Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Unary / SelectQueryOperator.cs / 1305376 / SelectQueryOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // SelectQueryOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// The operator type for Select statements. This operator transforms elements as it /// enumerates them through the use of a selector delegate. /// ////// internal sealed class SelectQueryOperator : UnaryQueryOperator { // Selector function. Used to project elements to a transformed view during execution. private Func m_selector; //---------------------------------------------------------------------------------------- // Initializes a new select operator. // // Arguments: // child - the child operator or data source from which to pull data // selector - a delegate representing the selector function // // Assumptions: // selector must be non null. // internal SelectQueryOperator(IEnumerable child, Func selector) :base(child) { Contract.Assert(child != null, "child data source cannot be null"); Contract.Assert(selector != null, "need a selector function"); m_selector = selector; SetOrdinalIndexState(Child.OrdinalIndexState); } internal override void WrapPartitionedStream ( PartitionedStream inputStream, IPartitionedStreamRecipient recipient, bool preferStriping, QuerySettings settings) { PartitionedStream outputStream = new PartitionedStream (inputStream.PartitionCount, inputStream.KeyComparer, OrdinalIndexState); for (int i = 0; i < inputStream.PartitionCount; i++) { outputStream[i] = new SelectQueryOperatorEnumerator (inputStream[i], m_selector); } recipient.Receive(outputStream); } //--------------------------------------------------------------------------------------- // Just opens the current operator, including opening the child and wrapping it with // partitions as needed. // internal override QueryResults Open(QuerySettings settings, bool preferStriping) { QueryResults childQueryResults = Child.Open(settings, preferStriping); return SelectQueryOperatorResults.NewResults(childQueryResults, this, settings, preferStriping); } internal override IEnumerable AsSequentialQuery(CancellationToken token) { return Child.AsSequentialQuery(token).Select(m_selector); } //--------------------------------------------------------------------------------------- // Whether this operator performs a premature merge. // internal override bool LimitsParallelism { get { return false; } } //--------------------------------------------------------------------------------------- // The enumerator type responsible for projecting elements as it is walked. // class SelectQueryOperatorEnumerator : QueryOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The data source to enumerate. private readonly Func m_selector; // The actual select function. //---------------------------------------------------------------------------------------- // Instantiates a new select enumerator. // internal SelectQueryOperatorEnumerator(QueryOperatorEnumerator source, Func selector) { Contract.Assert(source != null); Contract.Assert(selector != null); m_source = source; m_selector = selector; } //--------------------------------------------------------------------------------------- // Straightforward IEnumerator methods. // internal override bool MoveNext(ref TOutput currentElement, ref TKey currentKey) { // So long as the source has a next element, we have an element. TInput element = default(TInput); if (m_source.MoveNext(ref element, ref currentKey)) { Contract.Assert(m_selector != null, "expected a compiled operator"); currentElement = m_selector(element); return true; } return false; } protected override void Dispose(bool disposing) { m_source.Dispose(); } } //------------------------------------------------------------------------------------ // Query