Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Activities / Common / DelegateTypeInfo.cs / 1305376 / DelegateTypeInfo.cs
// Copyright (c) Microsoft Corporation. All rights reserved. // // THIS CODE AND INFORMATION IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, // WHETHER EXPRESSED OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE IMPLIED // WARRANTIES OF MERCHANTABILITY AND/OR FITNESS FOR A PARTICULAR PURPOSE. // THE ENTIRE RISK OF USE OR RESULTS IN CONNECTION WITH THE USE OF THIS CODE // AND INFORMATION REMAINS WITH THE USER. // /********************************************************************** * NOTE: A copy of this file exists at: WF\Common\Shared * The two files must be kept in [....]. Any change made here must also * be made to WF\Common\Shared\DelegateTypeInfo.cs *********************************************************************/ namespace System.Workflow.Activities.Common { using System; using System.CodeDom; using System.Collections; using System.Globalization; using System.ComponentModel; using System.ComponentModel.Design; using System.Reflection; using System.Diagnostics.CodeAnalysis; internal class DelegateTypeInfo { private CodeParameterDeclarationExpression[] parameters; private Type[] parameterTypes; private CodeTypeReference returnType; internal CodeParameterDeclarationExpression[] Parameters { get { return parameters; } } internal Type[] ParameterTypes { get { return parameterTypes; } } internal CodeTypeReference ReturnType { get { return returnType; } } internal DelegateTypeInfo(Type delegateClass) { Resolve(delegateClass); } [SuppressMessage("Microsoft.Globalization", "CA1307:SpecifyStringComparison", Justification = "EndsWith(\"&\") not a security issue.")] private void Resolve(Type delegateClass) { MethodInfo invokeMethod = delegateClass.GetMethod("Invoke"); if (invokeMethod == null) throw new ArgumentException("delegateClass"); Resolve(invokeMethod); } [SuppressMessage("Microsoft.Globalization", "CA1307:SpecifyStringComparison", Justification = "EndsWith(\"&\") not a security issue.")] private void Resolve(MethodInfo method) { // Here we build up an array of argument types, separated // by commas. ParameterInfo[] argTypes = method.GetParameters(); parameters = new CodeParameterDeclarationExpression[argTypes.Length]; parameterTypes = new Type[argTypes.Length]; for (int index = 0; index < argTypes.Length; index++) { string paramName = argTypes[index].Name; Type paramType = argTypes[index].ParameterType; if (paramName == null || paramName.Length == 0) paramName = "param" + index.ToString(CultureInfo.InvariantCulture); FieldDirection fieldDir = FieldDirection.In; // check for the '&' that means ref (gotta love it!) // and we need to strip that & before we continue. Ouch. if (paramType.IsByRef) { if (paramType.FullName.EndsWith("&")) { // strip the & and reload the type without it. paramType = paramType.Assembly.GetType(paramType.FullName.Substring(0, paramType.FullName.Length - 1), true); } fieldDir = FieldDirection.Ref; } if (argTypes[index].IsOut) { if (argTypes[index].IsIn) fieldDir = FieldDirection.Ref; else fieldDir = FieldDirection.Out; } parameters[index] = new CodeParameterDeclarationExpression(new