Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Recovery / DotNetATv1WindowsLogEntrySerializer.cs / 1 / DotNetATv1WindowsLogEntrySerializer.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains the knowledge of how to serialize a v1 WS-AT log entry record using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.IO; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.ServiceModel; using System.Text; using System.Xml; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.Recovery { [Flags] enum WsATv1LogEntryFlags : byte { OptimizedEndpointRepresentation = 0x01, UsesDefaultPort = 0x02, UsesStandardCoordinatorAddressPath = 0x04, UsesStandardParticipantAddressPath = 0x08, } enum WsATv1LogEntryVersion : byte { v1 = 1, v2 = 2, } class WsATv1LogEntrySerializer : LogEntrySerializer { ProtocolVersion protocolVersion; static readonly string standardCoordinatorAddressPath10 = "/" + BindingStrings.AddressPrefix + "/" + BindingStrings.TwoPhaseCommitCoordinatorSuffix(ProtocolVersion.Version10); static readonly string standardCoordinatorAddressPath11 = "/" + BindingStrings.AddressPrefix + "/" + BindingStrings.TwoPhaseCommitCoordinatorSuffix(ProtocolVersion.Version11); static readonly string standardParticipantAddressPath10 = "/" + BindingStrings.AddressPrefix + "/" + BindingStrings.TwoPhaseCommitParticipantSuffix(ProtocolVersion.Version10); static readonly string standardParticipantAddressPath11 = "/" + BindingStrings.AddressPrefix + "/" + BindingStrings.TwoPhaseCommitParticipantSuffix(ProtocolVersion.Version11); public WsATv1LogEntrySerializer(LogEntry logEntry, ProtocolVersion protocolVersion) : base(logEntry) { this.protocolVersion = protocolVersion; } public static string StandardCoordinatorAddressPath (ProtocolVersion protocolVersion) { ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, typeof(WsATv1LogEntrySerializer), "StandardCoordinatorAddressPath"); //assert valid protocol version switch (protocolVersion) { case ProtocolVersion.Version10 : return standardCoordinatorAddressPath10; case ProtocolVersion.Version11 : return standardCoordinatorAddressPath11; default : return null; // inaccessible path because we have asserted the protocol version } } public static string StandardParticipantAddressPath (ProtocolVersion protocolVersion) { ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, typeof(WsATv1LogEntrySerializer), "StandardParticipantAddressPath"); //assert valid protocol version switch (protocolVersion) { case ProtocolVersion.Version10 : return standardParticipantAddressPath10; case ProtocolVersion.Version11 : return standardParticipantAddressPath11; default : return null; // inaccessible path because we have asserted the protocol version } } protected override void SerializeExtended() { DebugTrace.TraceEnter(this, "SerializeExtended"); WsATv1LogEntryFlags flags = 0, pathFlags = 0; EndpointAddress address = this.logEntry.Endpoint; Uri uri = address.Uri; // Check for a remote enlistment id (and nothing else) in the ref params // If we don't have this, give up and fallback to serializing the entire EPR Guid remoteEnlistmentId; if (GetRemoteEnlistmentId(address, out remoteEnlistmentId)) { // Great. We can optimize the serialization of this EPR flags |= WsATv1LogEntryFlags.OptimizedEndpointRepresentation; // Make sure the EPR contains an https address // It is worth noting that we will not reach this point without an address // that the HttpsChannelFactory has accepted, but it's worth checking anyway if (string.Compare(uri.Scheme, Uri.UriSchemeHttps, StringComparison.OrdinalIgnoreCase) != 0) { DiagnosticUtility.FailFast("Endpoints must use the HTTPS scheme"); } // Check for the default port if (Configuration.DefaultHttpsPort == uri.Port) { flags |= WsATv1LogEntryFlags.UsesDefaultPort; } // Check for a standard address path pathFlags = GetPathFlags(address, this.protocolVersion); flags |= pathFlags; } // // Write extended information to the stream // if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "SerializeExtended flags: {0}", flags); } // Version byte version = 0; ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, this.GetType(), "SerializeExtended"); //assert valid protocol version switch (this.protocolVersion) { case ProtocolVersion.Version10 : version = (byte)WsATv1LogEntryVersion.v1; break; case ProtocolVersion.Version11 : version = (byte)WsATv1LogEntryVersion.v2; break; // no default - we already asserted the protocol version } this.mem.WriteByte(version); if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Wrote version: {0} bytes", this.mem.Length); } // Flags this.mem.WriteByte((byte)flags); if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Wrote flags: {0} bytes", this.mem.Length); } if ((flags & WsATv1LogEntryFlags.OptimizedEndpointRepresentation) == 0) { SerializationUtils.WriteEndpointAddress(this.mem, address, this.protocolVersion); } else { // Remote enlistmentId SerializationUtils.WriteGuid(this.mem, ref remoteEnlistmentId); if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Wrote enlistmentId: {0} bytes", this.mem.Length); } // HostName SerializationUtils.WriteString(this.mem, uri.Host); if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Wrote hostName: {0} bytes", this.mem.Length); } // Port if ((flags & WsATv1LogEntryFlags.UsesDefaultPort) == 0) { if (!