Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / DataGridRowAutomationPeer.cs / 1305600 / DataGridRowAutomationPeer.cs
using System; using System.Collections.Generic; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using MS.Internal; namespace System.Windows.Automation.Peers { ////// AutomationPeer for DataGridRow /// public sealed class DataGridRowAutomationPeer : FrameworkElementAutomationPeer { #region Constructors ////// AutomationPeer for DataGridRow /// /// DataGridRow public DataGridRowAutomationPeer(DataGridRow owner) : base(owner) { if (owner == null) { throw new ArgumentNullException("owner"); } } #endregion #region AutomationPeer Overrides ////// Gets the control type for the element that is associated with the UI Automation peer. /// ///The control type. protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.DataItem; } ////// Called by GetClassName that gets a human readable name that, in addition to AutomationControlType, /// differentiates the control represented by this AutomationPeer. /// ///The string that contains the name. protected override string GetClassNameCore() { return Owner.GetType().Name; } /// protected override ListGetChildrenCore() { // see whether the DataGridRow uses the standard control template DataGridCellsPresenter cellsPresenter = OwningDataGridRow.CellsPresenter; if (cellsPresenter != null && cellsPresenter.ItemsHost != null) { // this is the normal case List children = new List (3); // Step 1: Add row header if exists AutomationPeer dataGridRowHeaderAutomationPeer = RowHeaderAutomationPeer; if (dataGridRowHeaderAutomationPeer != null) { children.Add(dataGridRowHeaderAutomationPeer); } // Step 2: Add all cells DataGridItemAutomationPeer itemPeer = this.EventsSource as DataGridItemAutomationPeer; if (itemPeer != null) { children.AddRange(itemPeer.GetCellItemPeers()); } // Step 3: Add DetailsPresenter last if exists AutomationPeer dataGridDetailsPresenterAutomationPeer = DetailsPresenterAutomationPeer; if (dataGridDetailsPresenterAutomationPeer != null) { children.Add(dataGridDetailsPresenterAutomationPeer); } return children; } else { // in the unusual case where the app uses a non-standard control template // for the DataGridRow, fall back to the base implementation return base.GetChildrenCore(); } } /// override protected bool IsOffscreenCore() { if (!Owner.IsVisible) return true; Rect boundingRect = CalculateVisibleBoundingRect(); return DoubleUtil.AreClose(boundingRect, Rect.Empty) || DoubleUtil.AreClose(boundingRect.Height, 0.0) || DoubleUtil.AreClose(boundingRect.Width, 0.0); } #endregion #region Private helpers internal AutomationPeer RowHeaderAutomationPeer { get { DataGridRowHeader dataGridRowHeader = OwningDataGridRow.RowHeader; if (dataGridRowHeader != null) { return CreatePeerForElement(dataGridRowHeader); } return null; } } private AutomationPeer DetailsPresenterAutomationPeer { get { DataGridDetailsPresenter dataGridDetailsPresenter = OwningDataGridRow.DetailsPresenter; if (dataGridDetailsPresenter != null) { return CreatePeerForElement(dataGridDetailsPresenter); } return null; } } private DataGridRow OwningDataGridRow { get { return (DataGridRow)Owner; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
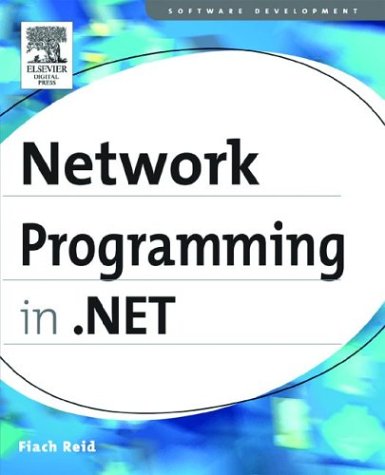
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentViewerConstants.cs
- OleDbConnectionInternal.cs
- TransformerConfigurationWizardBase.cs
- SyndicationDeserializer.cs
- RuntimeConfig.cs
- WebEventCodes.cs
- WebControlsSection.cs
- ExternalDataExchangeClient.cs
- TableSectionStyle.cs
- QuotedStringWriteStateInfo.cs
- SourceChangedEventArgs.cs
- ReaderOutput.cs
- StatusBarItemAutomationPeer.cs
- FixUp.cs
- PageSetupDialog.cs
- FontFamilyValueSerializer.cs
- NameTable.cs
- CqlBlock.cs
- ObjectSerializerFactory.cs
- SqlTypesSchemaImporter.cs
- CounterSample.cs
- InvalidFilterCriteriaException.cs
- PointLightBase.cs
- ManifestSignatureInformation.cs
- XXXOnTypeBuilderInstantiation.cs
- SHA512Managed.cs
- AuthStoreRoleProvider.cs
- TypeNameParser.cs
- XmlConverter.cs
- EntityContainerRelationshipSet.cs
- SlipBehavior.cs
- HttpWebRequest.cs
- TableLayoutPanelCellPosition.cs
- Stroke2.cs
- XPathDescendantIterator.cs
- FontFamilyValueSerializer.cs
- RepeatInfo.cs
- Facet.cs
- DbXmlEnabledProviderManifest.cs
- StructuredTypeEmitter.cs
- BitmapEffectDrawing.cs
- ManagementObject.cs
- ListBoxChrome.cs
- PersonalizablePropertyEntry.cs
- ModelTypeConverter.cs
- HighlightComponent.cs
- WinCategoryAttribute.cs
- SchemaElementLookUpTableEnumerator.cs
- ServiceParser.cs
- TextTabProperties.cs
- SafeRightsManagementHandle.cs
- AdRotator.cs
- Internal.cs
- RuntimeConfig.cs
- TextFormatterContext.cs
- ManualResetEvent.cs
- RecordsAffectedEventArgs.cs
- DifferencingCollection.cs
- WebPartCollection.cs
- AspCompat.cs
- GridItemCollection.cs
- IdentityNotMappedException.cs
- UnsafeNativeMethods.cs
- NamespaceEmitter.cs
- Calendar.cs
- ILGenerator.cs
- DashStyle.cs
- AssemblyBuilder.cs
- CharAnimationUsingKeyFrames.cs
- SchemaTableColumn.cs
- ScrollableControlDesigner.cs
- ColumnMapVisitor.cs
- DefaultPropertyAttribute.cs
- AndCondition.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- WpfWebRequestHelper.cs
- safesecurityhelperavalon.cs
- MainMenu.cs
- CssTextWriter.cs
- QueryException.cs
- WindowsContainer.cs
- ProfileGroupSettingsCollection.cs
- X509ServiceCertificateAuthenticationElement.cs
- DataSourceView.cs
- DrawingVisual.cs
- SqlVisitor.cs
- SelectorAutomationPeer.cs
- AnonymousIdentificationModule.cs
- PageAsyncTaskManager.cs
- XmlQueryRuntime.cs
- HttpRequest.cs
- DataConnectionHelper.cs
- EastAsianLunisolarCalendar.cs
- FileUtil.cs
- HyperLinkField.cs
- OdbcStatementHandle.cs
- DataGridViewUtilities.cs
- MemberAssignmentAnalysis.cs
- SecurityResources.cs
- GiveFeedbackEventArgs.cs