Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Syndication / XmlSyndicationContent.cs / 1 / XmlSyndicationContent.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Syndication { using System; using System.Text; using System.Xml; using System.Xml.Schema; using System.Runtime.Serialization; using System.Xml.Serialization; using System.Diagnostics.CodeAnalysis; using System.ServiceModel.Web; // NOTE: This class implements Clone so if you add any members, please update the copy ctor public class XmlSyndicationContent : SyndicationContent { XmlBuffer contentBuffer; SyndicationElementExtension extension; string type; // Saves the element in the reader to the buffer (attributes preserved) // Type is populated from type attribute on reader // Reader must be positioned at an element public XmlSyndicationContent(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } SyndicationFeedFormatter.MoveToStartElement(reader); if (reader.HasAttributes) { while (reader.MoveToNextAttribute()) { string name = reader.LocalName; string ns = reader.NamespaceURI; string value = reader.Value; if (name == Atom10Constants.TypeTag && ns == string.Empty) { this.type = value; } else if (!FeedUtils.IsXmlns(name, ns)) { base.AttributeExtensions.Add(new XmlQualifiedName(name, ns), value); } } reader.MoveToElement(); } this.contentBuffer = new XmlBuffer(int.MaxValue); using (XmlDictionaryWriter writer = this.contentBuffer.OpenSection(XmlDictionaryReaderQuotas.Max)) { writer.WriteNode(reader, false); } contentBuffer.CloseSection(); contentBuffer.Close(); } public XmlSyndicationContent(string type, object dataContractExtension, XmlObjectSerializer dataContractSerializer) { this.type = string.IsNullOrEmpty(type) ? Atom10Constants.XmlMediaType : type; this.extension = new SyndicationElementExtension(dataContractExtension, dataContractSerializer); } public XmlSyndicationContent(string type, object xmlSerializerExtension, XmlSerializer serializer) { this.type = string.IsNullOrEmpty(type) ? Atom10Constants.XmlMediaType : type; this.extension = new SyndicationElementExtension(xmlSerializerExtension, serializer); } public XmlSyndicationContent(string type, SyndicationElementExtension extension) { if (extension == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("extension"); } this.type = string.IsNullOrEmpty(type) ? Atom10Constants.XmlMediaType : type; this.extension = extension; } protected XmlSyndicationContent(XmlSyndicationContent source) : base(source) { if (source == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("source"); } this.contentBuffer = source.contentBuffer; this.extension = source.extension; this.type = source.type; } public SyndicationElementExtension Extension { get { return this.extension; } } public override string Type { get { return this.type; } } public override SyndicationContent Clone() { return new XmlSyndicationContent(this); } public XmlDictionaryReader GetReaderAtContent() { EnsureContentBuffer(); return this.contentBuffer.GetReader(0); } public TContent ReadContent() { return ReadContent ((DataContractSerializer) null); } public TContent ReadContent (XmlObjectSerializer dataContractSerializer) { if (dataContractSerializer == null) { dataContractSerializer = new DataContractSerializer(typeof(TContent)); } if (this.extension != null) { return this.extension.GetObject (dataContractSerializer); } else { Fx.Assert(this.contentBuffer != null, "contentBuffer cannot be null"); using (XmlDictionaryReader reader = this.contentBuffer.GetReader(0)) { // skip past the content element reader.ReadStartElement(); return (TContent) dataContractSerializer.ReadObject(reader, false); } } } public TContent ReadContent (XmlSerializer serializer) { if (serializer == null) { serializer = new XmlSerializer(typeof(TContent)); } if (this.extension != null) { return