Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Security / CookieProtection.cs / 1 / CookieProtection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Security.Cryptography; using System.Web.Configuration; using System.Web.Management; public enum CookieProtection { None, Validation, Encryption, All } internal class CookieProtectionHelper { internal static string Encode (CookieProtection cookieProtection, byte [] buf, int count) { if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { byte[] bMac = MachineKeySection.HashData (buf, null, 0, count); if (bMac == null || bMac.Length != 20) return null; if (buf.Length >= count + 20) { Buffer.BlockCopy (bMac, 0, buf, count, 20); } else { byte[] bTemp = buf; buf = new byte[count + 20]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); Buffer.BlockCopy (bMac, 0, buf, count, 20); } count += 20; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (true, buf, null, 0, count); count = buf.Length; } if (count < buf.Length) { byte[] bTemp = buf; buf = new byte[count]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); } return HttpServerUtility.UrlTokenEncode(buf); } internal static byte[] Decode (CookieProtection cookieProtection, string data) { byte [] buf = HttpServerUtility.UrlTokenDecode(data); if (buf == null || cookieProtection == CookieProtection.None) return buf; if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (false, buf, null, 0, buf.Length); if (buf == null) return null; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { ////////////////////////////////////////////////////////////////////// // Step 2: Get the MAC: Last 20 bytes if (buf.Length <= 20) return null; byte[] buf2 = new byte[buf.Length - 20]; Buffer.BlockCopy (buf, 0, buf2, 0, buf2.Length); byte[] bMac = MachineKeySection.HashData (buf2, null, 0, buf2.Length); ////////////////////////////////////////////////////////////////////// // Step 3: Make sure the MAC is correct if (bMac == null || bMac.Length != 20) return null; for (int iter = 0; iter < 20; iter++) if (bMac[iter] != buf[buf2.Length + iter]) return null; buf = buf2; } return buf; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Security.Cryptography; using System.Web.Configuration; using System.Web.Management; public enum CookieProtection { None, Validation, Encryption, All } internal class CookieProtectionHelper { internal static string Encode (CookieProtection cookieProtection, byte [] buf, int count) { if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { byte[] bMac = MachineKeySection.HashData (buf, null, 0, count); if (bMac == null || bMac.Length != 20) return null; if (buf.Length >= count + 20) { Buffer.BlockCopy (bMac, 0, buf, count, 20); } else { byte[] bTemp = buf; buf = new byte[count + 20]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); Buffer.BlockCopy (bMac, 0, buf, count, 20); } count += 20; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (true, buf, null, 0, count); count = buf.Length; } if (count < buf.Length) { byte[] bTemp = buf; buf = new byte[count]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); } return HttpServerUtility.UrlTokenEncode(buf); } internal static byte[] Decode (CookieProtection cookieProtection, string data) { byte [] buf = HttpServerUtility.UrlTokenDecode(data); if (buf == null || cookieProtection == CookieProtection.None) return buf; if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (false, buf, null, 0, buf.Length); if (buf == null) return null; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { ////////////////////////////////////////////////////////////////////// // Step 2: Get the MAC: Last 20 bytes if (buf.Length <= 20) return null; byte[] buf2 = new byte[buf.Length - 20]; Buffer.BlockCopy (buf, 0, buf2, 0, buf2.Length); byte[] bMac = MachineKeySection.HashData (buf2, null, 0, buf2.Length); ////////////////////////////////////////////////////////////////////// // Step 3: Make sure the MAC is correct if (bMac == null || bMac.Length != 20) return null; for (int iter = 0; iter < 20; iter++) if (bMac[iter] != buf[buf2.Length + iter]) return null; buf = buf2; } return buf; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
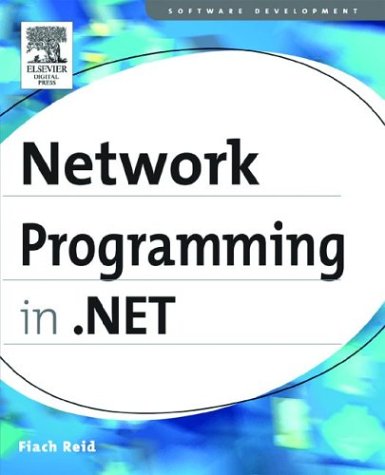
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SimpleWebHandlerParser.cs
- WebPartMenu.cs
- CanExecuteRoutedEventArgs.cs
- CqlParser.cs
- BrowserDefinition.cs
- UIElement.cs
- DependencyObjectType.cs
- StorageMappingItemLoader.cs
- FSWPathEditor.cs
- HtmlEncodedRawTextWriter.cs
- TrimSurroundingWhitespaceAttribute.cs
- HostedAspNetEnvironment.cs
- UIElementCollection.cs
- TextPointer.cs
- Byte.cs
- ObjectQuery_EntitySqlExtensions.cs
- MatrixCamera.cs
- SelectionListComponentEditor.cs
- NCryptSafeHandles.cs
- RSACryptoServiceProvider.cs
- Quaternion.cs
- Size3DConverter.cs
- FixedBufferAttribute.cs
- PriorityQueue.cs
- GridViewCancelEditEventArgs.cs
- PeerContact.cs
- WebResponse.cs
- ReliableInputConnection.cs
- Label.cs
- CompareValidator.cs
- WorkflowHostingResponseContext.cs
- LineMetrics.cs
- RuleElement.cs
- PolyBezierSegmentFigureLogic.cs
- Models.cs
- LookupBindingPropertiesAttribute.cs
- HebrewCalendar.cs
- CacheRequest.cs
- ObjectConverter.cs
- LogRestartAreaEnumerator.cs
- AffineTransform3D.cs
- SQLDateTime.cs
- TextDocumentView.cs
- MediaContextNotificationWindow.cs
- IgnoreSection.cs
- ConsoleCancelEventArgs.cs
- WindowsRichEditRange.cs
- ServiceContractViewControl.cs
- RemotingServices.cs
- DbConnectionInternal.cs
- FileStream.cs
- LayoutManager.cs
- ControlPager.cs
- OSFeature.cs
- CommandHelper.cs
- Lease.cs
- Pkcs7Signer.cs
- InvalidateEvent.cs
- BitVector32.cs
- ColumnMapCopier.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- HttpContext.cs
- MemberInfoSerializationHolder.cs
- ButtonBaseDesigner.cs
- IRCollection.cs
- DocumentViewerBase.cs
- Composition.cs
- SqlServer2KCompatibilityAnnotation.cs
- ScriptReferenceBase.cs
- XmlSchemaProviderAttribute.cs
- SqlClientWrapperSmiStream.cs
- DbConnectionHelper.cs
- PerformanceCounter.cs
- CodeLinePragma.cs
- XmlSerializableServices.cs
- IntSecurity.cs
- SubMenuStyle.cs
- CodeTypeReference.cs
- OdbcParameter.cs
- DiscreteKeyFrames.cs
- SessionStateModule.cs
- UnknownBitmapEncoder.cs
- ObjectDataSourceView.cs
- XmlAttributeProperties.cs
- MissingManifestResourceException.cs
- InfoCardProofToken.cs
- ExpressionBindings.cs
- ButtonField.cs
- DupHandleConnectionReader.cs
- IisTraceWebEventProvider.cs
- BamlLocalizationDictionary.cs
- Int16.cs
- CodeParameterDeclarationExpression.cs
- CustomCategoryAttribute.cs
- TimerTable.cs
- BookmarkCallbackWrapper.cs
- XmlLanguageConverter.cs
- StringSorter.cs
- BindingMemberInfo.cs
- DbConnectionPoolIdentity.cs