Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Canvas.cs / 1305600 / Canvas.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: Canvas.cs // // Description: Contains the Canvas class. // Spec at http://avalon/layout/Specs/Canvas.xml // // History: // 06/02/2003 : greglett - Added to WCP branch (was APEContainer.cs in old branch) // //--------------------------------------------------------------------------- using MS.Internal; using MS.Utility; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using System.Windows.Media; using System; namespace System.Windows.Controls { ////// Canvas is used to place child UIElements at arbitrary positions or to draw children in multiple /// layers. /// /// Child positions are computed from the Left, Top properties. These properties do /// not contribute to the size of the Canvas. To position children in a way that affects the Canvas' size, /// use the Margin properties. /// /// The order that children are drawn (z-order) is determined exclusively by child order. /// public class Canvas : Panel { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public Canvas() : base() { } #endregion //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Reads the attached property Left from the given element. /// /// The element from which to read the Left attached property. ///The property's value. ///[TypeConverter("System.Windows.LengthConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [AttachedPropertyBrowsableForChildren()] public static double GetLeft(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(LeftProperty); } /// /// Writes the attached property Left to the given element. /// /// The element to which to write the Left attached property. /// The length to set ///public static void SetLeft(UIElement element, double length) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LeftProperty, length); } /// /// Reads the attached property Top from the given element. /// /// The element from which to read the Top attached property. ///The property's value. ///[TypeConverter("System.Windows.LengthConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [AttachedPropertyBrowsableForChildren()] public static double GetTop(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(TopProperty); } /// /// Writes the attached property Top to the given element. /// /// The element to which to write the Top attached property. /// The length to set ///public static void SetTop(UIElement element, double length) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(TopProperty, length); } /// /// Reads the attached property Right from the given element. /// /// The element from which to read the Right attached property. ///The property's Length value. ///[TypeConverter("System.Windows.LengthConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [AttachedPropertyBrowsableForChildren()] public static double GetRight(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(RightProperty); } /// /// Writes the attached property Right to the given element. /// /// The element to which to write the Right attached property. /// The Length to set ///public static void SetRight(UIElement element, double length) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(RightProperty, length); } /// /// Reads the attached property Bottom from the given element. /// /// The element from which to read the Bottom attached property. ///The property's Length value. ///[TypeConverter("System.Windows.LengthConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [AttachedPropertyBrowsableForChildren()] public static double GetBottom(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(BottomProperty); } /// /// Writes the attached property Bottom to the given element. /// /// The element to which to write the Bottom attached property. /// The Length to set ///public static void SetBottom(UIElement element, double length) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(BottomProperty, length); } #endregion //-------------------------------------------------------------------- // // Public Properties + Dependency Properties's // //-------------------------------------------------------------------- #region Public Properties //having this invalidate callback allows to host UIElements in Canvas and