using System.Windows.Navigation; using System.Windows.Controls.Primitives; using System.Windows.Documents; namespace System.Windows.Controls { /// /// Collection of ///objects representing local file system or UNC paths. /// Methods that modify the collection also call into Speller callbakcs to notify about the change, so Speller /// could load/unload appropriate dicitonary files. /// /// Implements IList interface with 1 restriction - does not allow double addition of same item to /// the collection. /// For all methods (except Inser) that modify the collection behavior is to ignore add/set request, /// but still notify the Speller. /// In case of Insert an exception is thrown if the item already exists in the collection. /// /// There is a restriction on kinds of supported Uri objects, which is enforced in internal class CustomDictionarySources : IListmethod. /// Refer to this method for supported types of URIs. /// , IList { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region constructors internal CustomDictionarySources(TextBoxBase owner) { _owner = owner; _uriList = new List (); } #endregion constructors //------------------------------------------------------ // // Interface implementations // //----------------------------------------------------- #region Interface implementations #region IEnumerable Members public IEnumerator GetEnumerator() { return _uriList.GetEnumerator(); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return _uriList.GetEnumerator(); } #endregion #endregion Interface implementations #region IList Members int IList .IndexOf(Uri item) { return _uriList.IndexOf(item); } /// /// Implementation of Insert method. A restriction added by this implementation is that /// it will throw exception if the item was already added previously. /// This is to avoid ambiguity in respect to the expected position (index) of the inserted item. /// Caller will have to check first if item was already added. /// /// /// void IList.Insert(int index, Uri item) { if (_uriList.Contains(item)) { throw new ArgumentException(SR.Get(SRID.CustomDictionaryItemAlreadyExists), "item"); } ValidateUri(item); _uriList.Insert(index, item); if (Speller != null) { Speller.OnDictionaryUriAdded(item); } } void IList .RemoveAt(int index) { Uri uri = _uriList[index]; _uriList.RemoveAt(index); if (Speller != null) { Speller.OnDictionaryUriRemoved(uri); } } /// /// Sets value at specified index. /// Speller is notified that value at the index is being replaced, which means /// current value at given offset is removed, and new value is added at the same index. /// /// ///Uri IList .this[int index] { get { return _uriList[index]; } set { ValidateUri(value); Uri oldUri = _uriList[index]; if (Speller != null) { Speller.OnDictionaryUriRemoved(oldUri); } _uriList[index] = value; if (Speller != null) { Speller.OnDictionaryUriAdded(value); } } } #endregion #region ICollection Members /// /// Adds new item to the internal collection. /// Duplicate items ARE NOT added, but Speller is still notified. /// /// void ICollection.Add(Uri item) { ValidateUri(item); if (!_uriList.Contains(item)) { _uriList.Add(item); } if (Speller != null) { Speller.OnDictionaryUriAdded(item); } } void ICollection .Clear() { _uriList.Clear(); if (Speller != null) { Speller.OnDictionaryUriCollectionCleared(); } } bool ICollection .Contains(Uri item) { return _uriList.Contains(item); } void ICollection .CopyTo(Uri[] array, int arrayIndex) { _uriList.CopyTo(array, arrayIndex); } int ICollection .Count { get { return _uriList.Count; } } bool ICollection .IsReadOnly { get { return ((ICollection )_uriList).IsReadOnly; } } bool ICollection .Remove(Uri item) { bool removed = _uriList.Remove(item); if (removed && (Speller != null)) { Speller.OnDictionaryUriRemoved(item); } return removed; } #endregion #region IList Members /// /// See generic IList'Uri implementation notes. /// /// ///int IList.Add(object value) { ((IList )this).Add((Uri)value); return _uriList.IndexOf((Uri)value); } void IList.Clear() { ((IList )this).Clear(); } bool IList.Contains(object value) { return ((IList)_uriList).Contains(value); } int IList.IndexOf(object value) { return ((IList)_uriList).IndexOf(value); } void IList.Insert(int index, object value) { ((IList )this).Insert(index, (Uri)value); } bool IList.IsFixedSize { get { return ((IList)_uriList).IsFixedSize; } } bool IList.IsReadOnly { get { return ((IList)_uriList).IsReadOnly; } } void IList.Remove(object value) { ((IList )this).Remove((Uri)value); } void IList.RemoveAt(int index) { ((IList )this).RemoveAt(index); } object IList.this[int index] { get { return _uriList[index]; } set { ((IList )this)[index] = (Uri)value; } } #endregion #region ICollection Members void ICollection.CopyTo(Array array, int index) { ((ICollection)_uriList).CopyTo(array, index); } int ICollection.Count { get { return ((IList )this).Count; } } bool ICollection.IsSynchronized { get { return ((ICollection)_uriList).IsSynchronized; } } object ICollection.SyncRoot { get { return ((ICollection)_uriList).SyncRoot; } } #endregion //------------------------------------------------------ // // Private Properties // //------------------------------------------------------ #region Private Properties private Speller Speller { get { if (_owner.TextEditor == null) { return null; } return _owner.TextEditor.Speller; } } #endregion Private Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods /// /// Makes sure Uri is of supported kind. Throws exception for unsupported URI type. /// Supported URI types [please keep this list updated] /// 1. Local path /// 2. Relative path /// 3. UNC path /// 4. Pack URI /// /// private static void ValidateUri(Uri item) { if (item == null) { throw new ArgumentException(SR.Get(SRID.CustomDictionaryNullItem)); } if (item.IsAbsoluteUri) { if (!(item.IsUnc || item.IsFile || System.IO.Packaging.PackUriHelper.IsPackUri(item))) { throw new NotSupportedException(SR.Get(SRID.CustomDictionarySourcesUnsupportedURI)); } } } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private readonly List_uriList; private readonly TextBoxBase _owner; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
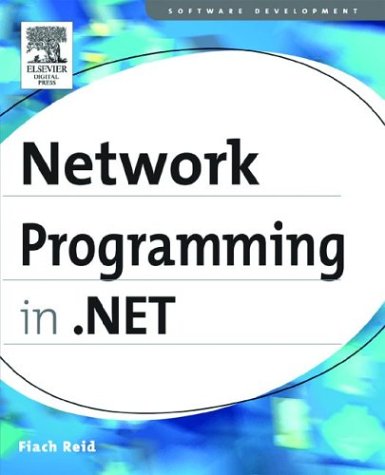
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GroupBox.cs
- ServiceHostFactory.cs
- DataSourceHelper.cs
- RealizationDrawingContextWalker.cs
- DragStartedEventArgs.cs
- PlanCompiler.cs
- DependencyPropertyKey.cs
- PersonalizationProviderCollection.cs
- Preprocessor.cs
- RectangleHotSpot.cs
- ReachDocumentReferenceSerializer.cs
- SystemGatewayIPAddressInformation.cs
- CodeIdentifier.cs
- CodeObject.cs
- StaticSiteMapProvider.cs
- X509SecurityTokenAuthenticator.cs
- GridViewRowCollection.cs
- WebPartConnection.cs
- _Semaphore.cs
- ByteStorage.cs
- SplineKeyFrames.cs
- MethodBody.cs
- HandlerBase.cs
- SqlDataSourceQueryEditor.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- DataGridViewBindingCompleteEventArgs.cs
- FormsIdentity.cs
- NativeRightsManagementAPIsStructures.cs
- TableLayoutSettingsTypeConverter.cs
- AutomationElement.cs
- SingleAnimationBase.cs
- TranslateTransform3D.cs
- JulianCalendar.cs
- TiffBitmapEncoder.cs
- RankException.cs
- Rotation3DAnimationBase.cs
- IDictionary.cs
- NativeMethods.cs
- Listener.cs
- UnsupportedPolicyOptionsException.cs
- EntitySqlQueryBuilder.cs
- QualificationDataItem.cs
- DocumentViewerBaseAutomationPeer.cs
- SelfIssuedAuthRSACryptoProvider.cs
- XsdBuildProvider.cs
- WebZone.cs
- PropertyConverter.cs
- ReadingWritingEntityEventArgs.cs
- OdbcConnectionFactory.cs
- ConditionBrowserDialog.cs
- TransformerConfigurationWizardBase.cs
- UpdateManifestForBrowserApplication.cs
- HttpAsyncResult.cs
- RegexGroupCollection.cs
- ParameterReplacerVisitor.cs
- NamespaceInfo.cs
- TimeSpanParse.cs
- ParallelEnumerableWrapper.cs
- SelectQueryOperator.cs
- ScriptReferenceBase.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- Vector3DValueSerializer.cs
- DataSourceControlBuilder.cs
- SharedUtils.cs
- SessionViewState.cs
- SQLChars.cs
- StreamWriter.cs
- Overlapped.cs
- ZipIOModeEnforcingStream.cs
- IDReferencePropertyAttribute.cs
- ClientTargetCollection.cs
- PreviewControlDesigner.cs
- GlobalProxySelection.cs
- InlineCollection.cs
- Thickness.cs
- ListBoxItemWrapperAutomationPeer.cs
- KeyValuePairs.cs
- CodeSnippetTypeMember.cs
- PropertyDescriptorComparer.cs
- HyperLinkColumn.cs
- BatchParser.cs
- NameValuePair.cs
- OverrideMode.cs
- NavigationEventArgs.cs
- CategoryNameCollection.cs
- Annotation.cs
- MessageQueuePermissionEntryCollection.cs
- CompiledQueryCacheEntry.cs
- AstNode.cs
- TemplateComponentConnector.cs
- ToolStripTextBox.cs
- GridViewRowCollection.cs
- MiniParameterInfo.cs
- ResourceDescriptionAttribute.cs
- XmlStringTable.cs
- EntityDesignPluralizationHandler.cs
- WindowsTooltip.cs
- AdornerDecorator.cs
- IPPacketInformation.cs
- XmlCharType.cs