Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripContainer.cs / 1 / ToolStripContainer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /// this is the UBER container for ToolStripPanels. namespace System.Windows.Forms { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; using System.Windows.Forms; using System.Collections; using System.Globalization; using System.Windows.Forms.Layout; [ComVisible(true)] [ClassInterface(ClassInterfaceType.AutoDispatch)] [Designer("System.Windows.Forms.Design.ToolStripContainerDesigner, " + AssemblyRef.SystemDesign)] [SRDescription(SR.ToolStripContainerDesc)] public class ToolStripContainer : ContainerControl { private ToolStripPanel topPanel; private ToolStripPanel bottomPanel; private ToolStripPanel leftPanel; private ToolStripPanel rightPanel; private ToolStripContentPanel contentPanel; public ToolStripContainer() { SetStyle(ControlStyles.ResizeRedraw | ControlStyles.SupportsTransparentBackColor, true); SuspendLayout(); try { // undone - smart demand creation topPanel = new ToolStripPanel(this); bottomPanel = new ToolStripPanel(this); leftPanel = new ToolStripPanel(this); rightPanel = new ToolStripPanel(this); contentPanel = new ToolStripContentPanel(); contentPanel.Dock = DockStyle.Fill; topPanel.Dock = DockStyle.Top; bottomPanel.Dock = DockStyle.Bottom; rightPanel.Dock = DockStyle.Right; leftPanel.Dock = DockStyle.Left; ToolStripContainerTypedControlCollection controlCollection = this.Controls as ToolStripContainerTypedControlCollection; if (controlCollection != null) { controlCollection.AddInternal(contentPanel); controlCollection.AddInternal(leftPanel); controlCollection.AddInternal(rightPanel); controlCollection.AddInternal(topPanel); controlCollection.AddInternal(bottomPanel); } // else consider throw new exception } finally { ResumeLayout(true); } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override bool AutoScroll { get { return base.AutoScroll; } set { base.AutoScroll = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Size AutoScrollMargin { get { return base.AutoScrollMargin; } set { base.AutoScrollMargin = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Size AutoScrollMinSize { get { return base.AutoScrollMinSize; } set { base.AutoScrollMinSize = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Color BackColor { get { return base.BackColor; } set { base.BackColor = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new event EventHandler BackColorChanged { add { base.BackColorChanged += value; } remove { base.BackColorChanged -= value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Image BackgroundImage { get { return base.BackgroundImage; } set { base.BackgroundImage = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new event EventHandler BackgroundImageChanged { add { base.BackgroundImageChanged += value; } remove { base.BackgroundImageChanged -= value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override ImageLayout BackgroundImageLayout { get { return base.BackgroundImageLayout; } set { base.BackgroundImageLayout = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new event EventHandler BackgroundImageLayoutChanged { add { base.BackgroundImageLayoutChanged += value; } remove { base.BackgroundImageLayoutChanged += value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerBottomToolStripPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripPanel BottomToolStripPanel { get { return bottomPanel; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerBottomToolStripPanelVisibleDescr), DefaultValue(true) ] public bool BottomToolStripPanelVisible { get { return BottomToolStripPanel.Visible; } set { BottomToolStripPanel.Visible = value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerContentPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripContentPanel ContentPanel { get { return contentPanel; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new bool CausesValidation { get { return base.CausesValidation; } set { base.CausesValidation = value; } } [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event EventHandler CausesValidationChanged { add { base.CausesValidationChanged += value; } remove { base.CausesValidationChanged -= value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new