still //receive invalidations when Left/Top/Bottom/Right properties change - //registering the attached properties with AffectsParentArrange flag would be a mistake //because those flags only work for FrameworkElements private static void OnPositioningChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { UIElement uie = d as UIElement; if(uie != null) { Canvas p = VisualTreeHelper.GetParent(uie) as Canvas; if(p != null) p.InvalidateArrange(); } } /// /// This is the dependency property registered for the Canvas' Left attached property. /// /// The Left property is read by a Canvas on its children to determine where to position them. /// The child's offset from this property does not have an effect on the Canvas' own size. /// Conflict between the Left and Right properties is resolved in favor of Left. /// public static readonly DependencyProperty LeftProperty = DependencyProperty.RegisterAttached("Left", typeof(double), typeof(Canvas), new FrameworkPropertyMetadata(Double.NaN, new PropertyChangedCallback(OnPositioningChanged)), new ValidateValueCallback(System.Windows.Shapes.Shape.IsDoubleFiniteOrNaN)); ////// This is the dependency property registered for the Canvas' Top attached property. /// /// The Top property is read by a Canvas on its children to determine where to position them. /// The child's offset from this property does not have an effect on the Canvas' own size. /// public static readonly DependencyProperty TopProperty = DependencyProperty.RegisterAttached("Top", typeof(double), typeof(Canvas), new FrameworkPropertyMetadata(Double.NaN, new PropertyChangedCallback(OnPositioningChanged)), new ValidateValueCallback(System.Windows.Shapes.Shape.IsDoubleFiniteOrNaN)); ////// This is the dependency property registered for the Canvas' Right attached property. /// /// The Right property is read by a Canvas on its children to determine where to position them. /// The child's offset from this property does not have an effect on the Canvas' own size. /// Conflict between the Left and Right properties is resolved in favor of Left. /// public static readonly DependencyProperty RightProperty = DependencyProperty.RegisterAttached("Right", typeof(double), typeof(Canvas), new FrameworkPropertyMetadata(Double.NaN, new PropertyChangedCallback(OnPositioningChanged)), new ValidateValueCallback(System.Windows.Shapes.Shape.IsDoubleFiniteOrNaN)); ////// This is the dependency property registered for the Canvas' Bottom attached property. /// /// The Bottom property is read by a Canvas on its children to determine where to position them. /// The child's offset from this property does not have an effect on the Canvas' own size. /// public static readonly DependencyProperty BottomProperty = DependencyProperty.RegisterAttached("Bottom", typeof(double), typeof(Canvas), new FrameworkPropertyMetadata(Double.NaN, new PropertyChangedCallback(OnPositioningChanged)), new ValidateValueCallback(System.Windows.Shapes.Shape.IsDoubleFiniteOrNaN)); #endregion //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Updates DesiredSize of the Canvas. Called by parent UIElement. This is the first pass of layout. /// /// Constraint size is an "upper limit" that Canvas should not exceed. ///Canvas' desired size. protected override Size MeasureOverride(Size constraint) { Size childConstraint = new Size(Double.PositiveInfinity, Double.PositiveInfinity); foreach (UIElement child in InternalChildren) { if (child == null) { continue; } child.Measure(childConstraint); } return new Size(); } ////// Canvas computes a position for each of its children taking into account their margin and /// attached Canvas properties: Top, Left. /// /// Canvas will also arrange each of its children. /// /// Size that Canvas will assume to position children. protected override Size ArrangeOverride(Size arrangeSize) { //Canvas arranges children at their DesiredSize. //This means that Margin on children is actually respected and added //to the size of layout partition for a child. //Therefore, is Margin is 10 and Left is 20, the child's ink will start at 30. foreach (UIElement child in InternalChildren) { if (child == null) { continue; } double x = 0; double y = 0; //Compute offset of the child: //If Left is specified, then Right is ignored //If Left is not specified, then