Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Xml / System / Xml / schema / XmlSchemaParticle.cs / 1 / XmlSchemaParticle.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Xml.Serialization; ////// /// public abstract class XmlSchemaParticle : XmlSchemaAnnotated { [Flags] enum Occurs { None, Min, Max }; decimal minOccurs = decimal.One; decimal maxOccurs = decimal.One; Occurs flags = Occurs.None; ///[To be supplied.] ////// /// [XmlAttribute("minOccurs")] public string MinOccursString { get { return (flags & Occurs.Min) == 0 ? null : XmlConvert.ToString(minOccurs); } set { if (value == null) { minOccurs = decimal.One; flags &= ~Occurs.Min; } else { minOccurs = XmlConvert.ToInteger(value); if (minOccurs < decimal.Zero) { throw new XmlSchemaException(Res.Sch_MinOccursInvalidXsd, string.Empty); } flags |= Occurs.Min; } } } ///[To be supplied.] ////// /// [XmlAttribute("maxOccurs")] public string MaxOccursString { get { return (flags & Occurs.Max) == 0 ? null : (maxOccurs == decimal.MaxValue) ? "unbounded" : XmlConvert.ToString(maxOccurs); } set { if (value == null) { maxOccurs = decimal.One; flags &= ~Occurs.Max; } else { if (value == "unbounded") { maxOccurs = decimal.MaxValue; } else { maxOccurs = XmlConvert.ToInteger(value); if (maxOccurs < decimal.Zero) { throw new XmlSchemaException(Res.Sch_MaxOccursInvalidXsd, string.Empty); } else if (maxOccurs == decimal.Zero && (flags & Occurs.Min) == 0) { minOccurs = decimal.Zero; } } flags |= Occurs.Max; } } } ///[To be supplied.] ////// /// [XmlIgnore] public decimal MinOccurs { get { return minOccurs; } set { if (value < decimal.Zero || value != decimal.Truncate(value)) { throw new XmlSchemaException(Res.Sch_MinOccursInvalidXsd, string.Empty); } minOccurs = value; flags |= Occurs.Min; } } ///[To be supplied.] ////// /// [XmlIgnore] public decimal MaxOccurs { get { return maxOccurs; } set { if (value < decimal.Zero || value != decimal.Truncate(value)) { throw new XmlSchemaException(Res.Sch_MaxOccursInvalidXsd, string.Empty); } maxOccurs = value; if (maxOccurs == decimal.Zero && (flags & Occurs.Min) == 0) { minOccurs = decimal.Zero; } flags |= Occurs.Max; } } internal virtual bool IsEmpty { get { return maxOccurs == decimal.Zero; } } internal bool IsMultipleOccurrence { get { return maxOccurs > decimal.One; } } internal virtual string NameString { get { return string.Empty; } } internal XmlQualifiedName GetQualifiedName() { XmlSchemaElement elem = this as XmlSchemaElement; if (elem != null) { return elem.QualifiedName; } else { XmlSchemaAny any = this as XmlSchemaAny; if (any != null) { string ns = any.Namespace; if (ns != null) { ns = ns.Trim(); } else { ns = string.Empty; } return new XmlQualifiedName("*", ns.Length == 0 ? "##any" : ns); } } return XmlQualifiedName.Empty; //If ever called on other particles } class EmptyParticle : XmlSchemaParticle { internal override bool IsEmpty { get { return true; } } } internal static readonly XmlSchemaParticle Empty = new EmptyParticle(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Xml.Serialization; ////// /// public abstract class XmlSchemaParticle : XmlSchemaAnnotated { [Flags] enum Occurs { None, Min, Max }; decimal minOccurs = decimal.One; decimal maxOccurs = decimal.One; Occurs flags = Occurs.None; ///[To be supplied.] ////// /// [XmlAttribute("minOccurs")] public string MinOccursString { get { return (flags & Occurs.Min) == 0 ? null : XmlConvert.ToString(minOccurs); } set { if (value == null) { minOccurs = decimal.One; flags &= ~Occurs.Min; } else { minOccurs = XmlConvert.ToInteger(value); if (minOccurs < decimal.Zero) { throw new XmlSchemaException(Res.Sch_MinOccursInvalidXsd, string.Empty); } flags |= Occurs.Min; } } } ///[To be supplied.] ////// /// [XmlAttribute("maxOccurs")] public string MaxOccursString { get { return (flags & Occurs.Max) == 0 ? null : (maxOccurs == decimal.MaxValue) ? "unbounded" : XmlConvert.ToString(maxOccurs); } set { if (value == null) { maxOccurs = decimal.One; flags &= ~Occurs.Max; } else { if (value == "unbounded") { maxOccurs = decimal.MaxValue; } else { maxOccurs = XmlConvert.ToInteger(value); if (maxOccurs < decimal.Zero) { throw new XmlSchemaException(Res.Sch_MaxOccursInvalidXsd, string.Empty); } else