ContextMenuStrip ContextMenuStrip { get { return base.ContextMenuStrip; } set { base.ContextMenuStrip = value; } } [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event EventHandler ContextMenuStripChanged { add { base.ContextMenuStripChanged += value; } remove { base.ContextMenuStripChanged -= value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override Cursor Cursor { get { return base.Cursor; } set { base.Cursor = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new event EventHandler CursorChanged { add { base.CursorChanged += value; } remove { base.CursorChanged -= value; } } protected override Size DefaultSize { get { return new Size(150, 175); } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new Color ForeColor { get { return base.ForeColor; } set { base.ForeColor = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new event EventHandler ForeColorChanged { add { base.ForeColorChanged += value; } remove { base.ForeColorChanged -= value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerLeftToolStripPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripPanel LeftToolStripPanel { get { return leftPanel; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerLeftToolStripPanelVisibleDescr), DefaultValue(true) ] public bool LeftToolStripPanelVisible { get { return LeftToolStripPanel.Visible; } set { LeftToolStripPanel.Visible = value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerRightToolStripPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripPanel RightToolStripPanel { get { return rightPanel; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerRightToolStripPanelVisibleDescr), DefaultValue(true) ] public bool RightToolStripPanelVisible { get { return RightToolStripPanel.Visible; } set { RightToolStripPanel.Visible = value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerTopToolStripPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripPanel TopToolStripPanel { get { return topPanel; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerTopToolStripPanelVisibleDescr), DefaultValue(true) ] public bool TopToolStripPanelVisible { get { return TopToolStripPanel.Visible; } set { TopToolStripPanel.Visible = value; } } ////// /// Controls Collection... /// This is overriden so that the Controls.Add ( ) is not Code Gened... /// [EditorBrowsable(EditorBrowsableState.Never)] [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public new Control.ControlCollection Controls { get { return base.Controls; } } [EditorBrowsable(EditorBrowsableState.Advanced)] protected override Control.ControlCollection CreateControlsInstance() { return new ToolStripContainerTypedControlCollection(this, /*isReadOnly*/true); } protected override void OnRightToLeftChanged(EventArgs e) { base.OnRightToLeftChanged(e); RightToLeft rightToLeft = this.RightToLeft; // no need to suspend layout - we're already in a layout transaction. if (rightToLeft == RightToLeft.Yes) { RightToolStripPanel.Dock = DockStyle.Left; LeftToolStripPanel.Dock = DockStyle.Right; } else { RightToolStripPanel.Dock = DockStyle.Right; LeftToolStripPanel.Dock = DockStyle.Left; } } protected override void OnSizeChanged(EventArgs e) { foreach (Control c in this.Controls) { c.SuspendLayout(); } base.OnSizeChanged(e); foreach (Control c in this.Controls) { c.ResumeLayout(); } } internal override void RecreateHandleCore() { //If ToolStripContainer's Handle is getting created demand create the childControl handle's // Refer vsw : 517556 for more details. if (IsHandleCreated) { foreach(Control c in Controls) { c.CreateControl(true); } } base.RecreateHandleCore(); } internal class ToolStripContainerTypedControlCollection : WindowsFormsUtils.ReadOnlyControlCollection { ToolStripContainer owner; Type contentPanelType = typeof(ToolStripContentPanel); Type panelType = typeof(ToolStripPanel); public ToolStripContainerTypedControlCollection(Control c, bool isReadOnly) : base(c, isReadOnly) { this.owner = c as ToolStripContainer; } public override void Add(Control value) { if (value == null) { throw new ArgumentNullException("value"); } if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripContainerUseContentPanel)); } Type controlType = value.GetType(); if (!contentPanelType.IsAssignableFrom(controlType) && !panelType.IsAssignableFrom(controlType)) { throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, SR.GetString(SR.TypedControlCollectionShouldBeOfTypes, contentPanelType.Name, panelType.Name)), value.GetType().Name); } base.Add(value); } public override void Remove(Control value) { if (value is ToolStripPanel || value is ToolStripContentPanel) { if (!owner.DesignMode) { if (IsReadOnly) throw new NotSupportedException(SR.GetString(SR.ReadonlyControlsCollection)); } } base.Remove(value); } internal override void SetChildIndexInternal(Control child, int newIndex) { if (child is ToolStripPanel || child is ToolStripContentPanel) { if (!owner.DesignMode) { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ReadonlyControlsCollection)); } } else { // just no-op it at DT. return; } } base.SetChildIndexInternal(child, newIndex); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /// this is the UBER container for ToolStripPanels. namespace System.Windows.Forms { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; using System.Windows.Forms; using System.Collections; using System.Globalization; using System.Windows.Forms.Layout; [ComVisible(true)] [ClassInterface(ClassInterfaceType.AutoDispatch)] [Designer("System.Windows.Forms.Design.ToolStripContainerDesigner, " + AssemblyRef.SystemDesign)] [SRDescription(SR.ToolStripContainerDesc)] public class ToolStripContainer : ContainerControl { private ToolStripPanel topPanel; private ToolStripPanel bottomPanel; private ToolStripPanel leftPanel; private ToolStripPanel rightPanel; private ToolStripContentPanel contentPanel; public ToolStripContainer() { SetStyle(ControlStyles.ResizeRedraw | ControlStyles.SupportsTransparentBackColor, true); SuspendLayout(); try { // undone - smart demand creation topPanel = new ToolStripPanel(this); bottomPanel = new ToolStripPanel(this); leftPanel = new ToolStripPanel(this); rightPanel = new ToolStripPanel(this); contentPanel = new ToolStripContentPanel(); contentPanel.Dock = DockStyle.Fill; topPanel.Dock = DockStyle.Top; bottomPanel.Dock = DockStyle.Bottom; rightPanel.Dock = DockStyle.Right; leftPanel.Dock = DockStyle.Left; ToolStripContainerTypedControlCollection controlCollection = this.Controls as ToolStripContainerTypedControlCollection; if (controlCollection != null) { controlCollection.AddInternal(contentPanel); controlCollection.AddInternal(leftPanel); controlCollection.AddInternal(rightPanel); controlCollection.AddInternal(topPanel); controlCollection.AddInternal(bottomPanel); } // else consider throw new exception } finally { ResumeLayout(true); } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override bool AutoScroll { get { return base.AutoScroll; } set { base.AutoScroll = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Size AutoScrollMargin { get { return base.AutoScrollMargin; } set { base.AutoScrollMargin = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Size AutoScrollMinSize { get { return base.AutoScrollMinSize; } set { base.AutoScrollMinSize = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Color BackColor { get { return base.BackColor; } set { base.BackColor = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new event EventHandler BackColorChanged { add { base.BackColorChanged += value; } remove { base.BackColorChanged -= value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Image BackgroundImage { get { return base.BackgroundImage; } set { base.BackgroundImage = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new event EventHandler BackgroundImageChanged { add { base.BackgroundImageChanged += value; } remove { base.BackgroundImageChanged -= value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override ImageLayout BackgroundImageLayout { get { return base.BackgroundImageLayout; } set { base.BackgroundImageLayout = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new event EventHandler BackgroundImageLayoutChanged { add { base.BackgroundImageLayoutChanged += value; } remove { base.BackgroundImageLayoutChanged += value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerBottomToolStripPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripPanel BottomToolStripPanel { get { return bottomPanel; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerBottomToolStripPanelVisibleDescr), DefaultValue(true) ] public