Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / Listener.cs / 1 / Listener.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Define the representation of a ServiceModel service and its associated metadata using System; using System.ServiceModel.Dispatcher; using System.ServiceModel.Description; using System.Diagnostics; using System.Globalization; using System.Security.Principal; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Security; using System.ServiceModel.Security.Tokens; using System.Transactions; namespace Microsoft.Transactions.Wsat.Messaging { interface ICoordinationListener { void Start(); void Stop(); EndpointAddress CreateEndpointReference(AddressHeader refParam); } class CoordinationServiceHost : ServiceHost, ICoordinationListener { CoordinationService service; EndpointAddress baseEndpoint; public CoordinationServiceHost(CoordinationService service, object serviceInstance) { this.service = service; base.InitializeDescription(serviceInstance, new UriSchemeKeyedCollection()); } // This is called during Start() // The idea is to obtain a base endpoint before we're actually on the network // This prevents races between the initialization path and incoming messages protected override void InitializeRuntime() { DebugTrace.TraceEnter(this, "OnCreateListeners"); base.InitializeRuntime(); if (!(this.SingletonInstance is IWSActivationCoordinator)) { CreateBaseEndpointAddress(); } else if (DebugTrace.Info) { for (int i=0; i(); CoordinationStrings coordinationStrings = CoordinationStrings.Version(this.service.ProtocolVersion); if (sbe != null) { if (!sbe.OptionalOperationSupportingTokenParameters.ContainsKey(coordinationStrings.RegisterAction)) { sbe.OptionalOperationSupportingTokenParameters.Add(coordinationStrings.RegisterAction, new SupportingTokenParameters()); } sbe.OptionalOperationSupportingTokenParameters[coordinationStrings.RegisterAction].Endorsing.Add(CoordinationServiceSecurity.SecurityContextSecurityTokenParameters); endpoint.Binding = customBinding; } } } this.Open(); if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Opened ServiceHost for {0}", this.SingletonInstance.GetType().Name); } } catch (CommunicationException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MessagingInitializationException( SR.GetString(SR.ListenerCannotBeStarted, this.baseEndpoint.Uri, e.Message), e)); } } public void Stop() { try { this.Close(); } catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Error); } catch (TimeoutException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Error); } } public EndpointAddress CreateEndpointReference(AddressHeader refParam) { if (this.baseEndpoint == null) { // Validating assumption that the base endpoint will be set. // We assume that this function will only be called when we're // done initializing. If we call this function earlier, the caller // has a bug. DiagnosticUtility.FailFast("Uninitialized base endpoint reference"); } EndpointAddressBuilder builder = new EndpointAddressBuilder(this.baseEndpoint); builder.Headers.Clear(); builder.Headers.Add(refParam); return builder.ToEndpointAddress(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
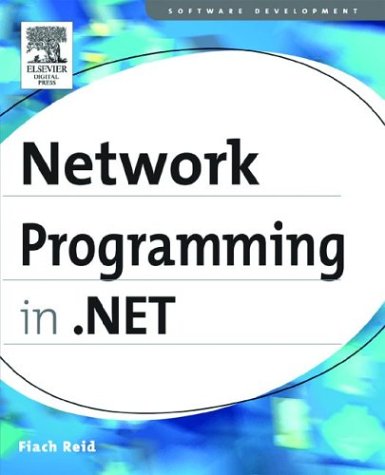
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypedElement.cs
- PropertyFilterAttribute.cs
- DefaultProfileManager.cs
- CommonGetThemePartSize.cs
- DelegateTypeInfo.cs
- SizeValueSerializer.cs
- FigureParaClient.cs
- ConversionValidationRule.cs
- UriTemplateMatch.cs
- TargetConverter.cs
- BrushValueSerializer.cs
- TextDecorationCollection.cs
- Root.cs
- loginstatus.cs
- ThemeableAttribute.cs
- ServiceNameElementCollection.cs
- DependsOnAttribute.cs
- UriParserTemplates.cs
- cookieexception.cs
- _LocalDataStore.cs
- HotSpot.cs
- NotSupportedException.cs
- ThreadExceptionEvent.cs
- HttpListenerContext.cs
- WorkflowApplicationAbortedException.cs
- TemplateParser.cs
- RenderContext.cs
- SafeProcessHandle.cs
- Lookup.cs
- ResolvedKeyFrameEntry.cs
- StringHandle.cs
- CompositeCollection.cs
- QilName.cs
- GenericTextProperties.cs
- Section.cs
- TemplateManager.cs
- ServerIdentity.cs
- ResolvedKeyFrameEntry.cs
- HttpCapabilitiesBase.cs
- FilterableAttribute.cs
- EntityTypeEmitter.cs
- ThousandthOfEmRealDoubles.cs
- XmlSchemaParticle.cs
- NameNode.cs
- CharEnumerator.cs
- ListMarkerSourceInfo.cs
- ControlPropertyNameConverter.cs
- CmsInterop.cs
- SafeArrayTypeMismatchException.cs
- DoubleAnimationUsingKeyFrames.cs
- CodeStatement.cs
- PrintPageEvent.cs
- UseLicense.cs
- LoadItemsEventArgs.cs
- DbModificationClause.cs
- BuildProviderAppliesToAttribute.cs
- WorkItem.cs
- PrtCap_Builder.cs
- HtmlAnchor.cs
- DifferencingCollection.cs
- ThicknessKeyFrameCollection.cs
- ImageListStreamer.cs
- ControlAdapter.cs
- PackageRelationship.cs
- SessionPageStateSection.cs
- DataListAutoFormat.cs
- TemplatedMailWebEventProvider.cs
- AsyncResult.cs
- WindowsSlider.cs
- InertiaRotationBehavior.cs
- XmlSchemaExternal.cs
- XmlDomTextWriter.cs
- AttachedPropertyBrowsableAttribute.cs
- BamlLocalizableResource.cs
- IUnknownConstantAttribute.cs
- SortKey.cs
- XamlWrappingReader.cs
- ErrorHandler.cs
- SupportingTokenParameters.cs
- TextAnchor.cs
- PresentationSource.cs
- DataServiceProviderWrapper.cs
- StringValidator.cs
- VisualCollection.cs
- DataRelationCollection.cs
- SecurityState.cs
- WsdlBuildProvider.cs
- QilFunction.cs
- ExpandCollapseProviderWrapper.cs
- PreservationFileReader.cs
- _CacheStreams.cs
- GridLengthConverter.cs
- Journaling.cs
- GeneralTransform3DCollection.cs
- IncrementalReadDecoders.cs
- ControlAdapter.cs
- PointLightBase.cs
- ParseHttpDate.cs
- LambdaCompiler.Logical.cs
- DbSetClause.cs