Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / CharEnumerator.cs / 1 / CharEnumerator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: CharEnumerator ** ** ** Purpose: Enumerates the characters on a string. skips range ** checks. ** ** ============================================================*/ namespace System { using System.Collections; using System.Collections.Generic; [System.Runtime.InteropServices.ComVisible(true)] [Serializable] public sealed class CharEnumerator : IEnumerator, ICloneable, IEnumerator{ private String str; private int index; private char currentElement; internal CharEnumerator(String str) { this.str = str; this.index = -1; } public Object Clone() { return MemberwiseClone(); } public bool MoveNext() { if (index < (str.Length-1)) { index++; currentElement = str[index]; return true; } else index = str.Length; return false; } void IDisposable.Dispose() { } /// Object IEnumerator.Current { get { if (index == -1) throw new InvalidOperationException(Environment.GetResourceString(ResId.InvalidOperation_EnumNotStarted)); if (index >= str.Length) throw new InvalidOperationException(Environment.GetResourceString(ResId.InvalidOperation_EnumEnded)); return currentElement; } } public char Current { get { if (index == -1) throw new InvalidOperationException(Environment.GetResourceString(ResId.InvalidOperation_EnumNotStarted)); if (index >= str.Length) throw new InvalidOperationException(Environment.GetResourceString(ResId.InvalidOperation_EnumEnded)); return currentElement; } } public void Reset() { currentElement = (char)0; index = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: CharEnumerator ** ** ** Purpose: Enumerates the characters on a string. skips range ** checks. ** ** ============================================================*/ namespace System { using System.Collections; using System.Collections.Generic; [System.Runtime.InteropServices.ComVisible(true)] [Serializable] public sealed class CharEnumerator : IEnumerator, ICloneable, IEnumerator { private String str; private int index; private char currentElement; internal CharEnumerator(String str) { this.str = str; this.index = -1; } public Object Clone() { return MemberwiseClone(); } public bool MoveNext() { if (index < (str.Length-1)) { index++; currentElement = str[index]; return true; } else index = str.Length; return false; } void IDisposable.Dispose() { } /// Object IEnumerator.Current { get { if (index == -1) throw new InvalidOperationException(Environment.GetResourceString(ResId.InvalidOperation_EnumNotStarted)); if (index >= str.Length) throw new InvalidOperationException(Environment.GetResourceString(ResId.InvalidOperation_EnumEnded)); return currentElement; } } public char Current { get { if (index == -1) throw new InvalidOperationException(Environment.GetResourceString(ResId.InvalidOperation_EnumNotStarted)); if (index >= str.Length) throw new InvalidOperationException(Environment.GetResourceString(ResId.InvalidOperation_EnumEnded)); return currentElement; } } public void Reset() { currentElement = (char)0; index = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
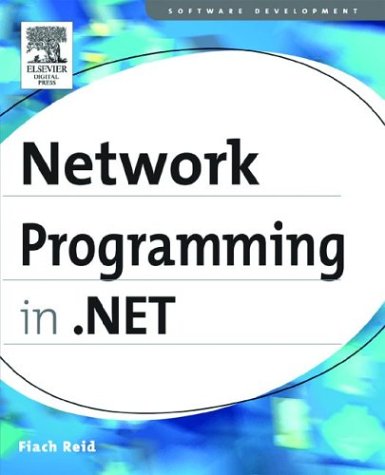
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssociatedControlConverter.cs
- AlternateView.cs
- Parameter.cs
- WindowsPrincipal.cs
- DomainConstraint.cs
- Pair.cs
- AccessViolationException.cs
- DataSet.cs
- ContextMarshalException.cs
- ContainsRowNumberChecker.cs
- BigInt.cs
- BuildManagerHost.cs
- Wizard.cs
- TextEditorLists.cs
- SelectionRangeConverter.cs
- wgx_exports.cs
- Comparer.cs
- KeyboardDevice.cs
- PropertySet.cs
- LayeredChannelListener.cs
- BehaviorEditorPart.cs
- ToolStripPanelRow.cs
- ArgumentDirectionHelper.cs
- SoundPlayer.cs
- XhtmlConformanceSection.cs
- UnsafeNativeMethods.cs
- RSACryptoServiceProvider.cs
- UnaryNode.cs
- PackageDocument.cs
- Condition.cs
- DrawItemEvent.cs
- ValidatedControlConverter.cs
- GridViewColumnHeader.cs
- DeviceContext2.cs
- FixedDocumentSequencePaginator.cs
- DataGridAutoFormat.cs
- SmiEventSink_DeferedProcessing.cs
- HeaderPanel.cs
- AutomationEventArgs.cs
- UrlEncodedParameterWriter.cs
- EditorZoneBase.cs
- mediapermission.cs
- WindowsScrollBar.cs
- ToolboxItemSnapLineBehavior.cs
- AffineTransform3D.cs
- SQLByteStorage.cs
- DataGridViewCellCollection.cs
- SoapAttributeOverrides.cs
- DeferredReference.cs
- SecureConversationSecurityTokenParameters.cs
- RawStylusInputCustomData.cs
- ShaderRenderModeValidation.cs
- XamlParser.cs
- BrowserCapabilitiesCompiler.cs
- DrawingServices.cs
- HandlerMappingMemo.cs
- BitmapEffect.cs
- FormsAuthentication.cs
- BindStream.cs
- PriorityChain.cs
- PixelFormatConverter.cs
- PackageRelationshipSelector.cs
- Schema.cs
- SQLCharsStorage.cs
- PeerEndPoint.cs
- PartBasedPackageProperties.cs
- ReadOnlyDataSourceView.cs
- ReferenceCountedObject.cs
- HostedHttpContext.cs
- CompatibleComparer.cs
- ToolStripDropDownButton.cs
- TextureBrush.cs
- SafeFileMapViewHandle.cs
- AddressingVersion.cs
- TreeView.cs
- ContractNamespaceAttribute.cs
- SBCSCodePageEncoding.cs
- CommandSet.cs
- TerminatorSinks.cs
- SecurityContext.cs
- safex509handles.cs
- SmiEventSink_DeferedProcessing.cs
- TextBoxLine.cs
- CultureSpecificStringDictionary.cs
- ChainOfResponsibility.cs
- SecurityManager.cs
- StringFreezingAttribute.cs
- IisTraceListener.cs
- IPEndPointCollection.cs
- versioninfo.cs
- columnmapfactory.cs
- InitializationEventAttribute.cs
- NaturalLanguageHyphenator.cs
- ValidatedControlConverter.cs
- TypeToken.cs
- HyperLink.cs
- TableRow.cs
- DrawingBrush.cs
- TextServicesCompartmentContext.cs
- XmlILModule.cs