Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / WebControls / TableRowCollection.cs / 1 / TableRowCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ Editor("System.Web.UI.Design.WebControls.TableRowsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TableRowCollection : IList { ///Encapsulates the collection of ///objects within a control. /// A protected field of type private Table owner; ///. Represents the collection internally. /// /// internal TableRowCollection(Table owner) { this.owner = owner; } ////// Gets the /// count of public int Count { get { if (owner.HasControls()) { return owner.Controls.Count; } return 0; } } ///in the collection. /// /// public TableRow this[int index] { get { return(TableRow)owner.Controls[index]; } } ////// Gets a ///referenced by the /// specified ordinal index value. /// /// public int Add(TableRow row) { AddAt(-1, row); return owner.Controls.Count - 1; } ////// Adds the specified ///to the end of the collection. /// /// public void AddAt(int index, TableRow row) { owner.Controls.AddAt(index, row); if (row.TableSection != TableRowSection.TableBody) { owner.HasRowSections = true; } } ////// Adds the specified ///to the collection at the specified /// index location. /// /// public void AddRange(TableRow[] rows) { if (rows == null) { throw new ArgumentNullException("rows"); } foreach(TableRow row in rows) { Add(row); } } ////// public void Clear() { if (owner.HasControls()) { owner.Controls.Clear(); owner.HasRowSections = false; } } ///Removes all ///controls from the collection. /// public int GetRowIndex(TableRow row) { if (owner.HasControls()) { return owner.Controls.IndexOf(row); } return -1; } ///Returns an ordinal index value that denotes the position of the specified /// ///within the collection. /// public IEnumerator GetEnumerator() { return owner.Controls.GetEnumerator(); } ////// Returns an enumerator of all ///controls within the /// collection. /// /// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies contents from the collection to the specified ///with the /// specified starting index. /// public Object SyncRoot { get { return this;} } ////// Gets the object that can be used to synchronize access to the collection. In /// this case, it is the collection itself. /// ////// public bool IsReadOnly { get { return false;} } ////// Gets a value indicating whether the collection is read-only. /// ////// public bool IsSynchronized { get { return false;} } ////// Gets a value indicating whether access to the collection is synchronized /// (thread-safe). /// ////// public void Remove(TableRow row) { owner.Controls.Remove(row); } ///Removes the specified ///from the collection. /// public void RemoveAt(int index) { owner.Controls.RemoveAt(index); } // IList implementation, required by collection editor ///Removes the ///from the collection at the specified /// index location. object IList.this[int index] { get { return owner.Controls[index]; } set { RemoveAt(index); AddAt(index, (TableRow)value); } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object o) { return Add((TableRow) o); } /// bool IList.Contains(object o) { return owner.Controls.Contains((TableRow)o); } /// int IList.IndexOf(object o) { return owner.Controls.IndexOf((TableRow)o); } /// void IList.Insert(int index, object o) { AddAt(index, (TableRow)o); } /// void IList.Remove(object o) { Remove((TableRow)o); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ Editor("System.Web.UI.Design.WebControls.TableRowsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TableRowCollection : IList { ///Encapsulates the collection of ///objects within a control. /// A protected field of type private Table owner; ///. Represents the collection internally. /// /// internal TableRowCollection(Table owner) { this.owner = owner; } ////// Gets the /// count of public int Count { get { if (owner.HasControls()) { return owner.Controls.Count; } return 0; } } ///in the collection. /// /// public TableRow this[int index] { get { return(TableRow)owner.Controls[index]; } } ////// Gets a ///referenced by the /// specified ordinal index value. /// /// public int Add(TableRow row) { AddAt(-1, row); return owner.Controls.Count - 1; } ////// Adds the specified ///to the end of the collection. /// /// public void AddAt(int index, TableRow row) { owner.Controls.AddAt(index, row); if (row.TableSection != TableRowSection.TableBody) { owner.HasRowSections = true; } } ////// Adds the specified ///to the collection at the specified /// index location. /// /// public void AddRange(TableRow[] rows) { if (rows == null) { throw new ArgumentNullException("rows"); } foreach(TableRow