(uri.Port >= System.Net.IPEndPoint.MinPort && uri.Port <= System.Net.IPEndPoint.MaxPort)) { // Between the time we captured the port and the time we write it out, // the value should still appear to be valid. If things change, we have // a bug somewhere and need to crash so that we don't write an invalid log entry. DiagnosticUtility.FailFast("TCP port must be valid"); } SerializationUtils.WriteInt(this.mem, uri.Port); if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Wrote port: {0} bytes", this.mem.Length); } } // Path if (pathFlags == 0) { SerializationUtils.WriteString(this.mem, uri.AbsolutePath); if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Wrote address path: {0} bytes", this.mem.Length); } } } DebugTrace.TraceLeave(this, "DeserializeExtended"); } static WsATv1LogEntryFlags GetPathFlags(EndpointAddress address, ProtocolVersion protocolVersion) { if (address.Uri.AbsolutePath == StandardCoordinatorAddressPath(protocolVersion)) { return WsATv1LogEntryFlags.UsesStandardCoordinatorAddressPath; } else if (address.Uri.AbsolutePath == StandardParticipantAddressPath(protocolVersion)) { return WsATv1LogEntryFlags.UsesStandardParticipantAddressPath; } else { return 0; } } static bool GetRemoteEnlistmentId(EndpointAddress address, out Guid remoteEnlistmentId) { AddressHeaderCollection refParams = address.Headers; if (refParams.Count == 1) { // We don't have to worry about catching ArgumentException here because // we already checked that there's only one header in the collection. AddressHeader refParam = refParams.FindHeader(EnlistmentHeader.HeaderName, EnlistmentHeader.HeaderNamespace); if (refParam != null) { XmlDictionaryReader reader = refParam.GetAddressHeaderReader(); using (reader) { try { ControlProtocol protocol; EnlistmentHeader.ReadFrom(reader, out remoteEnlistmentId, out protocol); return protocol == ControlProtocol.Durable2PC; } catch (InvalidEnlistmentHeaderException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Information); } } } } remoteEnlistmentId = Guid.Empty; return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
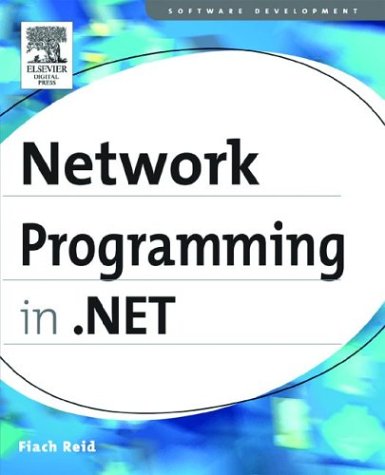
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServicePointManagerElement.cs
- TraceContextRecord.cs
- NextPreviousPagerField.cs
- TraceSource.cs
- SeekableMessageNavigator.cs
- CoTaskMemUnicodeSafeHandle.cs
- ReferencedAssembly.cs
- Queue.cs
- StrokeDescriptor.cs
- VisualProxy.cs
- DecoderFallbackWithFailureFlag.cs
- DelegatingTypeDescriptionProvider.cs
- ValidationError.cs
- EncoderFallback.cs
- ClassDataContract.cs
- SaveWorkflowAsyncResult.cs
- XmlDictionaryReaderQuotasElement.cs
- SerializationObjectManager.cs
- InstanceHandle.cs
- GcSettings.cs
- HighlightComponent.cs
- HttpCachePolicy.cs
- XmlSchemaImporter.cs
- Atom10FormatterFactory.cs
- SQLInt64Storage.cs
- LicenseManager.cs
- Visual.cs
- FlowLayoutPanelDesigner.cs
- XamlRtfConverter.cs
- CompilerResults.cs
- TextBoxBase.cs
- DetailsViewModeEventArgs.cs
- BindingValueChangedEventArgs.cs
- ControlPaint.cs
- ProfileEventArgs.cs
- SharedStream.cs
- TextSpan.cs
- AnimatedTypeHelpers.cs
- InternalControlCollection.cs
- Popup.cs
- SqlParameterCollection.cs
- ObjectListDesigner.cs
- GeometryHitTestResult.cs
- CompositionTarget.cs
- ListBox.cs
- OdbcConnectionFactory.cs
- XPathItem.cs
- InvalidEnumArgumentException.cs
- UnsafeNativeMethods.cs
- HashMembershipCondition.cs
- TextModifierScope.cs
- FlowDecisionLabelFeature.cs
- InputReport.cs
- RawMouseInputReport.cs
- RectConverter.cs
- cookie.cs
- NumericExpr.cs
- CodeParameterDeclarationExpression.cs
- ObjectKeyFrameCollection.cs
- CodeMethodInvokeExpression.cs
- ToolStripOverflowButton.cs
- DataGridViewColumn.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- ObservableDictionary.cs
- WindowsTokenRoleProvider.cs
- ErrorRuntimeConfig.cs
- RectKeyFrameCollection.cs
- PermissionSetEnumerator.cs
- WebPermission.cs
- UIPropertyMetadata.cs
- PopupRoot.cs
- DataGridDetailsPresenter.cs
- CryptoStream.cs
- LassoHelper.cs
- SetterBase.cs
- DoubleConverter.cs
- TrustSection.cs
- AppDomainManager.cs
- RewritingProcessor.cs
- WebPartTransformerCollection.cs
- CellTreeSimplifier.cs
- PreProcessInputEventArgs.cs
- DirtyTextRange.cs
- DataGridViewColumnCollection.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- ConfigurationValue.cs
- MultiByteCodec.cs
- SinglePageViewer.cs
- SQLBytes.cs
- CleanUpVirtualizedItemEventArgs.cs
- LifetimeServices.cs
- DataQuery.cs
- StreamGeometry.cs
- PropertyValidationContext.cs
- AttributeProviderAttribute.cs
- Message.cs
- EncryptedKey.cs
- PasswordRecovery.cs
- BamlTreeUpdater.cs
- ArraySortHelper.cs