Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / DurationConverter.cs / 1305600 / DurationConverter.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: DurationConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows { ////// Provides a type converter to convert Duration to and from other representations. /// public class DurationConverter : TypeConverter { ////// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// ///public override object ConvertFrom( ITypeDescriptorContext td, CultureInfo cultureInfo, object value) { string stringValue = value as string; // Override the converter for sentinel values if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Automatic") { return Duration.Automatic; } else if (stringValue == "Forever") { return Duration.Forever; } } TimeSpan duration = TimeSpan.Zero; if(_timeSpanConverter == null) { _timeSpanConverter = new TimeSpanConverter(); } duration = (TimeSpan)_timeSpanConverter.ConvertFrom(td, cultureInfo, value); return new Duration(duration); } /// /// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for Duration, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType != null && value is Duration) { Duration durationValue = (Duration)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; if (durationValue.HasTimeSpan) { mi = typeof(Duration).GetConstructor(new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { durationValue.TimeSpan }); } else if (durationValue == Duration.Forever) { mi = typeof(Duration).GetProperty("Forever"); return new InstanceDescriptor(mi, null); } else { Debug.Assert(durationValue == Duration.Automatic); // Only other legal duration type mi = typeof(Duration).GetProperty("Automatic"); return new InstanceDescriptor(mi, null); } } else if (destinationType == typeof(string)) { return durationValue.ToString(); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } private static TimeSpanConverter _timeSpanConverter; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
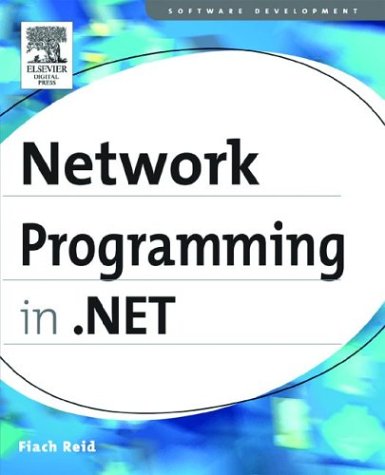
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ViewBox.cs
- HtmlInputHidden.cs
- Win32PrintDialog.cs
- XmlSchemaSimpleContent.cs
- Point3DConverter.cs
- PolyBezierSegment.cs
- DeploymentSectionCache.cs
- GenericTypeParameterConverter.cs
- NoPersistScope.cs
- GridPattern.cs
- NetPipeSection.cs
- SystemResourceKey.cs
- MruCache.cs
- NavigationService.cs
- BaseParser.cs
- UIElement3DAutomationPeer.cs
- AncestorChangedEventArgs.cs
- UserControlCodeDomTreeGenerator.cs
- ListParagraph.cs
- CreateUserWizardStep.cs
- CryptoStream.cs
- StartUpEventArgs.cs
- XmlIncludeAttribute.cs
- DeviceContexts.cs
- StreamInfo.cs
- RequestResizeEvent.cs
- RSAOAEPKeyExchangeFormatter.cs
- FunctionCommandText.cs
- UnsafeNativeMethods.cs
- DnsPermission.cs
- TracedNativeMethods.cs
- ExpressionHelper.cs
- OnOperation.cs
- ToolStripGrip.cs
- ImageListImage.cs
- ManualResetEvent.cs
- HighlightComponent.cs
- TreeBuilderBamlTranslator.cs
- HttpApplicationStateBase.cs
- TraceUtility.cs
- GridItemCollection.cs
- CompareValidator.cs
- ImageListStreamer.cs
- TemplateBaseAction.cs
- NotImplementedException.cs
- ArrayTypeMismatchException.cs
- LogRestartAreaEnumerator.cs
- XmlSignificantWhitespace.cs
- HtmlHistory.cs
- ExtensionQuery.cs
- ClientSettingsSection.cs
- Pens.cs
- DocumentPageHost.cs
- CalendarData.cs
- TypeDescriptionProvider.cs
- _NTAuthentication.cs
- XmlSecureResolver.cs
- ElementAtQueryOperator.cs
- SqlBooleanMismatchVisitor.cs
- D3DImage.cs
- ContainerControl.cs
- HtmlTable.cs
- BitmapMetadata.cs
- ItemCollection.cs
- GridViewColumnHeader.cs
- ToolstripProfessionalRenderer.cs
- StringResourceManager.cs
- TileBrush.cs
- SmiEventStream.cs
- XmlSerializerFactory.cs
- HttpHandlerActionCollection.cs
- XmlSchemaImport.cs
- RightsManagementResourceHelper.cs
- QilSortKey.cs
- UInt64Storage.cs
- XPathNodeIterator.cs
- FunctionNode.cs
- ADMembershipUser.cs
- ByteAnimationUsingKeyFrames.cs
- Metafile.cs
- ApplicationSettingsBase.cs
- oledbconnectionstring.cs
- DescriptionAttribute.cs
- ArraySubsetEnumerator.cs
- OutputCacheEntry.cs
- PackageRelationshipCollection.cs
- ListViewAutomationPeer.cs
- CryptoStream.cs
- KeyNotFoundException.cs
- WindowInteropHelper.cs
- HotSpotCollection.cs
- SimpleType.cs
- EntityDataSourceSelectingEventArgs.cs
- DataBoundControl.cs
- ConnectionStringSettingsCollection.cs
- AuthenticateEventArgs.cs
- ReturnType.cs
- MD5HashHelper.cs
- DocumentNUp.cs
- Shared.cs