Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / Interop / SafeHandles.cs / 1305376 / SafeHandles.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging.Interop { using System; using System.Runtime.InteropServices; using Microsoft.Win32.SafeHandles; internal class MessageQueueHandle : SafeHandleZeroOrMinusOneIsInvalid { public static readonly MessageQueueHandle InvalidHandle = new InvalidMessageQueueHandle(); MessageQueueHandle() : base(true) {} protected override bool ReleaseHandle() { SafeNativeMethods.MQCloseQueue(this.handle); return true; } public override bool IsInvalid { get { return base.IsInvalid || IsClosed; } } // A subclass needed to express InvalidHandle. The reason is that CLR notices that // ReleaseHandle requires a call to MQRT.DLL, and throws in the ctor if MQRT.DLL is not available, // even though CTOR ITSELF DOES NOT REQUIRE MQRT.DLL. // We address this by defining a NOOP ReleaseHandle sealed class InvalidMessageQueueHandle : MessageQueueHandle { protected override bool ReleaseHandle() { return true; } } } internal class CursorHandle : SafeHandleZeroOrMinusOneIsInvalid { public static readonly CursorHandle NullHandle = new InvalidCursorHandle(); protected CursorHandle() : base(true) {} protected override bool ReleaseHandle() { SafeNativeMethods.MQCloseCursor(this.handle); return true; } public override bool IsInvalid { get { return base.IsInvalid || IsClosed; } } // A subclass needed to express InvalidHandle. The reason is that CLR notices that // ReleaseHandle requires a call to MQRT.DLL, and throws in the ctor if MQRT.DLL is not available, // even though CTOR ITSELF DOES NOT REQUIRE MQRT.DLL. // We address this by defining a NOOP ReleaseHandle sealed class InvalidCursorHandle : CursorHandle { protected override bool ReleaseHandle() { return true; } } } internal class LocatorHandle : SafeHandleZeroOrMinusOneIsInvalid { public static readonly LocatorHandle InvalidHandle = new InvalidLocatorHandle(); protected LocatorHandle() : base(true) {} protected override bool ReleaseHandle() { SafeNativeMethods.MQLocateEnd(this.handle); return true; } public override bool IsInvalid { get { return base.IsInvalid || IsClosed; } } // A subclass needed to express InvalidHandle. The reason is that CLR notices that // ReleaseHandle requires a call to MQRT.DLL, and throws in the ctor if MQRT.DLL is not available, // even though CTOR ITSELF DOES NOT REQUIRE MQRT.DLL. // We address this by defining a NOOP ReleaseHandle sealed class InvalidLocatorHandle : LocatorHandle { protected override bool ReleaseHandle() { return true; } } } internal sealed class SecurityContextHandle : SafeHandleZeroOrMinusOneIsInvalid { internal SecurityContextHandle(IntPtr existingHandle) : base(true) { SetHandle(existingHandle); } protected override bool ReleaseHandle() { SafeNativeMethods.MQFreeSecurityContext(this.handle); return true; } public override bool IsInvalid { get { return base.IsInvalid || IsClosed; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
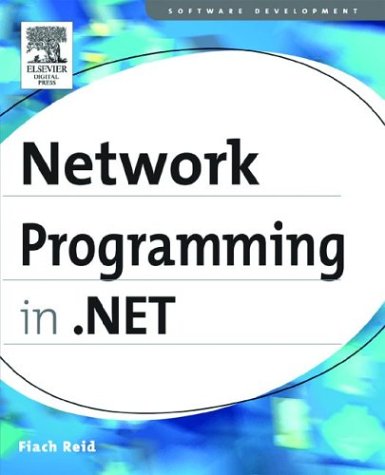
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- List.cs
- FileDetails.cs
- SqlDuplicator.cs
- EntityFunctions.cs
- InvokeHandlers.cs
- CardSpaceException.cs
- Int16Converter.cs
- BmpBitmapDecoder.cs
- Vector3DValueSerializer.cs
- TextRangeSerialization.cs
- XmlSchemaNotation.cs
- AuthenticatedStream.cs
- WebPageTraceListener.cs
- Delegate.cs
- DataGridViewCellStyleChangedEventArgs.cs
- _FtpDataStream.cs
- ToolboxItem.cs
- DefaultSection.cs
- ValueChangedEventManager.cs
- UrlAuthFailedErrorFormatter.cs
- DataGrid.cs
- ThreadStartException.cs
- AssertFilter.cs
- XmlObjectSerializer.cs
- UIElement3D.cs
- LinearGradientBrush.cs
- DataSourceProvider.cs
- ContentDisposition.cs
- ArrayList.cs
- DataGridCellItemAutomationPeer.cs
- DateTimeStorage.cs
- OutputScope.cs
- RMEnrollmentPage2.cs
- HeaderedContentControl.cs
- DoubleLinkListEnumerator.cs
- AVElementHelper.cs
- BatchParser.cs
- VideoDrawing.cs
- XmlBoundElement.cs
- EditorZone.cs
- DebugTraceHelper.cs
- bindurihelper.cs
- KeyFrames.cs
- DynamicRendererThreadManager.cs
- SystemIcmpV6Statistics.cs
- AsnEncodedData.cs
- DataGridViewCellStateChangedEventArgs.cs
- SqlServer2KCompatibilityAnnotation.cs
- XmlMapping.cs
- DynamicPropertyHolder.cs
- NetMsmqBindingCollectionElement.cs
- TextBox.cs
- AdPostCacheSubstitution.cs
- SamlAuthorizationDecisionStatement.cs
- SqlProfileProvider.cs
- SelectedDatesCollection.cs
- DashStyles.cs
- RefType.cs
- Invariant.cs
- OdbcEnvironment.cs
- Win32Exception.cs
- TextProperties.cs
- StructuredTypeEmitter.cs
- BevelBitmapEffect.cs
- BasePropertyDescriptor.cs
- FigureParagraph.cs
- ErrorFormatterPage.cs
- _PooledStream.cs
- EntityDataSourceStatementEditorForm.cs
- TTSEngineTypes.cs
- InstanceData.cs
- Privilege.cs
- BaseDataList.cs
- LinkLabel.cs
- SmiTypedGetterSetter.cs
- ExtensibleClassFactory.cs
- AncestorChangedEventArgs.cs
- DetailsViewCommandEventArgs.cs
- IPCCacheManager.cs
- TextElementEnumerator.cs
- FloaterParagraph.cs
- basenumberconverter.cs
- XPathConvert.cs
- SqlXml.cs
- ContentElementAutomationPeer.cs
- Variable.cs
- ToolStripDropDownItem.cs
- ExpressionNormalizer.cs
- XmlSchemaObject.cs
- AutoGeneratedField.cs
- BrowserInteropHelper.cs
- bindurihelper.cs
- ToolboxItem.cs
- LassoHelper.cs
- Point3DAnimationUsingKeyFrames.cs
- BoolExpressionVisitors.cs
- SmiContext.cs
- VirtualPath.cs
- DataGridViewDataConnection.cs
- TimeStampChecker.cs