bool BottomToolStripPanelVisible { get { return BottomToolStripPanel.Visible; } set { BottomToolStripPanel.Visible = value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerContentPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripContentPanel ContentPanel { get { return contentPanel; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new bool CausesValidation { get { return base.CausesValidation; } set { base.CausesValidation = value; } } [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event EventHandler CausesValidationChanged { add { base.CausesValidationChanged += value; } remove { base.CausesValidationChanged -= value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new ContextMenuStrip ContextMenuStrip { get { return base.ContextMenuStrip; } set { base.ContextMenuStrip = value; } } [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event EventHandler ContextMenuStripChanged { add { base.ContextMenuStripChanged += value; } remove { base.ContextMenuStripChanged -= value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override Cursor Cursor { get { return base.Cursor; } set { base.Cursor = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new event EventHandler CursorChanged { add { base.CursorChanged += value; } remove { base.CursorChanged -= value; } } protected override Size DefaultSize { get { return new Size(150, 175); } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new Color ForeColor { get { return base.ForeColor; } set { base.ForeColor = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public new event EventHandler ForeColorChanged { add { base.ForeColorChanged += value; } remove { base.ForeColorChanged -= value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerLeftToolStripPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripPanel LeftToolStripPanel { get { return leftPanel; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerLeftToolStripPanelVisibleDescr), DefaultValue(true) ] public bool LeftToolStripPanelVisible { get { return LeftToolStripPanel.Visible; } set { LeftToolStripPanel.Visible = value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerRightToolStripPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripPanel RightToolStripPanel { get { return rightPanel; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerRightToolStripPanelVisibleDescr), DefaultValue(true) ] public bool RightToolStripPanelVisible { get { return RightToolStripPanel.Visible; } set { RightToolStripPanel.Visible = value; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerTopToolStripPanelDescr), Localizable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public ToolStripPanel TopToolStripPanel { get { return topPanel; } } [ SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripContainerTopToolStripPanelVisibleDescr), DefaultValue(true) ] public bool TopToolStripPanelVisible { get { return TopToolStripPanel.Visible; } set { TopToolStripPanel.Visible = value; } } ////// /// Controls Collection... /// This is overriden so that the Controls.Add ( ) is not Code Gened... /// [EditorBrowsable(EditorBrowsableState.Never)] [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public new Control.ControlCollection Controls { get { return base.Controls; } } [EditorBrowsable(EditorBrowsableState.Advanced)] protected override Control.ControlCollection CreateControlsInstance() { return new ToolStripContainerTypedControlCollection(this, /*isReadOnly*/true); } protected override void OnRightToLeftChanged(EventArgs e) { base.OnRightToLeftChanged(e); RightToLeft rightToLeft = this.RightToLeft; // no need to suspend layout - we're already in a layout transaction. if (rightToLeft == RightToLeft.Yes) { RightToolStripPanel.Dock = DockStyle.Left; LeftToolStripPanel.Dock = DockStyle.Right; } else { RightToolStripPanel.Dock = DockStyle.Right; LeftToolStripPanel.Dock = DockStyle.Left; } } protected override void OnSizeChanged(EventArgs e) { foreach (Control c in this.Controls) { c.SuspendLayout(); } base.OnSizeChanged(e); foreach (Control c in this.Controls) { c.ResumeLayout(); } } internal override void RecreateHandleCore() { //If ToolStripContainer's Handle is getting created demand create the childControl handle's // Refer vsw : 517556 for more details. if (IsHandleCreated) { foreach(Control c in Controls) { c.CreateControl(true); } } base.RecreateHandleCore(); } internal class ToolStripContainerTypedControlCollection : WindowsFormsUtils.ReadOnlyControlCollection { ToolStripContainer owner; Type contentPanelType = typeof(ToolStripContentPanel); Type panelType = typeof(ToolStripPanel); public ToolStripContainerTypedControlCollection(Control c, bool isReadOnly) : base(c, isReadOnly) { this.owner = c as ToolStripContainer; } public override void Add(Control value) { if (value == null) { throw new ArgumentNullException("value"); } if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripContainerUseContentPanel)); } Type controlType = value.GetType(); if (!contentPanelType.IsAssignableFrom(controlType) && !panelType.IsAssignableFrom(controlType)) { throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, SR.GetString(SR.TypedControlCollectionShouldBeOfTypes, contentPanelType.Name, panelType.Name)), value.GetType().Name); } base.Add(value); } public override void Remove(Control value) { if (value is ToolStripPanel || value is ToolStripContentPanel) { if (!owner.DesignMode) { if (IsReadOnly) throw new NotSupportedException(SR.GetString(SR.ReadonlyControlsCollection)); } } base.Remove(value); } internal override void SetChildIndexInternal(Control child, int newIndex) { if (child is ToolStripPanel || child is ToolStripContentPanel) { if (!owner.DesignMode) { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ReadonlyControlsCollection)); } } else { // just no-op it at DT. return; } } base.SetChildIndexInternal(child, newIndex); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
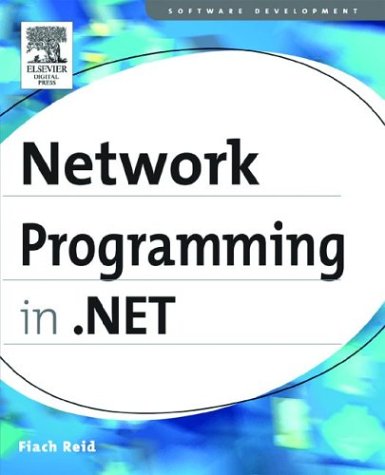
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SortQuery.cs
- SmiTypedGetterSetter.cs
- DataTableClearEvent.cs
- FormDesigner.cs
- TypedDataSetSchemaImporterExtensionFx35.cs
- TargetFrameworkUtil.cs
- QilExpression.cs
- ItemPager.cs
- FlowDocumentReaderAutomationPeer.cs
- selecteditemcollection.cs
- QueryProcessor.cs
- ServiceXNameTypeConverter.cs
- Drawing.cs
- TabletDeviceInfo.cs
- MappingModelBuildProvider.cs
- DataSourceCacheDurationConverter.cs
- _ConnectStream.cs
- WindowsHyperlink.cs
- CopyNamespacesAction.cs
- PartialArray.cs
- unsafenativemethodsother.cs
- EntityDataSourceMemberPath.cs
- AttachedPropertyMethodSelector.cs
- SafeNativeMethods.cs
- WindowsRegion.cs
- ParameterToken.cs
- TextRangeAdaptor.cs
- MapPathBasedVirtualPathProvider.cs
- EncodingTable.cs
- HttpCookieCollection.cs
- ScaleTransform3D.cs
- LogRecordSequence.cs
- WindowsSpinner.cs
- DeleteHelper.cs
- PrintControllerWithStatusDialog.cs
- BitmapEffectDrawingContextWalker.cs
- SafeViewOfFileHandle.cs
- CollaborationHelperFunctions.cs
- CodeDefaultValueExpression.cs
- XmlObjectSerializerContext.cs
- APCustomTypeDescriptor.cs
- ByteArrayHelperWithString.cs
- DecoderFallback.cs
- XmlSchemaElement.cs
- EntityContainerEntitySetDefiningQuery.cs
- NamedPermissionSet.cs
- NullToBooleanConverter.cs
- DBNull.cs
- XmlWhitespace.cs
- StorageMappingFragment.cs
- StorageComplexPropertyMapping.cs
- ExtensionQuery.cs
- OutputCacheProfile.cs
- XmlUrlEditor.cs
- WsdlBuildProvider.cs
- ArgumentNullException.cs
- HttpInputStream.cs
- Literal.cs
- InheritedPropertyChangedEventArgs.cs
- RandomNumberGenerator.cs
- CompositeActivityTypeDescriptorProvider.cs
- BulletedList.cs
- AppDomainAttributes.cs
- FrameworkElementFactoryMarkupObject.cs
- SpellerInterop.cs
- QilIterator.cs
- EventPrivateKey.cs
- CacheChildrenQuery.cs
- path.cs
- RtfToXamlReader.cs
- StructuredType.cs
- DataGridViewControlCollection.cs
- FileInfo.cs
- StatusBarPanel.cs
- CodePropertyReferenceExpression.cs
- SafeNativeMethodsCLR.cs
- CacheAxisQuery.cs
- TimeStampChecker.cs
- FormViewUpdateEventArgs.cs
- CompilationUnit.cs
- SelectionItemProviderWrapper.cs
- WindowsSlider.cs
- HitTestWithGeometryDrawingContextWalker.cs
- ImageEditor.cs
- Visual3D.cs
- PropertyMetadata.cs
- BindingCompleteEventArgs.cs
- JavaScriptString.cs
- GeneralTransform3DTo2D.cs
- DesignerWithHeader.cs
- WeakEventManager.cs
- ModuleBuilderData.cs
- DynamicVirtualDiscoSearcher.cs
- DefaultAuthorizationContext.cs
- SqlFunctionAttribute.cs
- Bidi.cs
- Overlapped.cs
- CodeAccessPermission.cs
- DesignerDataTableBase.cs
- RegularExpressionValidator.cs