CodeTypeReference(paramType), paramName); parameters[index].Direction = fieldDir; parameterTypes[index] = paramType; } this.returnType = new CodeTypeReference(method.ReturnType); } public override bool Equals(object other) { if (other == null) return false; DelegateTypeInfo dtiOther = other as DelegateTypeInfo; if (dtiOther == null) return false; if (ReturnType.BaseType != dtiOther.ReturnType.BaseType || Parameters.Length != dtiOther.Parameters.Length) return false; for (int parameter = 0; parameter < Parameters.Length; parameter++) { CodeParameterDeclarationExpression otherParam = dtiOther.Parameters[parameter]; if (otherParam.Type.BaseType != Parameters[parameter].Type.BaseType) return false; } return true; } public override int GetHashCode() { return base.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
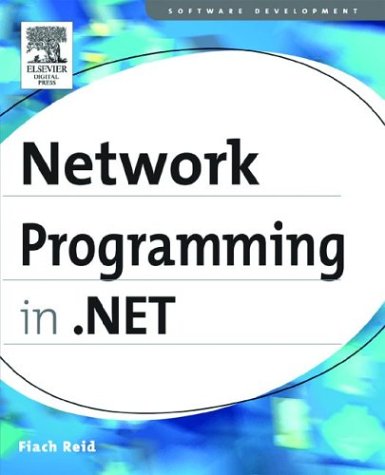
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlAttributeAttribute.cs
- SelectionService.cs
- DataListItemCollection.cs
- ApplicationDirectoryMembershipCondition.cs
- QilTernary.cs
- DurationConverter.cs
- HtmlInputFile.cs
- _CommandStream.cs
- AnimationClock.cs
- _DigestClient.cs
- ViewPort3D.cs
- Geometry.cs
- EditorPart.cs
- UnsafeNativeMethods.cs
- RewritingSimplifier.cs
- LightweightCodeGenerator.cs
- RawStylusActions.cs
- BindingEditor.xaml.cs
- ContentWrapperAttribute.cs
- ChildrenQuery.cs
- PropertyGridEditorPart.cs
- ItemAutomationPeer.cs
- FocusManager.cs
- SqlDataReader.cs
- SchemaAttDef.cs
- HttpValueCollection.cs
- ScalarOps.cs
- WebPartZoneBase.cs
- PackageRelationshipSelector.cs
- EventTrigger.cs
- RangeContentEnumerator.cs
- ContentPropertyAttribute.cs
- Encoding.cs
- OdbcFactory.cs
- __Filters.cs
- GridErrorDlg.cs
- FontUnit.cs
- validation.cs
- SafeProcessHandle.cs
- StorageInfo.cs
- WebZone.cs
- HandledMouseEvent.cs
- WindowsIPAddress.cs
- QueryGeneratorBase.cs
- SweepDirectionValidation.cs
- FlowLayoutPanelDesigner.cs
- IgnoreFileBuildProvider.cs
- UpnEndpointIdentity.cs
- LinkDescriptor.cs
- ToolStripLocationCancelEventArgs.cs
- ConfigurationFileMap.cs
- ProcessHostMapPath.cs
- IsolatedStorageFilePermission.cs
- BinaryObjectInfo.cs
- SerializationEventsCache.cs
- WrapperEqualityComparer.cs
- CodeConditionStatement.cs
- HttpContextServiceHost.cs
- XmlUrlResolver.cs
- TransformerInfo.cs
- TableRowCollection.cs
- EventListener.cs
- SqlTriggerAttribute.cs
- future.cs
- PageStatePersister.cs
- TiffBitmapDecoder.cs
- TemplateColumn.cs
- WMIInterop.cs
- SuppressMessageAttribute.cs
- LoginDesignerUtil.cs
- VolatileEnlistmentState.cs
- EpmSourcePathSegment.cs
- File.cs
- OutputBuffer.cs
- COM2IDispatchConverter.cs
- XmlHierarchicalEnumerable.cs
- HttpCapabilitiesSectionHandler.cs
- MachinePropertyVariants.cs
- SafeWaitHandle.cs
- LocalizedNameDescriptionPair.cs
- TextParagraphProperties.cs
- Rotation3D.cs
- ExternalCalls.cs
- ScrollViewer.cs
- Fx.cs
- WindowsFont.cs
- bidPrivateBase.cs
- BufferAllocator.cs
- GacUtil.cs
- MenuItem.cs
- LongPath.cs
- AppearanceEditorPart.cs
- MemberAccessException.cs
- VisualStyleRenderer.cs
- SchemaElementLookUpTable.cs
- WindowsHyperlink.cs
- FilterInvalidBodyAccessException.cs
- XmlTextEncoder.cs
- indexingfiltermarshaler.cs
- DataGridViewAdvancedBorderStyle.cs