Right is used //If both are not there, then 0 double left = GetLeft(child); if(!DoubleUtil.IsNaN(left)) { x = left; } else { double right = GetRight(child); if(!DoubleUtil.IsNaN(right)) { x = arrangeSize.Width - child.DesiredSize.Width - right; } } double top = GetTop(child); if(!DoubleUtil.IsNaN(top)) { y = top; } else { double bottom = GetBottom(child); if(!DoubleUtil.IsNaN(bottom)) { y = arrangeSize.Height - child.DesiredSize.Height - bottom; } } child.Arrange(new Rect(new Point(x, y), child.DesiredSize)); } return arrangeSize; } ////// Override of ///. /// Geometry to use as additional clip if LayoutConstrained=true protected override Geometry GetLayoutClip(Size layoutSlotSize) { //Canvas only clips to bounds if ClipToBounds is set, // no automatic clipping if(ClipToBounds) return new RectangleGeometry(new Rect(RenderSize)); else return null; } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 9; } } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: Canvas.cs // // Description: Contains the Canvas class. // Spec at http://avalon/layout/Specs/Canvas.xml // // History: // 06/02/2003 : greglett - Added to WCP branch (was APEContainer.cs in old branch) // //--------------------------------------------------------------------------- using MS.Internal; using MS.Utility; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using System.Windows.Media; using System; namespace System.Windows.Controls { ////// Canvas is used to place child UIElements at arbitrary positions or to draw children in multiple /// layers. /// /// Child positions are computed from the Left, Top properties. These properties do /// not contribute to the size of the Canvas. To position children in a way that affects the Canvas' size, /// use the Margin properties. /// /// The order that children are drawn (z-order) is determined exclusively by child order. /// public class Canvas : Panel { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public Canvas() : base() { } #endregion //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Reads the attached property Left from the given element. /// /// The element from which to read the Left attached property. ///The property's value. ///[TypeConverter("System.Windows.LengthConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [AttachedPropertyBrowsableForChildren()] public static double GetLeft(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(LeftProperty); } /// /// Writes the attached property Left to the given element. /// /// The element to which to write the Left attached property. /// The length to set ///public static void SetLeft(UIElement element, double length) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LeftProperty, length); } /// /// Reads the attached property Top from the given element. /// /// The element from which to read the Top attached property. ///The property's value. ///[TypeConverter("System.Windows.LengthConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [AttachedPropertyBrowsableForChildren()] public static double GetTop(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(TopProperty); } /// /// Writes the attached property Top to the given element. /// /// The element to which to write the Top attached property. /// The length to set ///public static void SetTop(UIElement element, double length) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(TopProperty, length); } /// /// Reads the attached property Right from the given element. /// /// The element from which to read the Right attached property. ///The property's Length value. ///[TypeConverter("System.Windows.LengthConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [AttachedPropertyBrowsableForChildren()] public static double GetRight(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(RightProperty); } /// /// Writes the attached property Right to the given element. /// /// The element to which to write the Right attached property. /// The Length to set ///public static void SetRight(UIElement element, double length) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(RightProperty, length); } /// /// Reads the attached property Bottom from the given element. /// /// The element from which to read the Bottom attached property. ///The property's Length