row in rows) { Add(row); } } ////// public void Clear() { if (owner.HasControls()) { owner.Controls.Clear(); owner.HasRowSections = false; } } ///Removes all ///controls from the collection. /// public int GetRowIndex(TableRow row) { if (owner.HasControls()) { return owner.Controls.IndexOf(row); } return -1; } ///Returns an ordinal index value that denotes the position of the specified /// ///within the collection. /// public IEnumerator GetEnumerator() { return owner.Controls.GetEnumerator(); } ////// Returns an enumerator of all ///controls within the /// collection. /// /// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies contents from the collection to the specified ///with the /// specified starting index. /// public Object SyncRoot { get { return this;} } ////// Gets the object that can be used to synchronize access to the collection. In /// this case, it is the collection itself. /// ////// public bool IsReadOnly { get { return false;} } ////// Gets a value indicating whether the collection is read-only. /// ////// public bool IsSynchronized { get { return false;} } ////// Gets a value indicating whether access to the collection is synchronized /// (thread-safe). /// ////// public void Remove(TableRow row) { owner.Controls.Remove(row); } ///Removes the specified ///from the collection. /// public void RemoveAt(int index) { owner.Controls.RemoveAt(index); } // IList implementation, required by collection editor ///Removes the ///from the collection at the specified /// index location. object IList.this[int index] { get { return owner.Controls[index]; } set { RemoveAt(index); AddAt(index, (TableRow)value); } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object o) { return Add((TableRow) o); } /// bool IList.Contains(object o) { return owner.Controls.Contains((TableRow)o); } /// int IList.IndexOf(object o) { return owner.Controls.IndexOf((TableRow)o); } /// void IList.Insert(int index, object o) { AddAt(index, (TableRow)o); } /// void IList.Remove(object o) { Remove((TableRow)o); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
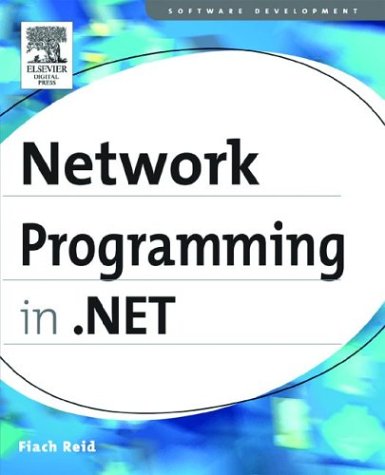
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _NestedMultipleAsyncResult.cs
- AddressUtility.cs
- ContractCodeDomInfo.cs
- WorkflowTransactionService.cs
- InternalConfigSettingsFactory.cs
- Console.cs
- BamlLocalizabilityResolver.cs
- PersonalizationStateQuery.cs
- PartBasedPackageProperties.cs
- BaseTemplateCodeDomTreeGenerator.cs
- Duration.cs
- WinFormsSecurity.cs
- PLINQETWProvider.cs
- ToolBarTray.cs
- PersonalizationDictionary.cs
- WriteFileContext.cs
- PermissionRequestEvidence.cs
- SrgsElementList.cs
- Accessors.cs
- SessionStateSection.cs
- Menu.cs
- SecurityPolicySection.cs
- ReturnValue.cs
- ToolStripSeparatorRenderEventArgs.cs
- DbConnectionStringBuilder.cs
- DelegateCompletionCallbackWrapper.cs
- TextLineResult.cs
- X509RawDataKeyIdentifierClause.cs
- PrintControllerWithStatusDialog.cs
- TypeSystem.cs
- Exceptions.cs
- StrokeCollection.cs
- WindowsPen.cs
- TextParagraphProperties.cs
- BoundPropertyEntry.cs
- HttpException.cs
- ConnectionConsumerAttribute.cs
- ClientTargetCollection.cs
- IncrementalCompileAnalyzer.cs
- WindowsIdentity.cs
- SchemaInfo.cs
- MultipartContentParser.cs
- StorageMappingItemCollection.cs
- RecognizedPhrase.cs
- BindingsCollection.cs
- SqlProvider.cs
- TypedColumnHandler.cs
- QilCloneVisitor.cs
- FlowDocumentReader.cs
- SafeEventLogReadHandle.cs
- PersonalizationProviderCollection.cs
- FacetDescription.cs
- TraceContext.cs
- AudioStateChangedEventArgs.cs
- ObjectStorage.cs
- MenuItem.cs
- TdsParserSafeHandles.cs
- ReadOnlyDictionary.cs
- Rect.cs
- TextBox.cs
- TextRange.cs
- FormViewDeleteEventArgs.cs
- SqlRewriteScalarSubqueries.cs
- DataServiceException.cs
- StickyNoteContentControl.cs
- MouseOverProperty.cs
- WindowsStatic.cs
- ObjectAnimationUsingKeyFrames.cs
- smtpconnection.cs
- XhtmlTextWriter.cs
- PropertyTabChangedEvent.cs
- InputLanguage.cs
- DataGridHelper.cs
- ConfigurationException.cs
- TextElementEditingBehaviorAttribute.cs
- MemberDomainMap.cs
- KeyTime.cs
- _FixedSizeReader.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
- SecureStringHasher.cs
- RegexRunner.cs
- BooleanSwitch.cs
- UserControlAutomationPeer.cs
- BitmapImage.cs
- InvalidDataContractException.cs
- RemoteWebConfigurationHostStream.cs
- PathSegmentCollection.cs
- ValidationRule.cs
- SqlEnums.cs
- FontFaceLayoutInfo.cs
- ServiceOperationDetailViewControl.cs
- AndAlso.cs
- EdmToObjectNamespaceMap.cs
- Cell.cs
- FaultDescription.cs
- ReflectionServiceProvider.cs
- CompositeFontFamily.cs
- DetailsView.cs
- ChooseAction.cs
- ChangeToolStripParentVerb.cs