Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / DocumentNUp.cs / 1 / DocumentNUp.cs
/*++ Copyright (C) 2003 Microsoft Corporation All rights reserved. Module Name: DocumentNUp.cs Abstract: Definition and implementation of this public feature/parameter related types. Author: [....] ([....]) 7/22/2003 --*/ using System; using System.IO; using System.Xml; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Printing; using MS.Internal.Printing.Configuration; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages namespace MS.Internal.Printing.Configuration { ////// Represents an NUp option. /// internal class NUpOption: PrintCapabilityOption { #region Constructors internal NUpOption(PrintCapabilityFeature ownerFeature) : base(ownerFeature) { _pagesPerSheet = PrintSchema.UnspecifiedIntValue; } #endregion Constructors #region Public Properties ////// Gets the NUp option's value of number of logical pages per physical sheet. /// public int PagesPerSheet { get { return _pagesPerSheet; } } #endregion Public Properties #region Public Methods ////// Converts the NUp option to human-readable string. /// ///A string that represents this NUp option. public override string ToString() { return PagesPerSheet.ToString(CultureInfo.CurrentCulture); } #endregion Public Methods #region Internal Fields internal int _pagesPerSheet; #endregion Internal Fields } ////// Represents NUp capability. /// abstract internal class NUpCapability : PrintCapabilityFeature { #region Constructors internal NUpCapability(InternalPrintCapabilities ownerPrintCap) : base(ownerPrintCap) { } #endregion Constructors #region Public Properties ////// Gets the collection object that represents document NUp options supported by the device. /// public CollectionNUps { get { return _nUps; } } /// /// Gets a Boolean value indicating whether the document NUp capability supports presentation direction. /// public bool SupportsPresentationDirection { get { return (PresentationDirectionCapability != null); } } ////// Gets the public NUpPresentationDirectionCapability PresentationDirectionCapability { get { return _presentationDirectionCap; } } #endregion Public Properties #region Internal Methods internal override sealed bool AddOptionCallback(PrintCapabilityOption baseOption) { bool added = false; NUpOption option = baseOption as NUpOption; // validate the option is complete before adding it to the collection if (option.PagesPerSheet > 0) { this.NUps.Add(option); added = true; } return added; } internal override sealed void AddSubFeatureCallback(PrintCapabilityFeature subFeature) { _presentationDirectionCap = subFeature as NUpPresentationDirectionCapability; } internal override sealed bool FeaturePropCallback(PrintCapabilityFeature feature, XmlPrintCapReader reader) { // no feature property to handle return false; } internal override sealed PrintCapabilityOption NewOptionCallback(PrintCapabilityFeature baseFeature) { NUpOption option = new NUpOption(baseFeature); return option; } internal override sealed void OptionAttrCallback(PrintCapabilityOption baseOption, XmlPrintCapReader reader) { // no option attribute to handle return; } ///object that represents the device's document NUp presentation direction capability. /// XML is not well-formed. internal override sealed bool OptionPropCallback(PrintCapabilityOption baseOption, XmlPrintCapReader reader) { NUpOption option = baseOption as NUpOption; bool handled = false; // we are only handling scored property here if (reader.CurrentElementNodeType == PrintSchemaNodeTypes.ScoredProperty) { if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.NUpKeys.PagesPerSheet) { try { option._pagesPerSheet = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } handled = true; } } return handled; } #endregion Internal Methods #region Internal Properties internal override sealed bool IsValid { get { bool valid = false; if (this.NUps.Count > 0) { valid = true; if (this.PresentationDirectionCapability != null) { valid = false; if (this.PresentationDirectionCapability.PresentationDirections.Count > 0) { valid = true; } } } return valid; } } internal abstract override string FeatureName { get; } internal override sealed bool HasSubFeature { get { return true; } } #endregion Internal Properties #region Internal Fields internal Collection_nUps; internal NUpPresentationDirectionCapability _presentationDirectionCap; #endregion Internal Fields } /// /// Represents job NUp capability. /// internal class JobNUpCapability : NUpCapability { #region Constructors internal JobNUpCapability(InternalPrintCapabilities ownerPrintCap) : base(ownerPrintCap) { } #endregion Constructors #region Internal Methods internal static PrintCapabilityFeature NewFeatureCallback(InternalPrintCapabilities printCap) { JobNUpCapability cap = new JobNUpCapability(printCap); cap._nUps = new Collection(); return cap; } #endregion Internal Methods #region Internal Properties internal sealed override string FeatureName { get { return PrintSchemaTags.Keywords.NUpKeys.JobNUp; } } #endregion Internal Properties } /// /// Represents an NUp presentation direction option. /// internal class NUpPresentationDirectionOption: PrintCapabilityOption { #region Constructors internal NUpPresentationDirectionOption(PrintCapabilityFeature ownerFeature) : base(ownerFeature) { _value = 0; } #endregion Constructors #region Public Properties ////// Gets the NUp presentation direction option's value. /// public PagesPerSheetDirection Value { get { return _value; } } #endregion Public Properties #region Public Methods ////// Converts the NUp presentation direction option to human-readable string. /// ///A string that represents this NUp presentation direction option. public override string ToString() { return Value.ToString(); } #endregion Public Methods #region Internal Fields internal PagesPerSheetDirection _value; #endregion Internal Fields } ////// Represents NUp presentation direction capability. /// internal class NUpPresentationDirectionCapability : PrintCapabilityFeature { #region Constructors internal NUpPresentationDirectionCapability(InternalPrintCapabilities ownerPrintCap) : base(ownerPrintCap) { } #endregion Constructors #region Public Properties ////// Gets the collection object that represents document NUp presentation /// direction options supported by the device. /// public CollectionPresentationDirections { get { return _presentationDirections; } } #endregion Public Properties #region Internal Methods internal static PrintCapabilityFeature NewFeatureCallback(InternalPrintCapabilities printCap) { NUpPresentationDirectionCapability cap = new NUpPresentationDirectionCapability(printCap); cap._presentationDirections = new Collection (); return cap; } internal override sealed bool AddOptionCallback(PrintCapabilityOption baseOption) { bool added = false; NUpPresentationDirectionOption option = baseOption as NUpPresentationDirectionOption; // validate the option is complete before adding it to the collection if (option._optionName != null) { int enumValue = PrintSchemaMapper.SchemaNameToEnumValueWithArray( PrintSchemaTags.Keywords.NUpKeys.DirectionNames, PrintSchemaTags.Keywords.NUpKeys.DirectionEnums, option._optionName); if (enumValue > 0) { option._value = (PagesPerSheetDirection)enumValue; this.PresentationDirections.Add(option); added = true; } } return added; } internal override sealed void AddSubFeatureCallback(PrintCapabilityFeature subFeature) { // no sub-feature