value. ///[TypeConverter("System.Windows.LengthConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [AttachedPropertyBrowsableForChildren()] public static double GetBottom(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(BottomProperty); } /// /// Writes the attached property Bottom to the given element. /// /// The element to which to write the Bottom attached property. /// The Length to set ///public static void SetBottom(UIElement element, double length) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(BottomProperty, length); } #endregion //-------------------------------------------------------------------- // // Public Properties + Dependency Properties's // //-------------------------------------------------------------------- #region Public Properties //having this invalidate callback allows to host UIElements in Canvas and still //receive invalidations when Left/Top/Bottom/Right properties change - //registering the attached properties with AffectsParentArrange flag would be a mistake //because those flags only work for FrameworkElements private static void OnPositioningChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { UIElement uie = d as UIElement; if(uie != null) { Canvas p = VisualTreeHelper.GetParent(uie) as Canvas; if(p != null) p.InvalidateArrange(); } } /// /// This is the dependency property registered for the Canvas' Left attached property. /// /// The Left property is read by a Canvas on its children to determine where to position them. /// The child's offset from this property does not have an effect on the Canvas' own size. /// Conflict between the Left and Right properties is resolved in favor of Left. /// public static readonly DependencyProperty LeftProperty = DependencyProperty.RegisterAttached("Left", typeof(double), typeof(Canvas), new FrameworkPropertyMetadata(Double.NaN, new PropertyChangedCallback(OnPositioningChanged)), new ValidateValueCallback(System.Windows.Shapes.Shape.IsDoubleFiniteOrNaN)); ////// This is the dependency property registered for the Canvas' Top attached property. /// /// The Top property is read by a Canvas on its children to determine where to position them. /// The child's offset from this property does not have an effect on the Canvas' own size. /// public static readonly DependencyProperty TopProperty = DependencyProperty.RegisterAttached("Top", typeof(double), typeof(Canvas), new FrameworkPropertyMetadata(Double.NaN, new PropertyChangedCallback(OnPositioningChanged)), new ValidateValueCallback(System.Windows.Shapes.Shape.IsDoubleFiniteOrNaN)); ////// This is the dependency property registered for the Canvas' Right attached property. /// /// The Right property is read by a Canvas on its children to determine where to position them. /// The child's offset from this property does not have an effect on the Canvas' own size. /// Conflict between the Left and Right properties is resolved in favor of Left. /// public static readonly DependencyProperty RightProperty = DependencyProperty.RegisterAttached("Right", typeof(double), typeof(Canvas), new FrameworkPropertyMetadata(Double.NaN, new PropertyChangedCallback(OnPositioningChanged)), new ValidateValueCallback(System.Windows.Shapes.Shape.IsDoubleFiniteOrNaN)); ////// This is the dependency property registered for the Canvas' Bottom attached property. /// /// The Bottom property is read by a Canvas on its children to determine where to position them. /// The child's offset from this property does not have an effect on the Canvas' own size. /// public static readonly DependencyProperty BottomProperty = DependencyProperty.RegisterAttached("Bottom", typeof(double), typeof(Canvas), new FrameworkPropertyMetadata(Double.NaN, new PropertyChangedCallback(OnPositioningChanged)), new ValidateValueCallback(System.Windows.Shapes.Shape.IsDoubleFiniteOrNaN)); #endregion //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Updates DesiredSize of the Canvas. Called by parent UIElement. This is the first pass of layout. /// /// Constraint size is an "upper limit" that Canvas should not exceed. ///Canvas' desired size. protected override Size MeasureOverride(Size constraint) { Size childConstraint = new Size(Double.PositiveInfinity, Double.PositiveInfinity); foreach (UIElement child in InternalChildren) { if (child == null) { continue; } child.Measure(childConstraint); } return new Size(); } ////// Canvas computes a position for each of its children taking into account their margin and /// attached Canvas properties: Top, Left. /// /// Canvas will also arrange each of its children. /// /// Size that Canvas will assume to position children. protected override Size ArrangeOverride(Size arrangeSize) { //Canvas arranges children at their DesiredSize. //This means that Margin on children is actually respected and added //to the size of layout partition for a child. //Therefore, is Margin is 10 and Left is 20, the child's ink will start at 30. foreach (UIElement child in InternalChildren) { if (child == null) { continue; } double x = 0; double y = 0; //Compute offset of the child: //If Left is specified, then Right is ignored //If Left is not specified, then Right is used //If both are not there, then 0 double left = GetLeft(child); if(!DoubleUtil.IsNaN(left)) { x = left; } else { double right = GetRight(child); if(!DoubleUtil.IsNaN(right)) { x = arrangeSize.Width - child.DesiredSize.Width - right; } } double top = GetTop(child); if(!DoubleUtil.IsNaN(top)) { y = top; } else { double bottom = GetBottom(child); if(!DoubleUtil.IsNaN(bottom)) { y = arrangeSize.Height - child.DesiredSize.Height - bottom; } } child.Arrange(new Rect(new Point(x, y), child.DesiredSize)); } return arrangeSize; } ////// Override of ///. /// Geometry to use as additional clip if LayoutConstrained=true protected override Geometry GetLayoutClip(Size layoutSlotSize) { //Canvas only clips to bounds if ClipToBounds is set, // no automatic clipping if(ClipToBounds) return new RectangleGeometry(new Rect(RenderSize)); else return null; } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 9; } } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
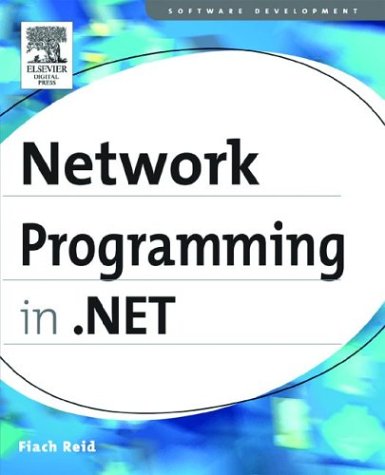
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridRowAutomationPeer.cs
- XmlNodeReader.cs
- RotateTransform.cs
- PointUtil.cs
- DataControlLinkButton.cs
- ListItemConverter.cs
- DesignerActionHeaderItem.cs
- Socket.cs
- EdmFunctionAttribute.cs
- AuthenticationModuleElementCollection.cs
- VBIdentifierName.cs
- TreeViewItem.cs
- Thread.cs
- XsltLoader.cs
- Receive.cs
- WebPartEditorOkVerb.cs
- Compensate.cs
- WebResourceAttribute.cs
- ThreadSafeList.cs
- UTF8Encoding.cs
- XamlReaderHelper.cs
- VariableReference.cs
- XpsSerializationException.cs
- CheckBoxList.cs
- Logging.cs
- XmlTypeMapping.cs
- CreateUserWizardStep.cs
- prompt.cs
- WorkflowInspectionServices.cs
- DockPattern.cs
- HwndAppCommandInputProvider.cs
- ControlEvent.cs
- _FtpDataStream.cs
- QueuePathDialog.cs
- UnsafeNativeMethods.cs
- InstancePersistenceContext.cs
- mactripleDES.cs
- Maps.cs
- HtmlTextArea.cs
- EditingCoordinator.cs
- NameValueConfigurationCollection.cs
- StatusBarPanelClickEvent.cs
- CaseStatementSlot.cs
- MouseOverProperty.cs
- ActivityBindForm.cs
- WindowsBrush.cs
- TreeViewImageKeyConverter.cs
- ObjectQueryState.cs
- WindowsSecurityToken.cs
- CodeNamespaceCollection.cs
- PageCatalogPart.cs
- DesignerTextBoxAdapter.cs
- SafeHandles.cs
- QilInvoke.cs
- ListViewTableRow.cs
- WebSysDescriptionAttribute.cs
- DbResourceAllocator.cs
- FigureParagraph.cs
- TemplateAction.cs
- KeyedHashAlgorithm.cs
- XmlSchemaSimpleContentRestriction.cs
- DataSourceCacheDurationConverter.cs
- RoutingUtilities.cs
- AuthenticationModuleElement.cs
- BaseHashHelper.cs
- XPathNavigatorKeyComparer.cs
- odbcmetadatafactory.cs
- CustomErrorCollection.cs
- ImageDrawing.cs
- QuarticEase.cs
- DataGridViewRowsAddedEventArgs.cs
- TextChange.cs
- NetWebProxyFinder.cs
- EmptyEnumerator.cs
- OdbcConnectionPoolProviderInfo.cs
- StructuralCache.cs
- CodeTypeMember.cs
- XmlSerializerAssemblyAttribute.cs
- HMACSHA256.cs
- FieldNameLookup.cs
- ToolStripContextMenu.cs
- SmtpNtlmAuthenticationModule.cs
- Int16Storage.cs
- BooleanExpr.cs
- ObjectStateEntry.cs
- CounterSample.cs
- XmlSchemaObjectCollection.cs
- QilStrConcatenator.cs
- SequenceFullException.cs
- ToolboxItemCollection.cs
- XPathNodeHelper.cs
- Throw.cs
- StringCollection.cs
- EntityDataSourceStatementEditorForm.cs
- WebReferencesBuildProvider.cs
- KnownTypes.cs
- StoreAnnotationsMap.cs
- SystemIPInterfaceProperties.cs
- ImageBrush.cs
- PersonalizationStateInfoCollection.cs