results for a Select operator. The results are indexible if the child // results were indexible. // class SelectQueryOperatorResults : UnaryQueryOperatorResults { private Func m_selector; // Selector function private int m_childCount; // The number of elements in child results public static QueryResults NewResults( QueryResults childQueryResults, SelectQueryOperator op, QuerySettings settings, bool preferStriping) { if (childQueryResults.IsIndexible) { return new SelectQueryOperatorResults(childQueryResults, op, settings, preferStriping); } else { return new UnaryQueryOperatorResults(childQueryResults, op, settings, preferStriping); } } private SelectQueryOperatorResults( QueryResults childQueryResults, SelectQueryOperator op, QuerySettings settings, bool preferStriping) : base(childQueryResults, op, settings, preferStriping) { Contract.Assert(op.m_selector != null); m_selector = op.m_selector; Contract.Assert(m_childQueryResults.IsIndexible); m_childCount = m_childQueryResults.ElementsCount; } internal override bool IsIndexible { get { return true; } } internal override int ElementsCount { get { return m_childCount; } } internal override TOutput GetElement(int index) { Contract.Assert(index >= 0); Contract.Assert(index < ElementsCount); return m_selector(m_childQueryResults.GetElement(index)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
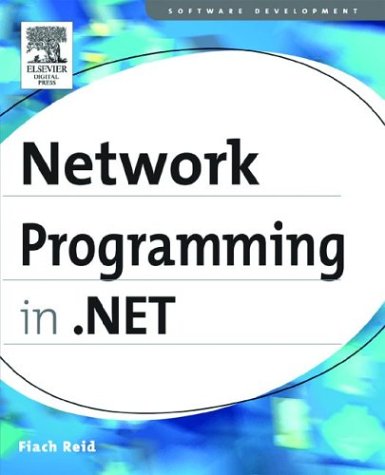
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LabelLiteral.cs
- Header.cs
- SHA512CryptoServiceProvider.cs
- TransactionFlowBindingElementImporter.cs
- InvalidOleVariantTypeException.cs
- DataServiceProviderMethods.cs
- EntityDataSource.cs
- TextClipboardData.cs
- TimeSpanSecondsConverter.cs
- DataGridViewLinkColumn.cs
- ResourcesBuildProvider.cs
- SqlTopReducer.cs
- HandleCollector.cs
- Material.cs
- NumericExpr.cs
- AncestorChangedEventArgs.cs
- CertificateReferenceElement.cs
- LocationUpdates.cs
- TemplatedWizardStep.cs
- ExecutionEngineException.cs
- LocalServiceSecuritySettings.cs
- XamlParser.cs
- ICspAsymmetricAlgorithm.cs
- Panel.cs
- URI.cs
- Model3D.cs
- NgenServicingAttributes.cs
- IndentedWriter.cs
- RuntimeConfigLKG.cs
- TripleDES.cs
- TdsParserSessionPool.cs
- MenuAutomationPeer.cs
- SymbolDocumentGenerator.cs
- DotExpr.cs
- MachineKeySection.cs
- SqlGatherConsumedAliases.cs
- CriticalHandle.cs
- DependencyObjectProvider.cs
- SQLInt32.cs
- CompilationUtil.cs
- CssTextWriter.cs
- ToolStripItemEventArgs.cs
- errorpatternmatcher.cs
- MouseEvent.cs
- SystemIcons.cs
- SchemaNamespaceManager.cs
- DesignerTransaction.cs
- StringSorter.cs
- OdbcFactory.cs
- TextViewSelectionProcessor.cs
- HashLookup.cs
- Array.cs
- ActivationWorker.cs
- ObjectHelper.cs
- FormsAuthenticationUserCollection.cs
- XPathNodePointer.cs
- ViewPort3D.cs
- PauseStoryboard.cs
- SystemMulticastIPAddressInformation.cs
- CollectionViewProxy.cs
- TypeElement.cs
- assertwrapper.cs
- DrawingContext.cs
- NativeMethods.cs
- Boolean.cs
- HttpWrapper.cs
- mda.cs
- EventLogPermissionAttribute.cs
- XslAst.cs
- DLinqTableProvider.cs
- PlanCompiler.cs
- ClientEventManager.cs
- OrthographicCamera.cs
- MeshGeometry3D.cs
- MemberAccessException.cs
- InstanceNormalEvent.cs
- NetworkInformationPermission.cs
- XmlAnyElementAttributes.cs
- FlagsAttribute.cs
- MenuItemStyleCollectionEditor.cs
- DataSetMappper.cs
- WebDisplayNameAttribute.cs
- VectorKeyFrameCollection.cs
- UInt16Converter.cs
- DataGridViewCheckBoxCell.cs
- KeysConverter.cs
- ApplicationSecurityInfo.cs
- PrintPreviewControl.cs
- XLinq.cs
- DotNetATv1WindowsLogEntrySerializer.cs
- CustomErrorCollection.cs
- Classification.cs
- ErrorView.xaml.cs
- DataGridViewColumnCollection.cs
- AttributeCollection.cs
- UrlAuthorizationModule.cs
- AxisAngleRotation3D.cs
- ContractListAdapter.cs
- WebPartZoneCollection.cs
- VectorAnimationBase.cs