if (maxOccurs == decimal.Zero && (flags & Occurs.Min) == 0) { minOccurs = decimal.Zero; } } flags |= Occurs.Max; } } } ///[To be supplied.] ////// /// [XmlIgnore] public decimal MinOccurs { get { return minOccurs; } set { if (value < decimal.Zero || value != decimal.Truncate(value)) { throw new XmlSchemaException(Res.Sch_MinOccursInvalidXsd, string.Empty); } minOccurs = value; flags |= Occurs.Min; } } ///[To be supplied.] ////// /// [XmlIgnore] public decimal MaxOccurs { get { return maxOccurs; } set { if (value < decimal.Zero || value != decimal.Truncate(value)) { throw new XmlSchemaException(Res.Sch_MaxOccursInvalidXsd, string.Empty); } maxOccurs = value; if (maxOccurs == decimal.Zero && (flags & Occurs.Min) == 0) { minOccurs = decimal.Zero; } flags |= Occurs.Max; } } internal virtual bool IsEmpty { get { return maxOccurs == decimal.Zero; } } internal bool IsMultipleOccurrence { get { return maxOccurs > decimal.One; } } internal virtual string NameString { get { return string.Empty; } } internal XmlQualifiedName GetQualifiedName() { XmlSchemaElement elem = this as XmlSchemaElement; if (elem != null) { return elem.QualifiedName; } else { XmlSchemaAny any = this as XmlSchemaAny; if (any != null) { string ns = any.Namespace; if (ns != null) { ns = ns.Trim(); } else { ns = string.Empty; } return new XmlQualifiedName("*", ns.Length == 0 ? "##any" : ns); } } return XmlQualifiedName.Empty; //If ever called on other particles } class EmptyParticle : XmlSchemaParticle { internal override bool IsEmpty { get { return true; } } } internal static readonly XmlSchemaParticle Empty = new EmptyParticle(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
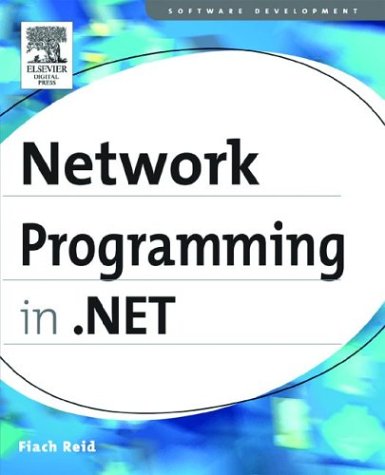
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParallelForEach.cs
- AttributeCollection.cs
- IPGlobalProperties.cs
- GridView.cs
- SapiAttributeParser.cs
- HostingEnvironment.cs
- SqlDependency.cs
- TreeViewEvent.cs
- Icon.cs
- OraclePermissionAttribute.cs
- WithStatement.cs
- SetterTriggerConditionValueConverter.cs
- DataGridItemEventArgs.cs
- HebrewNumber.cs
- EventProperty.cs
- TextChangedEventArgs.cs
- CannotUnloadAppDomainException.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- RightsManagementPermission.cs
- SubMenuStyle.cs
- ScriptResourceHandler.cs
- CalendarDesigner.cs
- HttpModulesSection.cs
- TypographyProperties.cs
- VirtualDirectoryMapping.cs
- ToolStrip.cs
- XmlReflectionMember.cs
- QuestionEventArgs.cs
- CodeFieldReferenceExpression.cs
- AdapterDictionary.cs
- SerializationHelper.cs
- IssuanceLicense.cs
- TextWriter.cs
- TrailingSpaceComparer.cs
- UIPermission.cs
- PropertyChangedEventArgs.cs
- PreviewPrintController.cs
- Stack.cs
- XmlBinaryReader.cs
- XMLSyntaxException.cs
- RangeContentEnumerator.cs
- LineServicesRun.cs
- ResourceExpressionBuilder.cs
- SetterBase.cs
- BaseCodePageEncoding.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- FatalException.cs
- DataKey.cs
- ClientApiGenerator.cs
- BindingList.cs
- DrawingAttributes.cs
- RadialGradientBrush.cs
- _NTAuthentication.cs
- StylusPlugInCollection.cs
- KnownBoxes.cs
- _CookieModule.cs
- ServiceModelEnumValidatorAttribute.cs
- DataGridViewControlCollection.cs
- DependencyObject.cs
- TableCellCollection.cs
- HScrollBar.cs
- OleDbPermission.cs
- GlyphRun.cs
- ProxyAttribute.cs
- ToolStripItemGlyph.cs
- KeyConverter.cs
- CustomAssemblyResolver.cs
- DoubleAnimationBase.cs
- FilteredDataSetHelper.cs
- ZoomComboBox.cs
- UnmanagedMemoryStreamWrapper.cs
- LinkLabel.cs
- _WebProxyDataBuilder.cs
- SplayTreeNode.cs
- CommandValueSerializer.cs
- UnicastIPAddressInformationCollection.cs
- SymbolEqualComparer.cs
- QilPatternFactory.cs
- SystemTcpStatistics.cs
- DataObjectCopyingEventArgs.cs
- EncoderFallback.cs
- CheckBox.cs
- MapPathBasedVirtualPathProvider.cs
- ComboBox.cs
- ResourceAssociationType.cs
- TextWriter.cs
- WebServiceReceive.cs
- PropagatorResult.cs
- DataBinder.cs
- ObjectReaderCompiler.cs
- ElementNotAvailableException.cs
- KeyboardDevice.cs
- XmlSchemaGroupRef.cs
- TabItemAutomationPeer.cs
- SystemInformation.cs
- InternalMappingException.cs
- ToolZone.cs
- Marshal.cs
- PasswordPropertyTextAttribute.cs
- HtmlToClrEventProxy.cs