return; } internal override sealed bool FeaturePropCallback(PrintCapabilityFeature feature, XmlPrintCapReader reader) { // no feature property to handle return false; } internal override sealed PrintCapabilityOption NewOptionCallback(PrintCapabilityFeature baseFeature) { NUpPresentationDirectionOption option = new NUpPresentationDirectionOption(baseFeature); return option; } internal override sealed void OptionAttrCallback(PrintCapabilityOption baseOption, XmlPrintCapReader reader) { // no option attribute to handle return; } internal override sealed bool OptionPropCallback(PrintCapabilityOption option, XmlPrintCapReader reader) { // no option property to handle return false; } #endregion Internal Methods #region Internal Properties internal override sealed bool IsValid { get { return (this.PresentationDirections.Count > 0); } } internal override sealed string FeatureName { get { return PrintSchemaTags.Keywords.NUpKeys.PresentationDirection; } } internal override sealed bool HasSubFeature { get { return false; } } #endregion Internal Properties #region Internal Fields internal Collection _presentationDirections; #endregion Internal Fields } /// /// Represents NUp setting. /// abstract internal class NUpSetting : PrintTicketFeature { #region Constructors ////// Constructs a new NUp setting object. /// internal NUpSetting(InternalPrintTicket ownerPrintTicket, string featureName) : base(ownerPrintTicket) { this._featureName = featureName; this._propertyMaps = new PTPropertyMapEntry[] { new PTPropertyMapEntry(this, PrintSchemaTags.Keywords.NUpKeys.PagesPerSheet, PTPropValueTypes.PositiveIntValue), }; } #endregion Constructors #region Public Properties ////// Gets or sets the value of number of logical pages per physical sheet. /// ////// If this setting is not specified yet, getter will return ///. /// /// The value to set is not a positive integer. /// public int PagesPerSheet { get { return this[PrintSchemaTags.Keywords.NUpKeys.PagesPerSheet]; } set { if (value <= 0) { throw new ArgumentOutOfRangeException("value", PTUtility.GetTextFromResource("ArgumentException.PositiveValue")); } this[PrintSchemaTags.Keywords.NUpKeys.PagesPerSheet] = value; } } ////// Gets a public NUpPresentationDirectionSetting PresentationDirection { get { if (_presentationDirection == null) { _presentationDirection = new NUpPresentationDirectionSetting(this._ownerPrintTicket); // This is a sub-feature so we need to set its parent feature field _presentationDirection._parentFeature = this; } return _presentationDirection; } } #endregion Public Properties #region Public Methods ///object that specifies the document NUp presentation direction setting. /// /// Converts the document NUp setting to human-readable string. /// ///A string that represents this document NUp setting. public override string ToString() { return PagesPerSheet.ToString(CultureInfo.CurrentCulture) + ", " + PresentationDirection.ToString(); } #endregion Public Methods #region Private Fields private NUpPresentationDirectionSetting _presentationDirection; #endregion Private Fields } ////// Represents job NUp setting. /// internal class JobNUpSetting : NUpSetting { #region Constructors internal JobNUpSetting(InternalPrintTicket ownerPrintTicket) : base(ownerPrintTicket, PrintSchemaTags.Keywords.NUpKeys.JobNUp) { } #endregion Constructors } ////// Represents NUp presentation direction setting. /// internal class NUpPresentationDirectionSetting : PrintTicketFeature { #region Constructors ////// Constructs a new NUp presentation direction setting object. /// internal NUpPresentationDirectionSetting(InternalPrintTicket ownerPrintTicket) : base(ownerPrintTicket) { this._featureName = PrintSchemaTags.Keywords.NUpKeys.PresentationDirection; this._propertyMaps = new PTPropertyMapEntry[] { new PTPropertyMapEntry(this, PrintSchemaTags.Framework.OptionNameProperty, PTPropValueTypes.EnumStringValue, PrintSchemaTags.Keywords.NUpKeys.DirectionNames, PrintSchemaTags.Keywords.NUpKeys.DirectionEnums), }; } #endregion Constructors #region Public Properties ////// Gets or sets the value of this NUp presentation direction setting. /// ////// If the direction setting is not specified yet, getter will return 0. /// ////// The value to set is not one of the standard public PagesPerSheetDirection Value { get { return (PagesPerSheetDirection)this[PrintSchemaTags.Framework.OptionNameProperty]; } set { if (value < PrintSchema.PagesPerSheetDirectionEnumMin || value > PrintSchema.PagesPerSheetDirectionEnumMax) { throw new ArgumentOutOfRangeException("value"); } this[PrintSchemaTags.Framework.OptionNameProperty] = (int)value; } } #endregion Public Properties #region Public Methods ///. /// /// Converts the NUp presentation direction setting to human-readable string. /// ///A string that represents this NUp presentation direction setting. public override string ToString() { return Value.ToString(); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
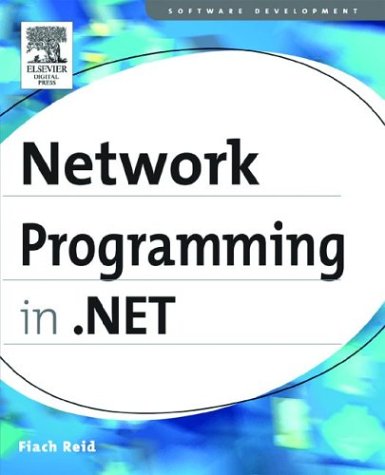
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlReader.cs
- InputReportEventArgs.cs
- DataGridViewCellValueEventArgs.cs
- shaperfactoryquerycacheentry.cs
- XamlValidatingReader.cs
- PageBreakRecord.cs
- TraceSection.cs
- MD5CryptoServiceProvider.cs
- SqlInternalConnectionTds.cs
- Property.cs
- SaveFileDialog.cs
- XmlAnyElementAttribute.cs
- RtType.cs
- SerializationBinder.cs
- UmAlQuraCalendar.cs
- TextureBrush.cs
- DocumentOrderQuery.cs
- Mutex.cs
- WindowsSspiNegotiation.cs
- DLinqColumnProvider.cs
- bindurihelper.cs
- SynchronizedCollection.cs
- LinearGradientBrush.cs
- TextFormatter.cs
- DocumentPaginator.cs
- NamespaceListProperty.cs
- CurrentChangedEventManager.cs
- ApplicationInfo.cs
- sqlpipe.cs
- COM2TypeInfoProcessor.cs
- CreateParams.cs
- ReachSerializationCacheItems.cs
- SmiConnection.cs
- CommandID.cs
- SecurityException.cs
- GridViewCancelEditEventArgs.cs
- IImplicitResourceProvider.cs
- DocobjHost.cs
- SeekStoryboard.cs
- DataTableMappingCollection.cs
- ScrollProperties.cs
- StateMachineAction.cs
- HttpCacheVaryByContentEncodings.cs
- MemoryStream.cs
- InvalidCastException.cs
- XmlSchemaSimpleContentExtension.cs
- VisualStyleInformation.cs
- StringDictionaryWithComparer.cs
- MemoryStream.cs
- InstanceBehavior.cs
- StringToken.cs
- ScaleTransform.cs
- FunctionGenerator.cs
- CustomAssemblyResolver.cs
- TypeDescriptionProvider.cs
- ResourceManagerWrapper.cs
- IisTraceListener.cs
- elementinformation.cs
- OperationCanceledException.cs
- FixedDocumentSequencePaginator.cs
- SignatureDescription.cs
- _BasicClient.cs
- ConfigUtil.cs
- RectValueSerializer.cs
- MediaPlayer.cs
- ContentElement.cs
- CodeObject.cs
- DynamicUpdateCommand.cs
- View.cs
- LoginAutoFormat.cs
- ImageListUtils.cs
- List.cs
- SqlProviderManifest.cs
- PasswordPropertyTextAttribute.cs
- Span.cs
- DataBindingHandlerAttribute.cs
- BaseAutoFormat.cs
- SqlLiftIndependentRowExpressions.cs
- ContainerParagraph.cs
- SettingsAttributes.cs
- QueryContinueDragEvent.cs
- HtmlTableCellCollection.cs
- TemplateBamlTreeBuilder.cs
- ScrollItemPatternIdentifiers.cs
- EntityModelSchemaGenerator.cs
- _OverlappedAsyncResult.cs
- NetworkStream.cs
- TreeViewImageKeyConverter.cs
- XappLauncher.cs
- FontSourceCollection.cs
- PlaceHolder.cs
- PanelContainerDesigner.cs
- regiisutil.cs
- PasswordTextNavigator.cs
- StyleHelper.cs
- XmlObjectSerializerWriteContextComplex.cs
- CalendarModeChangedEventArgs.cs
- FilterableAttribute.cs
- CompressionTransform.cs
- dsa.cs