this.extension.GetObject (serializer); } else { Fx.Assert(this.contentBuffer != null, "contentBuffer cannot be null"); using (XmlDictionaryReader reader = this.contentBuffer.GetReader(0)) { // skip past the content element reader.ReadStartElement(); return (TContent) serializer.Deserialize(reader); } } } // does not write start element or type attribute, writes other attributes and rest of content protected override void WriteContentsTo(XmlWriter writer) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (this.extension != null) { this.extension.WriteTo(writer); } else if (this.contentBuffer != null) { using (XmlDictionaryReader reader = this.contentBuffer.GetReader(0)) { reader.MoveToStartElement(); if (!reader.IsEmptyElement) { reader.ReadStartElement(); while (reader.Depth >= 1 && reader.ReadState == ReadState.Interactive) { writer.WriteNode(reader, false); } } } } } void EnsureContentBuffer() { if (this.contentBuffer == null) { XmlBuffer tmp = new XmlBuffer(int.MaxValue); using (XmlDictionaryWriter writer = tmp.OpenSection(XmlDictionaryReaderQuotas.Max)) { this.WriteTo(writer, Atom10Constants.ContentTag, Atom10Constants.Atom10Namespace); } tmp.CloseSection(); tmp.Close(); this.contentBuffer = tmp; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
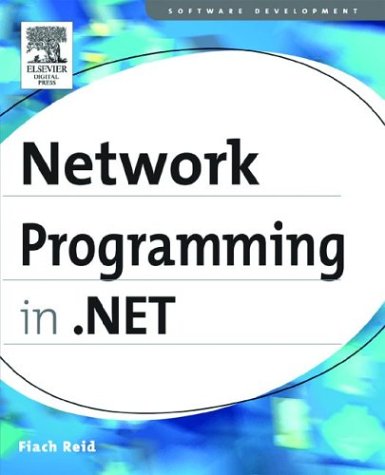
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpChannelHelpers.cs
- ColorPalette.cs
- BinaryCommonClasses.cs
- ReachDocumentSequenceSerializerAsync.cs
- FixedTextContainer.cs
- SqlTrackingService.cs
- DataObjectPastingEventArgs.cs
- DataGridViewRowCancelEventArgs.cs
- CodePageEncoding.cs
- FrameworkRichTextComposition.cs
- TemplateBindingExtensionConverter.cs
- SmtpNtlmAuthenticationModule.cs
- PageThemeBuildProvider.cs
- FontStyle.cs
- HostSecurityManager.cs
- ImportedNamespaceContextItem.cs
- Size.cs
- DbConnectionPoolGroup.cs
- CapabilitiesAssignment.cs
- AssemblyAssociatedContentFileAttribute.cs
- NoneExcludedImageIndexConverter.cs
- DataGridViewTopLeftHeaderCell.cs
- WebPartsSection.cs
- WindowsRichEdit.cs
- UnsafeMethods.cs
- Wizard.cs
- PortCache.cs
- DictionarySurrogate.cs
- BezierSegment.cs
- StorageModelBuildProvider.cs
- AnonymousIdentificationModule.cs
- LinkClickEvent.cs
- CompilationUtil.cs
- Classification.cs
- ToolStripControlHost.cs
- ToolTipAutomationPeer.cs
- InstanceKeyNotReadyException.cs
- OleDbMetaDataFactory.cs
- SQLChars.cs
- Queue.cs
- sortedlist.cs
- OLEDB_Enum.cs
- UpDownBase.cs
- TypeToken.cs
- CharacterShapingProperties.cs
- MarshalByRefObject.cs
- ComAdminWrapper.cs
- filewebrequest.cs
- PropertyCollection.cs
- Int32RectValueSerializer.cs
- BasicHttpSecurityMode.cs
- ExtendedProtectionPolicy.cs
- XXXOnTypeBuilderInstantiation.cs
- XmlElementElementCollection.cs
- HostedNamedPipeTransportManager.cs
- ReservationCollection.cs
- NativeObjectSecurity.cs
- XmlDictionary.cs
- XmlAtomicValue.cs
- HttpException.cs
- WebBrowserDocumentCompletedEventHandler.cs
- _NativeSSPI.cs
- BinHexEncoder.cs
- DragEvent.cs
- ExpandedProjectionNode.cs
- HashMembershipCondition.cs
- DocComment.cs
- TableAutomationPeer.cs
- BooleanFunctions.cs
- EmptyEnumerable.cs
- DataBinding.cs
- InkCanvasFeedbackAdorner.cs
- ComponentEditorForm.cs
- ColorMap.cs
- DataBindingValueUIHandler.cs
- MsmqException.cs
- CalendarKeyboardHelper.cs
- CqlParserHelpers.cs
- SchemaImporter.cs
- CheckableControlBaseAdapter.cs
- TraceSource.cs
- XmlILStorageConverter.cs
- RectValueSerializer.cs
- ThousandthOfEmRealDoubles.cs
- RowsCopiedEventArgs.cs
- FilteredAttributeCollection.cs
- GenericAuthenticationEventArgs.cs
- TableStyle.cs
- JsonReader.cs
- BinaryParser.cs
- ListViewGroup.cs
- AddValidationError.cs
- AstTree.cs
- PathTooLongException.cs
- ListDictionaryInternal.cs
- EventRoute.cs
- EntityWrapperFactory.cs
- UserCancellationException.cs
- XmlQuerySequence.cs
- oledbmetadatacolumnnames.cs